SEC S20W03 || Basic Programming Course: Lesson #3 Operations
This is my homework post for Steemit Engagement Challenge Season 20 Week 3 assignment of Professor @alejos7ven’s class, Basic Programming Course: Lesson #3 Operations.
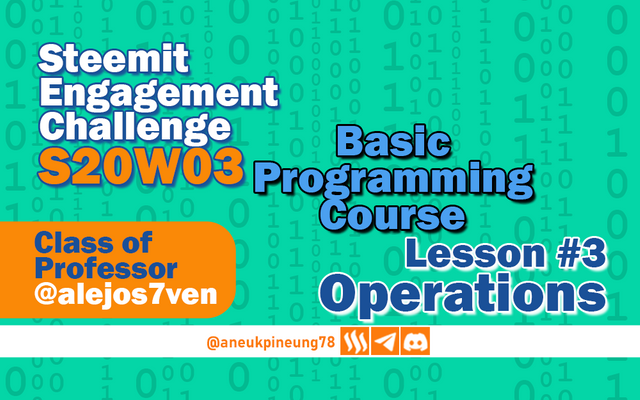
Note :
- I performed this task on Windows 10 PC, Google Chrome.
Task 1 - Give a brief summary of what arithmetic, comparison and logical operations are for.
Arithmetic Operation
Arithmetic - in terms of terms - is arguably the most fundamental branch in mathematics. Arithmetic covers all the basic operations on numbers, so it would not be wrong to say that it is the foundation of all mathematical operations. These operations are: 1) addition; 2) subtraction; 3) multiplication; 4) division, and 5) advanced operations (e.g. scaling, modulus, and floor).
It can be said that it is impossible for a human being to escape arithmetic in his life. Examples of human actions that fall into the category of arithmetic are: calculating the number of hours worked, multiplying the number of hours worked into the total salary to be paid, calculating the remaining debt according to the initial amount of debt and the amount that has been paid and the interest charged, organizing work schedules (for example allocating the use of daily time to achieve targets at the end of the week).
Likewise, in programming, arithmetic is used for the same purpose, which is to perform basic math operations. The user will enter numbers and the program will perform the calculations. This was briefly discussed in week two's lesson when we calculated the price of a certain amount of STEEM in USD. At that time we used the multiplication function of arithmetic.
Below is an example of using arithmetic in programming, covering almost all simple arithmetic operations. In this example I get creative by going a little different with the example given by Professor Alejos7ven in his lectur:
- I change the variables
n
andn2
withx
andy
, respectively; - I change some display messages;
- in his lecture, Professor defined the values of the numbers by default in the algorithm, then in this example I let the users specify the numbers they want;
- I add exponential operation in this example;
- I put all these 2-variable arithmetic operations into one algorithm, so that for any two numbers entered, there will be results for 6 arithmetic operations all togehter.
Algorithm Arithmetic_Ops
// 1 - Declare variables;
Define x, y, addition, subtraction, multiplication, division, module As Real;
// 2 – Ask user for input values
Print "Enter the value of x: "
Read x
Print "Enter the value of y: "
Read y
// 3 – Addition
addition = x + y;
Print "The result of “ x “ + “ y “ is " addition;
// 2 - Subtraction
subtraction = x - y;
Print "The result of “ x “ - “ y “ is " subtraction;
// 3 - Multiplication
multiplication = x * y;
Print "The result of “ x “ * “ y “ is " multiplication;
// 4 - Division
division = x / y;
If y <> 0 Then
Priint "The result of “ x “ / “ y “ is " division;
Else
Print "Division by zero is not allowed."
EndIf
// 5 - Modulus (remainder)
module = x % y;
Print " The remainder of dividing “ x “ with “ y “ is " module;
// 6 - Exponentiation
exponent = x ^ y;
Print "The result of “ x “ raised to the power of “ y “ is “ exponent;
EndAlgorithm
Through my internet search I found that, in line with the law of arithmetic that numbers are not divisible by 0, it is emphasized in the algorithm by inserting the function
If y <> 0 Then
Print "The result of “ x “ / “ y “ is " division;
Else
Print “Division by zero is not allowed.”
EndIf
This function serves as a command for the program to check whether the divisor (y) has a value equal to 0, if the divisor (y) does not have a value equal to 0, then the division is performed and the result is displayed, if the divisor (y) is 0 then the message Division by zero is not allowed.
is displayed. The command to end If
command is EndIf
.
When a user inputs data x
is 78
and y
is 4
, then the program will display result as follow:
The result of 78 + 4 is 82;
The result of 78 - 4 is 74;
The result of 78 * 4 is 312;
The result of 78 / 4 is 19.5;
The remainder of dividing 74 with 4 is 2;
The result of 74 raised to the power of 4 is 37,015,056;
Comparison Operation
As the name implies, the Comparison Operation is an operation that compares two values and returns a boolean result (TRUE
or FALSE
). This operation is often used in conditioning, repetition and logic. Professor Alejos7ven has talked about this quite a bit in his lectures, but I'll go back and note some of the commonly used types of comparison operations, which are:
- Equal to (
==
), this operation checks if two numbers have the same value. For examplex
is78
andy
is78
, thenx == y
will returnTRUE
. But ify
is47
, then the result of this operation isFALSE
. - Different from (
!=
), this operation checks whether two variables have different values, if they are, then the result isTRUE
, andFALSE
will be the result if the values of the two variables being compared are the same. - Greater than (
>
), this operation checks whether the value to the left of the>
sign is greater than the value to the right of it. Example: the operation78 > 47
will result inTRUE
. - Less than (
<
), this operation is the opposite of thegreater than
operation,TRUE
will result if the number to the left of the<
sign is smaller than the value to its right. For example:78 < 47
will result inFALSE
while47 < 78
will result inTRUE
. - Greater or equal to (
>=
) will return the resultTRUE
when the value to the left of the>=
sign is greater than or equal to the value to the right of it. Example:78 >= 47
will returnTRUE
in result, while47 >= 78
will returnFALSE
in result. - Smaller or equal to (
<=
), works the opposite of the above comparison operation. It will return aTRUE
result when the value to the left of the<=
sign is smaller or equal to the value to the right of it. The example47 <= 78
will return the resultTRUE
, as will47 <= 47
, but the resultFALSE
will be returned to87 <= 47
.
In a scenario where the values of x
, y
, and z
are 47
, 78
, and 47
respectively, the algorithm for the comparison operation for the data is :
Algorithm Comparision_Operation
// 1 - Declare variables;
Define x, y, z As Integer;
x=47;
y=78;
z=47;
// 2 – Equal to
Print x “is equal to “ y “ ? ” x==y;
Print x “is equal to “ z “ ? ” x==z;
// 3 – Different from
Print x “is different from“ y “ ? ” x!=y;
Print x “is different from“ z “ ? ” x!=z;
// 4 – Greater than
Print x “is greater than“ y “ ? ” x>y;
Print x “is greater than“ z “ ? ” x>z;
// 5 – Less than
Print x “is less than“ y “ ? ” x<y;
Print x “is less than“ z “ ? ” x<z;
// 6 – Greater or equal to
Print x “is greater or equal to “ y “ ? ” x>=y;
Print x “is greater or equal to “ z “ ? ” x>=z;
// 7 – Smaller or equal to
Print x “is smaller or equal to “ y “ ? ” x<=y;
Print x “is smaller or equal to “ z “ ? ” x<=z;
EndAlgorithm
Yes, I realize that in the lecture, Professor has chosen the data type Real
for all the data in the comparison operation example, but here I have chosen the data type Integer
, hopefully Professor will make a special note of this, as he did in grading the previous weeks' work.
If the above algorithm is executed, then the result is:
47 is equal to 78? FALSE
47 is equal to 47? TRUE
47 is different from 78? TRUE
47 is different from 47? FALSE
47 is greater than 78? FALSE
47 is greater than 47? FALSE
47 is less than 78? TRUE
47 is less than 47? FALSE
47 is greater or equal to 78? FALSE
47 is greater or equal to 47? TRUE
47 is smaller or equal to 78? TRUE
47 is smaller or equal to 47? TRUE
Logical Operation
According to what I read from Professor's lecture, coupled with my findings on the internet, I concluded that logical operations are operations used to evaluate 2 comparative operations or boolean statements or expressions (expressions that produce a value of TRUE
or FALSE
), logical operations will produce a value of TRUE
or FALSE
based on the evaluation. Logical operations use logical operators, namely:
AND
(&&
orand
). In this operation, if both statements areTRUE
then the result returned isTRUE
, if any or all statements's value isFALSE
, then this operation will return the resultFALSE
.OR
(||
oror
). This operator requires one of the statements to beTRUE
for the operation to return aTRUE
result. If all statements areFALSE
, then the operation will return aFALSE
result.- There are some other logical operators like
NOT
andXOR
.
Logical operations are used as one of the guidelines for decision-making. Case in point: to play level 78 in a game, there are two variables that are determining factors: 1) level 47 completion; 2) gamer’s sexuality (gender). With these two variables, then:
AND
logical operation will require both statements to have the valueTRUE
so that the logical operation will return the valueTRUE
, meaning that a gamer can only play level 78 (TRUE
) if he is a male (TRUE
) and has completed level 47 (TRUE
). If one or both of the statements’ value is (are)FALSE
, for example a gamer is a woman and/or has not completed level 47, then the logical operation will return the valueFALSE
(they cannot play level 78).- The logical operation
OR
requires at least one of the statements to have the valueTRUE
for the logical operation to return the valueTRUE
, meaning that if a gamer has completed level 47 then they can play level 78 (regardless of his gender) or if he is a male gamer then he can play level 78 without having to complete level 47 first. Only in the case where both statements areFALSE
(a gamer is not male and has not completed level 47) will the logical operationOR
return the valueFALSE
(she cannot play level 78).
An example program to check the values of the two operations AND
and OR
based on the sample data implemented into a pseudo-code algorithm is as follows:
Algorithm PlayLevel78
// 1 - Declare variables;
Define gender As Character;
Define level47Completed As Character;
Define is_male, is_completed As Logical;
// 2 - Ask for input from the user;
Print "Enter your gender (male, female): "
Read gender;
Print "Have you completed level 47? (yes/no): ";
Read level47Completed;
is_male = gender==male;
is_completed = level44Completed==yes;
// 3 - AND
Print "Does the user meet both conditions? " is_male and is_completed;
// 4 - OR
Print "Does the user meet at least one condition? " is_male or is_completed;
EndAlgorithm
When a user fills in the gender data male
and no
in the level47Completed variable, the program will display the following message:
Enter your gender (male, female):
> male
Have you completed level 47?:
> no
Does the user meet both conditions? FALSE
Does the user meet at least one condition? TRUE
If the data entered is female
and no
, then:
Enter your gender (male, female):
> female
Have you completed level 47?:
> no
Does the user meet both conditions? FALSE
Does the user meet at least one condition? FALSE

Task 2 - Make a program that asks the user for 2 numbers and evaluates if both numbers are the same.
Algorithm Check_numbers_equality
// 1 - Declare variables;
Define x, y As Integer;
// 2 - Ask user to fill in the numbers;
Print "Enter a number: ";
Read x;
Print "Enter another number: ";
Read y;
Print "Is " x " equal to " y "? " x==y;
EndAlgorithm
If a user enters the numbers 47
and 78
, the application will look like this:
Enter a number:
> 47
Enter another number:
> 78
Is 47 equals to 78? FALSE
When the user enters the numbers 78
and 78
, then:
Enter a number:
> 78
Enter another number:
> 78
Is 78 equals to 78? TRUE

Task 3 - Transform the following mathematical expressions into arithmetic expressions on your computer. Evaluate if the results of the 3 operations are greater than or equal to 0 (>=) and show it on the screen.
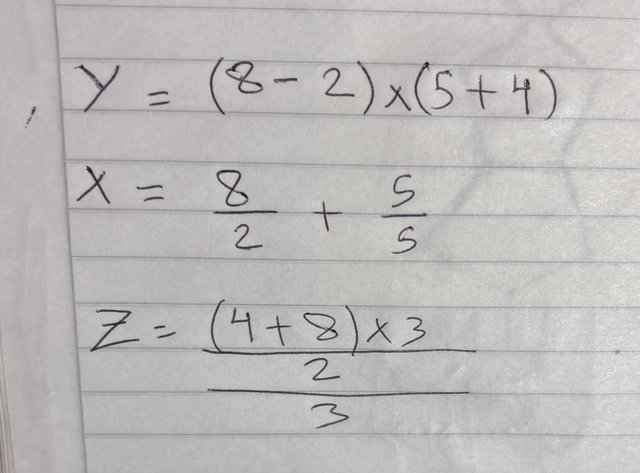
In arithmetic form, the mathematical equation in the figure above becomes like this:
y = (8-2)*(5+4)
x = (8/2) + (5/5)
z = (((4+8)*3)/2)/3
When adapted into a program algorithm, pseudo-code can have this anatomy:
Algorithm Calculate_and_Evaluate
// 1 - Declare variables;
Define y, x, z As Real;
// 2 – The calculation;
Print “Calculate y: ”
Read y;
Print “Calculate x: ”
Read x;
Print “Calculate z: ”
Read z;
// 3 - The results;
Print "The value of y is: ", y;
Print "The value of x is: ", x;
Print "The value of z is: ", z;
// 4 – Evaluation
Print "Is " y " greater than or equal to 0? " y>=0;
Print "Is " x " greater than or equal to 0? " x>=0;
Print "Is " z " greater than or equal to 0? " z>=0;
EndAlgorithm
The result that will be achieved on the program interface when executed is:
Calculate y:
> y = (8-2)*(5+4)
Calculate x:
> x = (8/2) + (5/5)
Calculate z:
> z = (((4+8)*3)/2)/3
The value of y is: 54
The value of x is: 5
The value of z is: 6
Is 54 greater than or equal to 0? TRUE
Is 5 greater than or equal to 0? TRUE
Is 6 greater than or equal to 0? TRUE

Conclusion
Math has never been my favorite subject, and after reading some literature on the internet, I now know that Math and Programming are inseparable. The position of Math is important for several reasons, including:
- Math trains logical and analytical thinking which is needed by Programming to solve problems;
- Number theories, geometry, and algebra are often used to optimize algorithms for searching, sorting, or analyzing data;
- Mathematical equations that are capable of performing calculations to determine the position, scale, rotation of objects in space, and so on, are what support rendering in 3-dimensional programs.
- Statistics, calculus, and linear algebra are the most important foundations in the development of Artificial Intelligence and Machine Learning.
- Computer system security takes the form of cryptography on number theory and other mathematical concepts.

Thanks
Even though Week 3 was starting to feel like the basic programming theory lessons were starting to “sting”, I found myself getting more and more interested. Programming turns out to require strong logic and analytical skills. And, there is Math. I started to think that maybe in 3 weeks (season 20 ends), I will grow some love for Math. It really has been an adventure of sorts, and Season 20 of SEC might have been the best season I've ever participated in. Thank you Professor Alejos7ven.

Sources and Reading Suggestion
- https://photomath.com/articles/basic-arithmetic-operations-the-four-fundamental-operators/
- https://byjus.com/maths/arithmetic-operations/
- https://www.ibm.com/docs/en/epfz/5.3?topic=expressions-arithmetic-operations
- https://www.cuemath.com/numbers/arithmetic-operations/
- https://www.microfocus.com/documentation/silk-test/205/en/silktestclassic-help-en/STCLASSIC-F02D493B-ARITHMETICOPERATORS-REF.html
- https://help.sap.com/doc/saphelp_ewm900/9.0/en-US/50/9eecf3152c4fb08d50e94faaf4a277/content.htm
- https://www.codecademy.com/article/fwd-js-comparison-logical
- https://www.ibm.com/docs/en/cics-ts/6.x?topic=expressions-comparison-operators
- https://www.w3schools.com/js/js_comparisons.asp
- https://www.ibm.com/docs/en/epfz/5.3?topic=expressions-comparison-operations
- https://press.rebus.community/programmingfundamentals/chapter/logical-operators/
- https://www.whitman.edu/mathematics/higher_math_online/section01.01.html
- https://www.w3schools.com/cpp/cpp_operators_logical.asp
- https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/boolean-logical-operators
- https://www.jstor.org/stable/30226590
- https://javascript.info/logical-operators
- https://www.ibm.com/docs/en/zdsfcp/2.1.0?topic=operators-logical-operations
- https://en.wikipedia.org/wiki/Boolean_algebra
- https://dev.to/codewithshahan/10-math-concepts-for-every-programmer-31n9
- https://www.kdnuggets.com/10-math-concepts-for-programmers
- https://link.springer.com/journal/10107
- https://medium.com/@sipocodes/the-role-of-math-in-computer-programming-an-in-depth-analysis-ea5d0c0fdff4
- https://nostarch.com/math-programming
- https://analyticsindiamag.com/tech-ai-blend/10-must-know-math-concepts-for-programmers/


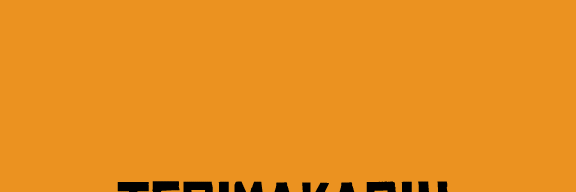

https://x.com/aneukpineung78/status/1839704259413393556
Belajar... Belajar... Belajar....
Iya, ayok ikutan belajar, Bang @lord-geraldi. Biarkan RSUD itu dengan kelakuannya, sudah nasib kita Bansa Teuleubeh Ateuh Rueng Donya. Hahah.
Click Here
Thank you.
Congratulations! Your post has been upvoted through steemcurator06.
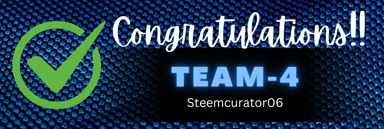
Good post here should be . . .
Curated by : @𝗁𝖾𝗋𝗂𝖺𝖽𝗂
Terimakasih banyak. Saya sangat menghargai apa yang Anda kerjakan.