How to enter the game industry (Part 10: Animation ) [tutorial]
part 1: your skills
part 2: Choosing an Engine
part 3: Ren'py Game Engine
part 4: Ren'py Scripting
part 5: Ren'py More Scripting
part 6: Ren'py Scripting, Visuals
part 7: Ren'py Scripting, Dialogue
Part 8: Ren'py Scripting, Conclusion
<< Part 9: images
As promised this part is about animation in Ren'py, but we will look into "computer animation" as the base knowledge you will need for any type of animation in any software and not only Ren'py.
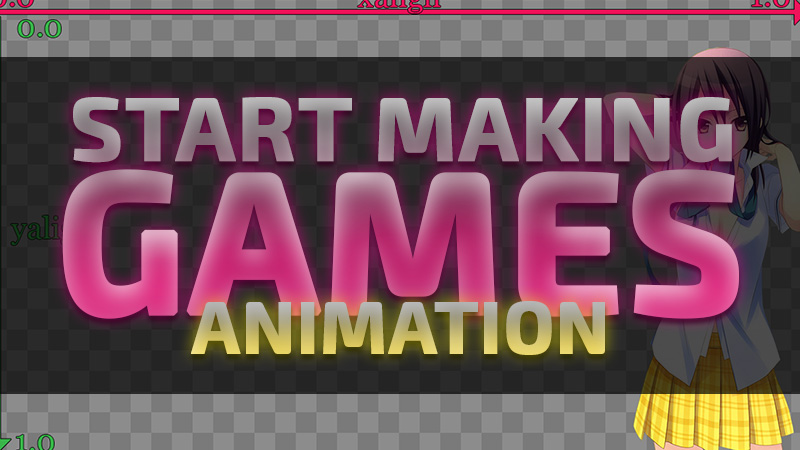
There's mainly two type of animation, frame by frame and "computer animation", while all animations are shown frame by frame the difference is mainly between drawn animation that is drawn frame by frame versus move,rotate,resize and skew animation that is the main ingredient of any animation software.
In Ren'py we can use both type of animation, we will need the same images as the previous part and few modified ones, Being lazy myself I know I'll have to provide the needed images and not ask you to go through the previous part for them, therefore, I'll provide the images: bg.jpg || c1_nrm.png || c1_blnk.png
Put them in img
folder.
Frame by frame:
Let's say we want our characters to blink, we need at least two images, one with open eyes and one with closed eyes. put both c1_nrm.png
and c1_blnk.png
into game/img
folder and open your script.rpy
we can define animations the same way we define images:
image blink:
"img/c1 nrm.png"
pause 0.2
"img/c1 blnk.png"
pause 0.2
repeat
label start:
show blink
pause
return
Don't worry I'll explain:
line 1
Here instead of =
we have a :
creating an indented block under it.
line 2
the image for the first frame of our animation. (0.2 in seconds)
line 3
the pause before the second frame is shown.
line 4
the second frames image
line 5
the delay before the next frame
line 6
tells the engine to repeat the whole thing
and the rest is explained before
we need to fix the constant blinking, let's simply increase the first pause
to 10
, much better right? however, blinking is random, making the characters to blink at truly random intervals is a bit complicated so let me give you the next best thing:
image blink:
"img/c1 nrm.png"
pause renpy.random.randint(5,10)
"img/c1 blnk.png"
pause 0.2
repeat
label start:
show blink
pause
return
This taps into Ren'pys random and makes sure every time your character is loaded she will be assigned a random number between five to ten seconds to blink at. you can change the numbers to the numbers you want.
to define an image with more frames you can simply add lines of images and pauses in your image definition:
image blink:
"frame1.png"
pause .1
"frame2.png"
pause 0.2
"frame3.png"
pause .1
"frame4.png"
pause 0.2
"frame5.png"
pause .1
"frame6.png"
pause 0.2
repeat
label start:
show blink
pause
return
and as you can guess removing the repeat
line will cause the animation play one time and stay on the last frame. to repeat an animation a specific number of loops you can simply add a number in front of repeat.
image blink:
"img/c1 nrm.png"
pause 3
"img/c1 blnk.png"
pause 0.2
repeat 3
label start:
show blink
pause
return
and that's all about frame by frame animation, there's not much to it, just showing images one after another.
Computer animation
In Ren'py we have access to usual computer animation actions move
, rotate
, resize
, alpha
but not skew
. Even though we will miss some interesting perspective animation without skew
, there's a ton of things we can do with the rest, so let's jump to it.
Move
image aika:
"img/c1 nrm.png"
xalign 0.0
linear 2.0 xalign 1.0
label start:
show aika
pause
return
Like always here's line by line explanation:
line 1
we defined an image (animated) named aika
line 2
our images address
line 3
we placed the image at the far left end of screen (0.0 on x axis)
line4
we created a linear
movement for 2.0
seconds from where the image is to the far right of screen (1.0 on x axis)
and the rest is the usual stuff. in case you've forgot "xalign is the position on the x (horizontal) axis on the screen, 0.0 is far left and 1.0 is far right", the vertical position is yalign
from top to bottom.
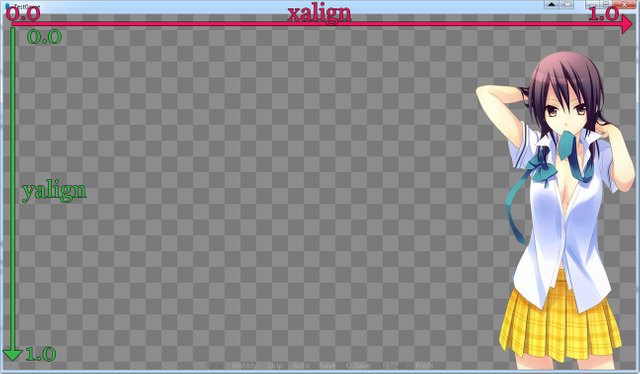
As you can see every point on the screen has two position value x position and y position. In computer animation the zero, zero position (AKA origin) is top left side of the screen. it's the same for images. speaking of position,
xalign
and yalign
are not the only way to place something on the screen, we also have xpos
and ypos
. let's do an experiment.
image aika:
"img/c1 nrm.png"
xpos 0.0
pause 0.5
linear 2.0 xpos 1.0
label start:
show aika
pause
return
as you can see xpos 0,0
will cause half of the image to go out of the screen, xpos 1.0
causes the other half to go out of the screen. the reason is xanchor
.
Every image has a point on it that acts as it's handle, when we tell the engine to move the image to xpos 0.0
the engine moves this handle to 0.0
. this handle is called anchor
and usually placed on the middle of image. to change this anchor you can use xanchor
and yanchor
,
image aika:
"img/c1 nrm.png"
xpos 0.0 xanchor 0.0
pause 0.5
linear 2.0 xpos 1.0 xanchor 1.0
label start:
show aika
pause
return
Works exactly like xalign
right? that's because xalign
is an xpos
plus xanchor
.
if you set the xalign
to 0.25
it move the xanchor
to a forth of image from left and move that anchor to a forth of the screen.
Note: as you can see in line 3
I've placed two properties on the same line, it's the same as placing each on a line, as long as you put them in correct order property
value
property
value
... you can stack them in the same line. of curse you can put each one on a line but in case of line 5
you have to put them after linear 2.0
to make them move at the same pace.
Those xpos
and ypos
look a bit useless when we have the align
version. however, they are intended for another use.
image aika:
"img/c1 nrm.png"
xpos -300
pause 0.5
linear 2.0 xpos 700
label start:
show aika
pause
return
up till now we have used a value between 0
and 1
to move images relative to the screen however we can use the number of pixels for position with xpos
and ypos
, that's how you move pictures out of the screen.
Positioning an image with align
has it's sweet benefits and often calculating a position in pixels on the screen to do a little animation can become a bit tiresome and inaccurate, let's consider this scenario:
you want your character to stay in middle of screen but keep swinging to the right and left, now you have to calculate middle of the screen, swing to right position, swing to left position all in pixels, or you can use xoffset
image aika:
"img/c1 nrm.png"
linear 1.0 xoffset -20
linear 1.0 xoffset 20
repeat
label start:
show aika at center
pause
return
As you can guess xoffset
just offsets the image from it's anchor, you can position the image with align
or pos
and it wouldn't care where it is, it just keeps doing what it's instructed to do.
align
, pos
, anchor
and offset
together give you every option you'll need to move things around (not only images)
Rotate
image aika:
"img/c1 nrm.png"
linear 1.0 rotate 360
rotate 0
repeat
label start:
show aika
pause
return
rotate
, will rotate images around their anchor, there's not much call for rotating your characters but when you get to designing your games interface, rotation will become a valuable tool.
we already covered the anchor
, to change the rotations anchor there's one extra line of code you need to add.
image aika:
"img/c1 nrm.png"
transform_anchor True
yanchor 0.8
linear 1.0 rotate 360
rotate 0
repeat
label start:
show aika
pause
return
line 3
transform_anchor True
will allow you to change the anchor which the image rotates around, however, changing the anchor will change the position of your image too. you need to experiment with it to get everything in the right position.
Resize
Another way to animate an object is changing the size:
image aika:
"img/c1 nrm.png"
linear 1.0 zoom 0.9
linear 1.0 zoom 1.0
repeat
label start:
show aika
pause
return
in case of zoom, you're not limited to a value between 0.0
and 1.0
, you can have an image twice it's size with zoom 2.0
and if you give a negative value to zoom the image will flip around it's anchor, it's really handy when you want to generate a flipped version of your character.
image aika:
"img/c1 nrm.png"
linear 0.2 xzoom -1.0
pause 1
linear 0.2 xzoom 1.0
pause 1
repeat
label start:
show aika
pause
return
unlike other properties, the default value for zoom
, xzoom
and yzoom
is 1.0
and not 0.0
Alpha
Fading is achieved by changing alpha:
image aika:
"img/c1 nrm.png"
linear 0.2 alpha 0.2
pause 1
linear 0.2 alpha 1.0
pause 1
linear 0.2 alpha 0.0
pause 1
repeat
label start:
show aika
pause
return
there's not much to it. alpha 1.0
is fully visible,alpha 0.0
is invisible and anything in between see trough.
Easing
Lets take a look at this linear
we've been using til now. linear
tells the engine that the speed to change between the previous value and the next value should be constant. there's no speeding up or down. you can replace linear with another "wrapper" for a different type of change.
the most popular options are:
ease
start slow, speed up then slow down at the end.
easein
start fast then slow down towards the end.
easeout
start slow and speed up towards the end.
let's see an example:
image aika:
"img/c1 nrm.png"
easein 1.8 xalign 0.1
pause 1
easeout 1.8 xalign 0.9
pause 1
repeat
label start:
show aika
pause
return
there's a whole bunch of different wrappers you can use in your animation on Ren'Py Documentation. try them out and choose the one you feel is better for your need.
Also on that page there's a whole lot of other stuff that I didn't include in this tutorial for the sake of simplicity, I will explain more complicated concepts when we get to animating in the interface but if you're keen to learn them faster that page is your guide.
Tip #13:
In the incident that happened while posting this post the tip about commenting got lost so I'll add it here because I'll be using it in the next tutorials.
any text after a number sign #
will be ignored by the engine while running and or compiling the game, a #
starts a comment line and you can add your comment about a line of code in your script.
# this is a comment
label start:
show aika #this shows aika
"Hi, I'm aika, nice to meet you"
# "do you come here often?"
# the line above is commented out and won't be shown in the game
return
The keyboard shortcut for comment/um-comment a line is ctrl+1
, you can select multiple lines and comment/um-comment them.
Note: on scripts with lots of lines sometimes this keyboard shortcut act buggy.
From here on, the tutorials will be about a specific topic, explaining how to do something, or solving a problem. the posts might become a bit shorter but the codes would become a bit longer and more complex. I will resume my Photoshop tutorials and since a big chink of the game is about images I suggest checking them to improve your skill and consequently improve your games visual appeal.
Like I always say, experiment and get into trouble, I'm here to help you and answer your questions.