SEC S20W4 - Introduction to PHP Part - 2
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @starrchris about the PHP. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
Section 1
Exceptions: Explain in detail what exceptions are, what they are used for, and how they handle errors in PHP. Include an example of using the try and catch blocks.
First of all I will take a real life example to explain exception
then I will explain it in programming and it will help to understand it in a better way.
In an online banking system imagine a user is trying to transfer money from their account to another account. We know that the transfer operation involves different steps such as checking the balance and validating account details. The it performs the transfer. Various exceptions can occur during this process such as insufficient funds, invalid account numbers or system issues. We can tale this example in steemit as well. If we are going to transfer steems to an account but while sending we write wrong username which does not exist so it is an exception. Similarly in the programming languages there are also exceptions.
In programming an exception is a mechanism which is used to handle errors and the unexpected behaviour which can occur during the execution of the program. Exceptions help to deal with the runtime errors without stopping the entire system. Exceptions allow the developers to catch and handle the errors instead of terminating the program.
Purpose of Exceptions
Exceptions are used for the several purposes which are given below:
Error Handing: Exceptions provide a structured approach to handle the runtime errors. They ensure the program can respond and recover from the unexpected situations without crashing the whole system.
Separation of concerns: Exceptions help the developers to separate the error handling code from the logic code. It makes the code look clear and easy to maintain.
Program Flow Control: Exceptions can also be used to control the flow of the program. When an error occurs they allow the developers to handle specific cases or log errors without disturbing the experience of the user. We know that user experience matters a lot so exceptions help to facilitate the user experience even when an error occurs.
Exception Handling in PHP
In PHP language exceptions are thrown using the throw
statement. These exceptions are caught with the help of the try
and catch
method. Same way is also used in the Dart language to handle the exceptions. The exception handling mechanism of PHP depends upon the object oriented principles. Because the exceptions are the objects and they represent an error or any abnormal condition.
In the PHP when an exception is thrown then it looks for the nearest catch
block that will be able to handle that exception during the runtime. But on the other hand if there is no any catch block which matches the exception then PHP will terminate the script and it will display an error. In this way PHP handle errors in runtime.
Key Terms in Exception Handling
We use different terms in the exceptions so we should have knowledge of those terms as well while using them. So here are the key terms which we use in exception handling:
try block: The block of code which can throw an exception is placed in the try block.
throw statement: This block of code is used to throw an exception when an error condition is met.
catch block: This block of code is used to handle the exception thrown from the try block. We can specify different catch blocks for the different types of exceptions.
finally block (optional): This block is executed regardless of whether an exception was thrown or not. It is often used for cleanup code.
Example of Using try
and catch
in PHP
Here is an example to show the usage of the try
and catch
blocks to handle the exceptions.
<?php
// Defining a custom exception class
class DivisionByZeroException extends Exception {
// Custom message formatting
public function errorMessage() {
return "Error: Division by zero is not allowed.";
}
}
function divide($numerator, $denominator) {
// Check for division by zero
if ($denominator == 0) {
// Throwing an exception if denominator is zero
throw new DivisionByZeroException();
}
return $numerator / $denominator;
}
try {
// Attempt to divide 10 by 0
echo divide(10, 0);
} catch (DivisionByZeroException $e) {
// Handle the exception by printing a custom error message
echo $e->errorMessage();
}
?>
Explanation of the Code
Custom Exception Class: I have defined a custom exception class DivisionByZeroException
. It extends the built in exception class of PHP. It allows us to define specific behaviours or messages related to the exception.
divide Function: The divide function
is taking two parameters such as $numerator
and $denominator
. Before performing division it checks if the denominator is zero. If zero is passed as the denominator then a DivisionByZeroException
is thrown.
try Block: The code inside the try
block divides 10 by 0. As the denominator is zero which is not allowed in division so the exception is thrown.
catch Block: The catch
block catches the DivisionByZeroException
. It handles it by calling the errorMessage()
. It displays relevant error message.
Output of the example
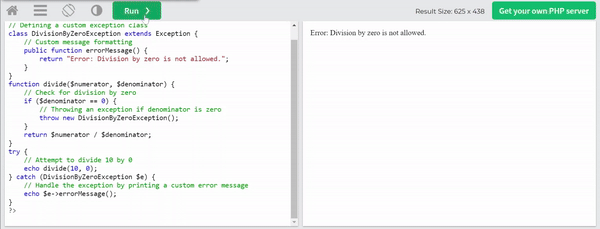
Error: Division by zero is not allowed.
How Exceptions Handle Errors
Catch specific exceptions: PHP allows catching specific exceptions. For this purpose we define multiple catch blocks. Each block handles different type of exception.
Graceful degradation: Exceptions do not terminate the script but they allow for alternative error handling logic to execute. They ensure the program will continue to run.
Enhanced Debugging: Exceptions provide detailed information about the error. And it will help us to debug the code easily because of the complete information.
Six Types of Errors
In programming we often face different types of errors. And these errors affect the complete code. These errors can occur due to the syntax issues, logic mistakes or environmental factors. Here are six errors which we face in PHP along with the examples:
Parse Errors (Syntax Errors)
Parse errors occur when there is a mistake in the syntax of the code. These errors prevent the PHP compiler from properly parsing the script. These error happen when a developer forget the semicolons, brackets and parentheses.
Example:
<?php
function sayHello() {
echo "Hello, World!";
?>
This code has a syntax error because the closing bracket of the function sayHello()
is not available. When we will compile the program then it will show an unexpected error and the program will not run.
Fatal Errors
These errors occur when PHP faces critical problem. It prevents further execution. These errors occur by using undefined functions. These may also occur because of the missing files. Fatal errors stop the script to run further.
Example:
<?php
// Attempt to call a function that does not exist
nonExistentFunction();
?>
In the above example I have just written a hypothetical example to show the program is trying to call the functions which is not available. So when we call those functions which are not part of the program then the program will not run and will stop its working. The above code will throw a fatal error ` call to undefined function nonExistentFunction() in script.php.
Warning Errors
Warning errors are also the part of the PHP errors. These occur when PHP faces a non fatal issue. It means that the script will run but with the existence of the problems. These warnings often appear when we deal with the file operations, variables or functions that have not been defined or initialized properly.
Example:
<?php
// Trying to include a non-existent file
include("nonExistentFile.php");
echo "This will still be executed despite the warning.";
?>
The above code will show a warning such as include(nonExistentFile.php): Failed to open stream. No such file or directory in script.php. But the program will run and the echo statement will be printed.
Notice Errors
These errors are not severe errors. They occur when PHP faces minor issues. These notice errors are often related to variables that have not been initialized. If we access the variables incorrectly then notice errors will also occur. But PHP will still run the script but along with this it issues a notice to alert the developer regarding the error.
Example:
<?php
// Using an undefined variable
echo $undefinedVariable;
?>
Here you can see that I did not intialize the variable but I am calling it so it will give a notice error that I have not initialized or declared the $undefinedVariable
.
Undefined Index/Undefined Offset Errors
We can also face undefined index error in PHP. It occurs when we try to access an array key or index which is not available or which does not exist in the program. In this regard PHP will throw an undefined index notice.
Example:
<?php
$person = ['name' => 'John', 'age' => 30];
echo $person['address'];
?>
In the above example there are 2 indexes at which there are two items stored as name
and age
. But I am trying to get address
from the above list which is not available in the list. In this way PHP will throw an error because the key address
is not defined for the array $person
.
Example of Undefined Offset
<?php
$numbers = [10, 20, 30];
echo $numbers[5];
?>
In the above example there are a total of 3 indexes from 0 to 2. But if you see I am trying to access index number 5 which is not available in the array $numbers
. So it will throw an error.
Logical Errors
Logical errors are very common in each program as we have to make a logic to solve the problems. And we can make the logic wrong by not completely understanding the conditions and requirements. Because of the logical errors the program runs but it produce incorrect results. And these errors are difficult to find because they are not related to syntax and the compiler does not inform us that there is any error.
We have to spot by ourselves the logical errors by comparing the output of the program with the actual expected output.
Example:
<?php
// Function to calculate the average of three numbers
function calculateAverage($a, $b, $c) {
// Incorrect logic: missing parentheses in the division calculation
$average = $a + $b + $c / 3;
return $average;
}
echo calculateAverage(10, 20, 30);
?>
In the above example I have written a simple program to calculate the average of the 3 given numbers. All the syntax is correct and the program is running and it is not throwing any exception or error. But surprisingly it is producing wrong output. It is giving average of 10, 20, 30 as 40 which is incorrect and the correct average of these numbers is 20.
This is an example of logical error because teh suntax is correct and the script is running smoothly without stopping but the output ios wrong because of the mistake in the calculation formula. The correct logic is $average = ($a + $b + $c) / 3
;
Personal Errors and Images
Errors are the parts of the programming. Everyone face errors in programming because no one is complete and anyone can face these problems in coding. Errors in programming are the issues and the flaws in the code. And these errors prevent a program from running. These errors can be caused by various factors such as syntax mistakes, incorrect logic or environmental problems. Environment problems are because of the missing files or insufficient permissions. These errors can halt the program or they cause the program to behave unexpectedly.
There are different types of the errors such as:
Syntax Errors
These are the mistakes in the structure of the code. We can miss the brackets or semicolons so these errors arise due to this problem. When we use wrong commands then it also a syntax error. So on the whole we can say that the syntax error occurs when we violate the syntax rules of the programming languages.
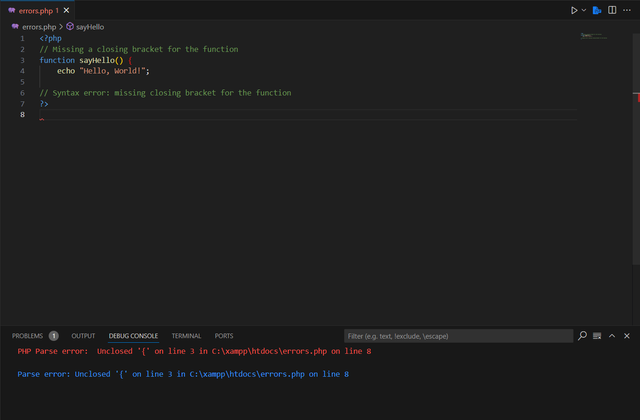
Here you can see the syntax error. I am compiling the program but because of the syntax error the program is not being compiled and throwing an error. Actually there is the syntax error of not using a closing bracket }
at the end. That is the reason the debugger is showing an error of Parse error: syntax error, unexpected end of file in script.php on line 8
.
Runtime Errors
These are the errors which occur while running the program. These are not the syntax errors. In these errors the program does not run.
I was writing a code to create a loan calculator. And while writing the calculation program I faced an error in the runtime while performing the division. I mistakenly set the divisor to zero during the calculation so the runtime error appeared.
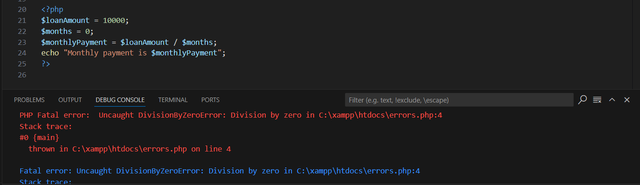
You can see I unintentionally set divisor as 0. And because of this the program is throwing an error during its compilation which is PHP Fatal error: Uncaught DivisionByZeroError: Division by zero in C:\xampp\htdocs\errors.php:4
. You can see this is a fatal error which restricts the program from being compiled. This is the runtime error because it only occurs when I am running the program.
Logical Error
I often face logical errors as well in which the program runs smoothly and I become very happy but when I see the output of the program I become disappointed. Because the output becomes wrong because of the logical error. Here I will quote an example and my similar experience of facing logical error.
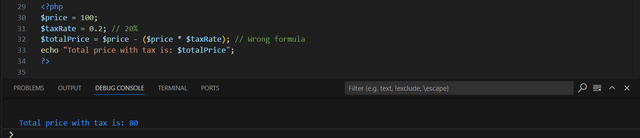
You can see that the above program is not throwing any error during compilation. It is running smoothly without any runtime error. But it is giving wrong output because of the logical error. It should produce a total price of 120 after adding 20% tax in the base amount 100.
I am using this formula $totalPrice = $price - ($price * $taxRate);
which is wrong because of a minor mistake which is the symbol of -
. I had to use addition +
symbol to sum up the base price along with the 20% tax.
This is how any programmer can face different types of errors. As you can see in the above examples and my own experiences of facing errors.
Includes and Importance
include
Syntax in PHP
The include statement in PHP is used to insert the data of one file to another file. It allows to reuse the separate parts of code. In this way it makes the code more organized and maintainable.
The syntax of include
is :include 'file_to_include.php';
We can also use parentheses but they are optional. If we use parentheses the it becomes include('file_to_include.php');
.
When PHP faces an include
statement t reads the content of that file. And after that it executes that content and file as part of the current script. If the file cannot be found a warning message will be generated. But the script will continue to run.
Importance of include
for Code Reuse
include
is important to use because of a number of reasons. It also has its strict counterpart which is require
. We use it especially for reusing the code in different parts of an application. Here is how we use it:
Modularity: We can use it to break the code into smaller parts. And making the code smaller and reusable files make the code more modular. This improves the readability and organization of the code. For example we can separate the header, footer as well as navigation bar into different files. We can also include them in multiple web pages. And it makes it easy to manage the website.
Maintainability: If we want to make a change in one part then we can do it easily. But if we want to change the data in multiple files which are same to each other then it will become difficult and time taking for us to do it. So it where we use include in the files and when we will change the data in just the include file then the change will be applied in all the files linked to the include file. So
include
avoids repetitive updates across different files.Efficiency: Include files allow us to reuse functions, HTML layouts or other parts of the code. So it save us from writing the code across different files. We can simply include a file which contains similar or common code.
Separation of Concerns: Include help us to keep the different parts of the code in separate files. And it makes the structure of the project more coherent and easy to understand. For example we can keep separate things such as database connections, configurations and functions in different files.
DRY Principle: The **Do not repeat yourself principle focuses to reduce the code duplication. So by using
include
we can avoid the repetition of the code. And it helps us to reduce the errors in the files.
Examples of Using include
in PHP
There are a number of examples of using include
in PHP. Some of them are given below:
Including a Header and Footer in Web Pages
Let suppose we have a website in which every page should have the same header and footer. So instead of writing the same code again and again in all the pages we can simply use include
by creating separate files of the header and footer to reuse them in other pages.
Here I have created 3 files index.php
, header.php
and footer.php
. IN these file index.php
is the main file and it needs header and footer. But as \I need to add the same header and footer on other pages as well so I have created separate files for the header and footer to reuse the single file at each page.
Header file

In the header file I have included CSS as well but I have neglected it to show here to keep the things simple and easy to read. Here only the content of the header is showing.
Footer file

This is the separate footer file which will be used in other pages using include
method.
Index file (main page)
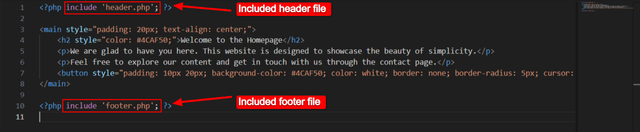
This is the main file where I have included both the separate files which header and footer. We can use these separate files in any other page as well such as in the contact page or in the About page. This is how we can avoid writing the same code again and again. But we can include the separate files using the include
method as I have used here.
Output
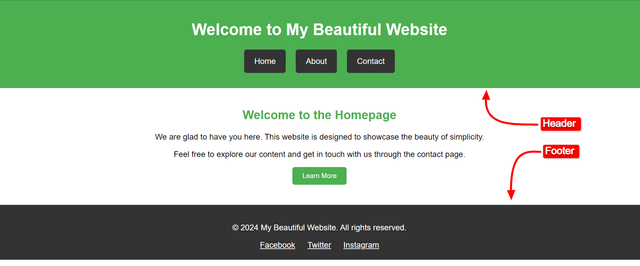
In this example the header.php
file is included at the top and similarly footer.php
is included at the bottom of the index.php
page.
Including a File with Functions
We can also include files that define common functions which are used throughout the application. For example we can have a file that contains utility functions for the database queries or for the form validation.
Functions file

This is the function file. Sometimes we need to greet the user at different pages of a website. So without writing the same code again and again in different pages we can simply include this function where it is needed.
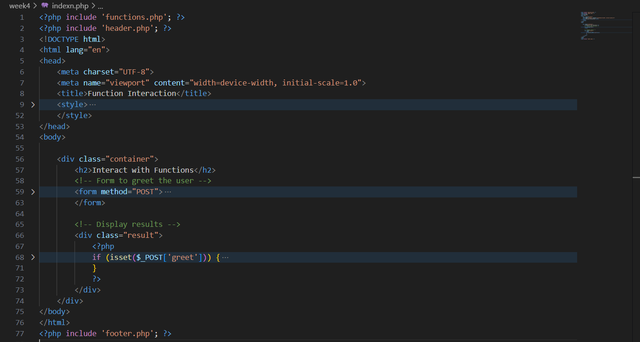
This is the main index file where I have to greet the user. So I have included the function file in this main page without writing the code again here. I have included the functions file at the top of this file <?php include 'functions.php'; ?>
to use it on the page. In this way we can use this function to other pages as well where we need to greet the user.
Output
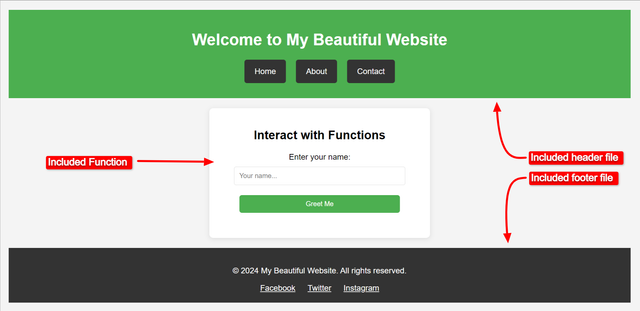
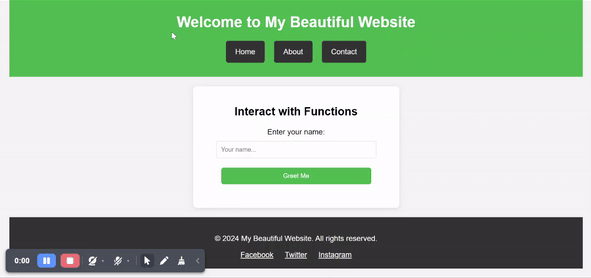
Database Connection
Database is an important element in the websites development to store the data. I use XAMPP server so here I will connect PHP file to database with the help of the XAMPP. And I will use the first method which is mysqli_connect()
and here the steps and code to connect through this method:
Step 1: Set up XAMPP
First of all I need to setup the XAMPP server. As mentioned earler I have already have this server so I will move to the next step. But if you do not have XAMPP then you need to download it and setup it to run.
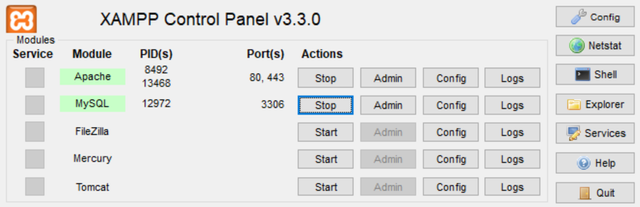
Then I am starting Apache and **MySQL in the control panel of the XAMPP. In the above picture you can see both the modules are running in the control panel.
Step 2: Create a MySQL Database
Then in order to create a database we need to follow these steps:
- Go to
http://localhost/phpmyadmin/
.
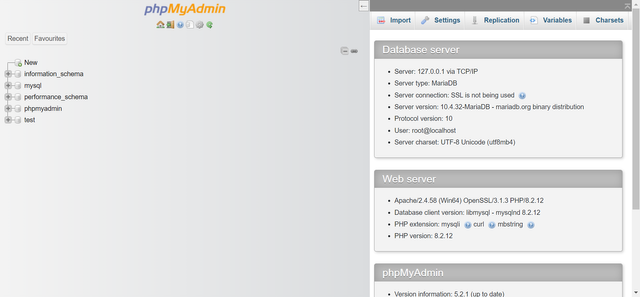
- We need to create a new database such as
test_db
and a table such asusers
.

Here I am creating a database with the name test_db
. It is very simple to create just select newand give the name to your database and click on
create` and you are all done.

Here I am creating a table with the name users
in the database test_db
.
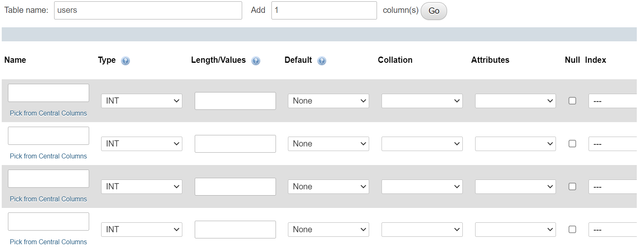
The table has been created successfully as you can see in the above picture.
Step 3: Connect to the Database using mysqli_connect()
In order to connect to the database using mysqli_connect()
we need to create a php file first. So I have created a php file including all the connection code. The name of my php file is db_connect_mysqli.php
.
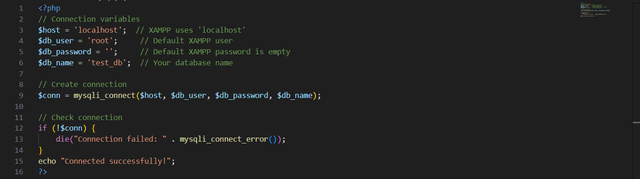
The above file is showing all the code related connection of the database. I have placed this file in the htdocs
folder for the successful connection.
Working
mysqli_connect()
function is used to establish connection to MySQL database.- All the variables such as
$host, $db_user, $db_password, $db_name
have been passed as parameter tomysqli_connect()
. - If the connectionof the database becomes successful without any error it will show a message Connected successfully!.
- If the connection fails the
mysqli_connect_error()
will display the error message.

Finally the connection has become successful with the database as while opening the php file http://localhost/week4/db_connect_mysqli.php
it is showing Connected successfully!. So in this way we can connect a php file with the database.
Section 2
Create a PHP page (learn.php) and use the include syntax to import the header, navigation bar, sidebar and footer.
We can create a PHP page learn.php
and then we can also import the header, navigation bar, sidebar and footer using include syntax. So here are the steps to follow to create the required page:
- First of all we need to create all the required files such as header, navigation bar, sidebar and footer to use them.
- Then we need to create the main page where we want to include other pages using include syntax.
Header file
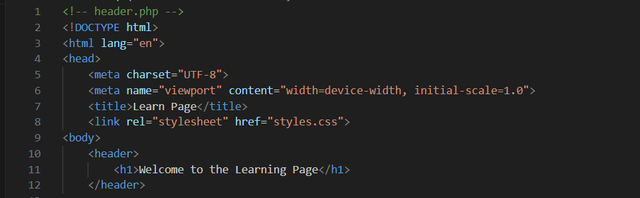
Navigation bar
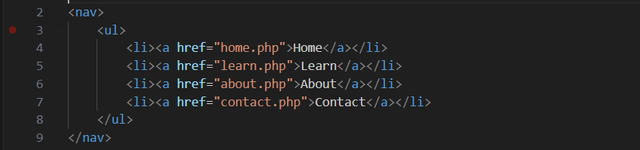
Footer file

Side bar
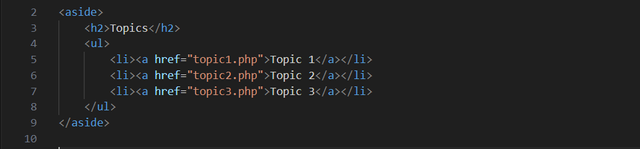
learn.php

Here you can see that I have not written any code in the this main page. I have just used include
method to import all the sections on the main page such as header, navigation bar, side bar
and footer
. So in this way we can import these pages anywhere we need them without writing the code again.
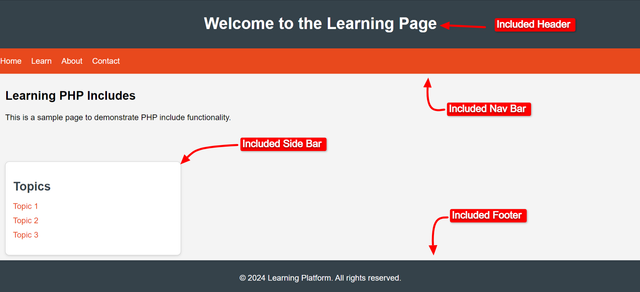
This above output is of learn.php
in which O have included all the sections. I have highlighted all the included section in the picture. **In have used CSS to make the page look beautiful and attractive but I have not shared the CSS code to avoid mess.
Create a database capable of storing the data for a registration page.
It is an easy task to create a database for the registration page. I will using XAMPP server for this purpose.
- First of all I will open
http://localhost/phpmyadmin/
. Here we can create the database.

First of all we need to create a new and separate database for the registration page. I have created a database with the name registration_db
as you can see in the above picture.
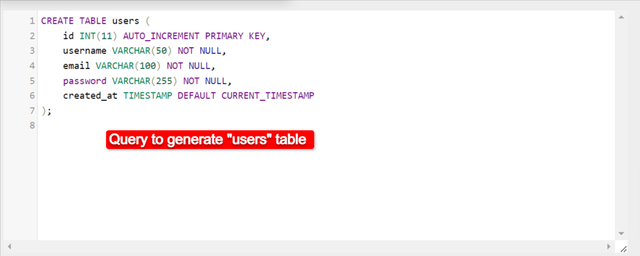

Here you can see the table has created with the name users
. Here the id
is incrementing automatically and it is a PRIMARY KEY
because the id is unique for each user. Each field has its own specific length.
So the database has been created to store the data from the registration form. In this way we can create any database to store different types of information.
Here I have created a simple registration form to check if the database is receiving the data from the registration form or not.
Here you can see that the registration form is sending the data to the database successfully and all the data is showing in the database. And the id is incrementing automatically as a unique primary key.
Create a PHP registration page to save the data of five students in a database.
Here is a simple example which will help us to understand that how we can create a PHP registration page to save the information of the 5 students in the database.
Step 1: Create a MySQL Database and Table
As usual the first step is to create the database and the table to store the data of the students. For this purpose I have opened http://localhost/phpmyadmin/
.

This is the creation of the database to store the information of the students.
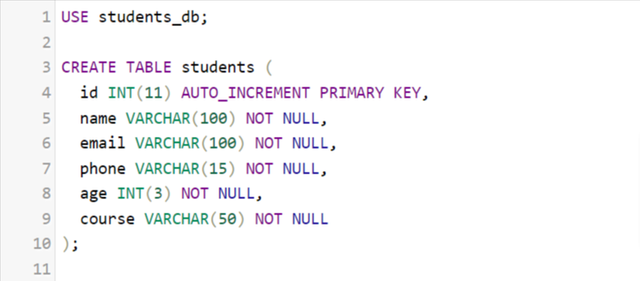
Here I have written SQL code for the creation of the table with the name students
and it is using the database which I have already created.
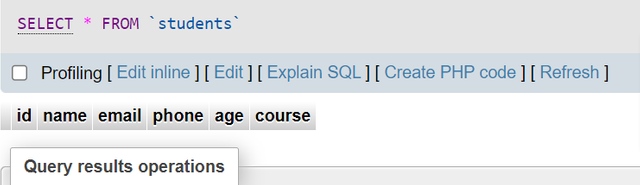
Here you can see that the table to store the information of the students has been created successfully and it is now ready to get the information of the students and it will store that information.
Step 2: Create the HTML Form for Registration
As we need to display a form to the users to get their data and information so we need an html form to get their data. This form will provide a structure of the form and it will facilitate the data processing.
Here is the registration form for the students. It has 6 input fields to get the data from the users. From these 6 inputs one is the submit button to send the data to the next file.
Step 3: Create the PHP Script to Process the Form Data
The 3rd and the most important step is to create a PHP script. This script will get the data from the user and then it will create a connection with the database. After the successful connection it will send the information of the students to the database. And the database will store the information of the students.
It is the complete PHP code which is creating a connection between the file and database. After that it is checking whether the connection successful or not. Then this checks the submission of the form. If the form is submitted then it will get data from the form.
After getting the students information the program will insert the data into the database. It will use $sql = "INSERT INTO students (name, email, phone, age, course)
for the insertion of the data. It is necessary to use the same name of the variables with the database otherwise the data will not be stored.
VALUES ('$name', '$email', '$phone', '$age', '$course')";
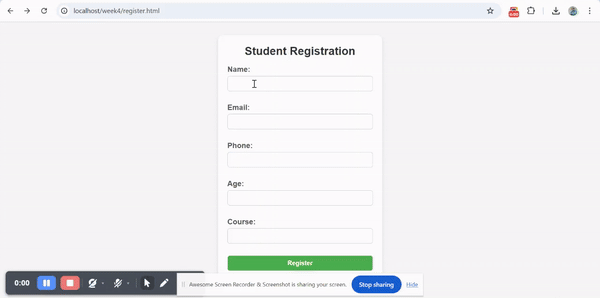
After creating all the required files and the database to store the information of the 5 students. I have just run the code and you can see the output. For the better user experience I have added CSS. You can see when I am adding the data of the students it is adding in the database and the id of each students is increasing automatically and it is unique for each student.
Create a PHP login page that verifies the login information with the ones stored in the database and redirects the user if successful.
Here is a step by step guide for creating a PHP login page that verifies user credentials stored in a MySQL database and then redirects the user upon successful login.
As previously I have already made a database and I have used the students table. But in the previous example students table did not have a password
field. So I am going to modify the previous table to save the time. I will use an SQL Query to include a password column in the table.
ALTER TABLE students ADD password VARCHAR(255) NOT NULL;
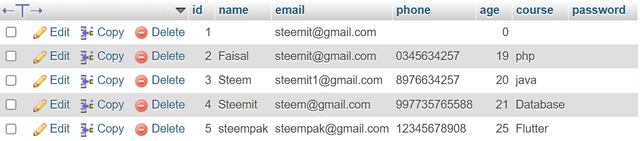
Here you can see that the password field has been added. It will be used to verify the credentials.
Registration Page
It is obvious that if the user registers itself then the user will be able to login. So I have created a register.php
page. It will collect the data from the user and then it will send this data to the database.
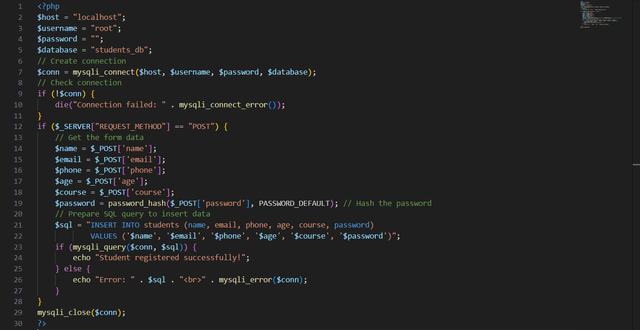
It is the only code of PHP and with this code the view will not appear on the website to get the data from the user. So I also created a register.html
page which will help us to give visual representation.
Log in Page
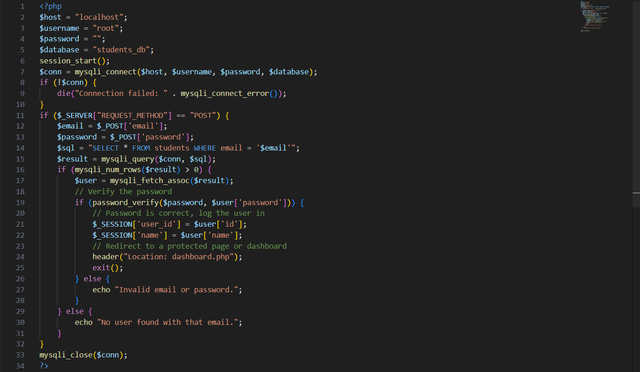
This is the PHP login page. This PHP code checks the email address and the password of the user. If the email address and the password of the matches to the database then the log in will be successful. And the user will be redirected to the dashboard.
I also created an HTML file such as login.html
which will work to display the input fields to the user. The user will input email and password in those fields. These will be extracted from the fields and then the PHP scripts validate these inputs by matching it with the database to decide whether the user should be given an access to the next page or not.
Dashboard Page
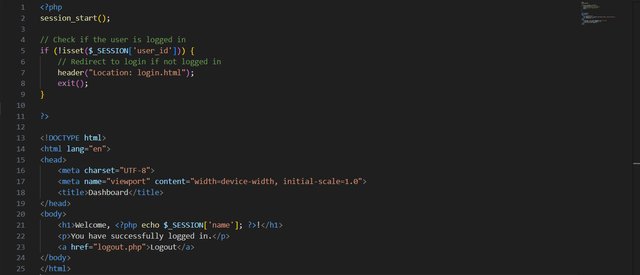
We need to redirect the user after the successful login to a page. So fro that purpose I have just created a simple page to which the user will be redirected after the successful login.
Logout Page

It is common that a user can also logout from the page. So I have added an option of the log out on the dashboard. And when the user will log out it will be redirected to the login page again. The user can again log in using the email and password.
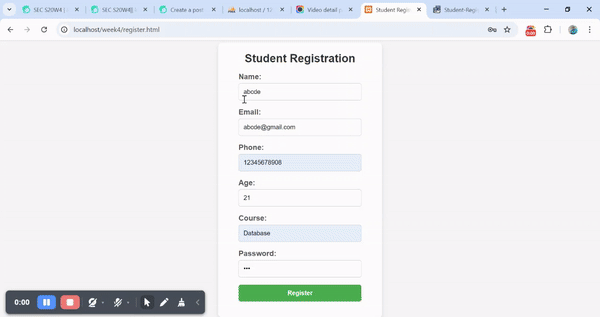
Here is the output and the complete working of all the above hard work. It took me a lot of time. But I am happy that my register page is getting data from the user and then it is storing it in the database. And when I am trying to login again sing the email address and password it is working and checking all the conditions if the data is correct or incorrect.
If the data of the user is satisfactory at the time of log in the user will be redirected to the dashboard as you can see in the above example. If the user enters wrong credentials then it will show an error.

Here you can see that database as well. It is storing all the information of the users. The passwords are being stored in the hashed form for the security purpose. And from that while login the PHP script verifies the credentials and redirects the user accordingly.
Disclaimer: All the screenshots have been taken from the Vs code and my own browser of my task works otherwise stated.
I invite @solaymann, @chant and @ruthjoe to join this contest about PHP.
X Promotion: https://x.com/stylishtiger3/status/1842502485531103563
Hello, sir. I appreciate the good job you are doing. I invite you to read my work. I'm using PDO instead of
mysqli_connect()
, as PDO is a more modern alternative.https://steemit.com/hive-167213/@kafio/sec-s20w4-or-or-introduction-to-php-part-2
Thank you so much for stopping by here. I will check your entry as soon as possible.
I appreciate that!
@kouba01, @starrchris