SEC S20W4|| Introduction to PHP Part -2
🌟 Enhancing My PHP Development Skills 🌟
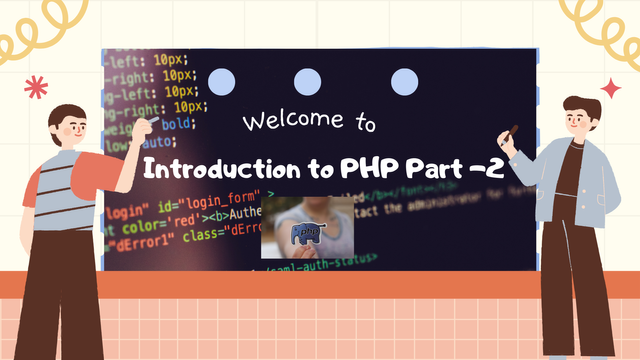
Image Source: canva
🔍 Section 1: Theoretical Questions
🚀 Understanding Exceptions in PHP
Exceptions in PHP help manage errors gracefully, allowing your applications to handle unexpected situations without crashing. Here's a basic example of using try
and catch
blocks to manage a division by zero error:
Division by Zero Error:
Image Placeholder: Handling Division by Zero Error
Image Placeholder: Result when dividing by zero
When dividing with a non-zero number, there is no error:
Image Placeholder: Example of successful division
Image Placeholder: Result of successful division
⚠️ Six Types of Errors in PHP
Parse Error:
These occur due to syntax errors in your code.
Image Placeholder: A common parse error
Image Placeholder: Output of codeFatal Error:
Stops script execution due to critical issues, such as calling undefined functions.
Image Placeholder: Example of a fatal error
Image Placeholder: Output of codeWarning:
Indicates potential problems without halting script execution.
Image Placeholder: A warning message indicating a non-critical issue
Image Placeholder: Output of code with warningNotice:
Alerts about less severe issues, often related to variables.
Image Placeholder: A notice about variable usage
Image Placeholder: Output of code with noticeDeprecated:
Indicates the use of deprecated functions or features that may be removed in future PHP versions.
Example:<?php if (ereg("pattern", $string)) { // do something } ?>
Output: Deprecated: Function
ereg()
is deprecated.Strict:
Suggests changes to code that may cause issues in future PHP versions.
Example:<?php function myFunction( ) { return; } $result = myFunction() + 5; ?>
Output: Strict Standards: Only variables should be passed by reference.
🧩 Personal Errors and Lessons Learned
Errors are part of the learning process. Here are three personal examples from my development journey:
Real-World Algorithm: Student Grade Processing System
Building a student grade processing system offers rich learning opportunities. Let's explore common errors faced:
Image Placeholder: Diagram illustrating the student grade processing system
- Division by Zero Error:
Why does the error show? When I pass an empty array,count($student['grades'])
will be 0, thus causing the division by zero error.
Image Placeholder: Caught in action: Division by zero error
Image Placeholder: Image of error message for Division by Zero
Image Placeholder: Solution for Division by Zero error
- Undefined Array Key Error:
Why does the error show? If we try to access a student that doesn't exist, we'll get an undefined array key error.
Image Placeholder: Error indicating an undefined array key
Image Placeholder: Error message for Undefined Array Key
Image Placeholder: Solution for Undefined Array Key error
- Incorrect Argument Passing:
Image Placeholder: Error due to incorrect argument passing
Image Placeholder: Image of error message for Incorrect Argument Passing
Image Placeholder: Solution for Incorrect Argument Passing
🔗 Includes and Their Importance
The include
statement in PHP allows you to incorporate code from one file into another, promoting reusability.
Example of Using Include:
// header.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Website</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
</body>
</html>
// footer.php
<footer>
<p>© 2024 My Website</p>
</footer>
🌐 Database Connection Example
Connecting to a database using PDO is crucial for data management
. Here’s how it’s done:
<?php
$host = 'localhost';
$dbname = 'mydb';
$username = 'user';
$password = 'password';
try {
$pdo = new PDO("mysql:host=$host;dbname=$dbname", $username, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch(PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
Here's your content with the Image Placeholder format added for each image, positioned below the images as requested:
🔍 Section 2: Practice Questions
📚 Page with Include
Let’s create a learn.php
page using includes to enhance maintainability.
Image Placeholder: Footer included in the learn page.
Image Placeholder: Navbar included in the learn page.
Image Placeholder: Header included in the learn page.
Image Placeholder: Sidebar content for easy navigation.
Image Placeholder: The main content of the learn page.
Image Placeholder: Final layout showcasing all components.
🗄️ Database Setup for Registration Page
First, configure the database:
Image Placeholder: Database configuration settings.
Then, create the students
table:
Image Placeholder: Structure of the students table in the database.
👤 Registration Page
Here’s a glimpse of the registration page for new students:
Image Placeholder: Registration code for the page.
Image Placeholder: User-friendly registration page design.
Successful Registration Message
Image Placeholder: Success message displayed after registration.
Database View Post-Registration
Image Placeholder: Database view after saving five students.
🔑 Login Page
Here’s the login page that verifies user credentials:
Image Placeholder: Login code for verification.
Image Placeholder: Simple and effective login page design.
Bonus: Redirecting to Welcome Page
Image Placeholder: Code for redirecting to welcome page.
Image Placeholder: Welcome page displayed after a successful login.
🎉 Conclusion
This showcase demonstrates the process of developing a PHP-based application, highlighting best practices, error handling, and effective design strategies. Each component plays a critical role in creating a seamless user experience.
I want to invite @khiwa , @shovro and @fauziah95 join the contest.
My introduction post on Steemit: link
About the Author
👋 Hi, I’m Kafio!
Software Engineer | Data Science Enthusiast | Trading Buff | Development Geek | Computer Science Lover 💻📊📈
I’m passionate about exploring the intersection of technology and innovation, with a special interest in data science, trading, and software development. Whether it’s diving into the latest in computer science or developing new projects, I’m always excited to learn and share insights. 🚀
I also love to travel
Got questions or just want to connect? Feel free to reach out to me at: [email protected] 🌟
Hi @kafio you have presented everything well and in order. Your PDO method to connect the database and PHP file is good. Great job while creating and linking the login page. Your work is appreciative. Best wishes.
Thank you so much for your kind word! I really appreciate your feedback on my PDO method and the login page. It’s always great to hear that my efforts are recognice. If you have any suggestions or tips for improvement, I’d love to hear them. Best wishes to you too!
the post is shared on Twitter: Twitter Link.
#steemexclusive
@ myteacher
no
no
Thank you for making a handy post in our community, we hope the verification from the teaching team will be more detailed to assess your post.
Good luck with your participation.
thank you for your feedback
Omar, my friend, as you've been telling us that programming is your world, I believe that you do understand this class well. I don't have sufficient knowledge on this subject, so I don't know what to comment on this article of yours. I wish you the best of luck, my friend. I am happy to see you have found joy and are growing on Steem.
Keep up the energy.
Thank you, my friend! I won't forget you helping me grow on Steemit.
No problem. Enjoy the journey and keep the spirit level good.
Hello @kafio I liked your post improving PHP skills. Your examples about errors, like division by zero, are really clear and helpful.
Yes it would be great if you could add a video to show how your code works.Wish you best of luck 🤗🌼🌸
Thanks a lot for your nice comment! I’m super happy you liked the examples, they were fun to make. 😊 I think adding a video would be a cool idea, I’ll try to do that next time! Wishing you the best too! 🌼🌸