Basic programming course: Lesson #5 Control structures. Part 2. Cycles.
Hello Everyone
I'm AhsanSharif From Pakistan
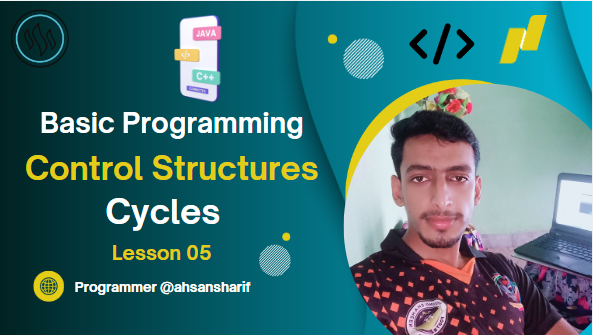
Dessign With Canva
Explain the cycles in detail. What are they and how are they used? What is the difference between the While and Do-While cycle? |
---|
Cycles
Cycles, also called loops in programming, are structures that repeat a block of code until a certain condition is true. They control the flow of programming very well and they manage and automate repetitive tasks very well.
Three types of cycles are commonly used in programming. Each of these loops has its purpose and is used in different situations accordingly.
Types
- While Loop
- Do While Loop
- For Loop
While Loop
This loop executes the code until it finds a true condition. First of all, it checks for the condition properly, but if any of the conditions are false, then it stops the loop.
Example In C++:
int i = 1;
while (i <= 5) {
cout << i << endl;
i++;
}
How does it work?
First, the while loop checks the condition to see if it is true. If it is true, it starts executing the code inside the loop. After each iteration, it increments the variables and it checks it again. When incrementing i becomes greater than five, the loop stops.
When to use?
It is used when the number of iterations is unknown or depends on a condition. As we read a user's input until he enters a certain value.
Do While Loop
This loop is similar to the while loop but one difference is that it checks the condition after the code block executes. That is, it must execute the code inside the loop once.
Example In C++:
int i = 1;
do {
cout << i << endl;
i++;
} while (i <= 5);
How does it work?
It works in a way that it executes the code of the block and then the condition is also checked if the condition is true then it executes it again and this process continues until a condition doesn't get wrong.
When to use?
We use this when we want our loop to execute at least once without any condition. An example of this is when we prompt a user for input. And he makes sure that he at least has to do this before he checks that something is valid.
For Loop
This loop is used when we already have the number of iterations. It often has a built-in initialization condition check and an increment and decrement step.
Example In C++:
for (int i = 1; i <= 5; i++) {
cout << i << endl;
}
How it works?
These people start from i to a. It checks if it is OK then it executes the block of code. If there is an increment after the iteration it continues until i exceeds five.
When to use?
This loop is used when you have information about how many times the loop should run. For example, iterating over the elements of an array.
Difference
While Loop:
A condition is checked before the code is executed.
If the condition at the beginning of it becomes false, the code inside the loop may not fire at all.
As used for input validation that requires a precondition check.
Example:
int x = 10;
while (x < 5) {
cout << "This will not print because the condition is false.";
}
Do While Loop:
Here the condition is checked after the code is executed.
Here the code inside the loop must be executed once whether the condition is false or true.
For example, this is used when you want to ensure that the code runs at least once, such as asking for input from a user.
Example:
int x = 10;
do {
cout << "This will print once even though x is not less than 5.";
} while (x < 5);
Practical Example Using While Loop, Do While Loop, and For Loop
C++ Code
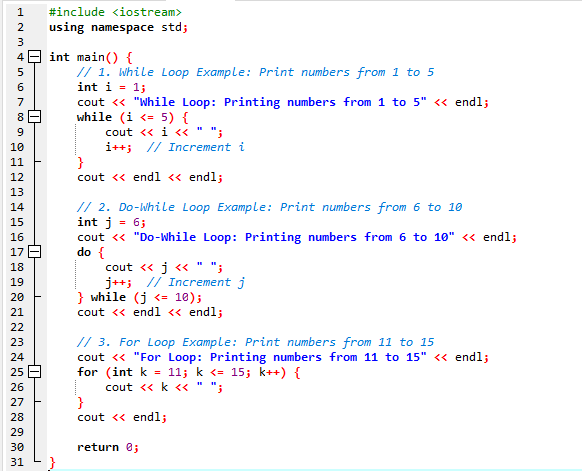
Output
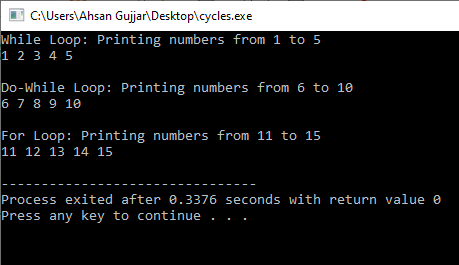
Investigate how the Switch-case structure is used and give us an example. |
---|
We use the switch case in C++ to make a decision that compares a variable with other possible values. And performs the action based on which values match. It is used in place of if-else statements and it makes our code more efficient and readable.
Structure of switch statement in C++:
switch (expression) {
case constant1:
// Code block for constant1
break;
case constant2:
// Code block for constant2
break;
case constant3:
// Code block for constant3
break;
default:
// Code block if none of the cases match
}
Explanation:
expression
: Here values and variables are being compared.
case constant X
: It is compared to each case with a constant value.
break
: The code here prevents going to the next case. If it is omitted here, it continues the execution of the block to the next case of the program.
default
: This is an extra block that is executed when the values in any of the cases do not match.
Here I am describing an example that takes input from the user and displays days based on that input using a switch case.
C++ Code
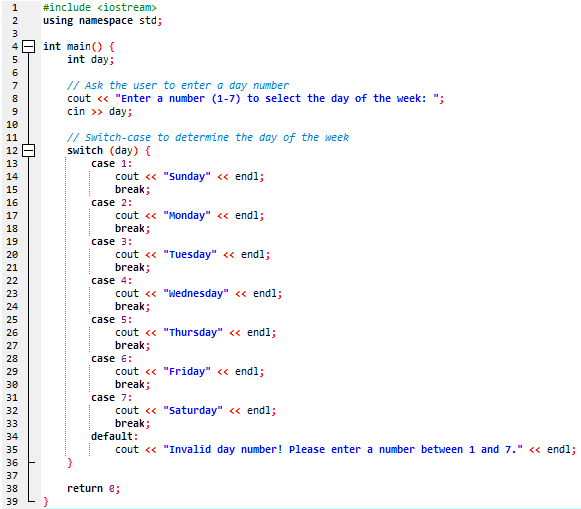
Output
User Enter 6
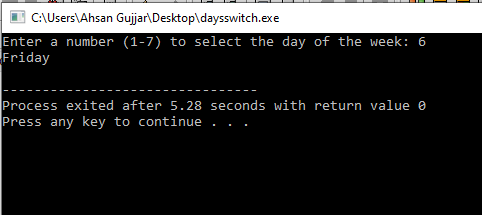
User Enter 2
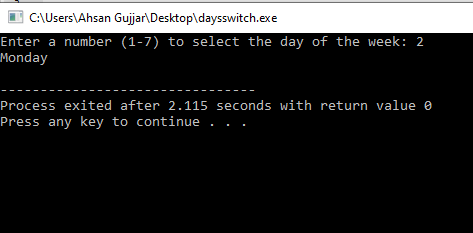
User Enter 9 Invalid Number
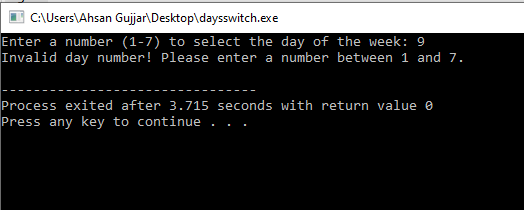
Explanation:
The program will first ask the user to enter a value between one and seven.
Now the program will use a switch case and accordingly, it will check the day if the user has pressed six then it will print Friday if the user has pressed two then it will print Monday thus it will be from one to seven. Will print the days in between.
If the user does not enter a value between one and seven, and if he enters eight or nine, the default case will be used and it will print the valid day number.
In the break statement, it will indicate that if it has matched the exact output using the think case, then it will block the think and show.
Explain what the following code does: |
---|
It is an algorithmic, simple menu-based program that allows the user to perform tasks as desired. And it continuously receives input from the user using a loop. It continues to receive input from the user until the user decides to exit the program.
How this program works is detailed below.
Variables:
Various types of variables are used in this program. The purpose of these variables is as follows.
- op:
This is the variable that holds the user's choice of option. - n:
This is the variable that holds the number of numbers the user wants to collect. - ns:
These variables each hold individual numbers to add. - a:
This variable acts as an accumulator in that it stores a rolling collection of numbers. - exit:
This is a boolean flag that controls whether the program should continue or terminate.
Process:
Initialize Variables:
- Variable
a
is set to zero to collect the sum of the numbers. - The
exit
flag is set tofalse
to keep the program running until the user decides to exit the program.
Start a Repetition (loop):
We use this block to iterate through the program's main menu. This works until our exit is correct.
Display Menu Option:
The program displays three options for the user to choose from.
Option 01:
Sum Numbers
Option 02:
Result of Sums
Option 31:
Exit Program
Get User Input:
The program observes the user's preferences and uses a thought case accordingly. And then determines what to do based on the op variable.
Case 01 (Sum Numbers):
First, the program will confirm the sum of numbers the user wants (n
).
When the combination is found, it then uses a loop to insert each number(ns
). And then adds it to the accumulator (a
).
When the numbers in the program are added, it then displays a message that says complete. And then waits for the user to press another button to continue.
Case 02 (Show Result):
It will print whatever amount is stored in a variable (a
) and then wait for a button to be pressed to continue.
Case 03 (Exit the Program):
Here a message will be written on the screen saying Allah Hafiz or Bye. Then the exit flag will be set to true to break the running loop and terminate the program.
Default Case (Invalid Input):
When the user selects an incorrect option, it displays a message indicating the incorrect option and then expects the user to press a button to continue.
Loop Until Exit:
The loop that runs here keeps prompting the user to select one of the options. It prompts the user until the exit is set to true. This happens when the user selects a third option.
Write a program in pseudo-code that asks the user for a number greater than 10 (Do-While), then add all the numbers with an accumulator (While) Example: The user enters 15. The program adds up to 1+2+3+4+5+6+7+8+9+10+11+12+13+14+15. |
---|
For a C++ Plus program, I am writing a pseudo code that will prompt the user to enter the next digit from 10 using a do-while loop. Then using a while loop will add them from one to the end of the entered number.
Pseudo-code:
START
DECLARE integer num
DECLARE integer sum = 0
DECLARE integer counter = 1
// Step 1: Input number greater than 10 using a do-while loop
DO
PRINT "Enter a number greater than 10: "
READ num
WHILE num <= 10
// Step 2: Add all numbers from 1 to num using a while loop
WHILE counter <= num
sum = sum + counter
counter = counter + 1
END WHILE
// Step 3: Display the result
PRINT "The sum of numbers from 1 to " + num + " is: " + sum
END
Explanation:
Step 01 (Do-While Loop):
Here the program will prompt the user to enter a digit greater than 10. If he enters any digit less than 10, it will ask for input again. It will not run next until he gives the input.
Step 02 (While Loop):
Once the correct number is entered here, then all the numbers from one to the number entered in the program will be added. If the user enters 20 then it will sum up all the digits from 1 to 20 in between. As one two three four five adding them all will reach 20.
Step 03:
The program will display its sum after performing all its activities.
C++ Implementation:
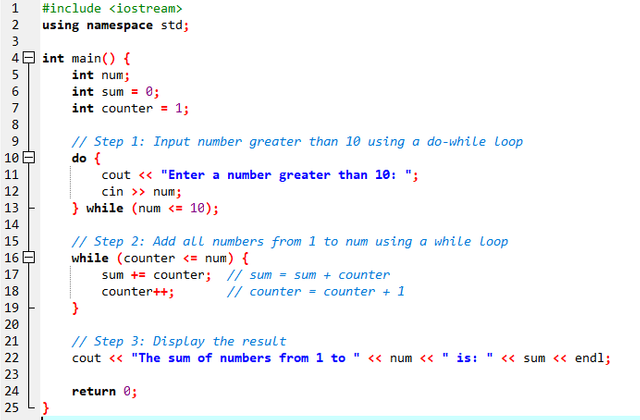
Output
User Enter 15
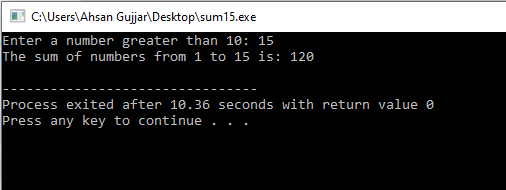
User Enter 56
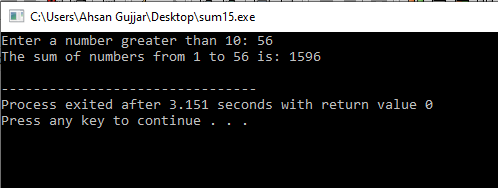
Here is my task end. Thanks all for staying here. I would like to invite my friends @ashkhan, @malikusman1, and @abdullahw2 to join this task.
Cc:
@alejos7ven
X:
https://x.com/AhsanGu58401302/status/1843646262991806591
Thank you so much dear @chant for your kind support and love.