Basic programming course: Lesson #3 Operations
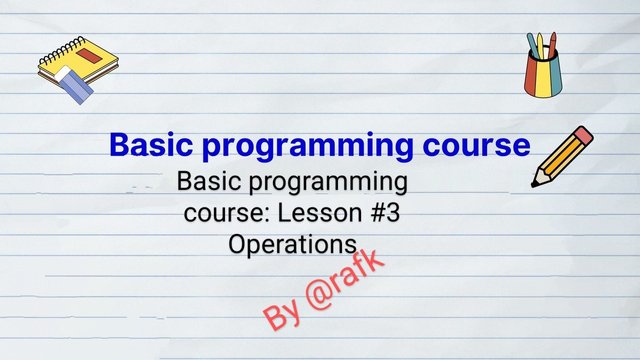
Operations
Hi everyone !
Welcome to this week's engagement challenge. I am happy to be participating on lesson #3 of this week's challenge. Let's get right in.
Programming is the involves the creating of instructions for a computer to understand and execute. These instructions are then written in a specific programming language.
There exist several variety of different programming languages, with each having its own pros and and cons. To write a program, you must understand the problem you want to resolve and then develop a specific plan to solve the problem. Once you have develop a plan, you can write the code that will instruct the computer to execute out your plan and it will do just as instructed.
Give a brief summary of what arithmetic, comparison and logical operations are for.
1•Arithmetic Operations
Arithmetic operations are the fundamental building blocks of mathematics. They allow us to perform simple calculations with numbers, such like:
Addition: Combining two or more quantities to find their total. Example,
2 + 3 = 5
.Subtraction: Finding the difference between two quantities. Example,
7 - 4 = 3.
Multiplication: Repeatedly adding a quantity to itself. For example,
3 * 4 = 12.
Division: Dividing a quantity into equal parts. Example,
10 / 2 = 5.
2• Comparison Operations
These operations are used to find the relationship between two values. They are often used in programming to make decisions based on conditions. Common comparison operators involve:
Equal to
==
: This command helps verify if two values are equal. Example,5 == 5
istrue.
Not equal to
!=
: Here the command verify if two values are not equal. Example,3 != 7
istrue
.Greater than
>
: the command verify if the first value is greater than the second. Example,8 > 5
is true.Less than
<
: confirms if the first value is less than the second. Example, 2 < 6 is true.Greater than or equal to
>=
: use in conditions where the first value is greater than or equal to the second. Example,7 >= 7
is true.Less than or equal to
<=
: it helps Confirm if the first value is less than or equal to the second. Example,4 <= 4
is true.
3• Logical Operations
These operations are used in programming to combine or evaluate logical expressions. They are often used in programming to make decisions based on multiple conditions. Common logical operators include:
AND
&&
: Returns true only if both conditions are true. For example,(2 < 5) && (3 > 1)
is true.OR
||
: This command will returnstrue
if at least one condition is true. Example,(4 == 4) || (7 < 2)
istrue.
NOT
!
: Reverses the logical value of an expression. For example,!(6 > 8)
is true.
These operations are necessary for various mathematical and computational tasks, ranging from simple calculations to complex problem-solving.
Make a program that asks the user for 2 numbers and evaluates if both numbers are the same.
Algorithm are_equal
Define num1, num2 As Integer
Write "Enter the first number:"
Read num1
Write "Enter the second number:"
Read num2
If num1 = num2 Then
Write "The numbers are equal."
Else
Write "The numbers are different."
EndIf
EndAlgorithm
This program performs the following actions:
Defines the variables: declares two integer variables called num1 and num2 to store the numbers entered by the user.
Requests the numbers: Displays messages on the screen asking the user to enter the first and second numbers.
Reads the numbers: Stores the numbers entered by the user in the variables num1 and num2.
Compares the numbers: Uses an If-Then-Or else structure to compare whether num1 is equal to num2.
Displays the result: If the numbers are equal, it displays the message "The numbers are equal". Otherwise, it displays the message "The numbers are different".
When running this program, the user will be prompted to enter two numbers. Then, the program will compare the numbers and display the corresponding result.
Transform the following mathematical expressions into arithmetic expressions on my computer:
Here are the arithmetic expressions for the given
mathematical expressions:
Y = (8 - 2) * (5 + 4)
This expression calculates the product of the difference between 8 and 2, and the sum of 5 and 4.
X = 8 / 2 + 5 / 5
This expression calculates the sum of 8 divided by 2 and 5 divided by 5.
Z = ((4 + 8) * 3) / 2 / 3
This expression calculates the result of dividing the product of the sum of 4 and 8, and 3 by 2, and then dividing that result by 3.
To evaluate these expressions on a computer, you can use a calculator or a programming language. In Python, the calculation looks thus:
Y = (8 - 2) * (5 + 4)
print(Y)
X = 8 / 2 + 5 / 5
print(X)
Z = ((4 + 8) * 3) / 2 / 3
print(Z)
Running this code will output the following results:
54
5.0
6.0
Evaluate if the results of the 3 operations are greater than or equal to 0 (>=) and show it on the screen.
The results of the calculations show that all three variables (Y, X, and Z) are greater than or equal to 0. Let's break it down:
Y Calculation:
First, we subtract:
Then, we add:
Finally, we multiply:
Result: Y = 54, which is greater than 0.
2 X Calculation:
First, we divide:
Then, we divide:
Finally, we add:
Result: X = 5, which is greater than 0.
Z Calculation:
First, we add:
Then, we divide:
Next, we divide:
Finally, we multiply:
Result: Z = 6, which is greater than 0.
Conclusion:
Y = 54, X = 5, and Z = 6 are all positive numbers (greater than 0). Therefore, each of these expressions evaluates to a positive value, confirming that all operations are valid and greater than or equal to zero.
Rounding up, I would like to invite the following persons. Join me participate in this contest. @fombae, @simonnwigwe and @sahmie
Cc: @alejos7ven
Crédit to: @rafk
@tipu curate
Holisss...
--
This is a manual curation from the @tipU Curation Project.
Upvoted 👌 (Mana: 5/8) Get profit votes with @tipU :)