[steemduino] An Open Source Arduino Library for Steem
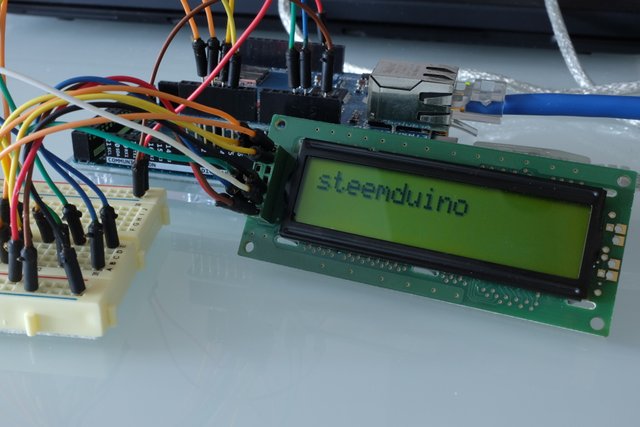
I'm pleased to announce the first alpha release of steemduino - an Arduino library for accessing the steem web socket API.
What is steemduino?
steemduino allows you to communicate with the Steem websocket API using an Arduino, the open source electronics platform.
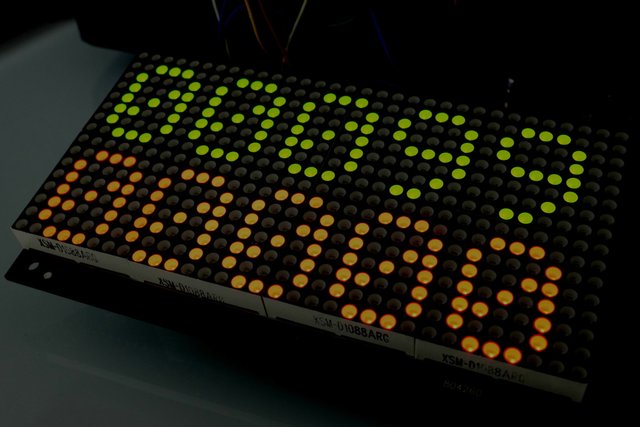
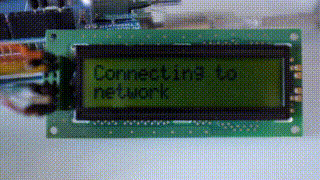
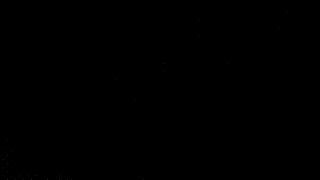
You can use the library to monitor incoming blocks and perform actions depending on the contents, such as displaying a message on an LCD screen or sounding an alarm.
With steemduino you can create a custom notification system that will alert you to anything that's happening in the steem blockchain. In future, as more functionality is added, the only limitations will be your imagination.
What's an alpha release?
An alpha release is a release where the code is still experimental and probably contains bugs. There are a lot of areas in steemduino that can be improved, but releasing the library allows me to get feedback and contributions from the community.
The number one big issue is memory use. The web socket library used by steemduino tries to load responses into memory, and since the Arduino doesn't have very much, blocks containing large posts or comments will fail. You've been warned.
The second issues on the list is functionality. At the moment its limited to getting blocks by their block number. You can already achieve a lot with this, but adding access to additional RPC methods will make it much more flexible.
Hardware requirements
steemduino was developed on the Arduino Mega 2560 and I recommend you use this. It's unlikely that anything will work on boards with less SRAM and Flash Memory, and it's not been tested on
All the examples use an Ethernet Shield to connect to The Internet. You can also try a WiFi shield, but this hasn't been tested and the examples will need adjusting.
Note: If you decide to use an Ethernet Shield, check carefully that it's compatible with an Arduino Mega. Not all version are.
Installation
Currently steemduino and its dependencies must be installed manually. You can download them from the links below:
The LED matrix examples depend on the following library:
All examples come with a makefile for building the project using Arduino-Makefile. With this you can avoid using the awful Arduino IDE.
Quick guide
This section will very quickly cover how the library works. In future posts I'll cover more specific tasks and components.
Each program you will have the following:
- An instance of
SteemRpc
for accessing the steem web socket API - An instance of
JsonStreamingParser
andOpListener
for parsing blocks; - An op handler function for processing parsed blocks.
The code below is a skeleton you can use when beginning a project. It contains all the code you need to do all the steps above.
#include <Ethernet.h>
#include <SPI.h>
#include <JsonStreamingParser.h>
#include <OpListener.h>
#include <SteemRpc.h>
EthernetClient client = EthernetClient();
SteemRpc steem(client, "node.steem.ws", "/", 80);
unsigned long current_block = 0;
void op_handler(const String& op_type, const String& op_data) {
Serial.println(op_type);
}
void setup() {
Serial.begin(9600);
Serial.println("Connecting to network");
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
Ethernet.begin(mac);
Serial.println("Connecting to Steem web socket");
if (steem.connect()) {
Serial.println("Connected to Steem web socket");
} else {
Serial.println("Failed to connect to Steem websocket");
while (1) {};
}
}
void loop() {
// Hang if the client disconnects
if (!steem.is_connected()) {
Serial.println("Client disconnected");
while (1) {}
}
// Get the last block number
unsigned long last_block = steem.get_last_block_num();
if (current_block == 0) current_block = last_block;
while (current_block <= last_block) {
// Get the block
String block = "";
if (!steem.get_block(current_block, block)) {
Serial.print("Failed to get block ");
Serial.println(current_block);
continue;
}
// Parse the block
JsonStreamingParser parser;
OpListener listener(&op_handler);
parser.setListener(&listener);
const char* block_c = block.c_str();
for (size_t i = 0; i < strlen(block_c); ++i) {
parser.parse(block_c[i]);
}
current_block += 1;
}
delay(5000);
}
For more information see the examples provided with the library. If you really get stuck and need help, you're welcome to come at chat with me on steemit.chat or open an issue on GitHub.
holy shit .. this is cooool!
Now let's add transaction signing to it .. then we can go IoT .. we'll be out in the public before the industry realizes what's going on!!
Thanks. There is a lot of potential with IoT. I started this just thinking of a more physical notifications. I'm sure there are many other uses I'm not aware of. If you have ideas, let me know :)
Edit: I just thought of one - with some additions to the library, you can make an actual bot. You could even make it move around for effect while it upvotes. Lol.
WOW. This is awesome.
I'd love to have one of those large displays made of LED lights, connected to rPI to show updates in realtime.
On an rPi you could probably get away with using Piston since you could use python :) Arduino's are much, much, much less powerful. This makes programming them a lot more challenging, but still fun.
The LED matrix in the post is made by Sure Electronics, and you can chain up to four of them together. That's 128x16!
Great project, I have actually programmed few autonomous robots on raspberry pi and I think exploring blockchain or Steem on it, would be great way to do some experiments. I would love to join experiments if you have some in mind?
For now I don't have any experiments in mind. The focus will be on improving the efficiency of the library. This will mean adapting the web socket library to work with streams rather than load data into memory, then the stream can just "pass through" the Arduino.
I'll also be adding more features in the sense of support for additional RPC calls. I'll couple this with additional components so people get a taste for what's possible.
Awesome project @bitcalm - Can't wait to play around with this. I'll update the Steem API Docs this is a great toolkit add-on.. The LED matrix is awesome and chaining them up would be epic :)
Thanks. Great that you'll add it to the docs. There are so many components you can do fun things with, I'm sure others will come up with creative projects. Can't wait to see them :)
do you know of any online/offline emulator of the arduino? for testing without the actual chipset...
I found this stack exchange post with a list of possibilities. I've not used any of them and have no idea how well they work.
The complicated side of emulating Arduino's is that you need to emulate the chip, and not all boards use the same one. An Uno uses a different chip to a Mega, for example. That complicates things. I've always said to myself if I ever end up a squadrillionaire (that's more zero's than there are atoms in the observable universe), I'd write one. It'd be a fun project, but not for the faint of heart.
But in all honesty these things are so cheap ($50 or so) you don't lose much if it ends up collecting dust in a cupboard. For me an emulator would help with automated testing.
WOW. This is awesome
This is fantastic! The applications are so vast it's hard to even fathom. Amazing work! Very exciting!
Awesome!
Great project! I might be helping out on the github repo!
great stuff. now we need something similar on the RPI? or is there already one for the RPI?
You can use python on an RPi so you can use existing libraries such as Piston. I'm not sure how you'd access the IO pins, but I'm sure it's possible, I've just never tried.