Advanced Flutter Project - Best Practices - Generic BLoC Providers - Part Three
Repository
https://github.com/flutter/flutter
What Will I Learn?
- You will learn about BloC Pattern's History
- You will learn about Generics in Dart
- You will learn the Best way to implement a BLoC Provider
- You will learn about the Time Complexity of searching for an Inherited Widget in the Widget tree
- You will learn about potential Memory leaks that can happen as a result of not closing Streams in Dart
- You will learn how to deal with performance errors in a BLoC component
Requirements
System Requirements:
- IDEA intellij, Visual Studio Code with the Dart/Flutter Plugins, Android Studio or Xcode
- The Flutter SDK on the latest Master Build
- An Android or iOS Emulator or device for testing
OS Support for Flutter:
- Windows 7 SP1 or later (64-bit)
- macOS (64-bit)
- Linux (64-bit)
Required Knowledge
- A basic knowledge Programming
- A fair understanding of Mobile development and Imperative or Object Oriented Programming
- Basic knowledge of the BLoC
Resources for Flutter and this Project:
- Flutter Website: https://flutter.io/
- Flutter Official Documentation: https://flutter.io/docs/
- Flutter Installation Information: https://flutter.io/get-started/install/
- Flutter GitHub repository: https://github.com/flutter/flutter
- Flutter Dart 2 Information: https://github.com/flutter/flutter/wiki/Trying-the-preview-of-Dart-2-in-Flutter
- Flutter Technical Overview: https://flutter.io/technical-overview/
- Dart Website: https://www.dartlang.org/
- Flutter Awesome GitHub Repository: https://github.com/Solido/awesome-flutter
- The Flutter Provider Library: https://github.com/rrousselGit/provider
Sources:
Flutter Logo (Google): https://flutter.io/
Difficulty
- Advanced
Description
In this Flutter Video Tutorial, we look at the history of the BLoC Pattern and we talk about how to properly implement a generic BLoC provider to harness the time complexity of an Inherited Widget and the Disposal method of a Stateful Widget. The tutorial also covers Dart Generic types and Parameterized Types, along with a few other performance enhancing concepts. This implementation of the BLoC provider follows an agreed upon best practice for the BLoC Pattern that just recently has started to see more heavy use and it is the final evolution of the Provider in this Pattern.
The History of the BLoC pattern and the Inherited vs Stateful Widget dilemma
The BLoC pattern was originally thought up by a group of developers at Google who were working on Angular projects using the Dart programming language. These developers wanted to port their Angular applications into the newly released Flutter Framework. They used the BLoC pattern to create a common Business Logic Component which they shared between the two applications. The original implementations of this BLoC pattern used the Inherited Widget as the BLoC Provider. This was mainly done because searching the Flutter Widget Tree for an Inherited Widget has a constant time complexity cost. In other words, all widgets on the widget tree will take the same amount of time to find the inherited widget.
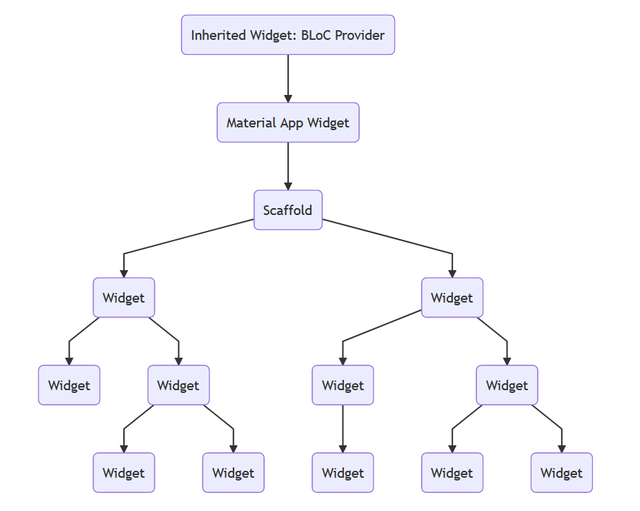
The architecture looked something like the flowchart above. Generally, the BLoC provider was embedded in the root widget of the application to allow all of the widgets to have access to the BLoC. As Flutter was refocused into a framework that can potentially build applications for not just mobile targets but also desktop and web targets, the size of applications increased. A problem in this architecture was discovered as a result. The BLoC component uses Streams and Sinks, but an Inherited Widget doesn't have a life cycle hook that will allow the user to release the resources used by the BLoC. This can lead to memory problems and in particular, it can cause memory leaks. The proposed solution was to use a Stateful Widget instead of the Inherited Widget.
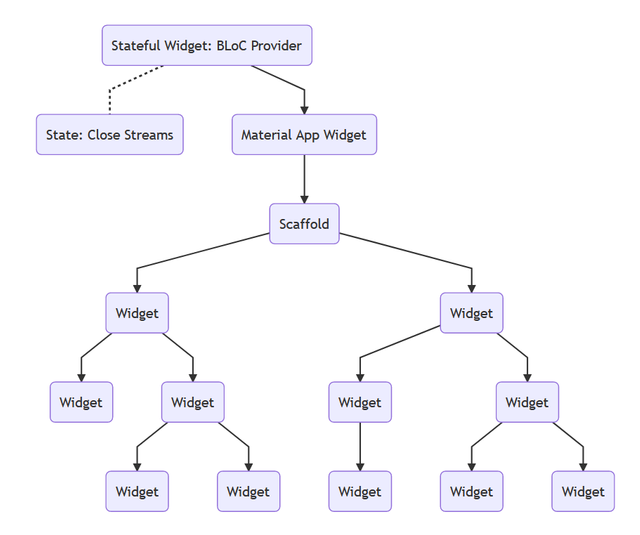
The above picture shows what this new architecture looked like. The Stateful Provider is placed in the root of the app and the State Object associated with the Stateful Widget is used to dispose of the Streams and Sinks in the BLoC. This architecture also has its own set of problems however. Developers can now dispose of resources and avoid memory leaks but the search Time Complexity to find the Provider is increased from Constant Time to Linear Time. The time to find the BLoC provider is now dependent upon where the widget is in the widget tree, with further away widgets taking more time to access the BLoC.
Building a Generic Provider with Inherited and Stateful Widgets
The Solution to the "Inherited Provider vs Stateful Provider" debate was to make use of both widget types to define the component. While this increased the size of the Provider significantly when compared to the old architectures, it removes both the Time Complexity problem and the Memory Leak problems. Also, because Dart features Generic Types, it is possible to build a Polymorphic and Generic Provider which can serve any number of different BLoC types.
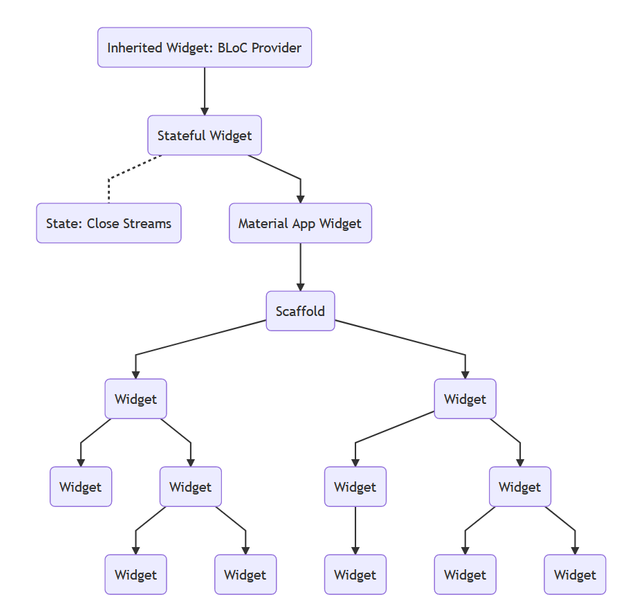
In the new architecture, the BLoC is directly embedded into an inherited widget which is wrapped in a Stateful widget. Like with the original architectures, the Provider is usually positioned at the root of the widget tree. The child widgets that need to access the BLoC use the search function of the Inherited widget which keeps the time complexity down at constant time and the BLoC has access to the outer Stateful Widget to dispose of its resources.
The Source Code for this video may be found here: https://github.com/tensor-programming/utopian-rocks-demo/tree/tensor-programming-patch-1
Video Tutorial
Curriculum
Related Videos
- Advanced Flutter Project - Setting Up the Basic Structure - Part One
- Advanced Flutter Project - Adding a Second BloC - Part Two
Projects and Series
Stand Alone Projects:
- Dart Flutter Cross Platform Chat Application Tutorial
- Building a Temperature Conversion Application using Dart's Flutter Framework
- Managing State with Flutter Flux and Building a Crypto Tracker Application with Dart's Flutter Framework
Building a Calculator
- Building a Calculator Layout using Dart's Flutter Framework
- Finishing our Calculator Application with Dart's Flutter Framework
Movie Searcher Application
- Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 1)
- Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 2)
- Building a Movie Searcher with RxDart and SQLite in Dart's Flutter Framework (Part 3, Final)
Minesweeper Game
- Building a Mine Sweeper Game using Dart's Flutter Framework (Part 1)
- Building a Mine Sweeper Game using Dart's Flutter Framework (Part 2)
- Building a Mine Sweeper Game using Dart's Flutter Framework (Part 3)
- Building a Mine Sweeper Game using Dart's Flutter Framework (Part 4, Final)
Weather Application
- Building a Weather Application with Dart's Flutter Framework (Part 1, Handling Complex JSON with Built Code Generation)
- Building a Weather Application with Dart's Flutter Framework (Part 2, Creating a Repository and Model)
- Building a Weather Application with Dart's Flutter Framework (Part 3, RxCommand (RxDart) and Adding an Inherited Widget)
- Building a Weather Application with Dart's Flutter Framework (Part 4, Using RxWidget to Build a Reactive User Interface)
- Localize and Internationalize Applications with Intl and the Flutter SDK in Dart's Flutter Framework
Redux Todo App
Curriculum
- Building a Multi-Page Application with Dart's Flutter Mobile Framework
- Making Http requests and Using Json in Dart's Flutter Framework
- Building Dynamic Lists with Streams in Dart's Flutter Framework
- Using GridView, Tabs, and Steppers in Dart's Flutter Framework
- Using Global Keys to get State and Validate Input in Dart's Flutter Framework
- The Basics of Animation with Dart's Flutter Framework
- Advanced Physics Based Animations in Dart's Flutter Framework
- Building a Drag and Drop Application with Dart's Flutter Framework
- Building a Hero Animation and an Application Drawer in Dart's Flutter Framework
- Using Inherited Widgets and Gesture Detectors in Dart's Flutter Framework
- Using Gradients, Fractional Offsets, Page Views and Other Widgets in Dart's Flutter Framework
- Making use of Shared Preferences, Flex Widgets and Dismissibles with Dart's Flutter framework
- Using the Different Style Widgets and Properties in Dart's Flutter Framework
- Composing Animations and Chaining Animations in Dart's Flutter Framework
- Building a Countdown Timer with a Custom Painter and Animations in Dart's Flutter Framework
- Reading and Writing Data and Files with Path Provider using Dart's Flutter Framework
- Exploring Webviews and the Url Launcher Plugin in Dart's Flutter Framework
- Adding a Real-time Database to a Flutter application with Firebase
- Building a List in Redux with Dart's Flutter Framework
- Managing State with the Scoped Model Pattern in Dart's Flutter Framework
- Authenticating Guest Users for Firebase using Dart's Flutter Framework
- How to Monetize Your Flutter Applications Using Admob
- Using Geolocator to Communicate with the GPS and Build a Map in Dart's Flutter Framework
- Managing the App Life Cycle and the Screen Orientation in Dart's Flutter Framework
- Making use of General Utility Libraries for Dart's Flutter Framework
- Interfacing with Websockets and Streams in Dart's Flutter Framework
- Playing Local, Network and YouTube Videos with the Video Player Plugin in Dart's Flutter Framework
- Building Custom Scroll Physics and Simulations with Dart's Flutter Framework
- Making Dynamic Layouts with Slivers in Dart's Flutter Framework
- Building a Sketch Application by using Custom Painters in Dart's Flutter Framework
- Using Dart Isolates, Dependency Injection and Future Builders in Dart's Flutter Framework
- Looking at the Main Features of the Beta Three Release of Dart's Flutter Framework
Hi @Tensor,
Thank you again for your contribution to the video -tutorial category.
Again your tutorial here is highly informative and educational.
The way you teach is very enjoyable because I hear a flow that comes in your style which help learners to grasp concepts easily from one step to another.
The outline that you give in the post is excellent.
I look forward to your next contribution.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
As always, thanks for moderating my contribution.
Thank you for your review, @rosatravels! Keep up the good work!
Thank you utopian
Posted using Partiko Android
Awesome man, been watching your tutorials on youtube, I'm glad you're on steem!!
Thank you, I quite like steem and I mostly use the Utopian platform and work with the steemstem group.
This story was recommended by Steeve to its users and upvoted by one or more of them.
Check @steeveapp to learn more about Steeve, an AI-powered Steem interface.
Hi, @tensor!
You just got a 8.37% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
This post has been voted on by the SteemSTEM curation team and voting trail in collaboration with @curie.
If you appreciate the work we are doing then consider voting both projects for witness by selecting stem.witness and curie!
For additional information please join us on the SteemSTEM discord and to get to know the rest of the community!
Hi @tensor!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @tensor!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Congratulations @tensor! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click here to view your Board of Honor
If you no longer want to receive notifications, reply to this comment with the word
STOP