Learn Basic Python Programming EP.6. - Arrays & File Operations
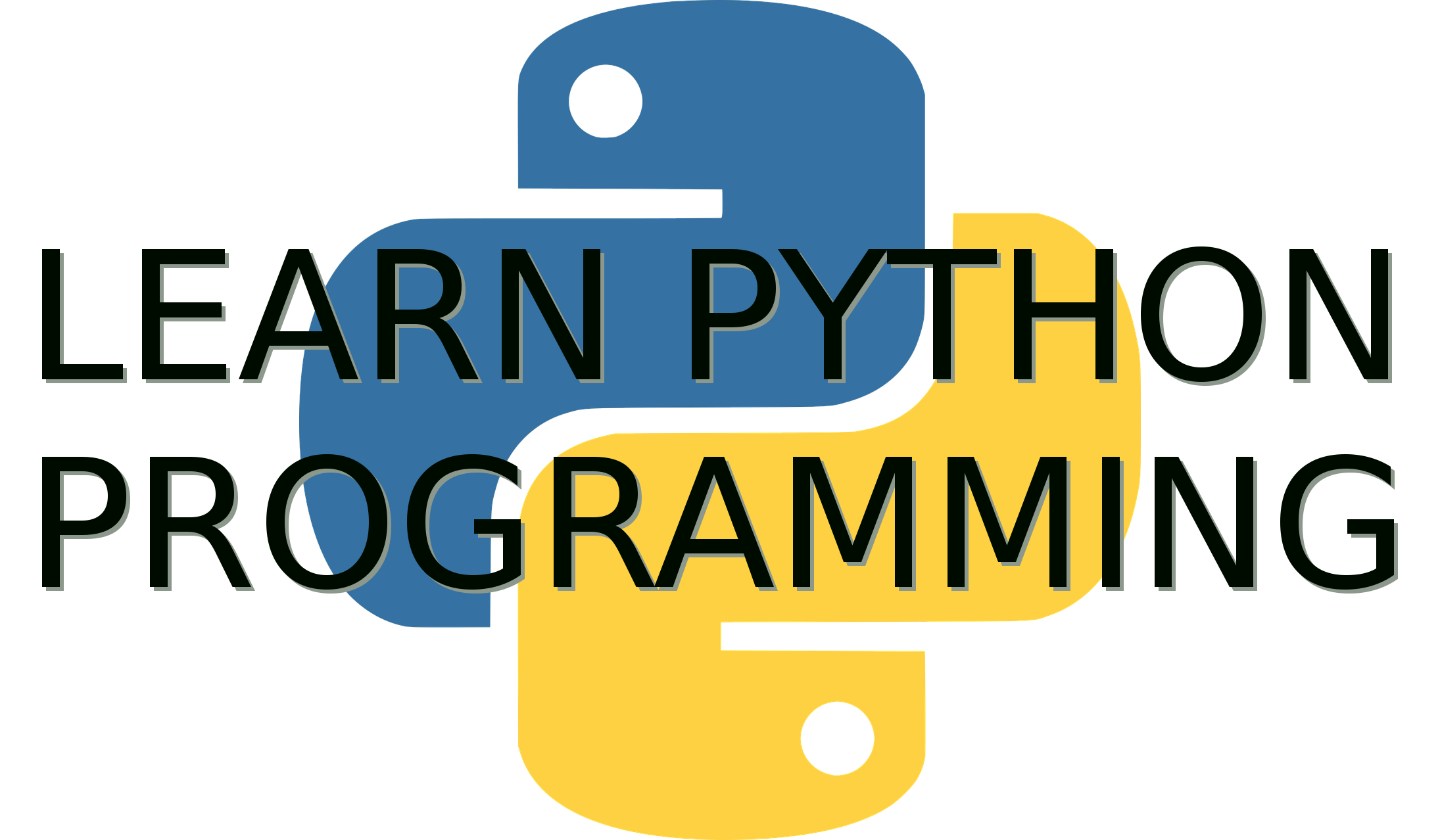
Maybe I dived too deeply into advanced stuff the last part, but I just wanted to show you how powerful Python is and you can code basically anything with it. Some tools can be extremely useful especially for crypytocurrency holders. You can even build a trading bot for Poloniex and Bittrex in Python, using their API system, it would be a piece of cake. It would be harder to just just make it profitable, but the code itself can be easily made.
Ok but let's go back to novice level and let me teach you more basic things like arrays and file operations. An array is like a variable that has multiple elements but each of them can be indexed. In Python even a string which is supposed to be an unitary variable can be indexed, but an array is specifically for indexing.
- An integer variable is like this: 12345
- An array variable is like this [1,2,3,4,5] (where each element has an index, the number 5 will have an index of 4 because the count starts always from 0)
An array can be initialized, which means that it's size is specified:
array_A = [0] *5
This will create an array of size 5, where each element is filled with 0. Of course the array can later be extended if needed.
Or you can create an empty array and fill it up later:
array_B = []
To fill up an array we can use the for
loop which we learned about in EP 3:
for x in range(5):
array_B.append(x)
This will create an array of size 5, and it will fill it up from 0 to 4.
File Operations
Working with file is also easy. There are 2 basic things, reading from a file and writing to a file. For simplicity we will use a plaintext .txt basic file. It will be a simple file just containing 3 hellos in 3 separate lines, like this:
The name of the file is simply hello
or you can name it hello.txt
if that is better:
Now let's read the contents of this file and print it out:
f = open("hello","r")
content = f.read()
f.close()
print (content)
f
is the file variable object, we open it, in the first argument we specify the name hello
or hello.txt
, then the method which is:
r
for readingw
for writingb
for binary operations
Then the content variable will contain all data, as the read()
function reads the entire file into memory. This is simple and easy but it might be problematic for big files (100 MB larger) where streamreading is recommended instead of reading everything in the memory.
It's also very important to close the file after finishing using it, otherwise the file can be corrupted by disk flushing and things like that. So this comment just return the content of the file:
But in this instance, the content variable is just a long string, the entire file is read in like a long string of text. You might want to read it in line-by-line instead and store each line in a separate array element, for that do it like this:
with open("hello","r") as f:
content = f.readlines()
f.close()
print (content[0])
This code will just read in the file line by line, until the end of the file, and here the content
variable is a string array not just a simple string. So by printing out content[0]
we print out only the first line:
If you want to print out the entire file this way you need to iterate through all of it like this:
with open("hello","r") as f:
content = f.readlines()
f.close()
for x in range(len(content)):
print (str(x)+" Line Content:" + content[x])
Where we specify the length of the array in the range with the len()
function, remember it's 3 since we have 3 lines. And then print out them line by line and also add some text before it. We show the x
variable which will count the index of it, which is always -1 of the size of it, since it starts from 0. So the size of the array is 3, but the index of the last element is 2.
That's it, I hope you learned something new, I am telling you these are just the basics, there are many more interesting things you can do with these tools.
Disclaimer: Code licensed under MIT license, use this code at your own risk, I will not be responsible for any losses.
Sources:
https://pixabay.com
Python is a trademark of the Python Software Foundation
Thanks @profitgenerator !!!
No problem my pleasure teaching people about python.
I will always think that learning python programming is a bad choice. It offers a lot of syntactic sugar so you do not learn how to solve programming problems
But you can interact easily with a Linux machine and that is what matters. People should not use Windows anyway due to security and privacy concerns, so if they arleady use Linux they might was well just learn Python programming.
Is is hard to use C on Linux machine? I want to show you example of "syntactic sugar" i mentioned for example plaindrome problem can be solved with python in such manner
str(n) == str(n)[::-1]
Anyway good tutorial voted up:)
Probably I don't know of any other software besides Visual Studio, which doesnt work on Linux I think. I am no programming experty by any means.
I am also not entirely familiar with the string operations either I have to learn more about
''.join
and the:
operations more. There are many characteristic operations to pythong compared to C, in C you have to do everything yourself in a function, so on that respect Python is a higher level programming language, but you can use it on lower level as well.Anything can be done with arrays and loops it's just the basic logic.
I have been learning python until i stoped.I love python and I will love to continue.Is there any e-book you can recommend for easy understanding?Thanks.
I dont know just watch some youtube videos I am sure there are many tutorials out there in a playlist, just learn from there, easier.