Python Client and Server Internet Communication Using UDP
A lot of programming tutorials focus on TCP/IP for networking. That makes sense because it is familiar, it is reliable, and also because if you learn the basics of TCP/IP then you can build web services.
We don't always need a robust connection, however. Sometimes we just need to throw packets around, and if some go astray, no biggie.
Consider multiplayer games, chat rooms, and remote control systems.
This is where the quick and dirty UDP comes in.
UDP stands for User Datagram Protocol. Whereas with TCP creates a robust connection, UDP is connectionLESS. Your UDP server can just sit and wait for any data sent to it over the correct port (arbitrarily chosen) from multiple clients at the same time, you don't even need multiple threads.
Let's see how it works ...
Create a UDP socket with the socket module
We need to specify we want an Internet Datagram DGRAM
import socket
my_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sendto and recvfrom
Sending messages and listening for connections is extremely simple. You don't have to negotiate any handshaking, just throw packets and hope they arrive!
This is the main difference between UDP and TCP. UDP does not stream - data is sent as random, short messages that can arrive out of order or not at all.
Your UDP messages must fit in under 65,507 bytes, and if you need to guarantee delivery then you will need to build that into your own code to check for and resend lost packets.
Sending is as simple as:
socket.sendto("Hello!".encode(), ("10.0.1.2", 9999))
In that command we send the encoded message to 10.0.1.2 on port 9999.
On the receiving end we just do a loop looking for data (specifying the size of the expected message):
data, ip = socket.recvfrom(1024)
print("{}: {}".format(ip, data.decode()))
Basic chat server
On the machine that will act as the server, run the following code:
import socket
# set up the socket using local address
socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
socket.bind(("", 9999))
while 1:
# get the data sent to us
data, ip = socket.recvfrom(1024)
# display
print("{}: {}".format(ip, data.decode(encoding="utf-8").strip()))
# echo back
socket.sendto(data, ip)
It will run forever waiting for messages from anyone using the correct port.
Notice as well as displaying the data received, we also echo it back to show how the communication can be two-way.
Chat client
Your clients will need to know the IP address of the server (in my case a Raspberry Pi). Once you have that you can customize the following to create a simple chat client.
import socket
# create our udp socket
try:
socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
except socket.error:
print("Oops, something went wrong connecting the socket")
exit()
while 1:
message = input("> ")
# encode the message
message = message.encode()
try:
# send the message
socket.sendto(message, ("10.0.1.29", 9999))
# output the response (if any)
data, ip = socket.recvfrom(1024)
print("{}: {}".format(ip, data.decode()))
except socket.error:
print("Error! {}".format(socket.error))
exit()
If the echoing back is annoying just comment out that print statement in the response section.
In a real application you would probably return "ok" and do something if ok was or was not returned within a certain time frame.
What next?
Now that you know the basics, you could create an interactive remote control system, perhaps using your smartphone, Raspberry Pi, or ESP8266
I never gave attention to Python when I was still studying but now I realize how much opportunity I missed. Python is a very useful language. I hope I realized it earlier :)
It's like a swiss army knife :D
Thanks for sharing the knowledge :) tipuvote! 0.2
Very well explained and a clever idea, i will give it a shot.
Thnx for sharing.
best app for view cryptocurrency stats and signals https://usignals.com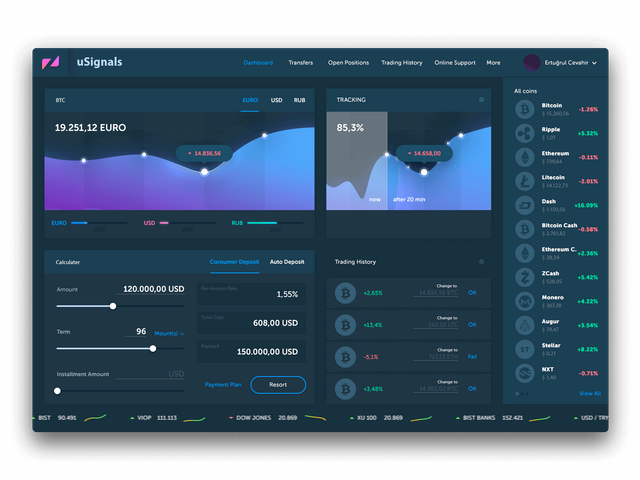
Hi @makerhacks! You have received 0.2 SBD @tipU upvote
from @cardboard !
@tipU pays 100% profit + 50% curation rewards to all investors and allows to automatically reinvest selected part of the payout.
UDP data have to be in order! Thats why it is used in VOIP and live streams for example.
Your voice have to transfered in the right order to be understandable.
A question:
Why do you have to specify the size of the package you want to recieve?
UDP is a stream that has no specified lenght... how should you know how long a phone call takes? or how long a livestream is broadcasted?
As he stated if you need them to arrive in order. You have to write code to check the order when it arrives..