[Tutorial]: Ionic Tutorials; Understanding How to use Providers and injectors
Repository: Ionic App Repository Github
Software Requirements:
Visual Studio Code(Or any preferred code editor)
Chrome(Or any preferred browser)
What you will learn:
In this tutorial you would learn how to live code a real ionic application with practical examples including these major concepts:
- Understanding What Providers Are
- Learning how and When to use providers
- Common errors that can cause errors while using injecatables
Difficulty: Intermediate
Tutorial
Today we'll be doing a tutorial to explain the concept of a sort of functionality in ionic that is called Services or Providers. Understanding these concepts is imperative for better mobile development with ionic and would prevent you from writing lots of redundant code. Today ill try explaining the concepts i found hard when i began development with ionic and it seemed like the solutions for this problems weren't on the internet. Fortunately for my reader today, this would help you use providers with ionic way more easily.
Lets start out with actually creating a provider
To do this we can use the ionic CLI or we could actually copy and paste code from any provider we may have created earlier. The difference between these methods is that in the latter we would have to manually import it into the app.module.ts
file which can be found in our app
folder. Of course when you generate with the CLI, this is done automatically.
So lets get Coding
Head to your command line within you folder and type the following command;
ionic g provider MyFirst //You could also use 'generate' instead of 'g'
Lets see what it would create
Within your src
folder, you should see a folder called providers
. This is where ionic will save all our created providers.
Providers come with a basic format that looks like this
import { HttpClient } from '@angular/common/http';//To enable us send server side requests
import { Injectable } from '@angular/core';
@Injectable()///This shows that this component can be injected into various other components
export class MyFirstProvider {
constructor(public http: HttpClient) {
console.log('Hello MyFirstProvider Provider');
}
}
So if we look in that folder this is what we would see.
I would describe providers as App sized modules. Meaning that they can contain code that will be used repeatedly.
To understand how to use this better, lets edit this provider to do calculations for our application. To keep it simple we will assume that this provider is a calculating provider which carries out the basic add subtract, multiply and divide functions in different pages.
lets write the code.
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable()
export class MyFirstProvider {
constructor(public http: HttpClient) {
}//Here within the constructor we put down some basic functions that we would be using for this provider.
add(a: number,b:number){//simple addition
return a + b
}
subtract(a: number, b: number){//simple subtraction
return a-b
}
multiply(a:number,b:number){//simple multiplication
return a * b
}
divide(a: number, b:number){//simple division
return a/b
}
}
Now before we get to using this within a page lets check out our app.module.ts
file.
import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { IonicStorageModule } from '@ionic/storage';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { ProductsPage } from '../pages/products/products';
import { NewproductPage } from '../pages/newproduct/newproduct';
import { CheckoutPage } from '../pages/checkout/checkout';
import { ProductdetailPage } from '../pages/productdetail/productdetail';
import { CartPage } from '../pages/cart/cart';
import { AboutPage } from '../pages/about/about';
import { ExpandableComponent } from '../components/expandable/expandable';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StoreProvider } from '../providers/store/store';
import { ProfitProvider } from '../providers/profit/profit';
import { MyFirstProvider } from '../providers/my-first/my-first';//This is where the CLI automatically imports our provider
@NgModule({
declarations: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage,
ExpandableComponent
],
imports: [
BrowserModule,
IonicModule.forRoot(MyApp),
IonicStorageModule.forRoot(),
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler},
StoreProvider,
ProfitProvider,
MyFirstProvider//This is where the CLI injects the provider so it can be used anywhere else in the application
]
})
export class AppModule {}
If you look through this you would see that i commented on the lines where our provider is imported and injected. This would be useful if you ever want to copy a provider from maybe another file or you would like to use the instance i mentioned at the beginning of this tutorial.
So now we can add this to any page of our choice. Lets add this to a simple page within the project i'm using to explain this.
Below shows the .ts
file of the page i would like to import this into and how i am going to do this.
import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams } from 'ionic-angular';
import { MyFirstProvider } from '../../providers/my-first/my-first';//First thing you do is to import the provider from the
source document
@IonicPage()
@Component({
selector: 'page-about',
templateUrl: 'about.html',
})
export class AboutPage {
test1: number =2;//Here we define two variables to use to test this out
test2: number = 4;
constructor(public calculate: MyFirstProvider,public navCtrl: NavController, public navParams: NavParams) {/This is where we include it to our constructor
}
ionViewDidLoad() {
console.log('ionViewDidLoad AboutPage');
this.calculate.add(this.test1,this.test2);//Example of use
}
closepage(){
this.navCtrl.pop();
}
}
So the file below show us using a provider in a page but there is quite a bit to take note of.
A common error when using providers in ionic is that when adding it to the constructor we would need to put it as the first element to be constructed. The producers of ionic created it in a way that the constructor evaluates this components based on the order with which they are placed in the constructor. During my early days of development, i tried using a provider and kept getting a Static Injection Error
, which is the common error you would face if you fail to include that first.
If you do everything right you could access any function and any variable within the provider and this should help you to not have to use navParams
to struggle to pass data between pages and components of your application, because this is a way cleaner, easier and better way to manage the data in your application.
You could look for the code for the test app in my Github Repository
See you in the next tutorial
Thank you for your contribution @yalzeee.
After analyzing your tutorial we suggest the following:
Thanks for your work on developing this tutorial. We are waiting for the next tutorial.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 6 contributions. Keep up the good work!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
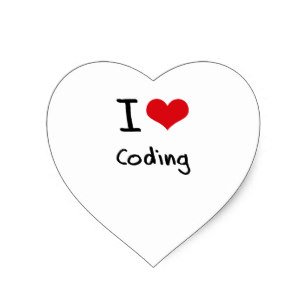
Reply !stop to disable the comment. Thanks!
@yalzeee, go and place your daily vote for Steem on netcoins! http://contest.gonetcoins.com/
Hi @yalzeee!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @yalzeee!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!