[Tutorial]Ionic Tutorials: Making http request and developing a backend for our ionic application( Part 2)
Repository: Ionic App Repository Github
Software Requirements:
Visual Studio Code(Or any preferred code editor)
Chrome(Or any preferred browser)
What you will learn:
In this tutorial you would learn how to live code a real ionic application with practical examples including these major concepts:
- Developing a simple back-end for your application
- Making HTTP requests in ionic
- Managing data with the appropriate database
Difficulty: Intermediate
Tutorial
So this tutorial is a continuation of the last in this series. And if we have a brief recap we would see that we were able to kick-start the development of a server end application for our ionic app. This server side would help us store data on a date base which in this case is MongoDb through HTTP requests made from the application. We were using postman for this tutorial to simulate server side requests, but in this tutorial we would use the ionic application directly and add json packaging.
The first thing we'll be doing today is adding the data base to our server-side.
To do this, we'll be using mlab. Quickly Click this link and set up a free account to use to manage your database.
Next thing you'll do is create a new database
When you create this database copy the url
![mlab 2.png]
()
That is the url below.
After this, create a new folder in the root directory of your folder and create a file called db.js
In this file link your created database like this
module.exports = {
url : YOUR URL HERE
};
Note: Replace the username and password areas with the username
and password
you used to create the mongoDb account.
You then update your server.js file to include the mongoDb database were adding
const express = require('express');//All our earlier requirements
const MongoClient = require('mongodb').MongoClient;
const bodyParser = require('body-parser');
const db = require('./config/db');//This is the line that shows where we are referring to the database from.
const app = express();
const port = 8000;
app.use(bodyParser.urlencoded({ extended: true }));
MongoClient.connect(db.url, (err, database) => {
if (err) return console.log(err)
require('./app/routes')(app, database);
app.listen(port, () => {
console.log('We are live on ' + port);
});
})
So lets analyze the code that helps us connect
MongoClient.connect(db.url, (err, database) => {//Here we create a database and use the database perculiar to our mongoDb url.
if (err) return console.log(err)
require('./app/routes')(app, database);
app.listen(port, () => {
console.log('We are live on ' + port);
});
Add this piece of code within your code if you are using a more recent version of mongoDb, which would be <3.0.0.
Now we can go ahead to creating a collection.
Collections are units of storage which mongoDb uses to store data in a adequately managed form that is easily accessible.
To create a new note which we would be adding to our database we will use the post
method.
Since i am creating an app that deals with business transactions and stores the data from stores, i will make a collection called stores
.
So let me start with a post method.
The idea is that whenever you send a post request with '/stores', it will add a new store to the store database.
Lets see how to do this.
module.exports = function(app, db) {
const collection =
app.post('/newStore', (req, res) => {
const note = { text: req.body.body, title: req.body.title };//Well send requests where the title is the name of the store and the body is the contents of the store
db.collection('store').insert(store, (err, result) => {
if (err) { //We also need to check for errors and log them to our console.
res.send({ 'error': 'An error has occurred' });
} else {
res.send(result.ops[0]);
}
});
});
};
So now lets try using this from our ionic services.
The first thing we'll do is run our server.
To do this head to your command line and run
npm run dev
This would run a development server and be ready to listen to any requests from our app.
So head to the provider for your app and lets code
In our store.ts
file, we will make our requests using the code below
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable()
export class StoreProvider {
store: string;
constructor(public http: HttpClient) {
this.store = 'My Store';
}
createNewUser(){
this.http.post(
'localhost:8000,
{userDetails}//This would be an object containing the array of the details collected which are required to create a new user
{}//At this point we would not send any header.
}
login(){
//This is where we would send a request to get the id for this particular user
}
//Ignore this function for now
/*addToStore(item){
this.http.put(/This is how we would send the request to add a product to this user
'localhost:8000/createStore',
{item},
{}
)*/
}
}
So as seen above we have created the first function in our service to create a new user.
You could try this out by importing this service into a dummy page and creating a simple interface that collects some data.
When you do this create a button that will trigger this service and pass data to your database.
To import a service into a page, simply;
import { StoreProvider} from '..providers/stroreprovider'
constructor(public store: StoreProvider){
addToStore(){
//use it here like this
this.store.createNewUser
}
So thats it for today,
You can see the prototype document i am using to create this app from my repository
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
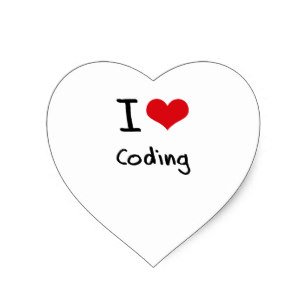
Reply !stop to disable the comment. Thanks!
As a follower of @followforupvotes this post has been randomly selected and upvoted! Enjoy your upvote and have a great day!
Hello! I find your post valuable for the wafrica community! Thanks for the great post! We encourage and support quality contents and projects from the West African region.
Do you have a suggestion, concern or want to appear as a guest author on WAfrica, join our discord server and discuss with a member of our curation team.
Don't forget to join us every Sunday by 20:30GMT for our Sunday WAFRO party on our discord channel. Thank you.
Hi @yalzeee!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @yalzeee!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!