[Tutorial] Ionic Framework Tutorials.(Creating you own custom components for all page types)
image source
Repository: Ionic Github Repository
Software Requirements:
Visual Studio Code(Or any preferred code editor)
What you will learn:
In this tutorial you would learn to build a custom component in ionic divided into these steps
- Understand What a custom component is
- Understanding how to use custom components in normal and lazy loaded pages
- How to send data from your template to your custom component
Difficulty: Intermediate
Tutorial
Todays tutorial is going to be dealing with how to use custom components in ionic.
Firstly What are custom components?
To understand this you have to know that the ionic framework is built on components. Everything from the pages, to the headers,footers and tabs are components. Components are basically reusable templates. So what is a custom component? A custom component as the name implies is a component made by a user which isn't part of the preset component list.
If you still don't understand this just keep following and things should get clearer as we go on.
Start up a new ionic application using
ionic start understanding-components blank
There wouldn't be need for any imports since this is a tutorial. So hop into the terminal and generate a component using the following command.
ionic g component myFirst
This would generate a component called 'Myfirst'.
If you hop into your app.module.ts
you would see that this would be automatically added to the imports and declarations.
This is the app.module.ts
file for a project with an expandable header component.
import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { IonicStorageModule } from '@ionic/storage';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { ProductsPage } from '../pages/products/products';
import { NewproductPage } from '../pages/newproduct/newproduct';
import { CheckoutPage } from '../pages/checkout/checkout';
import { ProductdetailPage } from '../pages/productdetail/productdetail';
import { CartPage } from '../pages/cart/cart';
import { AboutPage } from '../pages/about/about';
import { ProfitPage } from '../pages/profit/profit';
import { ExpandableComponent } from '../components/expandable/expandable';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StoreProvider } from '../providers/store/store';
import { ProfitProvider } from '../providers/profit/profit';
import { BusinesstipsProvider } from '../providers/businesstips/businesstips';
import { TransactionsProvider } from '../providers/transactions/transactions';
import { AuthServiceProvider } from '../providers/auth-service/auth-service';
@NgModule({
declarations: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage,
ProfitPage,
ExpandableComponent//It is stated here as a declaration
],
imports: [
BrowserModule,
IonicModule.forRoot(MyApp, {
tabsHideOnSubPages: true,
}
),
IonicStorageModule.forRoot(),
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage,
ProfitPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler},
StoreProvider,
ProfitProvider,
BusinesstipsProvider,
TransactionsProvider,
AuthServiceProvider,
]
})
export class AppModule {}
Understanding how to use custom components in normal and lazy loaded pages
From what we have done above, the component we created can be used in a normal page but not a lazy loaded page.
If you do not understand what a lazy loaded page is
A lazy loaded page is a page that is created at the instance of when it is needed and destroyed after that.This is done so that the application when compiled won't be too heavy and will function faster and smoother. Lazy loaded pages are recommended and generally better.
So lets get to how to use custom components in lazy loaded pages. In your directory structure, if you navigate to the path below
../app/component
You should see that a file called components.ts
was created. This file is imperative to using components in lazy loaded pages. Since lazy loaded pages have their own module, to use them you have to carry out two steps
- You have to import the
components.ts
file to yourapp.module.ts
. - You have to import the module into any lazy loaded page you would want to use the component in.
For instance, lets see our app.module when we want to use lazy loading within. See comments within code
import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { IonicStorageModule } from '@ionic/storage';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { ProductsPage } from '../pages/products/products';
import { NewproductPage } from '../pages/newproduct/newproduct';
import { CheckoutPage } from '../pages/checkout/checkout';
import { ProductdetailPage } from '../pages/productdetail/productdetail';
import { CartPage } from '../pages/cart/cart';
import { AboutPage } from '../pages/about/about';
import { ProfitPage } from '../pages/profit/profit';
import { ComponentsModule } from '../components.';//This is where we import the components module from the components folder.
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StoreProvider } from '../providers/store/store';
import { ProfitProvider } from '../providers/profit/profit';
import { BusinesstipsProvider } from '../providers/businesstips/businesstips';
import { TransactionsProvider } from '../providers/transactions/transactions';
import { AuthServiceProvider } from '../providers/auth-service/auth-service';
@NgModule({
declarations: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage,
ProfitPage,
],
imports: [
BrowserModule,
ComponentsModule,//Here you add the module as part of the imports
IonicModule.forRoot(MyApp, {
tabsHideOnSubPages: true,
}
),
IonicStorageModule.forRoot(),
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
ContactPage,
HomePage,
TabsPage,
ProductsPage,
NewproductPage,
CheckoutPage,
ProductdetailPage,
CartPage,
AboutPage,
ProfitPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler},
StoreProvider,
ProfitProvider,
BusinesstipsProvider,
TransactionsProvider,
AuthServiceProvider,
]
})
export class AppModule {}
Now lets use that component in a lazy loaded page
In the pages (pagename).module.ts
file, simply import the module and add it to the imports
import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { ProfitPage } from './profit';
import { ComponentsModule } from '../../components'
@NgModule({
declarations: [
ProfitPage,
],
imports: [
IonicPageModule.forChild(ProfitPage),
ComponentsModule
],
})
export class ProfitPageModule {}
And now you could use this component within this page.
How to send data from your template to your component
To use the component, you would need to use tags from the components name. For example this expandable header component i created can be used in my pages with the expandable
tag. The file below is a template for a created template.
import { Component, Input, ElementRef, Renderer } from '@angular/core';
@Component({
selector: 'expandable',
templateUrl: 'expandable.html'
})
So now lets use this in a page.
<ion-header>
<expandable [scrollArea]="mycontent" headerHeight="120">/These are the tags were using to indicate the component were using.
<h1 text-center class="profitmade">This Weeks Profit</h1>
<h2 text-center class="weeksProfit">{{ profitThisWeek }}</h2>
</expandable>
</ion-header>
<ion-content fullscreen padding:10px class="background" #mycontent>//This helps us send events that happen on the screen to the component
Notice the hash tags those links the areas that they are in to be linked to the components template. So we cant search form scroll and even click events within that area.
For now every thing within the component will not show so head to the components .html
file and add this within the content.
<div #contentWrapper><ng-content #expandable></ng-content></div>
The ng-content
within helps whatever content that is its parent file be displayed in the template.
So hope this helps
This is an image showing the result of creating this custom component
As an ionic developer they are various other components free for use and you could see some in Github
Thanks for reading
See you in the next
Thank you for your contribution. Below is our feedback:
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @mcfarhat! Keep up the good work!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
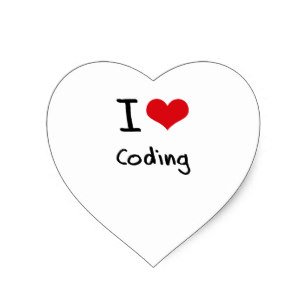
Reply !stop to disable the comment. Thanks!
Hello! I find your post valuable for the wafrica community! Thanks for the great post! We encourage and support quality contents and projects from the West African region.
Do you have a suggestion, concern or want to appear as a guest author on WAfrica, join our discord server and discuss with a member of our curation team.
Don't forget to join us every Sunday by 20:30GMT for our Sunday WAFRO party on our discord channel. Thank you.
As a follower of @followforupvotes this post has been randomly selected and upvoted! Enjoy your upvote and have a great day!