[Tutorial]: Ionic App Development: Building the Business app part 5
Repository: Ionic Framework Github Repository
Software Requirements: Visual Studio Code(Or any preferred editor)
A browser(Preferably Chrome)
What you will learn:
Going deeper into how events work
Designing a system to determine what most profitable goods.
Updating our balance property throughout our app.
Difficulty: Intermediate
Tutorial
So we have come quite a distance through our application development. And at this point this is how it looks.
Our Gui is looks good but we have a lot of functionality to add. First thing first, i will be going through how to keep our balance on the homepage updated at all times. And to do this we'll be using a events
. We started off with events last week but i did not go deeply into explaining how these events can actually work for a purpose in our application.
Events are ways of noting change or an update in data and broadcasting that change throughout our app. Note that events work both ways so an event broadcast from one point in the app can be modified from even another page so you should use them only when necessary. Here are a few conditions where they should be used.
- When the data is sensitive and affects every thing about the view: For example a login app. When a user inputs a username you would like to use that username to customize everything about what the user see. That username would determine the avatar that would be displayed(if any) and other friendly messages which would include the users name.
So lets get coding
When the user adds a new product from the product page you would want the price of that product to add to the overall balance of the app. To do that we have to :
product.ts
import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams, ModalController, Events } from 'ionic-angular';
import { NewproductPage } from '../newproduct/newproduct';
import { Storage } from '@ionic/storage';
@IonicPage()
@Component({
selector: 'page-products',
templateUrl: 'products.html',
})
export class ProductsPage {
public items:any=[];
public balance: number = 0;//Here we create a new variable called balance
constructor(public navCtrl: NavController, public navParams: NavParams, public modalCtrl: ModalController, public events: Events, public storage: Storage) {
}
createNewProduct(){
let newItemModal = this.modalCtrl.create(NewproductPage);
newItemModal.onDidDismiss((data) => {
if(data.name){
this.items.unshift(data);
this.balance = this.balance + parseInt(data.sellingprice);//Whenever new data is input the balance is updated
let balance = this.balance;
this.events.publish('balance',balance);//The balance is published so it can be read from any point in the application
If you observe, you will see that anytime we create a new product it adds the data collected to the balance
and publishes this event like this
let balance = this.balance;
this.events.publish('balance',balance);
This will publish this event through the whole application and you can subscribe to it everywhere. Lets go to the home page and subscribe to this event.
So navigate to the home.ts
Our code will look like this
import { Component } from '@angular/core';
import { NavController, Events } from 'ionic-angular';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
constructor(public navCtrl: NavController, public events: Events) {
}
ionViewDidLoad() {//This function runs whenever our ion view loads
this.events.subscribe('balance',(balance)=>{//This is where and how we suscribe to that event.
this.balance = balance;//this changes the balance variable on this page
})
So now we subscribed to that event and whenever it changes we it will be updated to our home screen. Lets test it out.
Head to this page in your testing tab in your browser and add a new product.
And watch how it adds this new products price updates to our apps home page.
And so it works perfectly.
So the next thing we would want to do is determine what goods are most profitable and display this on the home screen, and to do this we would have to calculate the profit on every good.
Head to your newproducts.ts
and add this code.
newproduct.ts
//Add another property to the products properties called the profit which calculates the profit made on each unit of good.
//Add another still that calculates the profit made for the whole stock
import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams, ViewController } from 'ionic-angular';
@IonicPage()
@Component({
selector: 'page-newproduct',
templateUrl: 'newproduct.html',
})
export class NewproductPage {
product: any={name: "",type: "",costprice: null,sellingprice:null,numOfGoods: null, profit: (this.product.sellingprice - this.product.costprice)* this.product.numOfGoods }
So now we can calculate the profit made on each good.
In the next tutorial we will implement our trending good section which will help compute which good is trending in the store, and also learn how to use storage
in ionic.
You can find my code in Github
Thank you for your contribution.
Below is our feedback:
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
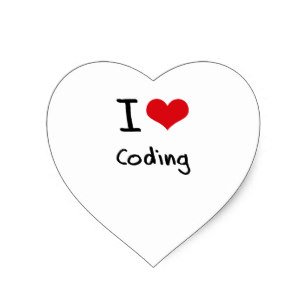
Reply !stop to disable the comment. Thanks!
Hello! I find your post valuable for the wafrica community! Thanks for the great post! We encourage and support quality contents and projects from the West African region.
Do you have a suggestion, concern or want to appear as a guest author on WAfrica, join our discord server and discuss with a member of our curation team.
Don't forget to join us every Sunday by 20:30GMT for our Sunday WAFRO party on our discord channel. Thank you.
As a follower of @followforupvotes this post has been randomly selected and upvoted! Enjoy your upvote and have a great day!
Hi @yalzeee!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server