Function generation in Eclipse by using Java
What Will I Learn?
Greetings, the aim of this tutorial is to show java programming language to transform tasks into functions for a simple, algorithmic computer based solutions. In order to do that we will use a software called Eclipse Java Oxygen capable of compiling and running java language.
- You will learn the basics of Eclipse Java ide,
- You will learn algorithm and function generation,
- You will learn method,class and void implementation in Java.
Requirements
- Eclipse IDE for java developers,
- Interest on coding and applications,
- Basic knowledge on Java.
Difficulty
- Intermediate
Tutorial Contents
To begin with, the task we will acomplish in today's lecture will be writing a Java program ATM.java that computes the total number of banknotes that an ATM should give. First, the program reads the total amount of money to withdraw. Then, it computes the minimum total number of banknotes to be given. At the moment, ATM has three types of banknotes: $1, $5 and, $20. In the end the sample program output will look like this,
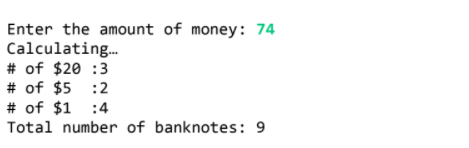
To do that we first call,define the function. For simplicity, the name of the function is picked as q1 representing question number one and a class formed by using this name,
public class q1
Then secondly, to get the user entered value, letter, number or any kind input we must use another pre defined library in java called scanner. That library is defined in the beginnig of the code to be called when user enters the desired input,
import java.util.Scanner
Then we must define a main part for our function where the operation takes place. To do that we must use static void main string which is capable of generating functions and constructors however it can not create or generate a main object. To use it simply below code was written,
public static void main(String[] args)
Now we need to implement our function. We must consider our problem again and translate it into algorithmic, java based structure. It is quite obvious that we should first get the value that user enters and then we need to work on it. Thereby the very first stage we need to do is to ask user enter an amount of money,
int money;
Scanner keyboard =new Scanner (System.in);
System.out.println("Enter the amount of money: ");
In the above code int money defines the money integer and says that 'money' can get any value. Second line enables scanner function of utill.Scanner and lets the user use scanner function whenevre a user input is necessary. Third and last line prompt a message "Enter the amount of money: " where the user understands that he should enter a number, preciselly integer. Then we need to take the user entered integer to do that below code was written,
money=keyboard.nextInt();
Now we know that money is equal to the integer that user enteredf. Now we need to procced, work on this number to divide it into twenty, five and one usd banknotes. Initially a calculation prompt message was written to let user know that the system is calculating. This is pretty useful when the user enters relatively huge number and the system has difficulties of calculating it.
System.out.println("Calculating...");
Now we need to define integers, values for banknotes. To do that we must first focus on 20$ since it is the highest amount of number and rest could be arranged according to the remainder when money divided to 20$. To begin we defined our integers as follow,
int x,y,z,a,b;
Now we need to do calculations with these integers. The first variable x will be used to divide the use entered value to twenty,
x=money/20;
Second variable y will be used to get the remainder when the main money divided to twenty.
y=money%20;
Then by looking the remainder we may divide it to five to get the amount of banknotes having 5$sign,
z=y/5;
Fourth variable will be the remainder when y divided to 5.
a=y%5;
Now we need to sum a, x and z to get the total amount of banknotes. To do that another variable 'b' was used,
b=a+x+z;
Finally we can know print the overall results by using a pre defined java function system.out.println,
System.out.println("# of $20: " +x);
System.out.println("# of $5 : " +z);
System.out.println("# of $1 : " +a);
System.out.println("Total number of banknotes: " +b);
So in overall our code became,
import java.util.Scanner;
public class q1 {
public static void main(String[] args) {
int money;
Scanner keyboard =new Scanner (System.in);
System.out.println("Enter the amount of money: ");
money=keyboard.nextInt();
System.out.println("Calculating...");
int x,y,z,a,b;
x=money/20;
y=money%20;
z=y/5;
a=y%5;
b=a+x+z;
System.out.println("# of $20: " +x);
System.out.println("# of $5 : " +z);
System.out.println("# of $1 : " +a);
System.out.println("Total number of banknotes: " +b);
}
}
To test the code we may simply run it with the top bar green play icon in Eclipse. Below results were obtained once the program was runned.
In our next tutorail we will extend this output for double entered numbers, for other banknotes and begin to apply statements.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @gerginho, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Hey @wodsuz I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x