Creating a graphical user interface in Python - Tkinter Part 1
Creating a graphical user interface in Python - Tkinter Part 1
Repository
https://github.com/python/cpython
What Will I Learn?
- Import from Tkinter
- Create a basic window
- Understand Marcos
- Create a top and bottom frame
- Design of a window
- Geometry Manager Pack
Requirements
You must have knowledge about the Python syntax.
- A PC/laptop with any Operating system such as Linux, Mac OSX, Windows OS.
Note: This tutorial is performed in Sublime Text 3 in laptop with Windows 7, 32 bit OS, Python 3.6.4.
Difficulty
- Intermediate
Creating a graphical user interface in Python - Tkinter Part 1
Welcome to the first part of the tutorial on how to create graphical interfaces with Tkinter in Python. The main objective of this tutorial is to introduce the developer who is not familiar with the programming of GUI in Tkinter. For this, we will follow a series of examples that show, in a progressive way, the use of the necessary elements to build a graphic application: windows, geometry managers, widgets, menus, event management, sources, styles and themes.
Let's start!
Tkinter
Tkinter is a Python programming language library oriented to the graphical interface for desktop applications. Tkinter comes installed by default in Python, so it is not necessary to install it.
Create a basic window
A window is the fundamental element of a graphic user interface application. It is the first step that is given and on this the rest of the objects is realized.
To import the library we use from tkinter import *
from tkinter import * #import the library
root = Tk () #Create the main window
root.mainloop () # Prevents the program from closing
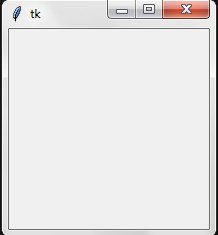
With
root = Tk ()
we assign to the variableroot
the most basic TkinterTk ()
function that generates a window.root.mainLoop ()
is the main loop and performs the function of constantly keeping the window on our desktop.
Modifying the window
In addition to Tk (), we can declare more variables to modify our window, such as:
config(bg=" ")
: Coloring the interior of the windowgeometry("NxN")
: Change the size of the window.title(" ")
: Assign a title to the window.Button( )
: Place a button in the windowLabel( )
: Place a texticonbitmap('.ico')
: Place icon to the windowToplevel( )
: Create a new windowFrame ( )
: Place the panels to sort the elementsCanvas ( )
: To draw and graph functions etcEntry ( )
: Place a text entry of a lineText ( )
: Place a text entry of several linesListbox ( )
: Place a list with clickable elementsMenu ( )
: Places a menu that can contain waterfalls and clickable elementsMessage ( )
: Place a text
For example:
from tkinter import * #We import the library
root = Tk() #We create the main window
root.title('Application') #Assign a title to the window
root.config(bg="black") #Coloring the interior of the window
root.geometry('300x300') #Change the size of the window
root.iconbitmap('favicon.ico')
root.mainloop() #Prevent the program from closing
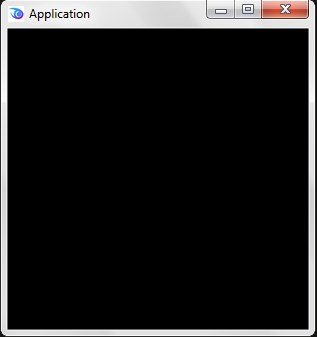
Designing graphic windows for a mini application
To define how objects should be placed within a window, geometry managers are used. Tkinter manages several geometry managers, among them are: package, grid and place. When an application has several windows, the geometry managers are used, each of them can be built with any of them.
When we create windows, widgets are used, such as frames, panels, among others, that act as containers for other widgets. These widgets are used to group several controls that fade the operation to the users.
Next, we will know the characteristics of the geometric manager Pack with an application.
The application to be developed consists basically of a typical window to access a system that shows the current user account of the computer in an entry box and presents another box to enter your password.
The Geometry Manager Pack
With this manager the organization of the widgets is done taking into account the sides of a window: up, down, right and left.
If there are several controls located on the top or on the left side of a window, we will build a vertical bar or a horizontal bar of controls. Although it is ideal for simple designs, it can also be used with complex designs.
We call the libraries to use in our project
from tkinter import *
from tkinter import ttk, font
import getpass
We will work with an object called application class Application ():
Initialize our attributes of the objects that we created in the init
method
def __init__(self):
self.root = Tk()
self.root.title("Acces")
We change the format of the letter with the Font
module that we import. source=font.Font(weight='bold')
We define the labels that accompany the input boxes and assign the previous font format:
self.etiq1 = ttk.Label(self.root, text="User:", font=source)
self.etiq2 = ttk.Label(self.root, text="Password:", font=source)
We declare two variables of type string to contain the user and the password:
self.user1 = StringVar()
self.key = StringVar()
We read the name of the user who initiates the session in the system and assign it to the variable self.user1
.
self.user1.set(getpass.getuser())
We define two input boxes that will accept strings with a maximum length of 30 characters.
- In the first one of them
'self.ctext1'
that will contain the name of the user, the variable'self.user1'
is assigned to the option'textvariable'
. Any value that the variable takes during program execution will be reflected in the input box. - In the second entry box, it will be the password, the same is done. In addition, the option
'show'
is established with an"*"
to hide the writing of the passwords:
self.ctext1 = ttk.Entry(self.root, textvariable=self.user1, width=30)
self.ctext2 = ttk.Entry(self.root, textvariable=self.key, width=30, show="*")
self.separ1 = ttk.Separator(self.root, orient=HORIZONTAL)
Two buttons are defined with two methods: The 'Accept'
button will call the 'self.accept'
method when pressed to validate the password; and the 'Cancel'
button will terminate the application if it happens to press:
self.button1 = ttk.Button(self.root, text="Accept", command=self.acept1)
self.button2 = ttk.Button(self.root, text="Cancel", command=quit)
The positions of the widgets within the window are defined. All controls are placed on the upper side, except for the last two buttons, which will be placed below the last "TOP"
: the first button on the left side and the second on the right.
self.etiq1.pack(side=TOP, fill=BOTH, expand=True, padx=5, pady=5)
self.ctext1.pack(side=TOP, fill=X, expand=True, padx=5, pady=5)
self.etiq2.pack(side=TOP, fill=BOTH, expand=True, padx=5, pady=5)
self.ctext2.pack(side=TOP, fill=X, expand=True, padx=5, pady=5)
self.separ1.pack(side=TOP, fill=BOTH, expand=True, padx=5, pady=5)
self.button1.pack(side=LEFT, fill=BOTH, expand=True, padx=5, pady=5)
self.button2.pack(side=RIGHT, fill=BOTH, expand=True, padx=5, pady=5)
The 'padx'
and 'pady'
options are used to add extra horizontal and / or vertical external space to the widgets to separate them from each other and from the edges of the window. There are other equivalents that add extra internal space: 'ipadx'
and 'ipady'
.
We place so that when starting the program the focus is assigned to the password entry box so that you can start writing directly:
self.ctext2.focus_set()
self.root.mainloop()
The 'accept1'
method will be used to validate the entered password. It will be called when the 'OK'
button is pressed. If the password matches the string 'utopian'
the message 'Access allowed'
and the accepted values will be printed. Otherwise, the message 'Access Denied'
will be displayed and the focus will return to the same place.
def acept1(self):
if self.key.get() == 'utopian':
print("Access allowed")
print("User: ", self.ctext1.get())
print("Password:", self.ctext2.get())
else:
print("Access denied")
Finally the variable 'self.key'
is initialized so that the widget 'self.ctext2'
is clean, the focus is reassigned to this widget in order to write a new password.
self.key.set("")
self.ctext2.focus_set()
def main():
mi_app = Application()
return 0
if __name__ == '__main__':
main()
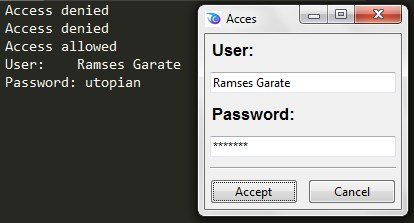
What did we learn?
This first episode was thought of as an introduction to the Python Tkinter library, which allows to create graphic interfaces in a simple way. We saw the basic syntax of Tkinter and created a mini-project of user authentication.
For the second part we will work with another Tkinter project, where we will elaborate the graphic interface of a calculator.
Thanks for your time!
Proof of Work Done
https://github.com/CerebroArtificial/tkinter/blob/master/tkinter_part1.md
@vidayaventura
I like your post but you did'nt give the link to your
So utopian can see your works at github.
You can find templates from here to how to link Our Github account in post.
I already put the link to my GitHub account
you have to put in all posts
Thanks, I will do it
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thanks @portugalcoin, I will improve in my next publications.
Hey @vidayaventura
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Excellent work. A very well explained tutorial.