Building a Basic RPC Server and Client with Go
Repository
What Will I Learn?
- You will learn about RPC (Remote Procedure Calls)
- You will learn how to build an RPC server
- You will learn how to build an RPC client
- You will learn how to create a CRUD app with Go
- You will learn how to use the net/rpc and net/http libraries in Go
Requirements
System Requirements:
Operating System:
- FreeBSD 10.3 or later
- Linux 2.6.23 or later with glibc
- macOS 10.10 or later
- Windows 7, Server 2008R2 or later
Required Knowledge
- An understanding of APIs
- A basic understanding of Go
- Some understanding of micro service architecture.
Resources for Go and this Project:
- Awesome Go Github: https://github.com/avelino/awesome-go
- Golang Installation Page: https://golang.org/dl/
- Golang Home Page: https://golang.org/
- Golang Documentation Page: https://golang.org/doc/
Credits/Sources:
- Go Logo: https://golang.org/
Difficulty
- Advanced
Description
In this Go video tutorial, we work at building a basic RPC Server and Client. This includes building the basic app without using the RPC library and then building both the Client and Server applications to showcase some of the basic ideas of RPC with Go. The RPC format is talked about at length and shown off using a CRUD application. This tutorial is in service of being able to talk about Microservices, gRPC and Protobuf.
Building a Basic CRUD Application in Go
Before adding the RPC logic to the application, we need to scaffold out the basic features of the service. In this case, that means creating a CRUD application. CRUD stands for create, read, update, and delete as those are the basic operations that we want to perform on the data. For the data in this application, we create a simple slice data structure which is used in lieu of a Database. This slice of the custom item type is just positioned as a global variable in the main CRUD and RPC server application.
In the above image, we have code that shows the application using the basic CRUD operations. First, we create a few items which will serve as the data that we want to manipulate. We then add that data to our "database" structure using the AddItem
function; this is the Create operation. We then are able to print out the state of this new Database to show the items inside of it. The DeleteItem
function is run to remove a item from the Database; this is the Delete operation. We then are able to modify an item using the EditItem
function; the Edit operation. And finally, we are able to grab items from the database by name using GetByName
; the Read operation.
Creating an RPC Server and Client
The basic idea of an RPC interface is to be able to call functions remotely as though they were local to the client application. In other words, unlike a standard REST API, an RPC doesn't require us to serialize and deserialize data to and from an intermediate datatype like JSON or XML. We don't need to worry about API endpoints and Domain Specific Languages with an RPC.
In Go, the net/rpc
library requires that the functions in the RPC interface follow a specific format. Each function must be a Method on an Exported Datatype. In the case of this application, that Datatype is just a simple int type Aliased to the name API
. Each of these methods must also be exported globally. The Methods must contain exactly two exported type arguments and the second argument must be a pointer. And the Methods must return an error type.
Above is an image containing the GetByName
RPC method. The method is attached to the API
type and it has an uppercase first letter which exports it globally. It also contains two arguments, a title string and a reply pointer Item type. The method also just returns an error type. This method can be called from an RPC client because it fits all of the RPC criteria. The RPC client passes in a string for the title that it wants to read from the database and a pointer to an Item type. This pointer is then used to populate the return type in the RPC client.
The Source Code for this video may be found here: https://github.com/tensor-programming/go-basic-rpc
Hello @tensor
Great to see you doing another set of video tutorials.
I like the way you present your tutorials - always straight to the point. Again, you have made another excellent tutorial for people to learn how to build RPC server and client. It doesn't seem too difficult. I appreciate the detail you put in the written tutorial there too.
Both audio and presentation are excellent.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thanks as always @rosatravels. I am very excited for this series. Microservices and gRPC are great technologies and I've been wanting to cover them for almost a year now.
Thank you for your review, @rosatravels! Keep up the good work!
Hi, @tensor!
You just got a 5.14% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 13 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 4 SBD worth and should receive 89 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
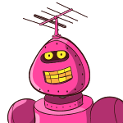
TrufflePig
Hi @tensor!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @tensor!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!