Node.js testing with assert library
Node has a build in testing with a library called 'assert'. In my previous project, I started to do some Test-Driven Development (TDD) approach with raw node.js. And then, I came accross with 'assert' that is build in with Node.js and it turns out that the API is easy to get started.
What Will I Learn?
- Basic Test-Driven Development (TDD) in Node.js
- Using of assert library which is build in library of Node.js
Requirements
- Node.js
- Node.js REPL
Difficulty
Intermediate
Tutorial Contents
'assert' is a light testing library that is build into Node.js. For this tutorial I am using Node v8.9.2 .
One of the thing I learn from Test-Driven Development is that you should always make sure your test code are always bug-free. So, always check few times for the test code.
In REPL mode
Run node
in terminal, and use assert
to see what functions are available.
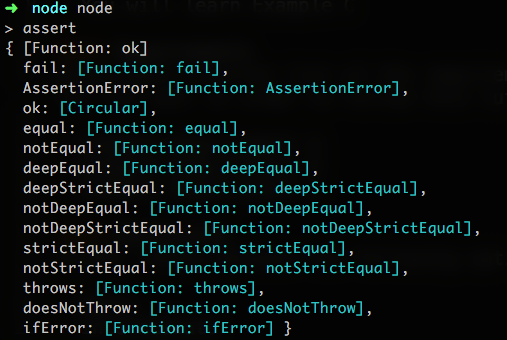
These are the exist function in assert
The reason running on REPL first is to try out how the library works.
The reason behind that you can just type assert
without using something like var assert = require('assert')
is because node.js has included assert into the REPL library.
OK
When assert.ok(value)
is used, assert will make sure that the value in it is always true.
$ assert.ok(1);
undefined
$ assert.ok(false);
AssertionError [ERR_ASSERTION]: false == true
$ assert.ok();
AssertionError [ERR_ASSERTION]: undefined == true
Equal, Not Equal
Equal
When assert.equal(LHS, RHS)
is used, assert will compare Left Hand Side value to the Right Hand Side value, which is very useful to test for behaviour.
$ assert.equal(7,7);
undefined
$ assert.equal(7,8);
AssertionError [ERR_ASSERTION]: 7 == 8
Not Equal
When assert.notEqual
is used, assert will do exactly the opposite of the Equal
$ assert.notEqual(1,2);
undefined
$ assert.notEqual(1,1);
AssertionError [ERR_ASSERTION]: 1 != 1
ifError
This function is used to throw if there is an error.
function throwError() {
throw('error');
}
assert.ifError(throwError());
Used it in js file
Sample Project 1: Testing Regex
Since REPL mode to try out is done, I would like to share a bit to change my existing testing code from raw node.js to assert library.
The testing code is used to test the REGEX for the project which the source code is on GitHub.
You do not need to really understand the variable name, basically I want to make sure the REGEX does what I expect it to do.
The old code
function regexTest() {
console.log('==========REGEX TEST START==========');
// Image test
let resultFile = imageParser(sourceFile);
console.log('imagePaser test:');
console.log(resultFile === imgFile);
// Link test
console.log('linkPaser test:');
console.log(linkParser(resultFile) === linkFile);
console.log('========== REGEX TEST END ==========');
}
The way I test is using console.log()
in node.js, which is suitable for simple thing as this.
By using assert
library, the source code can be like this.
Using assert library
const assert = require('assert');
function regexTest() {
// Image Test
assert.equal(imageParser(sourceFile), imgFile);
// Link Test
assert.equal(linkParser(resultFile), linkFile)
}
Sample Project 2: Testing with reading a file
In this second sample, we will make use of 'fs' library which is build in with Node.js.
const fs = require("fs");
const assert = require("assert");
function fileExist(cb) {
fs.readFile("test.txt", "utf8", (err, src) => {
if (err) {
return cb(err);
} else {
return cb(null, src);
}
});
}
fileExist((err, src) => {
assert.ifError(err);
console.log(src);
});
What this file do is that it read the file called test.txt
, and if it exist, it will return err
to be null
and src
to be what is in the file. On the other hand, if the file does not exist, it will return err
with the callback.
So, if error exist, we are going to use assert.ifError(err)
to throw out the error if the file does not exist.
Then, create a file called test.txt
to make the test success.
If you not sure wether the code exitted successfully, you can always run
echo $?
to see what exit code is it. In node.js,0
means it exit successfully.
Final thoughts
'assert' library is suitable for small scale testing. It is not suitable for large project because 'assert' library will stop execution when they met an error, and the test also will take longer time if there is many test cases.
Therefore, if sometimes when you need some extra feature like using HTTP method, it would be wise to use other testing framework like Tape, Mocha and Jasmine.
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved because it does not follow the Utopian Rules.
You can contact us on Discord.
[utopian-moderator]
Alright, thanks for your effort moderator.
i am not a coder but it sounds nice ,i have heard of node i will give a try
Its a great initiative from u there 😃
Thanks
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by superoo7 from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.