Discord Bot Tutorial: Making a discord bot (Detailed write up)
This is the detail write up for my previous tutorial posted on DTube.
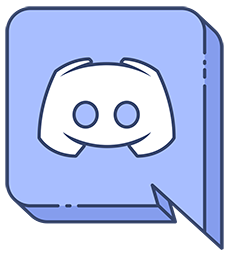
source
What Will I Learn?
- Discord.js API
- Trigger a discord bot
- Simple GET request with Axios
Requirements
- Node.js installed and discord account with developer's mode enabled
- Basic understanding on Node.js , NPM and Yarn
Difficulty
Basic
Description
Setting up Discord Account
Setting up the bot on https://discordapp.com and choose
Developers
> My App
> New App
>
Fill up the details
> Create a bot
>
Copy the Discord Token Generated and save in .env
>
Generate a link to add the bot
Install NPM packages
Run npm init
or yarn init
to initialise the project.
- Run
npm install discord.js dotenv axios --save
oryarn add discord.js dotenv axios
to install require library. - Run
npm install --save-dev nodemon
oryarn add --dev nodemon
to install required development library.
discord.js
- A library to make Discord bot.
dotenv
- to save private data in .env
file
axios
- A HTTP Request library
nodemon
- A library that watch .js
file, when on change it will restart node.
Start the project
In root folder create a file call index.js
.
const Discord = require('discord.js');
const dotenv = require('dotenv');
const axios = require('axios');
dotenv.config();
Import all required dependencied/library
const client = new Discord.Client();
Create a discord client
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
When the bot is connected to Discord shows in the console
client.on('message', msg => {
// MAIN CODE HERE
})
When the bot received a discord message, this client will listen to that event
client.login(process.env.DISCORD_TOKEN);
Login to the server with the token generated previously
In the main code (client.on('message', msg => {})
)
Check wether the message belongs to bot or not.
if (msg.author.id !== 'BOT_ID') {
// INSERT CODE HERE
}
If trigger ($) is called in the channel
let trigger = '$';
let content = msg.content;
let firstLetter = content.substring(0, 1);
if (firstLetter === trigger) {
// INSERT CODE HERE
}
After trigger check the option
let option = content.substring(1).split(' ')[0];
switch (option) {
case 'help':
msg.reply('help');
break;
case 'weather':
msg.reply('good weather');
break;
case 'api':
// DO SOMETHING
break;
default:
msg.reply('error');
}
So when $help
will make bot reply 'help', and $weather
the bot will reply 'good weather'.
In the case 'api':
we are going to call an API using Axios library.
axios
.get('https://reqres.in/api/users?page=2')
.then(data => data.data)
.then(data => {
msg.reply(`\`\`\`${data.total}\`\`\``);
});
break;
Axios will set a get request to https://reqres.in/api/users?page=2
and the data received in total
value will be return. Some advance stuff you can do with API like getting weather data, currency price in CoinMarketCap and more features.
The full source code
const Discord = require('discord.js');
const dotenv = require('dotenv');
const axios = require('axios');
dotenv.config();
const client = new Discord.Client();
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.on('message', msg => {
if (msg.author.id !== '417272012835258388') {
let trigger = '$';
let content = msg.content;
let firstLetter = content.substring(0, 1);
if (firstLetter === trigger) {
let option = content.substring(1).split(' ')[0];
switch (option) {
case 'help':
msg.reply('help');
break;
case 'weather':
msg.reply('good weather');
break;
case 'api':
axios
.get('https://reqres.in/api/users?page=2')
.then(data => data.data)
.then(data => {
msg.reply(`\`\`\`${data.total}\`\`\``);
});
break;
default:
msg.reply('error');
}
}
}
});
client.login(process.env.DISCORD_TOKEN); // Start server
To run this, simply just run node index.js
or nodemon index.js
Video Tutorial
DTube
YouTube
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks moderator, that was quick
Hey @amosbastian, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
You’ve been upvoted by TeamMalaysia community. Do checkout other posts made by other TeamMalaysia authors at http://steemit.com/created/teammalaysia
To support the growth of TeamMalaysia Follow our upvotes by using steemauto.com and follow trail of @myach
Vote TeamMalaysia witness bitrocker2020 using this link vote for witness
Awesome! Finally I got the chance to learn about discord bot. Thanks a lot @superoo7!
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by superoo7 from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
Hey @superoo7 I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
hi,
Nice post. Is there a way to run this in the cloud?
Thanks
I use DIgital Ocean to run my server