Introduction to C programming Language
The C Programming Language
C is a general-purpose programming language, closely associated with the UNIX systems since most of the programs and even the UNIX systems itself are written in C. Interestingly, the language is not tied to any operating system or machine, thus capable of writing compilers and operating systems in many different domains.
C is a relatively “low-level” language. This does not mean that it is simple and stupid; on the contrary it deals with the hard stuff at the level of the computer machine, namely characters, numbers, and addresses (memory addresses).
C operations limitation
C does not provide operations that deal directly with composite objects such as character strings, sets, lists, and array. No operations that can manipulate an entire string or array, although structures may be copied as a unit.
*The language does not define any storage allocations facility other than static definition and the stack discipline provided by the local variables of functions; there is no heap or garbage collection.
C itself provides no input/output facilities; there are no READ or WRITE statements, and no built-in file access methods.
Functions
All of the higher-level operations must be provided by explicitly called functions. Most C implementation has included a reasonably standard collection of such functions. Though the limitation may seem like a grave deficiency, keeping the language down to modest size has real benefits. Due to its small size and can be described in a small space, C can be learned quickly.
ANSI Standard
In 1983, the American National Standards Institute (ANSI) established a comprehensive definition of C. [1] The standard was based on the original reference manual, with relatively little changes. One of the goals of the standard was to make sure that most existing programs would remain valid.
Some of changes:
- Structure assignment and enumerations now officially a part of the language
- Floating point computations may now be done in single precision
- Introduction of a standard library
Getting Started
The best way to learn a language is by writing programs in it. It is a convention that the first program you’ll write is to
This a starting point of our journey, to leap over it we have to create the program text somewhere, compile it successfully, load it, run it, and find out where your output went.
Once this workflow is mastered, everything that follows is relatively easy.
IDE (integrated development environment)
For this tutorial, we will be using Bloodshed Dev-C++ as our IDE for the C programming language. Dev-C++ uses a Mingw port of GCC as its compiler. (if it doesn’t ring a bell, ignore that).
We need to install the IDE first, if you don’t have this software. Now go to: http://www.bloodshed.net/devcpp.html
Go the download section and select the “Dev-C++”.
Our first program
Now that you have successfully installed the Bloodshed Dev-C++, create a new file and type the following text:
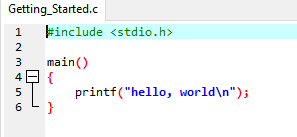
To compile the code, press Ctrl+F9 and to run the code, press Ctrl+F10, or if you want to compile then run the code, press Ctrly+F11
Explanations about the program itself
A C program is consists of functions and variables. A function contains statements that specify the computing operations to be done, and variables store values used during the computation.
In the example, our function was named main. Note, however, that main is special – your program always begins executing at the beginning of the main. Thus, it is required for the program to have a main somewhere. Data between functions can be communicated if one provides a list of values called arguments to the functions it calls. The parentheses after the function name surround the argument list.
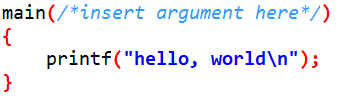
The function main calls other function, some of which you wrote and others from libraries provided, to perform some tasks. In our example, the first line,
commands the compiler to include the library about standard input/output functions.
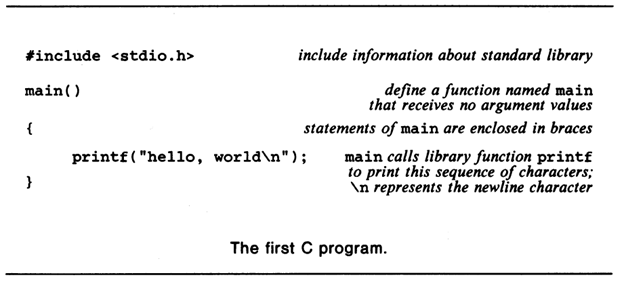
In our example, main is defined to be a function with no arguments, indicated by the empty list ().
The statements of a functions are enclosed in braces {}. In case of our example, the main function contains the statement,
Function calling
A particular function is called by naming it along with its list of required arguments inside the parentheses. In our example, this calls the function printf with the argument “hello, world\n”. printf is the standard function for printing outputs, for our example, the string character between the quotes.

Hello world output after compiling and running.
The \n (slash n) represents only a single character; it is an example of an escape sequence. Among others that are provided in C includes: \t for tab, \b for backspace, \” for the double quote, and \ for the backslash itself.
Variables and Arithmetic Expressions
Our next program uses the formula to print table of temperature and their centigrade or Celsius equivalents:
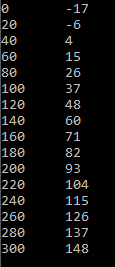
(left: Fahrenheit, right: Celsius)
The program still have the single function named main. And we now have introduced new ideas such as comments, declarations, variables, arithmetic expressions, loops, and formatted output.
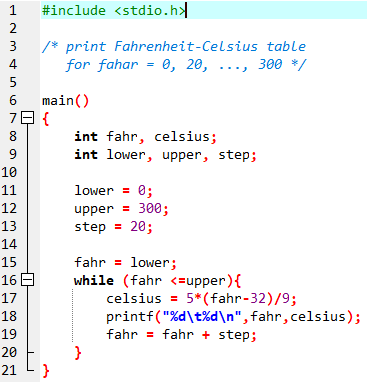
Explanations about the program itself
Comment
The two lines
are a comment commonly used to explain what the program does. A comment is characterized by /* to start and **/ to end it. Characters between / and ***/ are ignored by the compiler.
Variables and Declaration
In C language, all variables must be declared, usually at the beginning of the functions, before they are used. A declaration dictates the property of variables, consisting of a type name and a list of variables such as,
The type in our case are int which means that the variables listed are integers, by contrast with float for floating point (numbers that have a fractional part). The range of both int and float is dependent on your computer you are using; for 16-bit ints it lie between -32768 and +32767, there is also 32-bits ints. The float number, on the other hand, for a 32-bit quantity with ath least six significant digits and magnitude generally between about 10^-38 and 10^+38.
Other basic data types in C include the following:
These types sizes are also machine dependent. Other objects in C are arrays, structures and unions of these basic types, pointers to them, and functions that return them all.
Assignment statements
In our conversion program, computation begins with the assignment statements which set the variables to their initial values. Each statement is terminated by semicolons.
Loops
The conversion program uses the same equation for each line of the table, so we use a loop to calculate the output.
The while loop checks the condition in parentheses (fahr <= upper). If it is true the body of the loop statements is executed. Then, again, the condition is re-tested and if true the body is executed again. When the condition is false the loop ends and the next line that follows the loop will be executed.
The computations that we want were all done in the body of the loop. The Celsius is computed and assigned to a variable celsius by the statement,
Note that we multiply by 5 the difference and then divide by 9 instead of just multiplying by 5/9 as what our equations would say. The reason for this is that in C, integer division truncates; that is, any fractional part is discarded.
Since 5 and 9 are integers, 5/9 would be truncated to zero and so all the temperature would be reported as zero. So for this case:
The result shows that all Celsius temperature:
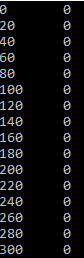
Formatted output
We have shown also how printf works. A printf first argument is a string of characters to be printed, with each % indicating where one of the other arguments is to be substituted, and in what form it is to be printed. Each % construction in the first argument of printf is paired with the corresponding next arguments.
In our case, the %d implies an integer argument. We also added a tab (\t) between the two integers fahr and celsius.
Floating Point Version of Temperature Conversion
The problem of our previous program was that we used integer arithmetic, resulting to Celsius temperatures that are not very accurate. For accurate results, we need to use a floating-point arithmetic instead of integers.
Our new version of the temperature conversion would now look like this:
Our fahr and celsius now are declared to be float, and our formula for the conversion is changed to a more natural way. The truncation problem of (5/9) of our previous version is now solved using decimal point in the constant (5.0/9.0), indicating a floating point.
Our output is formatted in a new way also. The %3.0f says that a floating-point number is to be printed at least 3 characters wide, with no decimal point and no fraction digits. The %6.1f describes that the output be printed at least six characters wide, with 1 digits after the decimal point. Hence our output looks like this:
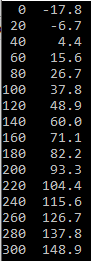
expected output
Width and Precision specification
For floating point output, we can use the following other specifications,
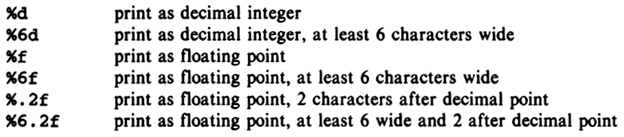
Symbolic Constants
In a conversion program we constants in our variables that are universally used. The values of 300 and 20 in our previous program are best put in a visible manner so that we can change it in a systematic way.
One way to deal with this is to give them meaningful names. C has a #define line that defines a symbolic name or symbolic constant to be a particular string of characters:
Any occurrence of the name will be replace by the value of the replacement text. Our new version will be,
The quantities LOWER, UPPER, and STEP are symbolic constants, not variables, so they do not appear in declarations. Symbolic constants are written in upper case so that they can be easily distinguished from the lower case variable names. Also, remember that there is no semicolon at the end of the symbolic constant declaration.
Posted on Utopian.io - Rewarding Open Source Contributors
You got a 9.78% upvote from @dailyupvotes courtesy of @sinbad989!
Please upvote this comment to support the service.
Your contribution cannot be approved because it does not follow the Utopian Rules.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]