Installing and Getting Started in Fortran using g95 compiler
What Will I Learn?
- You will learn how to install a g95 Fortran compiler
- You will learn how to compile and run Fortran program
- You will learn how the types, variables, constants, and operators of Fortran 95
- You will write a simple procedure in Fortran 95
Requirements
- The g95 compiler
- Sublime Text Editor
Difficulty
- Basic
Tutorial Contents
For this tutorial, we will be exploring the classy, old world of Fortran.
What is Fortran?
Fortran is a general-purpose, imperative programming language that is especially suited for numeric computation and scientific computing. Originally developed by a team at IBM in 1957 for scientific calculations. [1]
How to get started in Fortran?
The first thing we need is to download a compiler. For our case, we will download a g95 compiler. (This compiler transforms computer code written in one programming language (the source language) into another programming language (the target language) [2]. A g95 is a free open source Fortran 95 compiler.
Installation
Click this link here: The Fortran Company. At the end of the article you will find the executable file that we need - the g95 compiler.
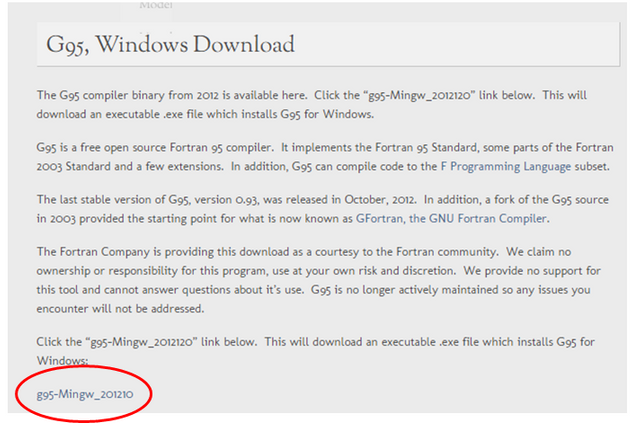
Once the download is completed, you just have to double click the executable file. That's it, you now have a Fortran 95 compiler.
Getting Started
The first program you write in any programming language is printing of the famous "hello world". Type the following in Sublime text editor (you can actually use notepad):
program hello
! this program prints "hello world"
print*, "hello world!"
end program hello
Save this code as "hello.f95". (Note that the file extension is ".f95".) To compile and run this program we need a command line, and type the following (in my I've save the file in D:\ ):
D:>g95 -c hello.f95
Then a new file is generated, the hello.o, which we need to convert to an executable file. This time, type the following:
D:> g95 -o hello hello.o
This command will generate an executable file named hello. To execute this program type,
D:> hello
You've just created your first program in Fortran! Let's explore more.
Types, Variables, Constants, and Operators
Consider the following program for the illustration of the ideas of types, variables, constants, and operators.
program vertical
! A one-dimensional motion under the influence of gravity
! constant/s (acceleration due to gravity)
real, parameter :: g =9.8 ! in m/s
! variables
real :: y ! diplacement
real :: t ! time
real :: v0 ! initial speed (m/s)
! set values of variables
t = 6.0
v0 = 60
! Operators, calculate the displacement
s =v0*t - g*(t**2)/2
! output results
print*, 'Time=',t,' Displacement =',s
end program vertical
- Variables should be declared at the beginning of the program in a type declaration statement (e.g. real::t).
- After declaring the type of the variables, you can then set its value (e.g. t=0)
- There are 5 intrinsic data types in Fortran:
- Integer type: e.g. -3,-2,-1, 0, 1, 2, 3 (integer::total)
- Real type: e.g. 3.14, -50.23 (real::average)
- Complex type: (complex::cx) cx = cmplx(0.5, -3.0) means cx = 0.5 - 3.0, that is, you need the intrinsic function cmplx(a,b)
- Logical type: .true. and .false. (yes you're seeing dots on both sides)
- Character type: this includes the characters and strings (character(len=80)::line !a string consisting of 80 characters)
- For a named constant, e.g. "real, parameter :: g =9.8", the variable must be declared with the "parameter" attribute.
- For the operators, we've used multiplication () and raising powers (*), for other operators refer to the table below.
Procedures
A program in Fortran must contain one main program, which has the familiar form:
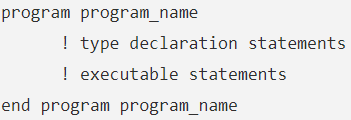
We can add additional program units such as procedures together with our main program.
A procedure is also known as a subprogram. A procedure is a computation that can be called from the program. It interacts with the main program via arguments. Usually, they perform a well-defined task. After performing the task, a procedure returns a single quantity.
Internal Procedures
Internal procedures are contained within a program unit. A main program containing internal procedures has the following form:
The program below demonstrates an internal procedure that calculates the area of the circle.
program area
implicit none
real :: radius, a
radius = 1.4
a = circle_area (radius)
print*, "The area of a circle with radius ", radius, " is" , a
contains
function circle_area (r)
! this function computes the area of a circle with radius r
implicit none
! function result
real :: circle_area
! dummy arguments
real, intent (in) :: r
! local variables
real, parameter :: pi = 3.141592654
circle_area = pi * r**2
end function circle_area
end program area
Similarly, you should save the program with a ".f95" extension (e.g. "circleArea.f95"). And to compile and run the program, type the following in your command line:
D:>g95 -c circleArea.f95
D:>g95 -o area circleArea.o
Explanations
- the function circle_area requires an argument r
- Fortran allows the specification of "intention" with which arguments are used in the procedure. The expression intent(in) means that the argument r only receives value from outside of the procedure.
intent(in) : arguments are only used and not changed
intent(out): arguments are overwritten
intent(inout): arguments are used and overwritten
- the function is called like: a = circle_area(radius)
Summary
We have installed a g95 compiler on our computer and was able to get started in Fortran programming with basic types, operators, variables, and constants. We then proceeded to writing internal procedures in a Fortran program. In addition, we introduce the concept of a compiler and how to compile our Fortran codes. Along the way, we have been introduced to the idea of intention in Fortran, such as intent(IN), intent(OUT), intent(INOUT).
References:
- http://www.chem.ucl.ac.uk/resources/history/people/vanmourik/images/Fortran%2095-manual.pdf
- https://www.mrao.cam.ac.uk/~rachael/compphys/SelfStudyF95.pdf
Posted on Utopian.io - Rewarding Open Source Contributors
You got a 4.00% upvote from @minnowvotes courtesy of @sinbad989!
Wow...
Fortran was my first love, which make me interested on Programming. Nice
Your contribution cannot be reviewed because it does not follow the Utopian Rules, and is considered as plagiarism. Plagiarism is not allowed on Utopian, and posts that engage in plagiarism will be flagged and hidden forever.
http://www.chem.ucl.ac.uk/resources/history/people/vanmourik/images/Fortran%2095-manual.pdf
https://www.mrao.cam.ac.uk/~rachael/compphys/SelfStudyF95.pdf
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]