TUTORIAL || HOW TO MAKE CRUD WITH PHP AND MYSQL USING METHOD DISPLAY FROM BATABASE
What Will I Learn?
- We will learn how to make crud with PHP
- We will learn how to make connect data base with crud, and input data so that can be accessed by crud.
- We will learn to display data from database into crud
- We will learn how to work from crud using PHP MYSQL
Requirements
- Xampp
- Text Editor (Sublime Text)
- Browser Application
Difficulty
- Intermediate
Tutorial Contents
What is CRUD ??
C (Create): which means creating a new data, for example we are registering in a web that is already a Create from CRUD because we create and store registration data to the database.
R (Read): Read or display a data that was located in MySQL database for example, then displayed in WEB using Php
U programming language (Update): well for this one process is editing a data from database which then edited using Php programming language in the form of WEB. Example edit facebook profile.
D (Delete): Surely you know what function is not it? Its function is almost the same as Update but this process is to do the deletion of data in the database through Php language. Examples on a blog sometimes have comments, then we delete the comment, well it includes the delete process in CRUD.
CRUD is often used in data processing applications that mostly use the CRUD function therein. This function is used to add data, delete data, and update data.
STEP MAKE OF CRUD
- Because in the crud-making process we will play with DataBase So the first step of crud creation is to create a database.
- Create a data base with the name "crud".
- Then create table with "user "
- And then create 4 colom in User Table (id, name, address, job)
- And input data like this
- to make it easy you can import the following SQL
"
INSERT INTO `crud`.`user` (`id`, `nama`, `alamat`, `pekerjaan`)
VALUES
('01', 'bahri', 'blangpulo lhokseumawe', 'web programmer'),
('02', 'samsul', 'cunda lhokseumawe', 'web programmer'),
('03', 'dolah', 'lhoksukon aceh utara', 'DBMS'),
('04', 'Dahlan', 'Kuta blang Lhokseumawe', 'pegawai'),
('05', 'Dilan', 'paya bakong aceh utara', 'pegawai');
"
How to display data from MYSQL database
- First we have to make a single look on the web page.
- Now we create a page with the name idex.php
index.php
"
< !DOCTYPE html>
< html>
< head>
< title>How To Make CRUD With PHP And MySQL - Displays data from database< /title>
< link rel="stylesheet" type="text/css" href="style.css">
< /head>
< body>
< div class="judul">
< h1>Make CRUD With PHP And MySQL< /h1>
< h2>Displays data from database< /h2>
< /div>
< br/>
<?php
if(isset($_GET['pesan'])){
$pesan = $_GET['pesan'];
if($pesan == "input"){
echo "Data berhasil di input.";
}else if($pesan == "update"){
echo "Data berhasil di update.";
}else if($pesan == "hapus"){
echo "Data berhasil di hapus.";
}
}
?>
<br/>
<a class="tombol" href="input.php">+ Tambah Data Baru</a>
<h3>Data user</h3>
<table border="1" class="table">
<tr>
<th>No</th>
<th>Nama</th>
<th>Alamat</th>
<th>Pekerjaan</th>
<th>Opsi</th>
</tr>
<?php
include "koneksi.php";
$query_mysql = mysql_query("SELECT * FROM user")or die(mysql_error());
$nomor = 1;
while($data = mysql_fetch_array($query_mysql)){
?>
<tr>
<td><?php echo $nomor++; ?></td>
<td><?php echo $data['nama']; ?></td>
<td><?php echo $data['alamat']; ?></td>
<td><?php echo $data['pekerjaan']; ?></td>
<td>
<a class="edit" href="edit.php?id=<?php echo $data['id']; ?>">Edit</a> |
<a class="hapus" href="hapus.php?id=<?php echo $data['id']; ?>">Hapus</a>
</td>
</tr>
<?php } ?>
</table>
"
- Then we will make a connection between Index.php with Crud Yang database that we created earlier
koneksi.php
"
<?php$host = mysql_connect("localhost","root","");
$db = mysql_select_db("crud");
"
SYNTAX PROGRAM Explanation
in the index.php file in the following syntax section
'
< ?php
if (isset($_GET['pesan'])){
$ pesan = $ _GET['pesan'];
if($pesan == "input"){
echo "Data berhasil di input.";
}else if($pesan == "update"){
echo "Data berhasil di update.";
}else if($pesan == "hapus"){
echo "Data berhasil di hapus.";
}
}
? >
'
- In this syntax serves to retrieve data memalalui URL to create a message notification data, for example the data has been successfully in the input, delete or in the update.
'
< table border="1" class="table">
< tr>
< th>No< /th>
< th>Nama< /th>
< th>Alamat< /th>
< th>Pekerjaan< /th>
< th>Opsi< /th>
< /tr>
< ?php
include "koneksi.php";
$query_mysql = mysql_query("SELECT * FROM user")or die(mysql_error());
$nomor = 1;
while($data = mysql_fetch_array($query_mysql)){
? >
< tr>
< td>< ?php echo $nomor++; ? >< /td>
< td>< ?php echo $data['nama']; ? >< /td>
< td>< ?php echo $data['alamat']; ? >< /td>
< td>< ?php echo $data['pekerjaan']; ? >< /td>
< td>
< a class="edit" href="edit.php?id=<?php echo $data['id']; ?>">Edit< /a> |
< a class="hapus" href="hapus.php?id=<?php echo $data['id']; ?>">Hapus< /a>
< /td>
< /tr>
< ?php } ?>
< /table>
'
- sintax above serves to Display in the table.
'
< ?php
include "koneksi.php";
$ query_mysql = mysql_query("SELECT * FROM user")or die(mysql_error());
$ nomor = 1;
while($data = mysql_fetch_array($query_mysql)){
? >
< tr>
< td>< ?php echo $nomor++; ?>< /td>
< td>< ?php echo $data['nama']; ?>< /td>
< td>< ?php echo $data['alamat']; ?>< /td>
< td>< ?php echo $data['pekerjaan']; ?>< /td>
< td>
< a class="edit" href="edit.php?id=<?php echo $data['id']; ?>">Edit</a> |
< a class="hapus" href="hapus.php?id=<?php echo $data['id']; ?>">Hapus</a>
< /td>
< /tr>
< ?php } ? >
'
- Notice the syntax above first we haverus connect the Index.ph file with koneksi.php because we need konesi into the index.php file to the database to retrieve data from the database and then in show in the table with us paste the file koneksi.php with the function include.
'
include "koneksi.php";
'
if everything is understood and finished next we will beautify the look of index.php in the following way.
style.css
"'
body{
font-family: 'roboto';
color: #000;
}
.judul{
background: #87D1D8;
padding: 10px;
text-align: center;
}
.judul h1,h2,h3{
height: 15px;
}
a{
/*color: #fff;*/
padding: 5px;
text-decoration: none;
}
.table{
border-collapse: collapse;
}
table.table th th , table.table tr td{
padding: 10px 20px ;
}
"
- or like in
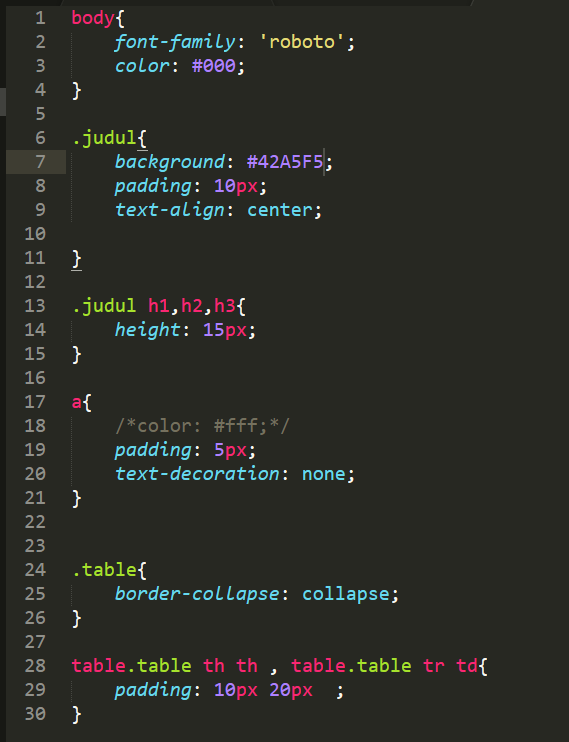
- if all the stages are done then the crud display on index.php will be like this
and finish
Curriculum
- Tutorial || How To Create UPLOAD MULTIPLE FILE WITH PHP MAKING DROPZONEJS MODE
- TUTORIAL || How To MAKE GRAPHS WITH PHP MYSQL AND WITH CHARTJS METHOD
- TUTORIAL || Cara Membuat Form Pencarian Dengan PHP Dan MYSQL [ How To Create Search Form With PHP And MYSQL]
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @rajamalikulfajar I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Congratulations @rajamalikulfajar! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP