Instantaneous Steemit Account Creation Script
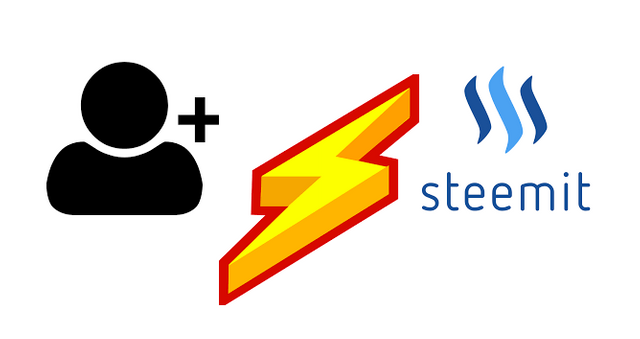
What Will I Learn?
Creating an account with Steemit and waiting for its approval can prove too time consuming. At times, approvals can extend up to 7 days, which could definitely cause a loss of interest for the person attempting to join in.
Yet, we can code our way out of it and make the process instantaneous as long as we have another existing steemit account to use for the creation.
In fact, steemjs the famous steemit API provides just what is needed to allow instantaneous creation of steemit accounts, yet the lack of documentation to the parameters and the process makes the task a bit difficult to accomplish without proper research and experimentation.
Let's document and go through the process to create a script that allows us to just do that!
Requirements
To be able to follow this tutorial, you would need some basic coding knowledge, particularly in javascript.
Difficulty
The tutorial is essentially an intermediate level for anyone who wants to learn the intricacies of the process, but also a complete version of the code is provided for you to use.
The Process
Loading steemjs
As highlighted above, steemjs library allows us to overcome the limitations of waiting for account creation/approval on steemit via using some built-in API calls.
To include steemjs, it only requires a single line of code into your HTML document, as follows:
<script src="https://cdn.steemjs.com/lib/latest/steem.min.js"></script>
Account Creation Function(s)
Now the key function to create accounts provided via the steemjs library comes under two shapes:
steem.broadcast.accountCreate
and
steem.broadcast.accountCreateWithDelegation
The first allows direct account creation, while the second clearly adds the option to create the account while delegating vests (Steem Power) onto it. An account does need a minimum amount of SP to be able to properly function, this being at the moment and AFAIK in the amount of 15 SP.
We will use the second method then to perform this process, which has the full signature as per below:
steem.broadcast.accountCreateWithDelegation(wif, fee, delegation, creator, newAccountName, owner, active, posting, memoKey, jsonMetadata, extensions, function(err, result) { console.log(err, result); });
Parameters Explanation
So there is a multitude of parameters (values ) that need to be sent to the function for it to properly work. Some are straightforward, while others might seem so, yet aren't and could cause errors in the process, and the explanation is below:
- wif: the new account's wif/password/private key used to access it. The longer the password, the better (I for one would recommend at least 50 character passwords, and preferably generated via http://passwordsgenerator.net/ - Just make sure you DO NOT INCLUDE symbols as those are invalid for steemit passwords and would yield an error.
- fee: the amount in STEEM which will be sent from the creator account to the new account. This comes in the format of XXX.XXX STEEM.
- delegation: the amount of delegated vests/steem power in VESTS units that will be delegated from the creator account to the new account. This comes in the format XXXXX.XXXXX VESTS. A standard amount would be "30663.815330 VESTS" equating to 15 SP.
- creator: the account creator name
- newAccountName: the new account name
- owner, active, posting, and memoKey: the relevant keys for the new account
- jsonMetadata and extensions: any additional meta data and/or extensions that need to be passed over to the new account
Testing for proper account name format
Before proceeding with calling out the account creation function, it is advisable to test out whether the account name follows the standards required by steem. To do so, we will perform a call to another function
steem.utils.validateAccountName(new_account)
The function will check if the format is valid, and if so, will return null, otherwise it will return the relevant error message. So comparing the return value of the function against null would allow us to make sure the account is actually valid and to move on.
console log below

Checking if account already exists
Another step before the actual account creation is ensuring the account is available. This can also be performed via calling the following function
steem.api.getAccounts([new_account], function(err, result) {
which basically would return the account if it exists. So we are actually also looking for an empty result set to make sure it doesn't!
Hence, the proper test for moving forward would be
if (result.length==0){
console log below

Key generation
While we are able to create our own password for the account, yet keys cannot and need to be properly generated and passed along in a format understandable to the function and steem.
Here again, we can rely on the generateKeys function:
steem.auth.generateKeys(new_account, wif, ['owner', 'active', 'posting', 'memo'])
To which we pass the new account name, wif/password, and require for it to generate all 4 keys components which will be utilized in the account creation ('owner', 'active', 'posting', 'memo')
The function would return an object with those 4 variations of keys, and can then be used as params for the creation function
var publicKeys = steem.auth.generateKeys(new_account, wif, ['owner', 'active', 'posting', 'memo']);
var owner = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.owner, 1]] };
var active = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.active, 1]] };
var posting = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.posting, 1]] };
And then eventually, after all this preparation, we are now ready to call our core function as follows
steem.broadcast.accountCreateWithDelegation(owner_wif, fee, delegation, owner_account, new_account, owner, active, posting, publicKeys.memo, jsonMetadata, extensions, function(err, result) { console.log(err, result); });
Full Code
Wrapping the whole code together, we will end up with the below code. For ease of access and usability, I have created a gist with the full code which can be found here
<html>
<head>
(html comment removed: including steemjs library for performing calls )
<script src="https://cdn.steemjs.com/lib/latest/steem.min.js"></script>
<script>
create_steemit_user();
function create_steemit_user(){
//set the proper steemit node to be used, otherwise default steem-js node will fail
steem.api.setOptions({ url: 'https://api.steemit.com' });
//variable containing the new password . Suggest to use http://passwordsgenerator.net/ and set a min size of 50 chars, and DO NOT INCLUDE symbols as those are invalid
var wif = "";
//WIF of the user creating the account
var owner_wif = "";
//name of user creating the account
var owner_account = "";
//new user account name
var new_account = "";
//the fee to be used when creating the account. Make sure the value is set according to this template. I haven't used a lower value and eventually the amount will be sent over to the receiving new account
var fee = "0.200 STEEM";
//set the amount of SP to delegate to this account. This is the min value being 15 STEEM for an account to be properly functional
var delegation = "30663.815330 VESTS";
//meta data and extensions can be left blank for now
var jsonMetadata = "";
var extensions = "";
/********************** process ****************************/
console.log('attempting creation of account:'+new_account);
//make sure account name is valid
var account_invalid = steem.utils.validateAccountName(new_account);
if (account_invalid == null){
//make sure account does not already exist
steem.api.getAccounts([new_account], function(err, result) {
console.log(err, result);
//no matches found
if (result.length==0){
/* if the code doesn't work, you might need to uncomment this, and change the wif at the top to password */
//var wif = steem.auth.toWif(new_account, pass, 'owner');
//generate the keys based on the account name and password
var publicKeys = steem.auth.generateKeys(new_account, wif, ['owner', 'active', 'posting', 'memo']);
var owner = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.owner, 1]] };
var active = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.active, 1]] };
var posting = { weight_threshold: 1, account_auths: [], key_auths: [[publicKeys.posting, 1]] };
//console.log(posting);
steem.broadcast.accountCreateWithDelegation(owner_wif, fee, delegation, owner_account, new_account, owner, active, posting, publicKeys.memo, jsonMetadata, extensions, function(err, result) {
console.log(err, result);
});
/*steem.broadcast.accountCreate(owner_wif, fee, owner_account, new_account, owner, active, posting, publicKeys.memo, jsonMetadata, function(err, result) {
console.log(err, result);
});*/
}else{
console.log('account already exists');
}
});
}else{
console.log(account_invalid);
}
}
</script>
</head>
</html>
Upon success of the account creation, you will receive in the console a confirmation of the transaction, as follows:

New account created
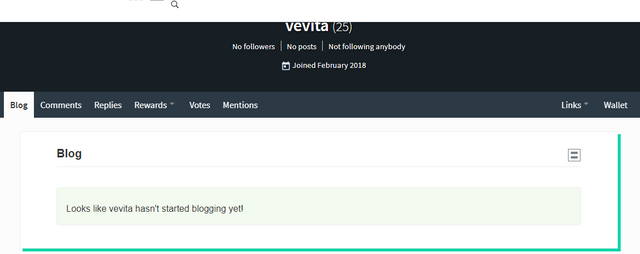
There you go, we successfully and instantaneously created the new account.
Hoping this comes in handy to you guys, and feel free to ask any questions or provide suggestions in the comments below!
Posted on Utopian.io - Rewarding Open Source Contributors
Great tutorial! There are a lot of new users waiting to get their email. It took me 7 days to get mine. Now I enough SP that I could use this and sign up all my friends..... Except it is like pulling teeth to get friends to sign up. HA!
Yes i hear you. You can still do that as a favor to friends, and then once they gather their own 15 SP, you can undelegate your SP and get it back a week later
Génial @mcfarhat,
Vraiment top !
A bientôt.
Christel
Thank you Christel ! :)
Wow. I just started reading. But is mind blowing...
thank you man. I think steemit should deal with this delay. because I really invite so many people and got bored from waiting till they forget about steemit lol. well done man. we are proud to have someone like you on steemit.
Thank you Khaled for the nice words ! :)
Awesome stuff @mcfarhat I will use this !!!
Thank you, glad to hear ! :)
This could be a great tool to build a frontend for. great work. maybe I can help build it as a standalone tool.
One question, do I need to delegate SP to get the account created?
Thank you.
Yes that is unfortunately correct. For an account to function, it needs at least 15 SP :)
I had made this available yesterday via a backend interface for wordpress plugin, if you're into wordpress, you can check my most recent post about this.
Looks good! WI give it a shot. Still however don't have enough steem to delegate.
Beautiful job @mcfarhat on all your posts, thanks so much for all the nice work👍🏻
You got a 100.00% upvote from @arabpromo courtesy of @mcfarhat!
Want to bookmark this so I can try it out later. Thank you for sharing.
You're welcome
@mcfarhat, I always try to support who contribute to open source project, upvote you.