HF20 Tutorial: Claiming & Creating Discounted Accounts
Repository
https://github.com/steemit/steem
Intro
Following HF20 that took place few days back, and as we are starting to operate normally again on the Steem blockchain, I had tested out the long awaited functionality of discounted accounts claim and creation, as we believe it has the potential to open the doors for the masses of Steem adoption.
Basically this functionality allows claiming spots of "discounted accounts creation" from a pool of spots, whereby you would consume "a large amount" of Resource Credits/RCs (Last time I checked those consumed anywhere between 12-22 MM RCs - but the numbers are still adjusting and subject to availability and different factors ) compared to prior approaches which required either paying for account creation, or delegating your SP to create the account. Once claimed, you could also create an account without any additional transaction cost.
Number of claims done shows on your account on steemd.com. The number reflects how many accounts you can create now
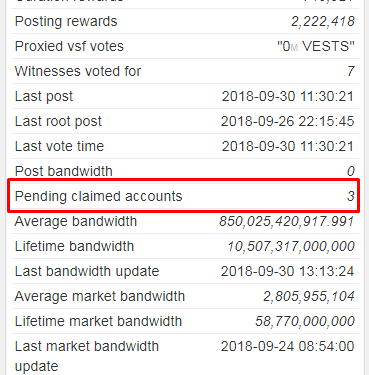
So few days back, I was able to claim a spot, and create an account successfully. Back then, the feature still had a bug, whereby created account has so little RCs (only 3,000 of them), which basically lead the account without any capacity to perform any activity on the BC.
Gladly this issue has now been fixed. Old affected accounts now have the minimum RCs, but also newly created accounts (we just tested this out today with another account) do have the proper minimum as well.
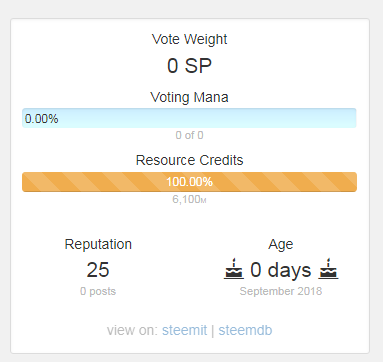
According to steemd.com, newly created accounts now have a full amount of 6,060,649,946 RCs, and are able to at create 3 comments. As they have 0 SP and 0 VM (Voting Mana), I do not believe they can cast any upvote. We haven't tested this out though, but it only makes sense.
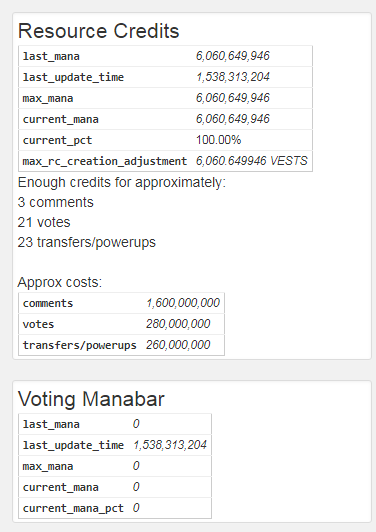
So to cut the story short and move to the tutorial, my good friend and Actifit ambassador @taskmaster4450 asked me to share the script that I created few days ago, so as to provide an easy way to anyone to be able to claim discounted accounts, and create them.
Requirements
To follow the tutorial, you would need some basic HTML and Javascript knowledge, preferably some interaction with dsteem library as we utilize it to perform the actual transactions and operations with the Steem blockchain.
Although and even if you do not have any such knoweldge, you could simply open a notepad, follow the tutorial, write the text, save as HTML file, and you're good!
You will also be able to download a copy of the full code, via accessing the gist available at the end of the tutorial.
Difficulty
The tutorial should not be too difficult to pull off as explained above, and could be rated anywhere between basic and intermediate.
Tutorial Contents
So let's get started with the tutorial. First off, let's setup a small interface to allow us to accomplish what is needed within a browser. We will allow 3 options for the activity:
- Only claiming a spot for discounted account creation, as you can always claim a spot, and keep it for later account creation.
- Only creating a discounted account, as you could rely on previously claimed spots to create the account
- Do both at the same time, claiming a spot and creating the account
HTML Interface
We will just create a very minimalistic interface, that allows the entry of 4-6 types of information, depending on what we want to exactly accomplish at this instance. So we will need to capture the following using basic :
- Main Account: The account to be used to claim or create the account (input text box).
- Private Active Key: Both operations require using your private active key (input text box).
- New Account Name: In case you are aiming to create an account at the moment (input text box).
- New Account Pass: The password for the new account. We recommend making the password as complex as possible. You could easily use http://passwordsgenerator.net/ for creating such complex password (input text box).
- Operation Checkboxes: Checkboxes for the operation(s) that will be carried out now, whether a Claim or a Create. (input checkbox).
- Proceed button: The button to kick start the activity. (input checkbox).
- Notification: A small div area to display error and success messages. (input button).
The below simply code accomplishes this
Main Account:<input type="text" id="main_account"><br/>
Private Active Key:<input type="text" id="private_key"><br/>
New Account Name:<input type="text" id="new_account"><br/>
New Account Pass:<input type="text" id="new_account_pass"><br/>
Claim <input type="checkbox" id="claim_op" name="claim_op">
Create <input type="checkbox" id="create_op" name="create_op"><br/>
<div id="error_section"></div>
<input type="button" value="proceed" id="create_claim">
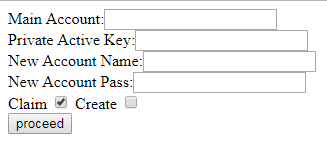
Import jQuery and dSteem libraries
I personally love working with jQuery, although we could make use without it, but we will utilize it for smooth usage and access to fields and function calls.
I recently started using dSteem since HF20 as SteemJS had some troubles with returning data during HF20, and I had to switch to it to get some of my scripts running. Although SteemJS is now fully functional, dSteem is better supported by Steemit, and also is reportedly faster.
Let us include both scripts into our code head section
<script src="https://unpkg.com/dsteem@latest/dist/dsteem.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
EDIT: As of recent changes to dsteem with the release of a newer version, the latest script no longer functions, the workaround/fix for this is instead of using the latest dsteem library, you need to use version 0.10.0 instead, so your code becomes
<script src="https://unpkg.com/[email protected]/dist/dsteem.js">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
Let's Perform Action!
So now that we setup the interface and pulled the libraries, we need to create our actual function that handles the claim and/or create, and finally connect it to our button click function.
So first, as some function calls to dSteem will be asynchronous, we will setup the function as async
async function claimCreate()
Following, we will clear all text messages at the start of the function, and then start pulling the data as filled in the interface we created above
//clear messages text
$('#error_section').text('');
let creator = $('#main_account').val();
//active key is required
let creatorKey = $('#private_key').val();
if (creator == '' || creatorKey == ''){
$('#error_section').text('Please enter your account name and private active key to proceed.');
return;
}
Once we grabbed the essential info, we will need to connect to a Steem node, and a typical node would be https://api.steemit.com .
//connect to node
var client = new dsteem.Client('https://api.steemit.com');
We will also need to properly format the Active key we pulled from the user to confirm to dSteem requirements, as follows:
//dsteem requires this to properly format keys
let privateKey = dsteem.PrivateKey.fromString(
creatorKey
);
Having done the above, we are now ready to check which operation has been selected, and to proceed accordingly. We do this by testing each checkbox and seeing if it is in checked state, if not we will skip this operation. If no operation is selected, we will not perform any action on the Steem BC.
Now if the claim operation is selected, we will need to create the relevant operation. The official statement by Steemit was that the "fee" parameter was optional, yet after a lot of testing, it turns out it needs to be passed, with the value of '0.000 STEEM'. So does the extensions param, although it is not used as the moment, making the code as follows
if ($('#claim_op').is(':checked')){
//the claim discounted account operation
const claim_op = [
'claim_account',
{
creator: creator,
fee: '0.000 STEEM',
extensions: [],
}
];
ops.push(claim_op);
proceed = true;
}
In case the create operation is selected, we will need to grab the user and pass fields, and then ensure the account does not already exist on the Steem BC, create the keys based on the provided password, and then prepare the relevant operation, as follows:
if ($('#create_op').is(':checked')){
let username = $('#new_account').val();
let password = $('#new_account_pass').val();
//check if account exists
const _account = await client.database.call('get_accounts', [[username]]);
//account not available to register
if (_account.length>0) {
console.log('account already exists');
console.log(_account);
$('#error_section').text('Account name already exists. Please choose a different one');
return;
}
console.log('account available');
//create keys for new account
const ownerKey = dsteem.PrivateKey.fromLogin(username, password, 'owner');
const activeKey = dsteem.PrivateKey.fromLogin(username, password, 'active');
const postingKey = dsteem.PrivateKey.fromLogin(username, password, 'posting');
let memoKey = dsteem.PrivateKey.fromLogin(username, password, 'memo').createPublic();
//create auth values for passing to account creation
const ownerAuth = {
weight_threshold: 1,
account_auths: [],
key_auths: [[ownerKey.createPublic(), 1]],
};
const activeAuth = {
weight_threshold: 1,
account_auths: [],
key_auths: [[activeKey.createPublic(), 1]],
};
const postingAuth = {
weight_threshold: 1,
account_auths: [],
key_auths: [[postingKey.createPublic(), 1]],
};
//the create discounted account operation
const create_op = [
'create_claimed_account',
{
creator: creator,
new_account_name: username,
owner: ownerAuth,
active: activeAuth,
posting: postingAuth,
memo_key: memoKey,
json_metadata: '',
extensions: [],
}
];
ops.push(create_op);
proceed = true;
}
Now that we have prepared the relevant operation(s), we are ready to execute them on Steem using the client.broadcast.sendOperations
dSteem function, and hence the remainder of the code would be as such
if (proceed){
//proceed executing the selected operation(s)
client.broadcast.sendOperations(ops, privateKey).then(
function(result) {
console.log(result);
$('#error_section').text('success');
},
function(error){
$('#error_section').text(error);
}
);
}else{
console.log('no operation selected');
$('#error_section').text('Please select either Claim, Create, or both');
}
So there you go! This completes the tutorial of utilizing this new amazing functionality.
Working Code
You can download a fully functional copy of this work via visiting this gist that I created, download it as an HTML file, and open it in your browser, and you're set to claim and/or create your first discounted account on the Steem Blockchain.
Thank you for your contribution @mcfarhat.
Your tutorial is very well explained and structured. This topic is very interesting and will help many users create discount accounts and with enough RCs.
Thank you very much for your tutorial and for your work on developing this tutorial.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 5 contributions. Keep up the good work!
We mice appreciate this tutorial very much mcfarhat.
Out of interest - were us simple mice to use your downloaded HTML copy in our browser, can we be assured that our active key will remain secure?
It never occurred to me that mice will be interested to use my script hehe
yea the code is written so that the key only gets submitted to Steem node via an https (s for secure) steem function call, it's like you putting your active key when logging into Steemit.com, so yea the code poses no risk :)
Thank you for the reassurance Mr McFarhat. We mice think you are wonderful.
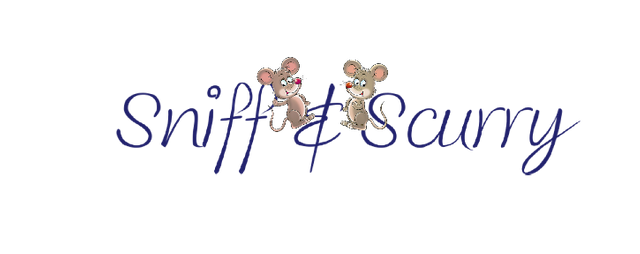
Pleasure :) !
Btw if you mice run (and I know mice run a lot lol), you might be interested in checking @actifit, my SMT project that incentivises people to be active and healthy.
Hey, you know what?
It WORKS !!!
I am a new born plankton, born less than ONE HOUR ago.
Been done exactly as per your tutorials, step-by-step.
O have 68 possible comments/posts in my pocket.
I don't even know what to do with them :)
If you don't believe me , check out yourself:
https://steemd.com/@kmr515
welcome to Steem then ! :)
You powering down mate?
Posted using Partiko Android
I just tested the gist and it indeed worked! I was able to get my girl her account after she's been waiting for the regular account creation to complete for over three weeks.
Thank you so much!
Proof:
Claim | Creation
Sir new acount now.we can create???
How can we check how much RC is needed to claim account
The exact amount varies. You can always run the script, if it fails you will receive a notification of the min amount required.
Trying it just right now required "7,052,965,245,857" RC which is much lower than the numbers I got yesterday.
Thanks for the info. Appreciate it
Thanks for this tutorial on creating accounts. I plan to try it out this week. I'll let you know how it worked out for me!
Very well explained, and easy to work with; claiming is a bit pricey though.
RPCError: Account: dr-frankenstein has 175149836574 RC, needs 9576881380344 RC. Please wait to transact, or power up STEEM.
Thanks bro ! yes it is :)
Problem, and Solution!
I've used this code several times, but when I tried today, it failed.
Using the Developers Console, I see an error in dsteem.js having to do with a promise.
This error happens in version 0.11 of dsteem.js but there is no error in the previous version of 0.10 so use that version. I did, and it then worked fine.
So change
<script src="https://unpkg.com/dsteem@latest/dist/dsteem.js">
to
<script src="https://unpkg.com/[email protected]/dist/dsteem.js">
and see it that works for you, as it worked for me.
Good luck!
Hey @kenny-crane,
Yes what you did is correct, several people had reached out to me last few days, and i pointed them to do the same change, as the new dsteem version appears to be failing for the prior function calls.
Omg all those codes. 😅 I'm still not sure I understand. Claiming accounts mean creating a new account and not owning unused accounts by someone else right?
Posted using Partiko Android
it means if you have enough SP (anywhere above 5-6K right now I believe), you can create free accounts on the Steem blockchain without anyone's assistance. So you claim a "free" spot, and create an account.
The script can be downloaded as an HTML file and run it in your browser, just few fields to fill and you're ready :)
Oh I see. 😅 I've a long way to go then. Thanks!
Posted using Partiko Android