Do All Numbers Converge? The Collatz Conjecture And A Wordpress Plugin Simulator
Hey guys,
If you've been following the recent mathematical paradox posts I had shared, today we got a similar yet a bit different concept. I will shed light on what is called The Collatz Conjecture - more on that in a second, but also share with you some coding work that i've developed as a simulator for the Collatz conjecture as a wordpress plugin in the second portion below, that you can get to play around with.
If you wish to skip to the code part (which I don't recommend till you get a glimpse of the cool stuff we got here already :) ), yet you can still click here to get to coding part
What is the Collatz conjecture
Okay, so the name itself sounds a bit bizarre. It even has few other cooler names: The Ulam conjecture, Kakutani's problem, Hasse's algorithm,... or probably the easiest, 3n+1 conjecture.
And as per any other mathematical theories, paradoxes, conjectures,... it was named after its initial promoter, German mathematician Lothar Collatz, who introduced the idea back in 1937.
The Collatz conjecture basically tries to tackle the following question: following specific behavior for calculations over numbers, would they converge?
And that behavior is specifically as follows:
Take a positive natural number (positive integer), if the number if even, divide it by 2, if the number is odd, multiply by 3 and add 1.
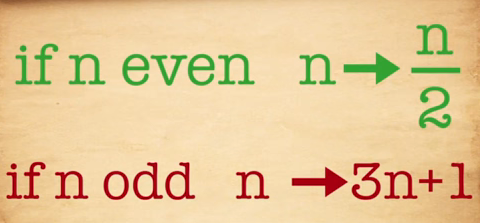
This needs to be performed repeatedly, taking as a starting point the last result of the prior operation, and then doing the process all over again.
So what happens in this case?
Example 1:
So let us try to answer that by taking a simple example.
let's take 5 as our starting point:
- 5 is an odd number, to proceed, we need to multiply by 3, and add 1, which yields 16
- 16, the result from prior round, is even, we need to divide it by 2, which yields 8
- 8 is also even, divide by 2, we get 4
- 4 is also even, divide by 2, we get 2
- 2 is also even, divide by 2, we get 1
From now on, we will notice a pattern though, if we attempt to continue the work with 1, we will fall into the same pattern all over again: - Take the last 1, since it's odd, we multiply by 3, and add 1, we got back to 4!
- And then 4 would lead to 2, and 2 to 1.
Example 2:
Let's take another example, this time starting with an even number, say 12
- 12 is even, we divide by 2, we get 6
- 6 is also even, we divide by 2, we get 3
- 3 is odd, multiply by 3 and add 1, we get 10
- 10 is even, divide by 2, we get 5
And since we got 5, we are back to example 1 above, which would converge back eventually to 4, 2, and 1.
And this is where the Collatz convergence stands, taking any positive integer, and performing those operations consecutively, we will eventually reach 1. No matter which number you start with, the outcome would apparently converge back to 1.
Here are few other examples to show different numbers and how they converge
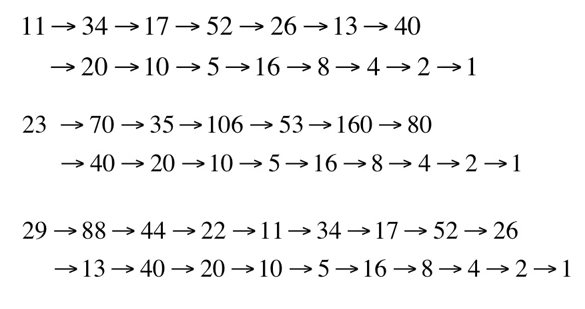
How long does it take to converge?
So the above few examples showed that each took different number of rounds to reach to 1. In fact, it has nothing to do with how large the number is. Take for example the following numbers 8192 and 27.
8192 converges in 13 steps, while 27 converges in 111 steps!
Cool fact:8,192 is actually a multiplier of 1,024, numbers usually used to covert GB, MB, KB,...into bits, so 8GB is actually 8,192 Gbits, which is why it acts as a special number in the conversion
In fact, looking at the below chart, you can view the differences in convergence of numbers for up to 10,000 as a starting number.
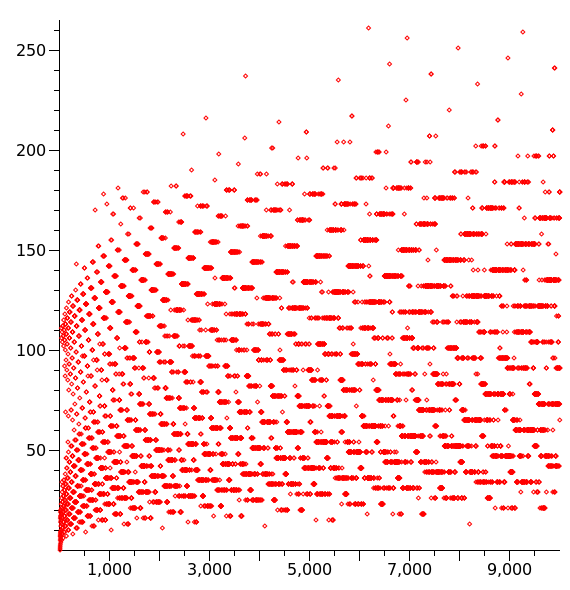
Why is it a conjecture then?
Well, simply because it has not been proven yet, nor can it be disproved. Yet experimental evidence point that the conjecture is true, as of yet.
In fact, Hungarian Mathematician, Paul Erdős, is quoted to have said:
Mathematics may not be ready for such problems
And that he also offered a monetary reward for anyone solving it.
Similarly, mathematician Jeffrey Lagarias said in 2010:
This is an extraordinarily difficult problem, completely out of reach of present day mathematics
In terms of experiments, and knowing that our above examples are probably not enough to confirm :), yet there has been tons of experiments with the Collatz conjecture, and up to this year, all numbers up to 87×260 have been tested, and shown to converge back to 1, similarly to our examples above, yet unfortunately this is no proof as large numbers at one point in time could "somehow" lead to different results. What could happen then? the number could approach approach a cycle different than the 4, 2, 1, or alternatively move towards infinitely larger numbers.
Experiment yourself!
So, just before we go to the technical details, here is a link of my code that I placed for you online to try and experiment with the conjecture ! http://www.greateck.com/the-collatz-conjecture-simulator/
In fact, several of the screenshots above where generated using this tool :)
Coding - The Technical Part
So I wanted to create a wordpress plugin that allows me, or anyone, to experiment with the Collatz conjecture, and be even able to host that on their wordpress installation.
The concept is rather simple, we need to, create a simple interface, that allows data entry of the number, validate it's a proper integer, perform all the calculations, loop through all the results, and render them on screen.
In order to do that, we simply can utilize HTML + JS/JQuery, along with the standard PHP code to encapsulate this into a reusable wordpress plugin, as well as make it into a shortcode that can be plugged into any page on wordpress.
The data entry and display code section can be scripted as follows, allowing the input, the button, the validation section, as well as the display area:
<body>
<label for="starting_number">Starting Number</label><input type="text" id="starting_number">
<span><i>Positive Integer (whole number) Only</i></span>
<br/><br/><input type="button" value="Let's Go!" id="calculate_btn">
<div id="error_notice">Number has to be a positive Integer - Please adjust</div>
<br/><div id="number_progress"></div>
</body>
And then the actual computations can be performed by capturing the click event of the button, grabbing the value, and performing the proper loop along with the two rules of the conjecture, if even divide by 2, if odd multiply by 3 and add 1.
jQuery(document).ready(function($){
//hooking to the click event to initiate the simulation
$('#calculate_btn').click(function(){
//grab the value of the input
var starting_number = $('#starting_number').val();
//assume it's not a number first
if ($.isNumeric(starting_number)){
if (Math.floor(starting_number) == starting_number){
$('#error_notice').hide();
perform_collatz(starting_number);
return;
}
}
$('#error_notice').show();
});
/* function to handle performing calculations based on collatz approach */
function perform_collatz(value){
//add the number to the start of the display
$('#number_progress').empty().text('Starting Number:'+value);
//loop until we get to 1
while(value>1){
if (value%2==0){
//if value is even, divide by 2
value /= 2;
// alert(value);
$('#number_progress').append('<br/>↓'+value);
}else{
//if value is odd, multiply by 3 and add 1
value = value * 3 + 1;
$('#number_progress').append('<br/>↑'+value);
}
}
}
});
To make this a reusable shortcode, pluggable into any page, you just need to utilize the command below, while passing the function that generates all the plugin's functionality
add_shortcode('collatz_simulator', 'display_collatz_simulator' );
You can find the full code by referring to my Git Hub Project here
References:
Photo Credits:
Hope you found this educational and entertaining as expected :)
Posted on Utopian.io - Rewarding Open Source Contributors
well explained @mcfarhat. i am not a mathematician or coder and therefore, my comment speaks volumes about your good work!
Thank you, seriously glad to hear :)
I am wondering how to code it very efficiently. Probably using caching within the mathematica framework may be the most efficient :p
i haven't used mathematica in over 15 years ! but yea i'm sure it would give great results too, at least better than simply using PHP/JS.
Am using it every day of my life. It is a so wonderful tool. A pity it is not free software :/
Well, today I don't feel so stupid very well done, love maths and still nothing better with the coding.
haha thank you, glad to hear you enjoyed it !
loved it I taught maths for many years before becoming headmaster and loved every minute.
Really an excellent post thank you for sharing this was most facinating.
Thank you bigbear, glad to hear ! :)
Thank you @mcfarahat , i don't know you can do that !
pleasure my friend ! :)
Thank you for the contribution. It has been approved.
[utopian-moderator]
thank you !
nice
good
Thank you Hassan
you welcome
Hey @mcfarhat I am @utopian-io. I have just super-voted you at 18% Power!
Achievements
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by mcfarhat from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews/crimsonclad, and netuoso. The goal is to help Steemit grow by supporting Minnows and creating a social network. Please find us in the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.