How to create a steem-python docker coaintainer app from scratch #2 Application
What Will I Learn?
You will learn how to:
- build your own apps with Docker Containers and steem-python.
- how to build disposable Docker Containers.
- how to run docker Docker Containers.
Requirements
- Linux, Windows 10 Professional or Enterprise 64-bit or Mac OS Yosemite 10.10.3
- Docker Community Edition 18.03.0-ce (older Versions and the enterprise edition should also work fine.)
- Standard texteditor
- Terminal
- Basic knowledge in handling the terminal. Change directory etc.
- Basic knowledge in Python
Difficulty
Intermediate
Tutorial Contents
Topics
- Python example program
- Write the Dockerfile for the application
- Build the application image
- Run the application
The tutorial is structured in a way that we divide the applications into three layers. The base, dependencies and application layer.
This tutorial focuses exclusively on the application layer.
Python example program
The following script displays some simple information about users.
It should serve as an example to explain disposable containers.
#!/usr/bin/env python3
from sys import argv
from steem.account import Account
def get_acc_info(username):
"""returns a dictionary with the following information:
name,
sp = Steem Power,
rep = reputation,
steem = STEEM in total,
sbd = SBD in total
"""
acc = Account(username)
return {
'name': acc.name,
'sp': acc.sp,
'rep': acc.rep,
'steem': acc.balances['total']['STEEM'],
'sbd': acc.balances['total']['SBD']
}
def print_info(acc_info):
"""requires the "get_acc_info" function.
Prints some information as a string.
"""
name = acc_info['name']
sp = acc_info['sp']
rep = acc_info['rep']
steem = acc_info['steem']
sbd = acc_info['sbd']
print(f'\n{name} has a reputation of {rep}.\n' +
f'The account has {sp} SP, {steem} STEEM and {sbd} SBD.')
def main():
if len(argv) < 2:
print('No username was passed as argument.')
exit(1)
for username in argv[1:]:
acc_info = get_acc_info(username)
print_info(acc_info)
exit(0)
if __name__ == '__main__':
main()
I will not go into every little detail here. However, I will explain some possibly unusual procedures.
- The first line
#!/usr/bin/env python3
is the shebang. The use of shebang is common and recommended for Linux scripts. With this, the script can be called directly from the console, because it determines with which program it is to be executed. - The script uses
argv
to pass the names of Steemit users as arguments.
I intentionally used f-strings to highlight a special feature of containers. Because f-strings are only available from Python version 3.6. For example, the LTS version of Ubuntu 16.04 only offers Python version 3.5 by default. That would give you some trouble here. However, because the content of the containers is independent of the packages of the installed operating system, this is not a problem.
At this point a thank you to @themarkymark, who brought me to it with his post.
Write the Dockerfile for the application
From here you should have read my first tutorial.
Alternatively, you can simply replace the first line of the docker file with FROM maxpatternman/steem-python-base:tut-2018-04
.
Before we start we should check our directory structure again.
Currently our folder structure should look something like this:
This view was generated with tree
command.
.
├── 00_base
│ ├── alpine.tar.gz
│ └── Dockerfile
├── 01_dependencies
│ └── Dockerfile
└── 02_applications
- Now we create a new directory in the directory "02_applications" with the name of the application. I have chosen "print_userinfo".
- In this directory we create a new directory called "data" and a new file named "Dockerfile".
- In the folder "data" we create a file name named "app.py" and copy the code from the previous step into it.
The directory structure should now look like this:
.
├── 00_base
│ ├── alpine.tar.gz
│ └── Dockerfile
├── 01_dependencies
│ └── Dockerfile
└── 02_applications
└── print_userinfo
├── data
│ └── app.py
└── Dockerfile
Now that our directory structure is ready, we can write the dockerfile for our app.
FROM steem-python
COPY ./data /app
RUN chmod u+x /app/app.py
WORKDIR /app
ENTRYPOINT ["/app/app.py"]
Code | Description |
---|---|
FROM steem-python | "steem-python" is the name we created in the previous tutorial for the dependency layer. |
COPY ./data /app | Creates a new directory /app in the container and copies the entire contents of /data into it. |
RUN chmod u+x /app/app.py | Makes app.py executable. |
WORKDIR /app | /app is now the default directory. |
ENTRYPOINT ["/app/app.py"] | Means that our program app.py is executed at container startup and all arguments we pass from Docker are sent directly to app.py. This saves us a command when executing the app later. |
Build the application image
We have already carried out this step twice.
Here is the procedure:
- Open your terminal. Windows users please use the PowerShell.
- Navigate to the "print_userinfo" directory.
docker build -t print_userinfo .
Sending build context to Docker daemon 4.608kB
Step 1/5 : FROM steem-python
---> 2095d0dc650a
Step 2/5 : COPY ./data /app
---> Using cache
---> b5b8f67e822c
Step 3/5 : RUN chmod u+x /app/app.py
---> Running in 64e39bf2e898
Removing intermediate container 64e39bf2e898
---> bcdebd054d26
Step 4/5 : WORKDIR /app
Removing intermediate container 17ccccd3e819
---> b002322c6e7d
Step 5/5 : ENTRYPOINT ["/app/app.py"]
---> Running in 945416f630e9
Removing intermediate container 945416f630e9
---> 30bdd0738cb1
Successfully built 30bdd0738cb1
Successfully tagged print_userinfo:latest
Run the application
Finally we are able to run our app.
I will demonstrate it using @ned, @themarkymark and @limesoda as examples.
docker run --rm -t print_userinfo ned themarkymark limesoda
Code | Description |
---|---|
--rm | Removes the container after it has done its work. This is exactly what is meant by disposable containers. |
-t | Is optional. This ensures that the outputs are always displayed immediately. Without this parameter, only the entire output is displayed when the script is finished. |
We're getting the following output:
ned has a reputation of 69.03.
The account has 3602597.869 SP, 111873.055 STEEM and 7790.541 SBD.
themarkymark has a reputation of 70.18.
The account has 10333.122 SP, 4812.131 STEEM and 30.486 SBD.
limesoda has a reputation of 55.7.
The account has 7060.824 SP, 4.713 STEEM and 20.454 SBD.
That's it, we have finally created our own Docker Container App.
Now, of course, you can create additional apps on this basis. Simply create a new directory with a new application in the "02_applications" directory and repeat the procedure from this tutorial.
In the next tutorial we will look at the orchestration of different containers.
Curriculum
Posted on Utopian.io - Rewarding Open Source Contributors
Thanks for the contribution, it has been approved.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Thanks for the contribution, it has been approved.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Awesome post! I like it :) 👍
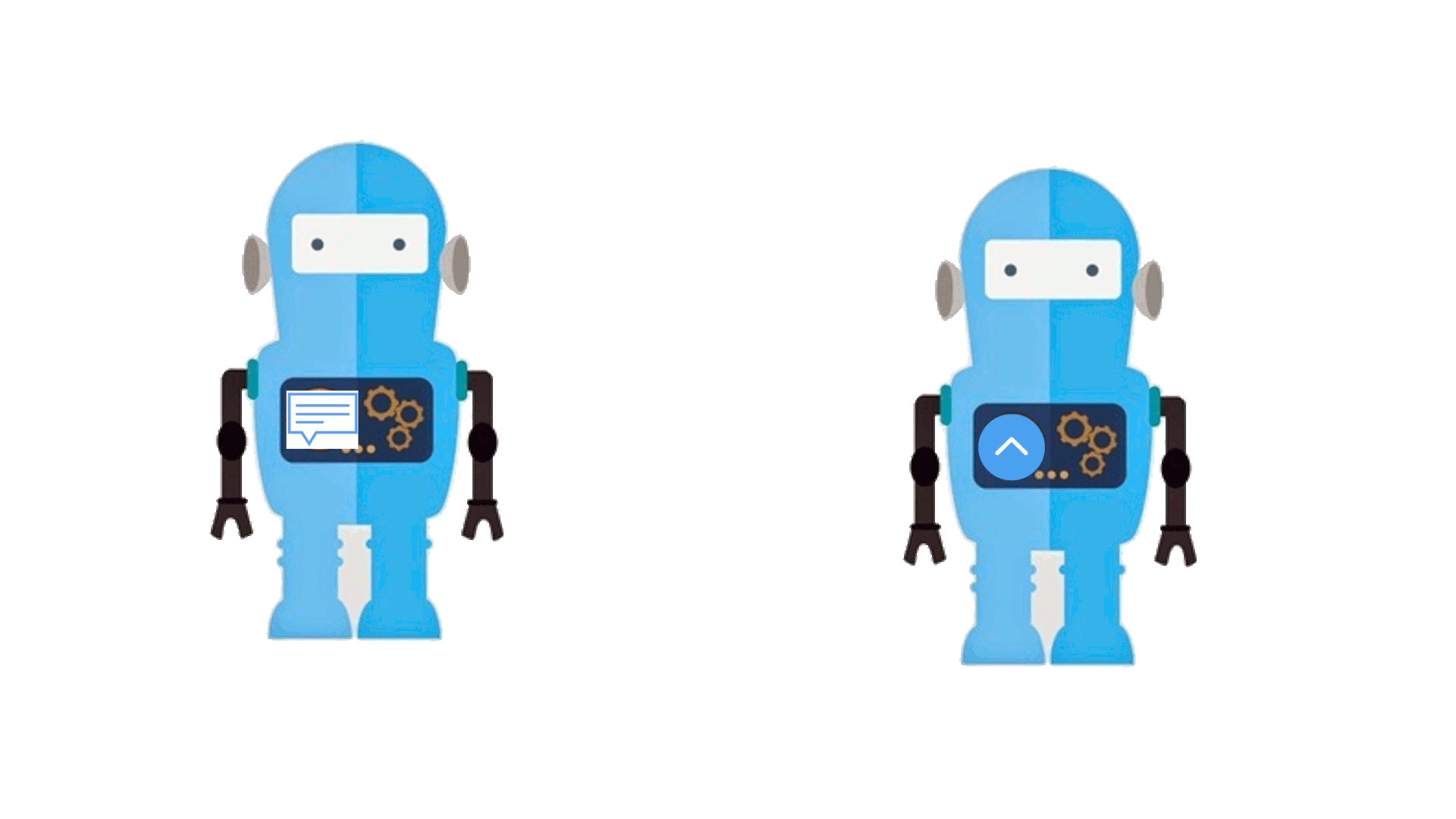
You got an upvote!
For more upvotes follow this account 👊🏼
Hey @maxpatternman I am @utopian-io. I have just upvoted you!
Achievements
Utopian Witness!
Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Nice Post dear. Looking forward to read your next post.
I have followed you, follow me back to be getting up votes from me on your future posts. https://steemit.com/@nwanne
Thank you.
Nice reputation level, dear. Not looking forward to read your next comments.