Java Tutorial: how to create simple java gui for chatting
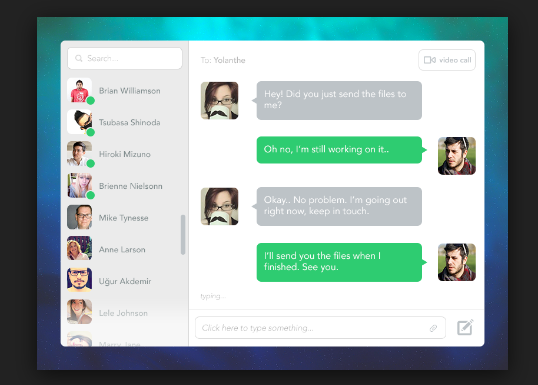
What Will I Learn?
- You will learn how to create graphics user interface GUI on netbeans.
- You will learn how to create program to send and recieve message using netbeans
- You will learn how to create simple chatting App
- You will learn how to implement program to jFrame project
Requirements
- you must know about java programming
- you must know about the items on NetBeans jFrame
- you must know about NetBeans tools
- NetBeans
Difficulty
- Intermediate
Tutorial Contents
okay, today I will make a tutorial how to make a simple chat application, here we will make two interconnected program so that can do chatting process using java programming language on netbeans.
okay, we just go into the tutorial.
- the first step you should do is open the netbeans application.
- create new project
- select java catagories, and project java application.
- then name it for your new project, then click finish
- then right click on the project you have created then select new, then select jFrame.
- then create JFrame class and name it for your jFrame class, then click finish.
- then, design your GUI application, here I just make a simple GUI for both server.
- then change the variable name to each component of the GUI you created by right clicking on the object you want to change the variable.
- then fill the code in the source section for your jFrame class.
- below is the code that you have to enter into the source JFrame class you have created
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.file.Path;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
public class ProgramKirim1 extends javax.swing.JFrame {
int Port =Integer.parseInt(JOptionPane.showInputDialog("Input Your Port : "));
String IP = JOptionPane.showInputDialog("Input Your IP Server : ");
public ProgramKirim1() {
initComponents();
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
pilihFile = new javax.swing.JButton();
sendFile = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
txtPath = new javax.swing.JTextArea();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
pilihFile.setLabel("Choose File");
pilihFile.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
pilihFileActionPerformed(evt);
}
});
sendFile.setLabel("Send File");
sendFile.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
sendFileActionPerformed(evt);
}
});
txtPath.setColumns(20);
txtPath.setRows(5);
jScrollPane1.setViewportView(txtPath);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(2, 2, 2)
.addComponent(pilihFile)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(sendFile)
.addGap(0, 0, Short.MAX_VALUE))
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 293, Short.MAX_VALUE)))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(66, 66, 66)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(pilihFile)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 103, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(sendFile)
.addContainerGap(90, Short.MAX_VALUE))
);
pack();
} private void pilihFileActionPerformed(java.awt.event.ActionEvent evt) {
JFileChooser pilih=new JFileChooser();
int confirm=pilih.showOpenDialog(null);
if(confirm==JFileChooser.APPROVE_OPTION){
Path path=pilih.getSelectedFile().toPath();
txtPath.append(path.toString());
}
}
private void sendFileActionPerformed(java.awt.event.ActionEvent evt) {
try {
int buffer_size=1024;
byte[] buffer = new byte[buffer_size];
ServerSocket servsock = new ServerSocket(Port);
Socket sock = servsock.accept();
System.out.println("koneksi terbentuk");
String folderFile=txtPath.getText();
BufferedInputStream in = new BufferedInputStream(new FileInputStream(folderFile));
BufferedOutputStream out = new BufferedOutputStream(sock.getOutputStream());
int len;
while((len = in.read(buffer)) > 0){
out.write(buffer,0,len);
}
out.flush();
servsock.close();
sock.close();
in.close();
out.close();
} catch (IOException ex){
Logger.getLogger(ProgramKirim1.class.getName()).log(Level.SEVERE, null, ex);
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(ProgramKirim1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) { java.util.logging.Logger.getLogger(ProgramKirim1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex){ java.util.logging.Logger.getLogger(ProgramKirim1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(ProgramKirim1.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new ProgramKirim1().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JButton pilihFile;
private javax.swing.JButton sendFile;
private javax.swing.JTextArea txtPath;
// End of variables declaration
}
- this is the declaration of the class name send public class Send writing class names must be capitalized, and should not use spaces.
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.file.Path;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
- this
Throws IOException
Is A Method That Reads String Data Input - this is a variable declaration process
port int Port =Integer.parseInt(JOptionPane.showInputDialog
withJOptionPane
input method. initComponent();
is the method generated by netbeans by default. Then there is also a methodgetter
andsetter
for the userList variable.- Modifier
private
on data: means data can only be accessed from the same class. JFileChooser
is a component of Java Swing that serves to provide convenience to the user to select / open the file.public int showOpenDialog(Component parent)
throws HeadlessException.BUFFER_SIZE 1024
Buffer management macros.java.net.ServerSocket
used by Server to listen to connections.java.net.Socket
used by Client for connection initialization.BufferedOutputStream
can be used for reading and writing binary data from a file.out.flush();
Allocate new memory to ObjectInputStream.servsock.close();
serves to close the file.- this is a mandatory class and can not be changed
// Variables declaration - do not modify
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JButton pilihFile;
private javax.swing.JButton sendFile;
private javax.swing.JTextArea txtPath;
- okay, next is full code for the recipient program, I do not explain anymore about this program because the syntax is same with previous program only different in some part only.
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.Socket;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
public class ProgramTerima extends javax.swing.JFrame {
int Port =Integer.parseInt(JOptionPane.showInputDialog("Input Your Port : "));
String IP = JOptionPane.showInputDialog("Input Your IP Server : ");
public ProgramTerima() {
initComponents();
}
<editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jScrollPane1 = new javax.swing.JScrollPane();
txtPath = new javax.swing.JTextArea();
saveCommand = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jPanel1.setBackground(new java.awt.Color(204, 153, 0));
txtPath.setColumns(20);
txtPath.setRows(5);
jScrollPane1.setViewportView(txtPath);
saveCommand.setLabel("Simpan");
saveCommand.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
saveCommandActionPerformed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(saveCommand)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 317, Short.MAX_VALUE)
.addContainerGap())
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(117, 117, 117) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(saveCommand)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 75, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(156, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
pack();
}// </editor-fold>
private void saveCommandActionPerformed(java.awt.event.ActionEvent evt) {
JFileChooser pilih=new JFileChooser();
pilih.setDialogTitle("Tentukan Tempat Penyimpanan");
int userSelection = pilih.showSaveDialog(null);
if(userSelection == JFileChooser.APPROVE_OPTION){
File fileToSave = pilih.getSelectedFile();
System.out.println("Save as file : "+ fileToSave.getAbsolutePath());
txtPath.append(fileToSave.getAbsolutePath());
try {
int buffer_size=1024;
byte[] buffer = new byte[buffer_size];
Socket socket = new Socket("127.0.0.1",Port);
BufferedInputStream in = new BufferedInputStream(socket.getInputStream());
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(fileToSave));
int len;
while((len = in.read(buffer)) > 0){
out.write(buffer,0,len);
}
out.flush();
socket.close();
in.close();
out.close();
} catch (IOException ex){
Logger.getLogger(ProgramTerima.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(ProgramTerima.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) { java.util.logging.Logger.getLogger(ProgramTerima.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(ProgramTerima.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(ProgramTerima.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new ProgramTerima().setVisible(true);
}
});
}
// Variables declaration - do not modify
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JButton saveCommand;
private javax.swing.JTextArea txtPath;
// End of variables declaration
}
- okay, we'll run two programs we've created
- then write the message you want to send.
- Okay, then we run the recipient program.
- okay, the message we sent successfully entered the recipient program, meaning that both programs we created successfully.
Pos yg bagus kawan saya selalu menikmati pos ini
Thank you
Terima kasih kembali kawan jangan lupa upvote aku ya
Very interesting
euh hai ke interesting
Very high quality post
Your contribution cannot be approved because it does not follow the Utopian Rules.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]