Using Steem-API with Ruby Part 5 — Print Current Median History Price
Repositories
SteemRubyTutorial
You can find all examples from this tutorial as fully functional scripts on GitHub:
steem-ruby
- Project Name: Steem Ruby
- Repository: https://github.com/steemit/steem-ruby
- Official Documentation: https://github.com/steemit/steem-ruby
- Official Tutorial: N/A
radiator
- Project Name: Radiator
- Repository: https://github.com/inertia186/radiator
- Official Documentation: https://www.rubydoc.info/gems/radiator
- Official Tutorial: https://developers.steem.io/tutorials-ruby/getting_started
What Will I Learn?
This tutorial shows how to interact with the Steem blockchain and Steem database using Ruby. When using Ruby you have two APIs available to chose: steem-api and radiator which differentiates in how return values and errors are handled:
- steem-api uses closures and exceptions and provides low level computer readable data.
- radiator uses classic function return values and provides high level human readable data.
Since both APIs have advantages and disadvantages I have provided sample code for both APIs so you can decide which is more suitable for you.
In this instalment of the tutorial you learn how to access the conversion rate of STEEM and Steem Backed Dollars (SBD).
Requirements
You should have basic knowledge of Ruby programming you need to install at least Ruby 2.5 as well as the following ruby gems:
gem install bundler
gem install colorize
gem install steem-ruby
gem install radiator
Note: Both steem-ruby and radiator provide a file called steem.rb
. This means that:
- When you install both APIs you need to tell ruby which one to use.
- You can't use both APIs in the same script.
If there is anything not clear you can ask in the comments.
Difficulty
Provided you have some programming experience this tutorial is basic level.
Tutorial Contents
In the last part of the tutorial we took a look at the account wallet where we noted that steem power is shown on STEEM and not VESTS. If you look at the screen shot again you will notice that in the last row the estimated account value is quoted in '$':
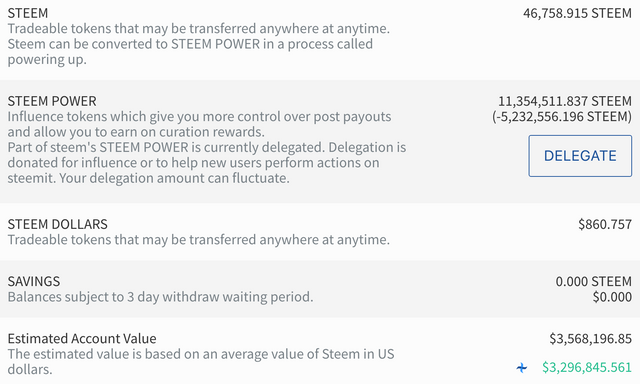
It is important to know that this is not the account value in US$. Instead it's the account value in Steem Backed Dollars (SBD). One SBD is supposed to be about one US$ but this can vary. Which leads to the question: What is the exchange rate between SBD and STEEM?
This isn't documented in the official API documentation or the official tutorials. Unlike VESTS to STEEM conversion which is well documented.
What is documented is get_current_median_history_price
. This value is a rolling median price for SBD and is provided by the Witnesses. Being a rolling median it's smother but also a little behind the actual price.
The get_current_median_history_price
can be read using call to the Condenser_Api
API. As you can see it returns not one but two values called base
and quote
and they are both ridiculously large:
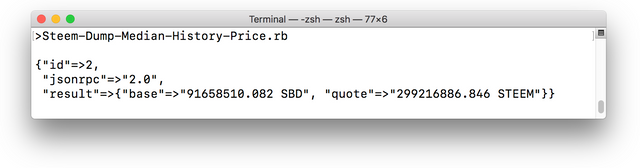
How they are used isn't documented but it's possible to deduct the usage from the types: base
is in SDB and quote
in STEEM so a division is the way to go:
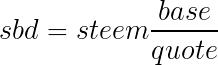
Implementation using steem-ruby
Reading the get_current_median_history_price
is pretty straight forward and similar to what you have learned Part 3. The actual code samples are rather short after this much theory.
begin
Create instance to the steem condenser API which will give us, among other things, access to the median history price.
Condenser_Api = Steem::CondenserApi.new
Read the median history. Yes, it's as simple as this.
Median_History_Price = Condenser_Api.get_current_median_history_price
Calculate the conversion Rate for STEEM to SBD. We use a trick here: to_f
ignores the text after the space which simplifies the code.
_base = Median_History_Price.result.base
_quote = Median_History_Price.result.quote
Conversion_Rate_Steem = _base.to_f / _quote.to_f
rescue => error
I am using Kernel::abort
so the code snipped including error handler can be copy pasted into other scripts.
Kernel::abort("Error reading global properties:
".red + error.to_s)
end
Pretty print the result. It might look strange to do so outside the begin / rescue but the value is now available in a constant for the rest of the script. Do note that using a constant is only suitable for short running script. Long running scripts would need to re-read the value on a regular basis.
pp Median_History_Price
Show some actual sample conversion rates:
puts ("1.000 STEEM = %1$15.3f SBD") % (1.0 * Conversion_Rate_Steem)
puts ("1.000 SBD = %1$15.3f STEEM") % (1.0 / Conversion_Rate_Steem)
Hint: Follow this link to Github for the complete script with syntax highlighting: Steem-Dump-Median-History-Price.rb.
The output of the command (for the steem account) looks like this:
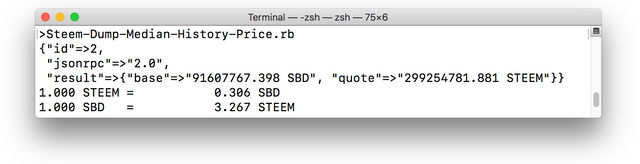
Implementation using radiator
It's pretty much indentical to the radiator implementaiton.
begin
Create instance to the steem condenser API which will give us access to the median history price.
Condenser_Api = Radiator::CondenserApi.new
Read the median history. Yes, it's as simple as this.
Median_History_Price = Condenser_Api.get_current_median_history_price
Calculate the conversion Rate for STEEM to SBD. We use a trick here: to_f
ignores the text after the space which simplifies the code.
_base = Median_History_Price.result.base
_quote = Median_History_Price.result.quote
Conversion_Rate_Steem = _base.to_f / _quote.to_f
rescue => error
I am using Kernel::abort
so the code snipped including error handler can be copy pasted into other scripts.
Kernel::abort("Error reading global properties:
".red + error.to_s)
end
Pretty print the result. It might look strange to do so outside the begin / rescue but the value is now available in a constant for the rest of the script. Do note that using constant is only suitable for short running script. Long running scripts would need to re-read the value on a regular basis.
pp Median_History_Price
Show some actual sample conversion rates:
puts ("1.000 STEEM = %1$15.3f SBD") % (1.0 * Conversion_Rate_Steem)
puts ("1.000 SBD = %1$15.3f STEEM") % (1.0 / Conversion_Rate_Steem)
Hint: Follow this link to Github for the complete script with syntax highlighting: Steem-Print-Median-History-Price.rb.
The output of the command (for the steem account) looks identical to the previous output:
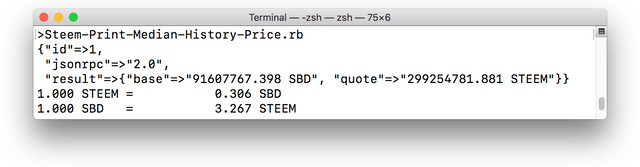
Curriculum
First tutorial
Previous tutorial
Next tutorial
Proof of Work
Image Source
- Ruby symbol: Wikimedia, CC BY-SA 2.5.
- Steemit logo Wikimedia, CC BY-SA 4.0.
- Screenshots: @krischik, CC BY-NC-SA 4.0
Beneficiary







Thank you for your contribution @krischik.
After analyzing your tutorial we suggest the following points below:
Thanks for following some suggestions from your last tutorial.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin! Keep up the good work!
The theory of VESTS vs STEEM vs SBD is indeed quite complicated and difficult to describe. The next part will have a lot more code. Sill i might go over the text again and reword for shorter simpler sentences.
@portugalcoin I have simplified the sentence construction a bit. There was also a bit of sample code missing which I corrected.
Hey, @krischik!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @krischik!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hi, @krischik!
You just got a 0.94% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.