Creating Slideshow Images using C#
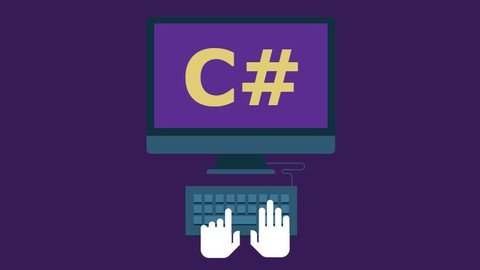
C# is programming language designed for creating a variety of applications that run on the .NET Framework. These language is productive, multi-purpose, type-safe, open source, and object-oriented, They are built on the .NET Compiler Platform which provides rich code analysis APIs. image source
In this tutorial, we will create a simple slideshow with the use of Windows Forms pictureBox and timer written in C#. It can play basic slideshow for images without using special effects.
What Will I Learn?
- To use the pictureBox and Timer in C#
- To create slideshow without effects in window mode.
Requirements
To run, you need
- .NET 3.5 (or above)
To compile, you need
- C# Compiler such as: Visual Studio
Difficulty
- Basic
Tutorial Contents
Open the Visual Studio and Create New Project(In my case, i'm using Visual Studio 2010)
A new window will appear, click the Windows Forms Applications to choose what type of project application you want to use. Change the Name of the Project and it's Location you want to it be save. And click OK.
You will now see your template of design in project application using the Windows Forms
To put the pictureBox in the Form, click the Toolbox at the left corner and find the PictureBox then drag it to the Form.
To import image to the pictureBox, go to the Properties located at the lower right of the window and find Image then click the (...)
The Select Resource Window will appear, click Import to import images and select files you want to import.
Images will be now put into the Project Resource file.
Now change the SizeMode of PictureBox into Zoom to have good view on image.
Now manually put an image to the picturebox, go to Image and choose the desired image you want to put in a certain picturebox. So in my case, i have 10 images so i must have also 10 pictureboxes which i created and just overlapping each other.
Next is to set the interval of each picture or the delay on switching the images, to do this go to Toolbox and find the Timer then drag it to the Form.
Set the interval to 1 second, to do this go to the Properties of timer and change the Interval to 1000.
The Code
To go to the code, double click the Form and this new window will appear.
Notice that it already import all the libraries we need and created the functions that we will use.
First we have to do is to set all of the picturebox not visible so that if the form will load all images will not be seen. To do this create a void function setting all the visibility of picturebox to false.
public void pictureboxFalse()
{
pictureBox10.Visible = false;
pictureBox9.Visible = false;
pictureBox8.Visible = false;
pictureBox7.Visible = false;
pictureBox6.Visible = false;
pictureBox5.Visible = false;
pictureBox4.Visible = false;
pictureBox3.Visible = false;
pictureBox2.Visible = false;
pictureBox1.Visible = false;
}
Next is to put the code looping the images to be visible and not visible in the timer function, to do this double click the timer in the design and you will be redirected to the function code of the timer.
private void timer1_Tick(object sender, EventArgs e)
{
if (pictureBox1.Visible == true)
{
pictureBox1.Visible = false;
pictureBox2.Visible = true;
}
else if (pictureBox2.Visible == true)
{
pictureBox2.Visible = false;
pictureBox3.Visible = true;
}
else if (pictureBox3.Visible == true)
{
pictureBox3.Visible = false;
pictureBox4.Visible = true;
}
else if (pictureBox4.Visible == true)
{
pictureBox4.Visible = false;
pictureBox5.Visible = true;
}
else if (pictureBox5.Visible == true)
{
pictureBox5.Visible = false;
pictureBox6.Visible = true;
}
else if (pictureBox6.Visible == true)
{
pictureBox6.Visible = false;
pictureBox7.Visible = true;
}
else if (pictureBox7.Visible == true)
{
pictureBox7.Visible = false;
pictureBox8.Visible = true;
}
else if (pictureBox8.Visible == true)
{
pictureBox8.Visible = false;
pictureBox9.Visible = true;
}
else if (pictureBox9.Visible == true)
{
pictureBox9.Visible = false;
pictureBox10.Visible = true;
}
else if (pictureBox10.Visible == true)
{
pictureBox10.Visible = false;
pictureBox1.Visible = true;
}
}
And lastly, put these line of codes to the function Form1_Load to begin the slideshow.
private void Form1_Load(object sender, EventArgs e)
{
pictureboxFalse(); //this sets all the picturebox to false
pictureBox1.Visible = true; //sets the pictureBox1 visiblity true to begin the loop
timer1.Start(); // this begins the loop
}
Thanks for Reading and Happy Coding
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for your contribution, yet it cannot be approved. AFAIK C# is not open source and has no matching github repo, and the repository you selected actually relates to your code, this is no longer permissible as per the new rules effective yesterday.
You can contact us on Discord.
[utopian-moderator]
Hey @mcfarhat, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
It's an excellent tool, but I use other services for slideshows too. Our company is developing new formulas to create facial skincare products. We present each new idea to company executives in a presentation. Visuals and presentation play one of the key roles, so we need to present a quality, well-thought-out product. Creating a slideshow of images, we use the service https://www.fastreel.com/slideshow-maker.html. There you can make a slideshow and edit the finished video. We create fantastic presentations of our new products and surprise company executives.