Implementing Syde, An online Forum in Flutter (Update 4: Chatroom, Notification,Bookmark a story)
Repository
https://github.com/enyason/Syde
History
Implementing Syde An online Forum in Flutter (Update 3 : Add Comments to a story)
Implementing Syde An online Forum in Flutter (Update 2 : Post A Story, Retrieve All Stories)
Implementing Syde An online Forum in Flutter (Update: Authenticate With Google)
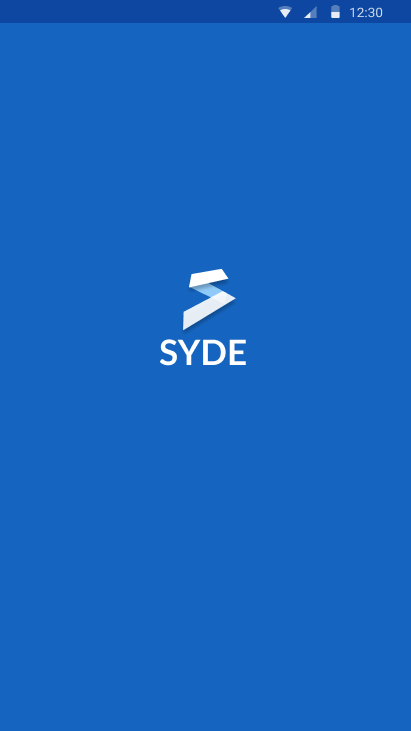
New Features
Chat Room(Syde Chat)
Bookmarking a story
User Dash Board
Adding Image Content(Chat Room and Creating a Story)
Chat Room
An interesting part of syde is the chatroom feature. Users on the platform can all communicate right from the chatroom.
Implementation
Implementing this part of the platform, FireStore of FireBase was used. FireStore NoSQL cloud database to store and sync data for the client- and server-side development. Firebase handles most of the back-end complexities allow the developer to focus on implementing important features of a product. In the chatroom, users can send two types of messages, either a text or an image.
- Getting Image from Phone
This was done by the help of a photo picker library https://pub.dartlang.org/packages/image_picker
The code block below shows this:
Future getImage() async {
_image = await ImagePicker.pickImage(source: ImageSource.gallery);
uploadFile();
}
Basically, I call to the device gallery to pick an image and the response is stored to a global variable of type File.
Uploading Image to storage
Image contents from the phone is sent to firebase and stored in the storage bucket
Future uploadFile() async { //upload file to server String fileName = DateTime.now().millisecondsSinceEpoch.toString()+_image.path; StorageReference reference = FirebaseStorage.instance.ref().child("syde_chat").child(fileName); StorageUploadTask uploadTask = reference.putFile(_image); StorageTaskSnapshot storageTaskSnapshot = await uploadTask.onComplete; storageTaskSnapshot.ref.getDownloadURL().then((downloadUrl) { _imageUrl = downloadUrl; setState(() { // isLoading = false; _handleSubmitted(_imageUrl, 1); }); }, onError: (err) { Toast.show('This file is not an image',context,duration: 1,backgroundColor: Colors.grey); }); }
The file to upload is renamed to the current time in millisecond plus its file path
reference to the storage is made and then upload the image.
From the snapshot return, I get the link to the URL then pass it to the function that sends the entire message content to the database.
Send message content to server
The code block below handles sending the message content to the server
void _handleSubmitted(String text, int type) async { //submit message to server if (text .trim() .length < 1) return; _textController.clear(); var map = { "photoUrl": currentUser.photoUrl, "message": text, "sender": currentUser.displayName, "type": type, "sender_id": currentUser.uid, "timestamp": DateTime .now() .millisecondsSinceEpoch .toString() }; DocumentReference documentReference = Firestore.instance .collection("chatroom") .document(DateTime .now() .millisecondsSinceEpoch .toString()); Firestore.instance.runTransaction((transaction) async { await transaction.set(documentReference, map); }); } }
The message content is structured in a map object.
A reference to the chat Collection is gotten then a transaction query is made.
A transaction is used, to prevent any potential race condition.
Reveal Images in Detailed Form
In the chat room screen, image content shared is shown in a little space. To reveal this image in detail, users can click on it, then another screen comes up displaying the image in full. The code block below reveals that
class PhotoDialog extends StatelessWidget { final String _image; const PhotoDialog(this._image); @override Widget build(BuildContext context) { return Scaffold( backgroundColor: Colors.black, body: Center( child: Container( height: 500.0, width: double.infinity, color: Colors.black, child: PhotoView( imageProvider: CachedNetworkImageProvider(_image), ) ), ), ); } }
- The photo view is a widget that permits zooming of images. This widget is made available from this library https://pub.dartlang.org/packages/photo_view
Bookmark a story
Users can now save a post to read later
Implementation
First, get users list of bookmark stories then use the id to fetch the individual stories to display a list;
Widget getId() {
return FutureBuilder(
future: Firestore.instance
.collection("saved_story")
.document(uid)
.collection("bookmark")
.orderBy("time_stamp", descending: true)
.getDocuments(),
builder: (context, snapShot) {
if (snapShot.connectionState == ConnectionState.waiting) {
return Center(
child: CircularProgressIndicator(
strokeWidth: 2,
valueColor: AlwaysStoppedAnimation<Color>(
Colors.black)),
);
}
QuerySnapshot shot = snapShot.data;
if (snapShot.hasData) {
if ((shot.documents.length) < 1) {
return Center(child: Text("No Story found"));
}
return makeNotificationItem(shot.documents);
} else {
return Center(child: Text("No Story found"),);
}
}
);
}
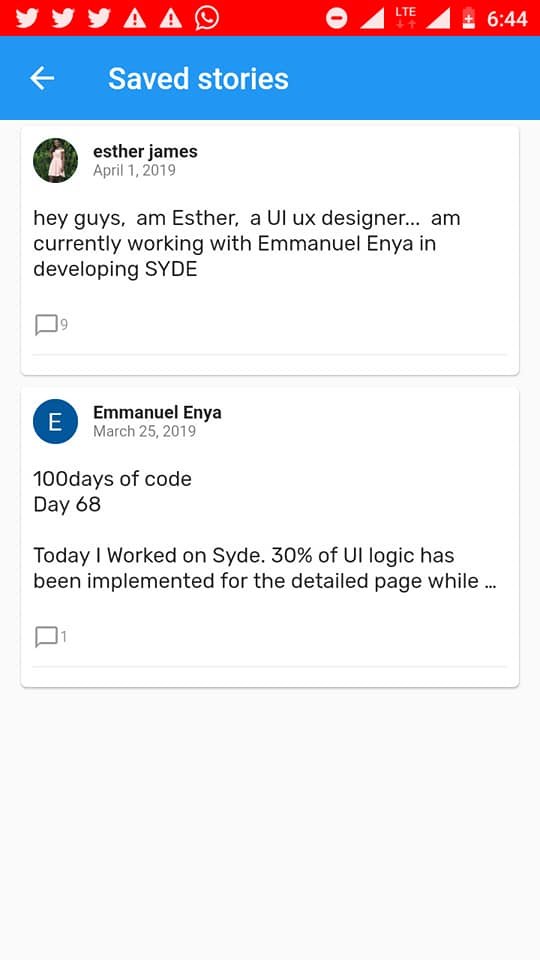
User Dash Board
User can view the profile and that of others on the platform
***Implementation ***: This was done using the users ID. with the user ID, information regarding a particular user is extracted. When the current user navigates to his profile, he as the access to edit his bio while other users can only view it
Notification
Part of syde is for users to get notified of upcoming events and job opportunities. The image below shows the implementation done.
Adding Image Content When Creating a Story
Recently updated syde to allow users to create a story with image content. The maximum number of images is two and they can see a preview of the images at the bottom of the page before preceding to make a post. Image below reveals this. To see this implementation, check the demo video to get a good view.
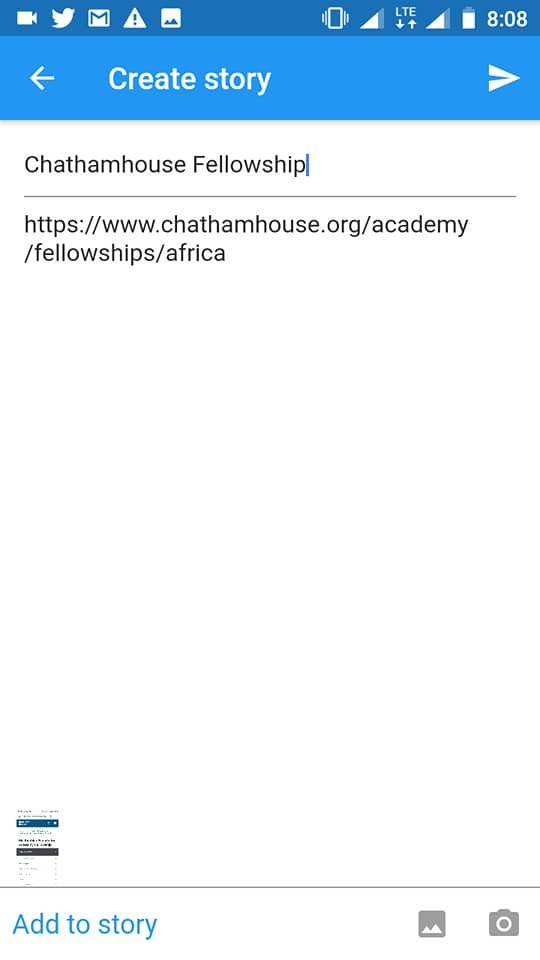
N/B
- I have introduced the bloc library to help implement the bloc pattern for effective state management. So far, i have re-factored the authentication and log in to work with the library.
- Also, I have included a license to the repository, as advised by utopian moderators.
Demo Video
IMPORTANT RESOURCES
*Github *: https://github.com/enyason/Syde
Commits
Commits on Apr 19, 2019
Commits on Apr 12, 2019
Commits on Apr 11, 2019
- created notification detailed page to display full notification details
- updated the UI of the notification list page
- get data snapshot with future builder rather than a stream builder
- get data snapshot with future builder rather than a stream builder
- added a delay of 200ms in between closing the nav drawer and opening … …
- refactored the bookmark screen to work with future builder rather tha… …
- implemented the bookmark screen
RoadMap
Authenticate UserPersisting user postDisplaying all postDetailed screen implementationStory Reaction Implementation (like and unlike a story)- Searching all posts
Providing different layoutsDisplay Notification- ToDo for users to track their daily progress
- Push Notifications
- Posts sharing
Users dashboard- Searching all users
- Direct Messaging with Users
ChatGroupBookmarking post
How to contribute?
You can reach me by commenting on this post or send a message via [email protected] you want to make this application better, you can make a Pull Request.
//
, why is that?Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thanks @helo for your suggestions. I'll improve on my presentation on the next update
Thank you for your review, @helo! Keep up the good work!
Congratulations @ideba! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
You can view your badges on your Steem Board and compare to others on the Steem Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Vote for @Steemitboard as a witness to get one more award and increased upvotes!
Hi, @ideba!
You just got a 1.03% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
Hi @ideba!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @ideba!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!