Steem-js and Materialize Css Join Forces #4: Showing posts with different tags
Repository
- https://github.com/steemit/steem-js
- https://github.com/Dogfalo/materialize
- https://github.com/jquery/jquery
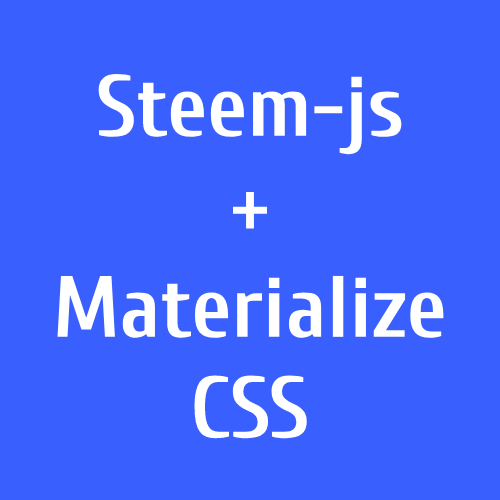
In #4 what i cover...
- Adding beautiful navbar to a webpage where steemit text is the logo
- Create a side panel and a hamburger button to open it.
- Listing steemit trending tags inside side panellist.
- Showing all different types of posts based on tags.
The Major Update
- Removed old inconsistent features in code and the code is fresh now.
Requirements :
- Get Materialize Css from Here and set up in your project directory.
- Get steem-js from here and follow the official GitHub page instructions to setup steem-js in your project directory.
- Get Jquery minified version from Here and the website instruction to add it into your project.
- Any Text Editor is required (Vs code/ Atom or any other).
- Basic Understanding of Materialize CSS collections
- Basic Understanding of Materialize CSS tabs
- Basic understanding of Sidenav panel sidenav
- Basic understanding of nav bar setup nav
Difficulty
Choose one of the following options:
- Intermediate
Tutorial Contents
I will be covering that how to add nav bar to the web page then, how to maintain list of trending tags inside side nav panel and then showing posts by tags
Make sure to follow along. So, to do that you have to read this part #1 then part #2 and then part 3 .

Tutorial Starts From Here:
Download the latest repository at branch 3 or go to here and the latest one with this tutorial is here
The tutorial is divided into two parts :
Part 1:-
Add the following code in index.html
by removing all the previous code.
<!DOCTYPE html>
<html>
<head>
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
</head>
<body>
<nav>
<div class="nav-wrapper red lighten-2">
<a href="#" class="brand-logo center">Steemit</a>
</div>
</nav>
<ul id="slide-out" class="sidenav">
</ul>
<a href="#" data-target="slide-out" class="sidenav-trigger btn-floating btn-large waves-effect waves-light white lighten-2" style="margin-top : 1em;">
<i class="material-icons black-text">menu</i>
</a>
<div class="container">
<div class="row">
<div class="col s12">
<ul class="tabs">
<li class="tab col s3" ><a class="active" id='tab1' onclick="showView(0)">Trending</a></li>
<li class="tab col s3"><a onclick="showView(1)">Created</a></li>
<li class="tab col s3"><a onclick="showView(2)">Hot</a></li>
<li class="tab col s3"><a onclick="showView(3)">Promoted</a></li>
</ul>
</div>
</div>
<ul class="collection">
</ul>
<div class="row center">
<a class="btn-floating btn-large waves-effect waves-light red lighten-2" id="btn-a"><i class="material-icons">arrow_drop_down</i></a>
</div>
</div>
<script type="text/javascript" src="js/steem.min.js"></script>
<script type="text/javascript" src="js/jquery-3.3.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<script type="text/javascript" src="js/index.js"></script>
</body>
</html>
Explaining code:-
The first part in the body tag
<nav>
<div class="nav-wrapper red lighten-2">
<a href="#" class="brand-logo center">Steemit</a>
</div>
</nav>
It is a nav bar that is showing steemit at the top as header of the page.
It is a sidepanel
<ul id="slide-out" class="sidenav">
</ul>
A side panel will be hidden and only show at the click of a button which is this:
<a href="#" data-target="slide-out" class="sidenav-trigger btn-floating btn-large waves-effect waves-light white lighten-2" style="margin-top : 1em;">
Inside ul
i will append li
with getting trending tags.
Everything else has been covered in previous parts.
Done here, Too short? :) , Now it's Javascript time.

Part 2:-
Add this code in index.js while removing anything else in the file
var tag = "";
$(document).ready(function() {
$('.tabs').tabs();
showView(0);
$('.sidenav').sidenav();
//Getting list of trending tags at the start
steem.api.getTrendingTags("", 100, function(err, result) {
for (let i = 0; i < result.length; i++) {
if (result[i].name == "") {
$('.sidenav').append("<li><a class='waves-effect' href='#!' onclick=tagSet(\"" + result[i].name + "\")>All Tags</a></li>");
} else {
$('.sidenav').append("<li><a class='waves-effect' href='#!' onclick=tagSet(\"" + result[i].name + "\")>" + result[i].name + "</a></li>");
}
}
});
});
function tagSet(ct) {
tag = ct;
$('.collection').empty();
console.log("Deldsdsdeted");
console.log(tag);
window.location = $('#tab11').attr('class'); //To refocus tabs over first tab
showView(0);
}
//Running steem method on document ready when script tag initialized.
//There is not a need for document.ready funcion but you can use them for practice.
function showView(val) {
switch (val) {
case 0:
steem.api.getDiscussionsByTrending({
"tag": tag,
"limit": 10
}, function(err, result) {
console.log("trending");
setValues(err, result, 0);
});
$("#btn-a").unbind();
$("#btn-a").bind("click", function() { // Otherwise there will be if/else statement...
//If we have to optimize it a little more.
console.log("trending clicked");
steem.api.getDiscussionsByTrending({
"tag": tag,
"limit": 10,
"start_permlink": window.permlink,
"start_author": window.author
},
function(err, result) {
setValues(err, result, 1);
});
});
break;
case 1:
steem.api.getDiscussionsByCreated({
"tag": tag,
"limit": 10
}, function(err, result) {
console.log("c");
setValues(err, result, 0);
});
$("#btn-a").unbind();
$("#btn-a").bind("click", function() {
console.log("c clicked");
steem.api.getDiscussionsByCreated({
"tag": tag,
"limit": 10,
"start_permlink": window.permlink,
"start_author": window.author
},
function(err, result) {
setValues(err, result, 1);
});
});
break;
case 2:
steem.api.getDiscussionsByHot({
"tag": tag,
"limit": 10
}, function(err, result) {
setValues(err, result, 0);
});
$("#btn-a").unbind();
$("#btn-a").bind("click", function() {
console.log("h clicked");
steem.api.getDiscussionsByHot({
"tag": tag,
"limit": 10,
"start_permlink": window.permlink,
"start_author": window.author
},
function(err, result) {
setValues(err, result, 1);
});
});
break;
case 3:
steem.api.getDiscussionsByPromoted({
"tag": tag,
"limit": 10
}, function(err, result) {
setValues(err, result, 0);
});
$("#btn-a").unbind();
$("#btn-a").bind("click", function() {
console.log("p clicked");
steem.api.getDiscussionsByPromoted({
"tag": tag,
"limit": 10,
"start_permlink": window.permlink,
"start_author": window.author
},
function(err, result) {
setValues(err, result, 1);
});
});
break;
}
}
function setValues(err, result, post) {
// vm is used to get either the function is called from showing first posts or next...
if (post == 0) {
$('.collection').empty();
}
for (i = 0; i < result.length - 1; i++) {
//Looking for image, if existed then use the first of the post and if not then the use defined image.
let imageUrl = JSON.parse(result[i].json_metadata);
let image = "https://48tools.com/wp-content/uploads/2015/09/shortlink.png";
if (imageUrl.hasOwnProperty('image')) {
console.log("Has property image");
if (imageUrl.image[0] !== "") {
image = imageUrl.image[0];
} else {
image = "https://48tools.com/wp-content/uploads/2015/09/shortlink.png";
}
}
// Replace if any html tag occur in post
let bodyContent = result[i].body;
let prefined = bodyContent.replace(/(<([^>]+)>)/g, "");
//Append <ul> tag body with proper steem post image, title and description.
$(".collection").append("<li class='collection-item avatar'> \
<a href='http://steemit.com" + result[i].url + "' style='color: inherit;'> \
<img src='" + image + "' alt='' class='circle'> \
<span class='title' style='font-weight:bold;'>" + result[i].root_title + "</span> \
<p class='truncate'>" + prefined + "</p> \
</a> \
</br>\
</li>");
}
window.permlink = result[i].permlink;
window.author = result[i].author;
}
Explaining Code
Declared a variable at top
tag
var tag = "";
So by default it can start showing posts by selecting All Tags
Inside document ready function:
$('.tabs').tabs();
showView(0);
$('.sidenav').sidenav();
//Getting list of trending tags at the start
steem.api.getTrendingTags("", 100, function(err, result) {
for (let i = 0; i < result.length; i++) {
if (result[i].name == "") {
$('.sidenav').append("<li><a class='waves-effect' href='#!' onclick=tagSet(\"" + result[i].name + "\")>All Tags</a></li>");
} else {
$('.sidenav').append("<li><a class='waves-effect' href='#!' onclick=tagSet(\"" + result[i].name + "\")>" + result[i].name + "</a></li>");
}
}
});
I am initializing tabs and then calling function in start with default 0
parameter and then initializing Side panel and Getting all trending tags and appending under sidepanel ul
. In each tag also a
tag assigned so it will call a function with suitable parameter result[index].name
and also two conditions apply, firt if ""
comes in name it means to show All tags
and other wise keepup with same tag name.
Inside function
tagSet()
tag = ct;
$('.collection').empty();
console.log("Deldsdsdeted");
console.log(tag);
window.location = $('#tab11').attr('class'); //To refocus tabs over first tab
showView(0);
A suitable parameter passed having name of the tag and then removing all elements inside ul
so it can append from new and then useless console.log statements for you but useful for me beucase it helped a lot :p . Then focusing the tab view again on trending
with id becuase whenever it will load it will show posts on trending first (you can change it later but neither does steem not you want to (maybe)). After that we call showView(0)
to show the posts by passing conditional statement.
Added btn.bind and unbind method.
$("#btn-a").unbind();
While assigning different events to button click (you can use if/ else if you want) I have to remove the button's old listeners, as well, so I used unbind
before binding()
. More at jquery
Instead of passing
steemit
i am now usingtag
variable.I also removed the extra code from document ready function, Thanks to @portugalcoin to always pointing me out to my mistakes.
I have to use multiple block at somepoint or i can re structure the whole project because now i need to use two types of methods of steem one with simple query and other having
"start_permlink": window.permlink,
"start_author": window.author"
It can be removed but require more amazing amount of time that i think experts can handle it :p . Till then you can use the current structure of code and once find out a way or want to know how to reduce this then contact me on my discord server or you can get any type of help at utopian-io server (Make sure to put your request on the right channel) It's great community there.
Output:
Save all the files and then double click on
index.html
to open it inside the browser. Then the output display over it.
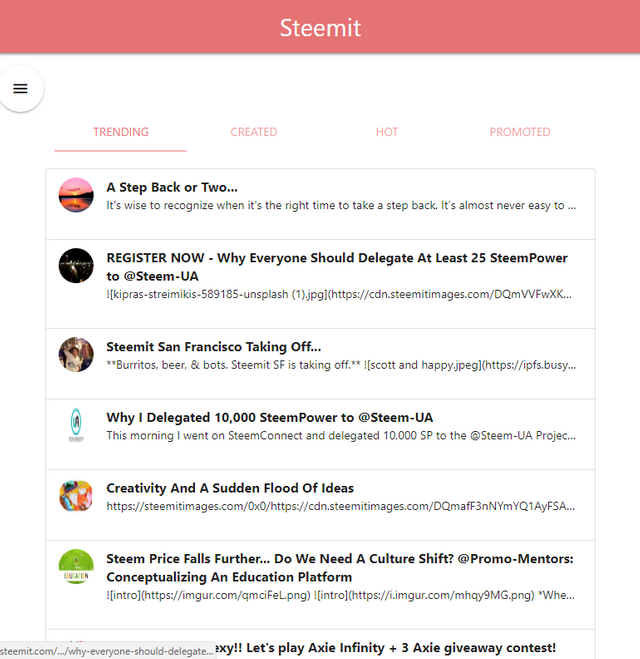.png)
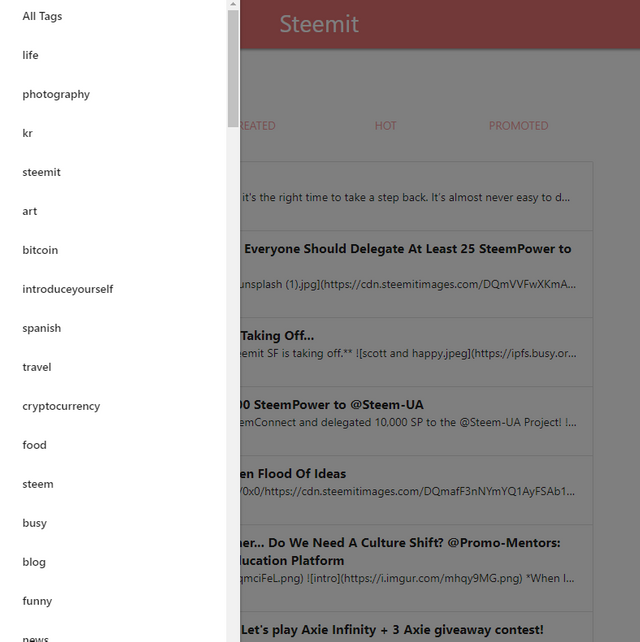.png)
Next:- This took around 3-4 hours direct while finding functions of materialize but i finally manage to get around and created it, If i get a chance then creates a preloader and showing more posts when user swiped at the height of the screen ( i mean when user gets at the end). You can create a mobile app with that code too, just put your code in cordova www. Will explain later if i get some time to do it.
Proof of Work Done
https://github.com/genievot/steem-posts-tut

Please dm or pm me if something needed to be changed, thank you so much.
I thank you for your contribution. Here are my thoughts;
What you really have shown is doing it by yourself, not teaching. You can avoid this by approaching more theoretical/technical to the subject. If you need examples, there are sites which offer professional tutorials. I won't include them because I don't want to advertise them, but checking them might change your approach to the tutorials and increase the value of your post.
The volume of your tutorial is too sparse. To increase it, please reduce the amount of the filler content (like too long code blocks - not all of them were filler, but nearly half of your post consists code blocks-) and add several (up to 4-5) substantial concepts.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @yokunjon!
So far this week you've reviewed 1 contributions. Keep up the good work!
well thank you for taking time and considering this post worth an upvote, I want to discuss a little about the points :
intermediate
for a point i am sure.I above post i have covered around 2 topics in neat details while creating ui as well but i will try to improve them , thanks.
And can you please tell what does exactly
substantial topic
means to you when you rate over it. Or some examples can help as well.Sorry, I had health issues so wasn't able to answer quickly.
First of all, I would like to state that the reviewing process and my personal feedback are usually unrelated. Because what dedicates your score is determined by a series of questions and my answers to them. So, that's in mind, what I suggested are my personal thoughts on how to improve your post in general. So, my answers to your post should be considered as the real review. Now, we can talk about your questions.
Here are the answers to your questions:
Yes, using examples is a way of teaching. But, this method must be used carefully. Because the student should be able to distinguish what you are doing and what you will accomplish when you finish the process. If a student does not have these qualifications, that is considered as spoon-feeding them as they don't know what they really do. Of course, in the end, the student might learn how and why he/she's doing that. But, that is a dangerous way and may lead to cargo-cult code, which would never be required. So, my feedback on that suggests this. You might or not follow it, but it is better to do it as many courses follow that approach.
Yes, I agree. A tutorial must be straightforward; and unnecessary, filler parts must be eradicated. But, the issue in your post isn't that. As we value volume of substantial concepts to determine the amount of work on the post has been done, your post is kinda small. That isn't an issue as some tutorials might be briefer or include less substantial concepts. But as I said, we use the volume of the substantial concepts to determine the amount of the work that has been done.
Substantial concepts are distinguishable concepts that cover different parts of a post. There is no standard for it, but usually, it can be easily distinguished. I considered that your post contains only one substantial concept as it is a brief example of using CSS and jquery together. It is usually harder to determine substantial concepts when practical is shown rather than theoretical. I can give an example of theoretical substantial concepts. It's a video tutorial rather than a tutorial, but I hope you can understand what I mean.
As I said in the first place, thanks for your feedback. If you have any more questions on the topic, feel free to ask.
That's good to hear that you fine now, thanks for reviewing, You points or exceptions are perfectly alright and i am agree and will learn to reduce mistakes that i shouldn't want to make on next post. Your review or any mod review are always helpful to open source contribution society :)
I read what you wrote and wanted to inform you that I will reply when possible. (probably in a day) Thanks for your feedback on my moderation.
Congratulations @genievot! You have completed the following achievement on Steemit and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Hi @genievot!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @genievot!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!