How To Create A Post From Steem.js JavaScript Library (Steem.js #3)
How To Create A Post From Steem.js JavaScript Library
Repository
https://github.com/steemit/steem-js
What Will I Learn?
In this tutorial, I will teach you how to post to Steem blockchain by Steem.js.
- You will learn how to post from Steem.js
- You will learn how to create simple Steem front-end website.
At the end you will know how to create website like http://fbslo.net/tools/steem.html (GitHub code)
Requirements
You need:
Difficulty
- Basic
Tutorial Contents
Let's start with HTML skeleton. This part is standard HTML and this scrip in head will load Steem.js JavaScript library. It will load from file steem.min.js. I also added CDN, but it fail to load sometimes.
<head><title>Create Post</title>
<script src="./steem.min.js"></script>
<script src="//cdn.steemjs.com/lib/latest/steem.min.js"></script>
</head>
<body>
</body>
</html>
Into this HTML skeleton we need to add input fields for posting key, tags, author etc...
<input type="text" id="account" onkeyup="return forceLower(this);" placeholder="Account (e.g. fbslo)" />
<input type="text" id="key" placeholder="Private Posting Key" />
<input type="text" id="permlink" placeholder="Unique Permlink (e.g. my-post-title)" />
<input type="text" id="title" placeholder="Title" />
<textarea placeholder="Post Content (Markdown/HTML)" onkeyup="adjust_textarea(this)" id='article'></textarea>
<input type="text" id="tag1" placeholder="Tag 1 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag2" placeholder="Tag 2 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag3" placeholder="Tag 3 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag4" placeholder="Tag 4 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag5" placeholder="Tag 5 (Must start with a letter, only lowercase letters )" />
<center><input type="button" value="POST" onClick='postArticle()'/></center>
You can see that you will be able to add 5 tags, but this is not limit. You can just add more input fields in HTML (and Javascript) and you can use 100 tags if you want.
This part of code onkeyup="return forceLower(this);"
is new in my tutorials. It will force all input to be lower case letter. And this code onkeyup="adjust_textarea(this)"
will expand text area for body of post.
When we have HTML skeleton ready, we need to addJavaScript. There is not different function for comments and top posts.
steem.broadcast.comment
Now we need to add full function with all details:
document.getElementById('key').value, // posting wif from your wallet
'', // author, leave blank for new post
document.getElementById('tag1').value, // first tag / category
document.getElementById('account').value, // username
document.getElementById('permlink').value, // permlink
document.getElementById('title').value, // Title
document.getElementById('article').value, // Body of post
// json metadata (additional tags, app name)
{ tags: [document.getElementById('tag2').value, document.getElementById('tag3').value, document.getElementById('tag4').value, document.getElementById('tag5').value], app: 'utopian-test' },
function (err, result) {
if (err)
alert('Failure! ' + err);
else
alert('Success!');
}
);
All text after // are comments, so you can understand parts of the code. (document.getElementById('id').value
will get input from input fields in HTML.)
Now we need to add function. We already have button that will run postArticle() function (<input type="button" value="POST" onClick='postArticle()'/>
)
function postArticle() {
steem.broadcast.comment(
document.getElementById('key').value, // posting wif from your wallet
'', // author, leave blank for new post
document.getElementById('tag1').value, // first tag / category
document.getElementById('account').value, // username
document.getElementById('permlink').value, // permlink
document.getElementById('title').value, // Title
document.getElementById('article').value, // Body of post
// json metadata (additional tags, app name)
{ tags: [document.getElementById('tag2').value, document.getElementById('tag3').value, document.getElementById('tag4').value, document.getElementById('tag5').value], app: 'utopian-test' },
function (err, result) {
if (err)
alert('Failure! ' + err);
else
alert('Success!');
}
);
}
This is everything . Now we need to put all this code together (Javascript code must be in <script></script>
) and we must add CSS, because this is just raw HTML and webpage is not nice. CSS will make it beautiful.
<link href='http://fonts.googleapis.com/css?family=Open+Sans+Condensed:300' rel='stylesheet' type='text/css'>
<style type="text/css">
.form-style-8{
font-family: 'Open Sans Condensed', arial, sans;
width: 500px;
padding: 30px;
background: #FFFFFF;
margin: 50px auto;
box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
-moz-box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
-webkit-box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
}
.form-style-8 h2{
background: #4D4D4D;
text-transform: uppercase;
font-family: 'Open Sans Condensed', sans-serif;
color: #797979;
font-size: 18px;
font-weight: 100;
padding: 20px;
margin: -30px -30px 30px -30px;
}
.form-style-8 input[type="text"],
.form-style-8 textarea,
.form-style-8 select
{
box-sizing: border-box;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
outline: none;
display: block;
width: 100%;
padding: 7px;
border: none;
border-bottom: 1px solid #ddd;
background: transparent;
margin-bottom: 10px;
font: 16px Arial, Helvetica, sans-serif;
height: 45px;
}
.form-style-8 textarea{
resize:none;
overflow: hidden;
}
.form-style-8 input[type="button"],
.form-style-8 input[type="submit"]{
-moz-box-shadow: inset 0px 1px 0px 0px #45D6D6;
-webkit-box-shadow: inset 0px 1px 0px 0px #45D6D6;
box-shadow: inset 0px 1px 0px 0px #45D6D6;
background-color: #2CBBBB;
border: 1px solid #27A0A0;
display: inline-block;
cursor: pointer;
color: #FFFFFF;
font-family: 'Open Sans Condensed', sans-serif;
font-size: 14px;
padding: 8px 18px;
text-decoration: none;
text-transform: uppercase;
}
.form-style-8 input[type="button"]:hover,
.form-style-8 input[type="submit"]:hover {
background:linear-gradient(to bottom, #34CACA 5%, #30C9C9 100%);
background-color:#34CACA;
}
</style>
And now let's put all code together:
Full code:
<html>
<head><title>Create Post</title>
<script src="./steem.min.js"></script>
<script src="//cdn.steemjs.com/lib/latest/steem.min.js"></script>
<link href='http://fonts.googleapis.com/css?family=Open+Sans+Condensed:300' rel='stylesheet' type='text/css'>
<style type="text/css">
.form-style-8{
font-family: 'Open Sans Condensed', arial, sans;
width: 500px;
padding: 30px;
background: #FFFFFF;
margin: 50px auto;
box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
-moz-box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
-webkit-box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.22);
}
.form-style-8 h2{
background: #4D4D4D;
text-transform: uppercase;
font-family: 'Open Sans Condensed', sans-serif;
color: #797979;
font-size: 18px;
font-weight: 100;
padding: 20px;
margin: -30px -30px 30px -30px;
}
.form-style-8 input[type="text"],
.form-style-8 textarea,
.form-style-8 select
{
box-sizing: border-box;
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
outline: none;
display: block;
width: 100%;
padding: 7px;
border: none;
border-bottom: 1px solid #ddd;
background: transparent;
margin-bottom: 10px;
font: 16px Arial, Helvetica, sans-serif;
height: 45px;
}
.form-style-8 textarea{
resize:none;
overflow: hidden;
}
.form-style-8 input[type="button"],
.form-style-8 input[type="submit"]{
-moz-box-shadow: inset 0px 1px 0px 0px #45D6D6;
-webkit-box-shadow: inset 0px 1px 0px 0px #45D6D6;
box-shadow: inset 0px 1px 0px 0px #45D6D6;
background-color: #2CBBBB;
border: 1px solid #27A0A0;
display: inline-block;
cursor: pointer;
color: #FFFFFF;
font-family: 'Open Sans Condensed', sans-serif;
font-size: 14px;
padding: 8px 18px;
text-decoration: none;
text-transform: uppercase;
}
.form-style-8 input[type="button"]:hover,
.form-style-8 input[type="submit"]:hover {
background:linear-gradient(to bottom, #34CACA 5%, #30C9C9 100%);
background-color:#34CACA;
}
</style>
<script>
function postArticle() {
steem.broadcast.comment(
document.getElementById('key').value, // posting wif from your wallet
'', // author, leave blank for new post
document.getElementById('tag1').value, // first tag / category
document.getElementById('account').value, // username
document.getElementById('permlink').value, // permlink
document.getElementById('title').value, // Title
document.getElementById('article').value, // Body of post
// json metadata (additional tags, app name)
{ tags: [document.getElementById('tag2').value, document.getElementById('tag3').value, document.getElementById('tag4').value, document.getElementById('tag5').value], app: 'utopian-test/fbslo' },
function (err, result) {
if (err)
alert('Failure! ' + err);
else
alert('Success!');
}
);
}
</script>
</head>
<body>
<div class="form-style-8">
<h2><center>Create a Post on the STEEM Blockchain</center></h2>
<form>
<input type="text" id="account" onkeyup="return forceLower(this);" placeholder="Account (e.g. fbslo)" />
<input type="text" id="key" placeholder="Private Posting Key" />
<input type="text" id="permlink" placeholder="Unique Permlink (e.g. my-post-title)" />
<input type="text" id="title" placeholder="Title" />
<textarea placeholder="Post Content (Markdown/HTML)" onkeyup="adjust_textarea(this)" id='article'></textarea>
<input type="text" id="tag1" placeholder="Tag 1 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag2" placeholder="Tag 2 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag3" placeholder="Tag 3 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag4" placeholder="Tag 4 (Must start with a letter, only lowercase letters )" />
<input type="text" id="tag5" placeholder="Tag 5 (Must start with a letter, only lowercase letters )" />
<center><input type="button" value="POST" onClick='postArticle()'/></center>
</form></div>
</body>
</html>
And this is our website:
You can find this website on http://fbslo.net/tools/steem.html
If you have any questions, ask in comments. You can find full code on my GitHub
Curriculum
My previous Steem.js tutorials.
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @portugalcoin
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Woow thanks @fbslo for that post, actually you have reminded me of something we were taught during in my University course of java scrip and this was clear headache to everybody that many of my friends had retakes.
This is incredible that you are too good at this, i wish icould join your league in resuming such studies of Java, i believe it presents big open jobs out there because of its complexity.
Am just remembering the loops, commands, the characters and yy plus and C hash but atleast i can read something from you writing like the comments.
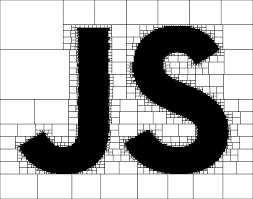
Hey @fbslo
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
thanks totorial him, this very mamfaat bgi us all.
Sorry, I don't understand.