Intermediate Tutorial - How To Calculate Area And Circumference of Circle Using Java Swing
What Will I Learn?
- You will learn about Java programming
- You will learn about Java Swing
- You will learn about GUI
- You will learn about Area and Circumference of a circle
Requirements
Difficulty
- Intermediate
Tutorial Contents
This time, we will learn about calculating the area and circumference of the circle using java swing feature (GUI).
To remember, Swing is a library in java that serves to create a GUI view.
After knowing what Swing is, you also need to know about the GUI. GUI is an interface display to help humans communicate with computers.
To continue this tutorial, we must also know the concept of circles, especially formulas to calculate the area and circumference. Here are the formulas of area and circumference of a circle.
So, to determine the result of the area and circumference of the circle, we need only enter the radius value and the value of phi into the formula. And the value of phi is 3.14 or 22/7, this is already a provision in mathematics. For this time I will use the value 3.14, but if you want to use the value 22/7 also no problem.
We go into the process to create the program. Please note the following steps:
The first step is to call Java Swing library. Here is the command.
import javax.swing.JOptionPane;
Next is the public class naming. The public class name should also be the same as the java file name you created. For example, I will use the name circle.
public class circle
Then, we will enter the command for the main method. Without main method, java programming will not work.
public static void main(String[] args)
{
Next, we will do a declaration for the variable. Variables are places to accommodate data. This time I will declare some variables like as:
- radius ==> Will be used to accommodate Input Dialog. So we can use the String data type, because the data we input is a word or sentence.
- value ==> Will be used to accommodate a radius value. So we can use the double data type, because the data we input is a number especially fraction number.
- area ==> Will be used to accommodate a circle area result. So we can use the double data type, because the result of the circle area can be a fraction number.
- circumference ==> Will be used to accommodate a circle circumference result. So we can use the double data type, because the result of the circle circumference can be a fraction number.
Its syntax is as follows.
String radius;
double value ;
double area;
double circumference;
Next is enter the command to input the radius value through Input Dialog, and save the value of the radius to the variable.
radius=JOptionPane.showInputDialog("Enter the radius value (in centimeter) : ");
value=Double.parseDouble(radius);
This time we will enter the formulas to determine the area of the circle and the circumference of the circle.
area=3.14 * value * value;
circumference=3.14 * 2 * value;
The last step is to enter a command to display Message Dialog. In this Message Dialog too, results from the area of the circle and the circumference of the circle will be displayed.
String result="- Area of a circle is "+area+" cm \n- Circumference of a circle is "+circumference+" cm";
JOptionPane.showMessageDialog(null,result);
}
}
Result
- Inputting the radius value
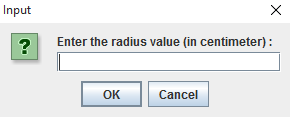
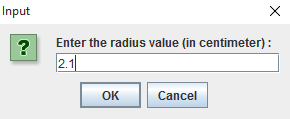
- Message Dialog view
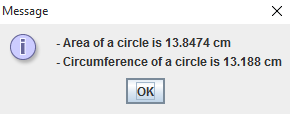
Here is the full syntax:
import javax.swing.JOptionPane;
public class circle
{
public static void main(String[] args)
{
String radius;
double value ;
double area;
double circumference;
radius=JOptionPane.showInputDialog("Enter the radius value (in centimeter) : ");
value=Double.parseDouble(radius);
area=3.14 * value * value;
circumference=3.14 * 2 * value;
String result="- Area of a circle is "+area+" cm \n- Circumference of a circle is "+circumference+" cm";
JOptionPane.showMessageDialog(null,result);
}
}
Posted on Utopian.io - Rewarding Open Source Contributors
I am in love with your tutorial 😊
Thanks bro, don't forget to always support me :v
Your contribution cannot be approved because it does not follow the Utopian Rules.
Violated Rules:
Tutorials must be technical instructions that teach non-trivial aspects of an Open Source project.
Same contributions will never be accepted in Utopian twice by the same or different user.
Design or video editing related tutorials, gameplay, simple on-screen instructions, ubiquitous functions (Save, Open, Print, etc.) or basic programming concepts (variables, operators, loops, etc.) will not be accepted.
My Opinion:
A tutorial must be informative and explanatory, but also "tutor". This tutorial does "tutor", but shows the same concept with your older posts in this curriculum but just with different variables. A user can easily read your older posts and alter it to match his/her needs.
Too trivial.
Also, it's called Pi, not Phi. :)
You can contact us on Discord.
[utopian-moderator]
Congratulations @elfida! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Congratulations @elfida! You received a personal award!
Click here to view your Board
Congratulations @elfida! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!