Blinkit v2.3: Arduino integration | Custom Boot Pattern
Our goals for Blinkit project are high and one of the goals is to have Blinkit in it's own case and make a stand alone version of Blinkit using Arduino boards, and for both of this goals it would be very usefull and nice to have always during intitial startup a light pattern, this would help the user knowing the status of the devivce by just looking at it, together with @techtek we looked into what would suit best and i started on this new feutures because it will be a great adition to the Blinkit project.
Smooth light effects for a more friendly experience
After several testing of our last release of the RGB led mode in the Arduino integration into Blinkit project we have pointed out that a boot lighting pattern would be usefull from many points of view (technically, aesthetically, etc...) thus will give an added value to the whole integration of Arduino. In fact, hereafter we will be talking about this new added feature in terms of mathematical model, coding and advantages/benefits.
What is Blinkit?
Blinkit is a notification software that can be used to give to regular and widely available devices a Steem purpose.
Supported devices:
- USB Sticks (status light)
- Philips HUE lamps
- Sonoff devices
- Arduino Boards or compatible (Genuino, etc...)
- Camera status LED blink
- Take photos on upvote/post/follow
- Logitech RGB/Backlight Keyboards (new)
Blinkit can look for new Steem account Upvotes and Followers, and it can notify on new Posts made by a user.
More devices will be added in the near future.
Blinkit is free and open source, and can be downloaded from the Blinkit Github page:
https://github.com/techtek/Blinkit
For more information about the new version of Blinkit please visit the following link
COMPATIBILITY
Current release has been tested only on an Arduino UNO board. Other boards like Mega and Nano should be supported (for Nano different PWM pins should be selected, for Mega nothing to change) but have not been tested yet. Users interested in using this new feature with other boards could submit a test contribution and let us know the result of their test.
New Coded Features:
- Light pattern during Arduino booting or after button reset
After several testing with our last release of the new RGB led mode a little concern had arisen:
- How to make sure that connections are well established ?
- How to make sure that wiring had been made correctly ?
- How to give some liveliness to the Blinkit related Arduino boards notifiying users that the board is ready to accept new incoming triggers ??
After some brainstorming with @techtek we decided to do a custom made booting light pattern for Blinkit which will fade the RGB led between the main colours of Blinkit's style: Violet and White.
In this way we would be solving the 3 concerns mentioned above. In fact, only if connections are well established and wiring is correctly done the led fading will be correctly visible.
If single leds are to be used instead of an RGB one, the colours of the fading pattern will obviously not be seen but it will be a good way to test if connections are well established and wiring is correctly done as all the leds should light up and fade a little back and forth thus notifying the user that they are well/correctly connected hence ready to get triggered.
Hereafter i will explain the whole process behind the coding which is related to the creation of a mathematical model to be used in the code and will explain how the code works to let it work as desired.
Study and Creation of the mathematical model/function
As per their conceived use, PWM pins (Pulse-Width Modulation) are used to dim leds by varying the duty cycle of a constant current in the string to effectively change the average current in the string.
Using this particular feature we are able to dim any RGB led and let it light up on a specific RGB colour. The values that could be assigned go from 0 (fully off, brightness = 0) to 255 (fully on, brightness= maximum).
Assuming that we want to fade a led from colour A(r,g,b) to colour B(R,G,B) what we could think about is using a linear function. This can be done but will not be a smooth fading since humans percieve brightness logarithmicly and not linearly.
The best and most practical way to fade a led using PWM is to implement a logarithmic or exponential function that handles the transition from the colour A to the colour B (not couting white colour as a colour as it could be handled linearly on RGB leds for many reasons)
In the case of Blinkit what we wanted to achieve is fading from Violet to White and vice versa (the colours of Blinkit interface).
Below is how the mathematical model has been created (i will not go very deep in the study but will highlight the main aspects that we used in our code):
Assuming the RGB colour space as below (a cube with origin black colour [0,0,0] and biggest value [255,255,255] equal to white colour with 3 axis [R,G,B] as shown below ),
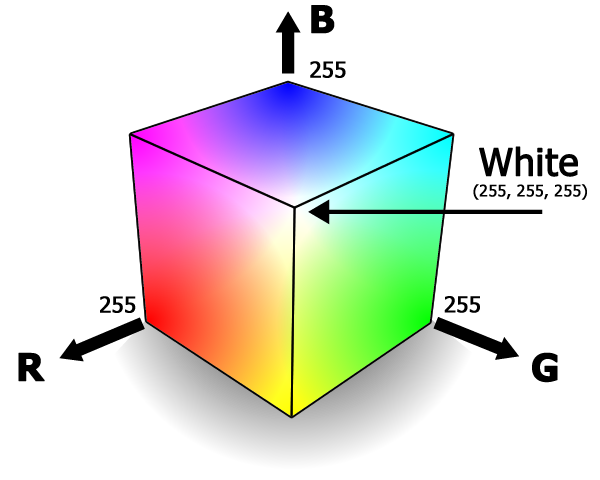
Our target colour for Blinkit Violet is (R=110 G=0 B=255) which is located on the top face of the cube where Blue Intensity [B] is equal to 255.
Remaining variables R and G will be then the case of the study thus the base of our logarithmic function.
The starting colour we wanted to begin the fading with is, reiterate, the Violet with R=110 G=0 B255 which will have to change smoothly to a much brighter Violet located on R=255 G=10 B=255. For that reason we implemented the logarithmic function to determine G value based on R using the general form "G= A * ln('R')+H" and by doing the proper calculations and proper aproximation we will find values for "A" and "H", ending up with "G=11.8937*ln("R")-55.9050".
Afterwards we implemented a linear function with starting point R=255 G=10 B=255 to move alongside the cube top side to transit from that colour to the white colour(since we are working in the white spectrum a linear function will not be noticed by human eyes in that field). For that last part a simple FOR loop in the code with adeguate step will do the job.
How is it implemented?
The whole code structure has not been modified and thus is still devided in 6 parts:
- Declare/set the variables, the arrays etc
- Initializing the setups (Serial Comunication and Output pins) + booting light pattern
- Receive serial data to be processed
- Receive serial data and split it in tokens declaring the functions that will handle such data
- Read splitted data and based on some marker store them in the right setting array
- Wait for triggers (upvote, follower, post) over serial and activate the led
However in the setup loop the new feature has been added so that the whole code runs just once during the boot phase of Arduino.
Initializing the setups (Serial Comunication and Output pins)+ Boot light fading
void setup()
{
Serial.begin(9600);
Serial.flush();
pinMode(RedPIN, OUTPUT); //set the selected pins as output
pinMode(GreenPIN, OUTPUT);
pinMode(BluePIN, OUTPUT);
int redIntensity = 0; //variable to handle the brightness of the red colour of the RGB led
int blueIntensity = 0;// variable to handle the brightness of the blue colour of the RGB led
int greenIntensity = 0; // variable to handle the brightness of the green colour of the RGB led
while (Serial.available() == NULL){ // repeat the below pattern while no data is received over serial
for (int i = 0; i <=240; i+=1){ //This loop will light up/dim linearly the led till it goes from off state to a value close to
//R=110 G=0 B=255 the starting value for our fading pattern
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity = i/2;
blueIntensity = i ;
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10);
}
for (int t = 0; t<2; t++){ //this FOR loop will let use repeat the below (whole) fade-in, fade-out pattern twice
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
for (int i = 110; i <=255; i+=1){ //In this for loop we will handle the first transition from R=110 G=0 B=255 (darker Violet) to
//R=255 G=10 B=255 (much brighter Violet) and have chosen to use the redIntensity as the variable part of the FOR loop
//based on the value of R = i we will calculate the Green brightness.
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity = i;
greenIntensity = ((118937/10000)*log(i)-(559050/10000)); //The neperien logarithmic function usually wrote as ln(x)
//is not recognized in Arduino coding by using ln but log
if(greenIntensity> 10){ //based on the aproximation in calculations we do this just in case not to pass the values
greenIntensity =10;
}
if(greenIntensity< 1){ //based on the aproximation in the calculations we do this so that value of Green
greenIntensity =0; //does not turn negative in anyway thus creating an error in the display
}
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10); //
}
for (int i = 10; i <=240; i+=1){ //using this For loop we handle the linear transition between the bright Violet (R=255 G=10 B=255)
//and the White colour (R=255 G=255 b=255)
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity=255;
blueIntensity=255;
greenIntensity=i;
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10); //
}
//below we reverse the loops used before to fade from Violet to White and use them to do the other way (from White to Violet)
for (int i = 255; i >=10; i-=1){ //using this For loop we handle the linear transition between the White colour (R=255 G=255 b=255)
//and the bright Violet (R=255 G=10 B=255)
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity=255;
blueIntensity=255;
greenIntensity=i;
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10); //
}
for (int i = 255; i >=110; i-=1){ //In this for loop we will handle the transition from R=255 G=10 B=255 (much brighter Violet) to
// R=110 G=0 B=255 (darker Violet) and as before have chosen to use the redIntensity as the variable part of the FOR loop
//based on the value of R = i we will calculate the Green brightness.
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity = i;
greenIntensity = ((118937/10000)*log(i)-(559050/10000));
if(greenIntensity<1){ //based ont he aproximations this will keep us on the safe side.
greenIntensity =0;
}
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10); //
}
}
for (int i = 255; i >=0; i-=1){ //This loop will dim linearly the led till it goes totally of
//since we went back to R=110 G=0 B=255 with a quick calculation and a good aproximation
//we set the step to take for each brightness decrease so that they get dimmed simultaneously and of the same ammount each time
if (Serial.available() > 0){ // turn off the led when data is received and exit the loop
analogWrite(RedPIN, 0); //
analogWrite(GreenPIN, 0); //
analogWrite(BluePIN, 0); //
break;
}
redIntensity = i/3;
blueIntensity = i ;
analogWrite(RedPIN, redIntensity); //
analogWrite(GreenPIN, greenIntensity); //activate with proper delay the led using the currently stored values for R G B
analogWrite(BluePIN, blueIntensity); //
delay(10);
}
}
}
After having initiated the serial comunication and after having defined the pins as output, Arduino will enter the fading effect phase. This effect will continuously loop thanks to the WHILE loop statement until the first settings are sent to the Arduino board through Blinkit. As soon as data are received the pattern will not be reproduced anymore unless the Serial comunication is opened without sending data to the board (ex. open console and no data being sent). As seen in the commented parts of the code, we can see that this light effect, occurring after the WHILE loop, is devided in 5 main parts in the following order:
- Initial FOR loop to do the fading from fully off to R=110 G=0 B=255 which will be the initial colour from which the fading to white will start
- a FOR loop to repeat the whole fading pattern several times
- 2 consecutive FOR loops to do the fading from Violet to White as advised above in the study of the mathematical model
- 2 consecutive FOR loops to do the reverse fading effect (from White to Violet)
- 1 final FOR loop to slowly turn off/slowly dim the led from Violet to fully off
The new Arduino sketch file (.INO) can be downloaded from my Github repo Blinkit-Arduino-integration
The Wiring Diagram
Below i would like to remind the wiring that needs to be done as this is one of the main reasons why we have implemented the start-up light effect (obviously also for easthetical reasons). The below wiring diagrams show how wiring should be done based on the code(in this case the output pins chosen are PIN 3, PIN 5 and PIN 6). Since we are going to also handle RGB leds, pins must be chosen as PWM pins in order to be able to get all the shades and colours of the RGB spectrum. If normal (non PWM pins) are used this could not be possible. Another aspect to take into consideration is that RGB led must be a common cathode (common ground [-]).
int RedPIN = 3; //pin for led 1 (single colour led) or pin for the Red led in the RGB led
int GreenPIN = 5; //pin for led 2 (single colour led) or pin for the Green led in the RGB led
int BluePIN = 6 ; //pin for led 3 (single colour led) or pin for the Blue led in the RGB led
[some other variables are in the code]
void setup()
{
Serial.begin(9600);
Serial.flush();
pinMode(RedPIN, OUTPUT); //set the selected pins as output
pinMode(GreenPIN, OUTPUT);
pinMode(BluePIN, OUTPUT);
[...]
}
Materials needed:
- 3x Single Colour LED (Any colour) or 1 RGB led (Must be a Common Cathode pinout)
- 3x Resistor 150 Ohm
- Jumper wires
The following files are added/updated to the repository
As suggested by the mod in my last contribution i did more evident the kind of commits i did and had used better commenting to make it understandeable.
- ArduinoBlinkitSketch.ino (new file with start-up light effect)
- Readme ( as suggested by the mod. ,updated the version by adding the "how to upload a sketch to Arduino boards using Arduino IDE")
Many updates have been planned concerning Arduino integration. In fact our aim is to let Arduino reach a point where it can become fully automated and do not require to be directly attached to a pc running Blinkit software but will run itself the same tasks as Blinkit software by its own thus becoming a portable stand alone device for Steem related notifications.
Technical Support
Technical support is available, if you may encounter a problem, or if you want to know if your device is supported or will be supported in the near future.
How to contribute?
Do you have a question, or suggestion about the integration of Arduino in Blinkit ? Feel free to contact me on Discord (eliaraysalem#0829) or leave a comment below.
Thank you for your contribution. A great post with a lot of explanation.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you @codingdefined for your moderation !! I am pleased to hear that you retain that my post and code are well explained !! Unfortunately the same cannot be said while reading the answers given to the questions. I am referring to "How do you rate the quality of the comments in the code?
Average - most of the code is commented and most if it adds to the readability of the code." eventhough every single line of the code has a comment explaining what is it for and what does it do. Just wanted to point out that point without any controversy of any sort !! Anyway, thank you again for your moderation !! much appreciated !!
It's a difficult metric and quite personal. My contributions never score well on comments because I hardly add any. It's a very debated topic and personally I'm a comment minimalist. I like to use the WTF metric:
That is a fantastic metric indeed!! What i was highlighting with my comment is that i wanted coherence between the comment made and the answer given to the questions answered on utopian format.
Join our Discord Channel to connect with us and nominate your own or somebody else's posts in our review channel.
Help us to reward you for making it ! Join our voting trail or delegate steem power to the community account.
Your post is also presented on the community website www.steemmakers.com where you can find other selected content.
If you like our work, please consider upvoting this comment to support the growth of our community. Thank you.
Thank you @steemmakers !! Much appreciated!!
Hey @electronicsworld
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Congratulations @electronicsworld! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your Board of Honor.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last announcement from @steemitboard!
نسيت استعمال تاغ arab هذه المرة :)
حصلت على تصويت من
@arabsteem curation trail !
يمكنك الحصول على تصويت اضافي عبر ارسال مبلغ اقله
0.05
ستيم او اسبيدي الى حساب التصويت الالي
@arabpromo
مع رابط المقال في حقل المذكرة (memo)
مما يتيح لك الحصول على تصويت مربح بحوالي 2.5 اضعاف :)
thank you @arabsteem !! much appreciated !!
Hello, electronicsworld, I'm @ArtTurtle, an upvote bot run by @Artopium. I just upvoted this post and resteemed it because I'm following you and, well, that's just what I do. Reciprocation is always appreciated! If you no longer wish to have @ArtTurtle follow you please reply to this comment with 'STOP'.
Thank you @artturtle !! much appreciated !!