How To Get The Payment Rewards Of A Steemit User, In Less Than 50 Lines
PHP is still a widely used programming language and its versatility makes it perfect for server-side coding. That's why I decided to write a set of tools interacting with the Steem blockchain in PHP, and today I'm going to walk you through a very short tutorial in which you'll learn the following:
- use the
php-steem-tools
package via composer - instantiate one of the classes in your project
- calculate the total payout for a certain user
This tutorial requires moderate knowledge of php and composer.
Without further ado, let's start.
Step 1: Add php-steem-tools
in composer.php
Install composer (this is out of the scope of this tutorial, but if you need a good start, the Composer website has a very good documentation). Open composer.php
and add the following lines:
{
"minimum-stability" : "dev",
"require": {
"dragos-roua/php-steem-tools": "v0.0.4-alpha"
}
}
The current version of the package is 0.0.4-alpha, and you need the "minimum-stability" setting to be at the "dev" level.
Step 2: Require the package and instantiate the Steem API
Create a file (in the same folder as composer.php
) and require the package:
require __DIR__ . '/vendor/autoload.php';
Now you'll have access to all the classes you included in the composer.php
file. Let's instantiate the API. Add the following lines:
$config['webservice_url'] = 'steemd.minnowsupportproject.org';
$api = new DragosRoua\PHPSteemTools\SteemApi($config);
Now, the $api
variable will contain a new API object which will query the data from the webservice_url
address. If you want to query a different node, just change this address.
Step 3: Pick an author and make a call to the API
Ok, we have an object, but we didn't make any call. Let's pick an author, in our case utopian-id
and then prepare the API call:
$author = "utopian-io";
date_default_timezone_set("UTC");
$dateNow = (new \DateTime())->format("Y-m-d\TH:i:s");
$date_8days_ago = date('Y-m-d\TH:i:s', strtotime("-8 days", strtotime($dateNow)));
$params = [$author, "", $date_8days_ago, 100];
$remote_content = $api->getDiscussionsByAuthorBeforeDate($params, "websocket");
Basically, we set up an array of 4 objects, containing the $author
, the date from which we start, which is 8 days ago, and the number of posts to search that we start with, in this case 100. If there are less than 100 posts, the API call will return just the posts that are there, if there are more, than it will initiate another call until it grabs all the posts.
With that array set up, we call the function getDiscussionsByAuthorBeforeDate($params, 'websocket')
. The second parameter is the transport layer used, in this case websocket
. You can also use curl
if you want, I find websocket
to be faster.
Step 4: Iterate through posts and build the total
Now that we made the call, all we need to do is to browse through the returned posts and build the total:
if(isset($remote_content)){
if (array_key_exists('error', $remote_content)){
echo "Something is not ok. You should investigate more.\n";
}
else
{
$total_value = 0;
foreach($remote_content as $rkey => $rvalue){
if($rvalue['pending_payout_value'] !== '0.000 SBD' && $rvalue['max_accepted_payout'] != 0){
$tmp = explode(" ", $rvalue['pending_payout_value']);
$payout = $tmp[0];
// check to see if we have something in the beneficaries array
if(count($rvalue['beneficiaries'] != 0)){
foreach($rvalue['beneficiaries'] as $kk => $vv){
if($vv['account'] == $author){
$payout = $payout*($vv['weight']/10000);
}
}
}
$author_payout = $payout * 0.75;
echo "Author payout: ".$author_payout.", Total post payout: ".$payout."\n";
$total_value += $author_payout;
}
}
echo "Total payout for $author: ".$total_value." (SBD + STEEM)\n";
}
}
The error checking is really minimal in this example, but in real life you would want to take into account all the possible problems that may appear. The error that we're treating here is just a malfunction of the blockchain, but we may also catch a non-existent user, for instance (this one would make a good homework).
Then we just iterate over the array of posts and look into the payout
field. If there is something to be paid and if the author didn't decline payout all together, we start building a total. As a precaution, we also look into the beneficiaries
array and see if there's anything there.
The result of the command php authorPayments.php
:
Ta-daa! In less than 50 lines you got the payments of a Steemit user. Of course, there are many other improvements that can be made: add the comments payout, calculate the SBD / STEEM ratio, error checking and so on.
If you want to get the source code form this tutorial, check out the folder "examples" from the php-steem-tools Github repository.
I'm a serial entrepreneur, blogger and ultrarunner. You can find me mainly on my blog at Dragos Roua where I write about productivity, business, relationships and running. Here on Steemit you may stay updated by following me @dragosroua.
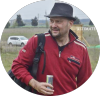
https://steemit.com/~witnesses
If you're new to Steemit, you may find these articles relevant (that's also part of my witness activity to support new members of the platform):
- Rewards: Were Does The Money Come From?
- Netiquette: How To Avoid Being A Steem Douche?
- Witnesses: What Are They And Why Should You Care?
- How Can I Mine Steem And What CPU / GPU Power Do I Need For That?
- The Beginner Guide To Not Getting Hacked On Steemit
Posted on Utopian.io - Rewarding Open Source Contributors
Holy Shit!!! I'm learning so much, i'm becoming a steem nerd with you man!
This post is not for me but my supports goes for you as you're continuously doing a fantastic job.
keep it up dear @dragosroua.
Steem On!
I really love these kind of tutorials. Thank you for sharing the wisdom with us :)
my pleasure, hope it helps :)
It sure does, can't wait to get coding on steem! But my weapon of choice is python ;)
how i see programming lol
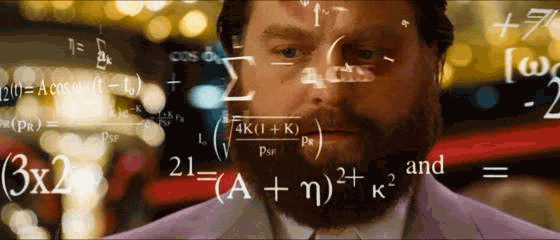
thanks alot sir..your tutorials teach us alot..it's a great support for beginners like us :)
Thanks for this clean example. I might use it someday in the near future.
For now, I am playing with plain and pure javascript. I've already managed to build a sort of aggregator web page with posts from all or one user. Some might call this a condenser :)
Next year will be full with steem programming :)
Thanks again!
My pleasure, mate!
I find the blockchain development to be a completely different beast from all I learned so far. I tend towards a development stack with JS on the client side and PHP / Python on the server side.
JS greatly fits the privacy requirements, because the private keys never leave the browser. But on the server side PHP and Python are still rocking, IMHO.
Looking forward to see what you'll build :)
You are right. A proper mix of frontend magic with backend processing might do the trick. I'll let you know, of course.
Here is my first try: https://steemit.com/utopian-io/@ervin-lemark/steemdata-webapi-example-1-posts-metadata-fetching-and-display
Done with SteemData API. Explanation follows in a form of tutorial :)
Working example: http://steemgnome.com/steemdata_example_aggregator_01.html?page=1
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thank you, it's been a pleasure :)
nice and very informative post as usual keep sharing @dragosroua
it's difficult to me :(
I like your tutorial. Thanks for sharing it