Steem Delegations Tutorial Part Three
Delegating your Steem Power to another user can be a great way to invest in Steem and Steemit. You can get an excellent annual percentage rate (APR) on your investment if you delegate your Steem Power to another user.
This post is part three of a tutorial series about Steem delegations. This post is the last part of the series. If you want to read the first two tutorials, you can do so by following the links in the curriculum.
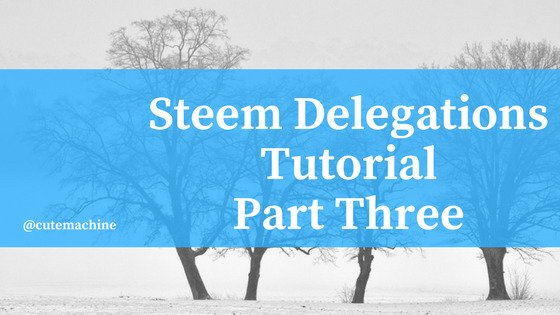
Curriculum
Part One
You will learn how to …
- get the current delegations for a user from the Steem blockchain
- convert VESTS to Steem Power (SP)
- show the information in a simple web application
Part Two
You will learn how to …
- get the transaction history for an account
- how to filter relevant data from the historical transaction data
- calculate the annual percentage rate for your investments
- show the information in a simple web application
Part Three (this article)
You will learn how to …
- make a simple form for new delegations
- delegate your Steem Power to another Steem user
- to remove your delegation
- to increase or decrease your delegation
Technologies Used
- SteemJS—Steem.js the official JavaScript library for the Steem blockchain
- Next generation JavaScript
- Hyperapp—1 KB JavaScript library for building frontend applications
- Hyperapp Router—Declarative routing for Hyperapp using the History API
- Bulma—free and open source CSS framework based on Flexbox
- Fontawesome—the web’s most popular icon set
- Parcel—Blazing fast, zero configuration web application bundler
Steem Delegation Tutorial Three
In the second part of this tutorial series, we looked at how we can build a delegation history. The delegation history lists all delegations and the transfers we received from the delegatees.
But how can we make a delegation from our account to another in the first place? This question will be answered in this article.
First I will show you how easy it is to make a delegation and then we will add a form to our existing application to collect the necessary data, like the delegator, the delegatee, and the amount of Steem Power we want to delegate.
You will also learn how to convert Steem Power to Vests because all delegations are done in Vests and not in SP.
How To Delegate Steem Power
When I started this tutorial series I thought that the most difficult part would be the actual delegation from one account to another. Well, it turns out that the delegation is achieved quite easily when you utilize steemconnect.com.
The only thing you need to have to make a delegation programmatically is the right URL.
Let me give you an improbable example: Jerry Banfield is delegating 100 SP to the postpromoter bot. This screenshot shows what Jerry will see in his browser:
And the URL in the browser would be similar to this one:
https://steemconnect.com/sign/delegate-vesting-shares?delegator=jerrybanfield&delegatee=postpromoter&vesting_shares=204201.531923%20VESTS
You see that the URL has all the information which is necessary to conduct a delegation: the delegator, the delegatee, and the amount.
As you can see the amount in the URL is not given in SP but VESTS. So there is only one question left for us to be able to build such an URL. How do we convert the 100 SP to the 204201.531923 VESTS?
Well, easy. I give you a function :)
// /src/common/utils.js
// Convert Steem to Vests
export const steem2Vests = (steem, dynamicGlobalProperties) => {
const { total_vesting_fund_steem, total_vesting_shares } = dynamicGlobalProperties
const totalVestingFundSteemNumber = unitString2Number(total_vesting_fund_steem)
const totalVestingSharesNumber = unitString2Number(total_vesting_shares)
const vests = steem / (totalVestingFundSteemNumber / totalVestingSharesNumber)
return vests.toFixed(6)
}
This function is very similar to the utility function vests2Steem
we used before in this tutorial series.
Building The Delegation Form
Now that we know how to make a delegation in our program and how we prepare the data to build the URL for the correct call to steemconnect, there is only one thing left we need to do. We need to have a form where the user can enter all the data and then submit the form to initiate the delegation.
For this, we will build a new view which we call the DelegationView
. We will put the delegation view which shows the delegation form on its own page. The form will look like this:
The delegation form needs a new piece of the global app state. Look at the delegationForm slice. The form will save its data there.
// src/state/index.js
export default {
error: {
message: ''
},
delegationForm: {
delegator: '',
delegatee: '',
amount: ''
},
location: location.state,
isNavbarMenuActive: false,
username: '',
delegations: [],
dynamicGlobalProperties: null,
accountHistory: [],
delegationHistory: []
}
And here are the actions that will change the state object.
// src/actions/index.js
…
delegationForm: {
changeDelegator: value => ({ delegator: value }),
changeDelegatee: value => ({ delegatee: value }),
changeAmount: value => ({ amount: value })
},
…
The code for the delegation form is self-explanatory. You can see the actions wired up to the input fields. Then there is the delegateSteemPower
function which will be called when the user clicks the delegate button.
The delegateSteemPower
function takes the delegator, delegatee, and amount from the state and constructs the URL. The URL will then be called with window.open
.
// src/views/DelegationView.js
import {h} from 'hyperapp'
import config from '../common/config'
import { unitString2Number, vests2Steem, steem2Vests } from '../common/utils'
export default ({state, actions}) => {
const delegateSteemPower = () => {
const delegationURL = `https://steemconnect.com/sign/delegate-vesting-shares?delegator=${state.delegationForm.delegator}&delegatee=${state.delegationForm.delegatee}&vesting_shares=${steem2Vests(state.delegationForm.amount, state.dynamicGlobalProperties)} VESTS`
window.open(delegationURL)
}
if (!state.dynamicGlobalProperties) {
actions.requestDynamicGlobalProperties()
return (
<div class='container'>
<div class='column is-half is-offset-one-quarter'>
<h1>Loading …</h1>
</div>
</div>
)
}
return (
<div class='container'>
<div class='column is-half is-offset-one-quarter'>
<section class='section'>
<div class="field is-horizontal">
<div class="field-label is-normal">
<label class="label">Delegator</label>
</div>
<div class="field-body">
<div class="field is-expanded">
<div class="field has-addons">
<p class="control">
<a class="button is-static">
@
</a>
</p>
<p class="control is-expanded">
<input
onkeyup={event => actions.delegationForm.changeDelegator(event.target.value)}
class="input" type="text" placeholder="Your Steemit username"
/>
</p>
</div>
<p class="help">The account you want to delegate SP from. Normally you enter your Steemit username here.</p>
</div>
</div>
</div>
<div class="field is-horizontal">
<div class="field-label is-normal">
<label class="label">Delegatee</label>
</div>
<div class="field-body">
<div class="field is-expanded">
<div class="field has-addons">
<p class="control">
<a class="button is-static">
@
</a>
</p>
<p class="control is-expanded">
<input
onkeyup={event => actions.delegationForm.changeDelegatee(event.target.value)}
class="input" type="tel" placeholder="Steemit username"
/>
</p>
</div>
<p class="help">The account you want to delegate SP to.</p>
</div>
</div>
</div>
<div class="field is-horizontal">
<div class="field-label is-normal">
<label class="label">Amount</label>
</div>
<div class="field-body">
<div class="field is-expanded">
<div class="field has-addons">
<p class="control">
<a class="button is-static">
SP
</a>
</p>
<p class="control is-expanded">
<input
onkeyup={event => actions.delegationForm.changeAmount(event.target.value)}
class="input" type="tel" placeholder="Amount in Steem Power"
/>
</p>
</div>
<p class="help">The amount of SP you want to delegate.</p>
</div>
</div>
</div>
<div class="field is-horizontal">
<div class="field-label">
</div>
<div class="field-body">
<div class="field">
<div class="control">
<button class='button is-primary' onclick={delegateSteemPower}>
Delegate
</button>
</div>
</div>
</div>
</div>
</section>
</div>
</div>
)
}
Room For Improvement
There is no error handling in the sample code. Validation of the various form fields is also missing.
It would also be nice when the user would be redirected to our application after having done the delegation on steemconnect.
If you like, you can make a contribution the above issues on GitHub. I will be happy to merge these in.
Conclusion
If you want to follow along you can check out the source codes by using the Git tags I added to the repository.
List tags with git tag
.
Checkout part one with git checkout part1
.
Checkout part two with git checkout part2
.
Checkout part three with git checkout part3
.
Click this link to see the application we built in this series.
This article concludes the Steem Delegation tutorial series. I hoped you learned something new.
Stay awesome,
Jo
Posted on Utopian.io - Rewarding Open Source Contributors
Do any still pay 1.5 Sbd on 1000?
Daily, weekly? 1.5 SBD on 1000 SP daily would be excellent. Do you know any?
I know delegates who are paying over 30% APR; which is still impressive.
Good attention to details. This review touches on an important issue and explains it in a way that people will understand.
Thanks for your kind words. I'm glad you like the tutorial series.
Change it !
You can contact us on Discord.
[utopian-moderator]
Reviewed and accepted in 5 hours!? Someone get this guy a cigar.
Thanks for the quick review and your motivational words to make more contributions.
Here is the cigar @r351574nc3 was talking about: 🚬
Decision has been modified. Please check my comment below.
Thanks sir
I've enjoyed seeing these tutorials. I haven't got enough SP for myself never mind delegating them. Hopefully one day when I build it up a bit :)
Hey Dan, thanks for your kind words. It is good to have real people comment on my work. Normally only bots are showing by :)
Just peeked at your blog, nice work. I'm sure that you will increase your SP as long as you persevere here on Steemit.
Have you written about EOS as well?
Where can I find part 2?
Hello @cicca, there is a link to part one and part two in the curriculum. But here is the link for your convenience :)
Ahhh now i understand 100% you just Skript Kid
try be must big but you = LOW LEVEL INTELECT PERSONE = THIS WHY YOU PUT YOUR FACE MY LIFE
who are tyou? if you come provocation me?
Why reason
you put your face my life
i hope you freal my pain
i never tuch you i not know you you come provocation me? why you msg your abrakadabra my page? who are you?
Yes, I feel your pain. Love, peace, and happiness to you.
Hy I see your tutorial post it's very long and very interested.i enjoye your information thanks for sharing
Thanks a lot.
Thank you for your contribution, yet it cannot be approved because it does not follow the Utopian Rules.
While I liked what you are trying to teach and accomplish here, yet few things led to the rejection, as follows:
Also note that steemconnect actually accepts SP instead of vests, under the same param, so no need for the conversion function and you can send 100 SP as the value the param and it would be accepted.
You can contact us on Discord.
[utopian-moderator]
Hmm, the contribution has been approved six hours ago by @cha0s0000. So you are saying that you are revoking the approval? I will contact you on Discord.
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by mslifesteem from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
You got a 4.53% upvote from @postpromoter courtesy of @cutemachine!
Want to promote your posts too? Check out the Steem Bot Tracker website for more info. If you would like to support the development of @postpromoter and the bot tracker please vote for @yabapmatt for witness!