Code::Blocks for Beginners | Identifiers, Operators and I/O statements
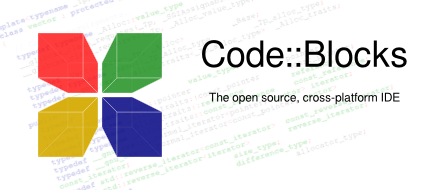
What Will I Learn?
In this tutorial, i will discuss on basic identifiers, operators and I/O statements in C program. So, by the end of this tutorial, you will be able to:
- learn how to assign a correct and valid identifiers for a user data in your C program;
- use valid data types and their declaration in your C program;
- learn how to use assignment statements in your C program;
- learn how to properly use printf and scanf function placeholder; and,
- apply all this basic syntax into a simple C program t
Requirements
To be able to follow this tutorial, you should have a desktop PC or laptop installed with a Windows (7, 8, 10) operating system. You also need to install the following applications:
Software | Download Here |
---|---|
Code:: Blocks Open Source Cross-platform IDE | codeblocks.org |
MinGW compiler | mingw.org |
Difficulty
Basic
Tutorial Content
Topic 1: Assigning Valid Identifier
Variables and constant names are important so that data objects can be accessed and identified properly in a computer program. Naming all the data objects is done by the use of an identifiers.
Valid Identifiers | Invalid Identifier |
---|---|
address_1 , your_age, members_name, p1 | 3My_Address, Student-10, printf |
As a general rule, valid identifier is composed of :
- meaningful names that starts with an alphabet;
- lower case characters;
- numbers may be used with in the variable name e.g. address_1; and,
- underscore ( _ ) can be used to come up with meaningful names.
Invalid identifier uses:
- number at the beginning of an identifier;
- mathematical operations (e.g. - , + , / , and * ); and,
- reserve and library identifiers such as printf and scanf.
Topic 2: Variable Data Types and Their Declaration in C programs
Aside from constant whose values does not change in the program execution, there are data whose values changes in the program execution. This are called variable data.
Declaration of identifiers | Data definition |
---|---|
char char_1, char_2, char_3; | enter three character name |
int year; | entered age in a year |
int age_in_days | converted age in days |
To declare an variable data identifier as shown from the preceding table, the basic C program syntax is "data type" (space) "identifier". For example, I want to get the age of the user; and, identify the age using the data identifier user_age. So, i will type in in the code::blocks :
int user_age;
The int
signifies that for user_age contains integer. Some of the commonly used data types syntax are presented in the table below.
Type | Description | Sample Values |
---|---|---|
char | character type data | "A", "b", "!" |
int | integer type(whole number) data | "300", "20", "-1" |
float | float precision data | "3.14.16", ".12e+6" |
Topic 3: Assignment Statements in C programs
The assignment "=" operator or the assignment statements is used to store values into a variable name.
Syntax display for Assignment Operators
Syntax | Example |
---|---|
result = expression | sum = a + b; |
For example, you will convert age (in days) to age(in years). The assignment statement is
age_in_days=year*days_in_a_year;
which assign the value of the computation into a variable name age_in_days.
Some of the arithmetic operators
Arithmetic Operator | Meaning |
---|---|
+ | addition |
- | subtraction |
* | multiplication |
/ | division |
Topic 4: Using scanf and print f function
scanf and print if are input and ouput functions that came from <stdio.h> library file. From their name scanf inputs data from the keyboard and **printf ** outputs data on the computer screen.
**Syntax display for scanf ** |
---|
scanf(format, input list) |
scanf("%f",&user_age);
It makes the computer ask a floating point number and stores it inside the variable user_age.
scanf Placeholder | Meaning |
---|---|
%f | float data type |
%d | integer data type |
%c | single character |
%c%c%c | multiple character |
Example: scanf("%f%c",&user_age,&user_name);
**Syntax display for printf ** |
---|
printf(format, print list) |
Example: printf("Hi %c your age is %d,&user_name,&user_age
This is to display the input data using the scanf function discuss earlier.
Topic 5: Application of discuss topics to Code::Blocks
Now, we will create a simple program asking the user's name and age; and display the age in days.
1| Open Code:: Blocks from your desktop or from your windows menu. Click NEW PROJECT > Console application to start a new project.
2 | Go to Project in the right pane and select main.c
3 | The main.c in the code::block has an existing C program structure that includes declaration of <stdio.h> and <stdlib.h>.
4 | Now type in first the declaration of directives and constant variable to be used in this example C program that will convert age in years to days.
#include <stdio.h>
#include <stdlib.h>
#define days_in_years 365
int main()
{
char user_name1,user_name2,user_name3;
float user_age;
float user_age_days;
printf("May I know your 3 letter nickname:");
scanf("%c%c%c", &user_name1,&user_name2,&user_name3);
printf("How old are you:");
scanf("%f",&user_age);
user_age_days=(user_age*days_in_years);
printf("Hi %c%c%c your age is %f in days:\n",user_name1,user_name2,user_name3,user_age_days);
return 0;
}
From the code above lets define the elements that we have discussed earlier:
Elemets | Syntax used in the C program | Identifier/ Placeholder used | Complete code |
---|---|---|---|
Declaration of directives | #include | <stdio.h>, <stdlib.h> | #include <stdio.h> |
Declaration of constant variables | #define | days_in_years 365 | #define days_in_years 365 |
Identifiers | char , float | user_name 1, user_name2, user_name3, user_age, user_age_days | char user_name1,user_name2,user_name3; |
Operators | Assignment " = " operator | user_in_days | user_in_days=user_age*days_in_years; |
I/O statements | scanf, printf | %c%c%cc , %f | printf("May I know your 3 letter nickname:"); |
This is the code in the code::blocks
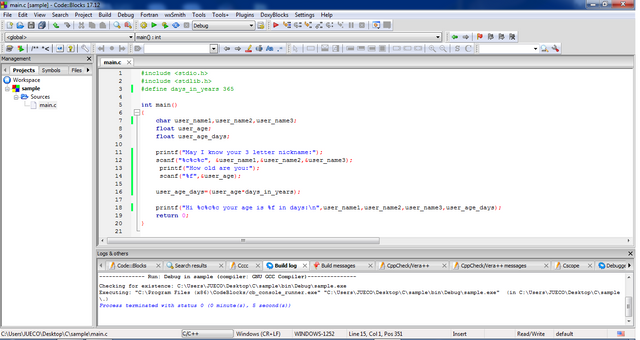
5 | Build the C program by clicking .
6 | Run the program by clicking .
7 | Enter 3 letter nickname
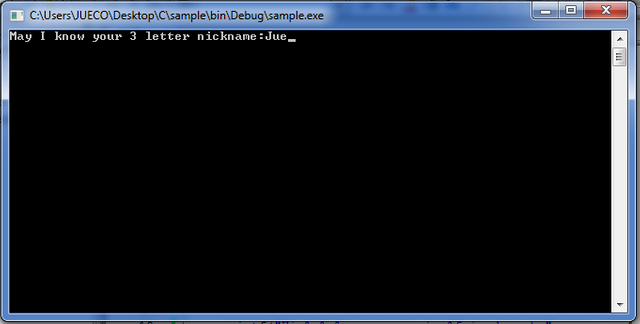
8 | Enter user's age.
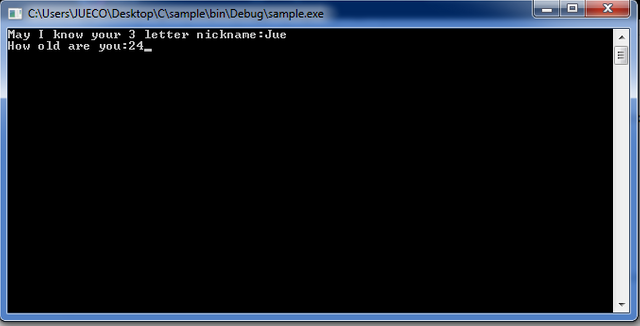
9 | Enter to show the result of the program.
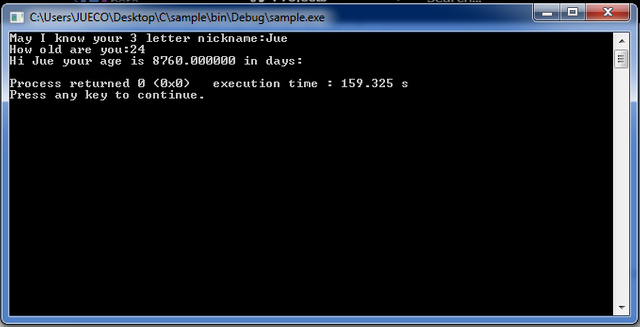
This is the snapshot of the code::blocks environment and the running C program.
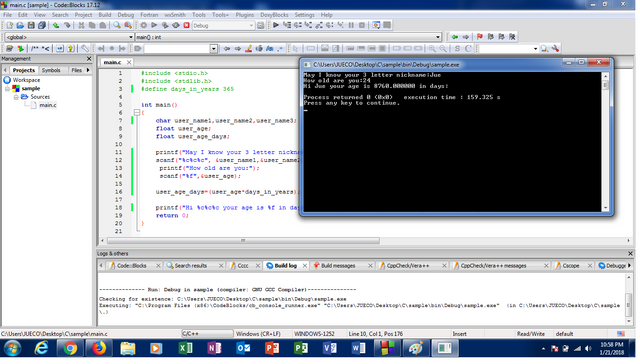
Thank you for reading this tutorial!
@curiousson
Posted on Utopian.io - Rewarding Open Source Contributors
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by curiousson from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
Your contribution cannot be approved because it does not follow the Utopian Rules.
You can contact us on Discord.
[utopian-moderator]
Congratulations @curiousson! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP