Learn Java FXML [Part 1]- Creating Scenes with email validation and scene switch
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn to Create a Dark themed UI Application with Java FX Scene Builder
- You will learn to switch FXML Scenes
- You will learn to Check if Email is valid
Requirements
- IntelliJ IDEA
- Basic Knowlege of JavaProgramming
Difficulty
- Intermediate
Tutorial Contents
In this tutorial, I will be putting you through creating a Dark UI, for BioData management Application using JavaFX. To begin with, first create a new JavaFx project in your IntelliJ IDEA Platform or any platform of your choice, you might require installing a scene builder for other IDE's. To create a JavaFx project do the following:
- Click on File - New - Project
- Click Java FX - Java Fx Application
- Click Next
- Fill in the Application Name and Project file location
- Click Finish
Select JavaFx an JavaFx Application
Input name and Location and click finish
You have just created a new Java FX Application.
Stage 1 - Create two FXML files
First, we will be adding two FXML files in our project, these files will contain the Login and Register Screens. By default, Java FX creates a FXML file called sample.fxml
. I have created another FXML file and named that addAccount to represent user registration and customized the sample.fxml file to suit login purposes.
Here's a simple screenshot of how to create a FXML file.
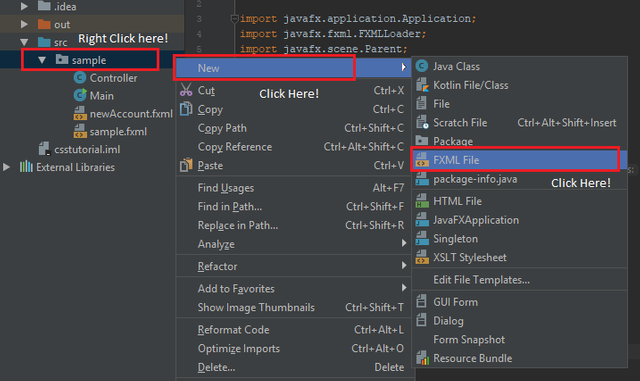
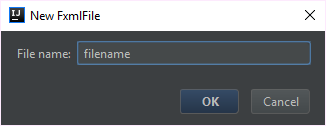
Once this is complete, go to the sample.fxml file an follow these steps. Our aim is to get a deep blue dark theme look for the user Interface.
Add an Anchor pane to the empty screen or If there is one present already, it should appear in the bottom left area of the scene builder screen as in the screenshot below. Simply delete its content. Now go to style in the properties pane, this is on the right side of your screen, enter
-fx-background-color
this is to enable us to change the color of the anchor pane from white to any desired color. I've used color code#9eacba
, looks like pale blue.
From the controls section of the pallete, add A textfield, Passwordfield, Labels and a button for the sign in option. Your screen should look like this now.
I've set the font size of all the labels to 14.Finally add a panefrom the containers section, add it to the left side of the anchor pane and set the background color to
#001224
as we have done with the anchor pane, also add other labels and a button Register tot he new panel, also set the backgroun color of the button to the same as the panel. At this stage,your screen should look like this.
Do the same for the second fxml file, make your screen look like this;
Now our login and regiser screens are set and we are ready to code. Note: The Logo look alike having Lx and corp beneath it are also labels but with different text formatting, easy to achieve anyways.
Stage 2 - Edit the main.java file.
The purpose of this stage is to avoid us from having half of our scene displayed, this is because Java FXMLmain files come with a specified dimension for the stage in whch the scene would be displayed and we don't want that affecting our work.
All we will be editing out of the code in this file are the specified dimension provided by default here Scene scene = new Scene(root,250,122);
, we'll be taking out the numbers so we are not disturbed. This way we are left with Scene scene = new Scene(root);
.
Here's the rest of the code in the main.java file, most of which is automatically generated.
package sample;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
primaryStage.setTitle("Luxencorp");
Scene scene = new Scene(root);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Yep just like every parent out there, to become a parent you must have children, the same works for JavaFx as the Parent carries the FXML file and reads the content as seen here Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
but then before a parent is called, there has to be a stage, this has been called in the start method public void start(Stage primaryStage)
After which we now edit the title of the Stage to desired using the stage.setTitle("Stage title");
method as done in this line primaryStage.setTitle("Luxencorp");
. Finally a scene is create from the parent which is then added to the stage and displayed, this is prety easy to understand and I'll be doing more on it later in the tutorial.
Stage 3 - Creating the email verification method
To make our application more user friendly, when a user enters username instead of email, the application shows a not valid text beside the space provided for the email,this can be seen onthe login screen. To be ableto achieve this, we would be making use of a text patter recognition method, to check if it is true or false that the email entered is valid or not.
To do this we'll be creating a method that will return a true or false value i.e boolean. First open controller.java and do the following;
- Create a boolean method with the syntax
public static boolean isValid(String email){}
in the main method. You can use any other name for your method, just make sure you can remember it. - Go to the imports and add these imports
import java.util.regex.Matcher;
, andimport java.util.regex.Pattern;
, these are the required imports for the validation. - GO back to the method and create a string variable to hold the format for the email, the syntax is
String stringname = "^[a-zA-Z0-9_+&*-]+(?:\\."+ "[a-zA-Z0-9_+&*-]+)*@" + "(?:[a-zA-Z0-9-]+\\.)+[a-z" + "A-Z]{2,7}$";
This String contains all alphanumeric characters, followed by '@' character, then another string of alphanumeric characters. - For now the string above is still simply a string and cannot be used for verification purposes until it is converted to a pattern. SO next we use the
Pattern.compile(string);
method to convert the string into a pattern, which can now be used for verfification. To create a pattern from the string, the syntax isPattern patternname = Pattern.compile(String);
, our pattern is now ready to be used for any test. - next we carry out the test using an If statement, what our test does is If-the email is not following the pattern-then it is not correct, this gives us a syntax of
if(email==null) return false;
simple right. - Finally we want to return the value to the system when an email is added as required by every method to return a value so we use
return patternname.matcher(email).matches();
syntax, the tells the system to return a true or false value depending if the email entered matches the pattern. The code there would bepat.matcher(email).matches();
Your full code for this method should look like this,anad show no errors.
public static boolean isValid(String email)
{
String emailRegex = "^[a-zA-Z0-9_+&*-]+(?:\\."+
"[a-zA-Z0-9_+&*-]+)*@" +
"(?:[a-zA-Z0-9-]+\\.)+[a-z" +
"A-Z]{2,7}$";
Pattern pat = Pattern.compile(emailRegex);
if (email == null)
return false;
return pat.matcher(email).matches();
}
Stage 4 - Testing the verification method with email to change text and color of label
After creating the method, now we use it in our code.But before it can be used, we have to set up an ActionEvent in which it will be used. The action event will be çonnected to the SignIn button for now, such that when the email is entered and the button is clicked, the system uses the method to check if it is valid anf if yes it sets the text beside the email textfield to "Valid email" with a green font color. I'll explain how o do all that.
First we have to create the ActionEvent listener, in the main class of controller.java create three FXML variables for the email textfield, signin button and the label beside the email textfield. The syntaxfor the textfield is @FXML private Textfield textfieldname;
, for Signin button @FXML private Button signinbtn;
and for the label @FXML private Label labelname;
, at this point you should have this;
@FXML
private TextField emailtxt;
@FXML
private Button signinbtn;
@FXML
private Label emailveri;
Next we create the Event handle, the syntax for creating an event handler is @FXML private void eventname(Eventtype eventvariable) throws Exception{ yourcode }
, for this method the event type we will be using is ActionEventas it is preferable when it comes to button actions. This leaves us with @FXML private void signinbtnclick(ActionEvent event) throws Exception {}
.
Inside the curly bracked, Create a String to hold the email i.e String email;
now we are getting the email from the email textfield so it will be String email = emailtxt.getText();
, this simply gets the text in the email field once the button is clicked.
Finally we call the isValid()
method into an if statment to carry out the test using if(isValid(email))
, this way the email string is tested as the email string in the method, now what do we want the system to do if the email is valid? the syntax if a complete if statement is if(condition){Do this}
, what I want the system to do is set the text of emailveri label to "Valid Email" with green color. To set the text we simply use emailveri.setText("Valid Email");
after this is done I wnat the color to change to green also so I'll just add emailveri.setTextFill(Color.web("#004d08"));
generally is should just be Color.anycolor
but then that is limited so I added the web so I can use the color code.
Your code should look like this now;
@FXML private TextField emailtxt;
@FXML private Button signinbtn;
@FXML private Label emailveri;
@FXML private void signinbtnclick(ActionEvent event) throws Exception{
String email = emailtxt.getText();
if(isValid(email)){
emailveri.setText("Valid Email");
emailveri.setTextFill(Color.web("#004d08"));
}
}
For now, the code will not work because the Button, Label and textfield variable names have not been added to the items in our scene, so go to your sceneand click on the Email textfield providedand under the code section, you will find fx;id
there enter the Textfield variable name you have created,like in the screenshot below.
Do the same for the other two. Now we add the ActionEvent listener to the signin button,just as we did to the variablenames above, but this time we add the event handle to the onAction space provided, the screenshot below shows this.
If you run your main.java now and enter a wrong email, there will beno change on the label but if the enter the right format for an email, you will notice the change asthe text will turn green.
Stage 4 - Switch scenes
Generally every new user has to register before he or she can be allowed access to user privileges, to be able to switch from the signin screen to the registration screen we have created, wewould also have to create an event handler for the register button we have placed in our Sign in screen. As we did for the signin button, follow the same syntax but this time we are using a differnt name for the event.
@FXML private Button regbtn;
@FXML private void regbtnclick(ActionEvent event) throws Exception{
Parent newparent = FXMLLoader.load(getClass().getResource("newAccount.fxml"));
Scene newscene = new Scene(newparent);
Stage window = (Stage)((Node)event.getSource()).getScene().getWindow();
window.setScene(newscene);
window.show();
}
@FXML private Button regbtn;
Thisis the variable name for the Registration button on the Sign in Screen.Parent newparent = FXMLLoader.load(getClass().getResource("newAccount.fxml"));
I have previously explained the concept of a parent, what it does is load the content of the fxml so it can be added to a scene, that is what the getClass and getResource does.Scene newscene = new Scene(newparent);
creates the new scene from the data pulled by parent.Stage window = (Stage)((Node)event.getSource()).getScene().getWindow();
Previously in the main method, the stage has been created and we want to use the same stage and not create another stage, so we get the Window from the same stage used previously in the main, using(Stage)(Node)event.getSource()
tells the system that the main.java for which this event is created contains a stage while.getScene().getWindow()
pulls the dimensions of the stage, therefore when the button is clicked, a new stage is not formed instead the system uses the same stage upon which the button was clicked.window.setScene(newscene);
Now this places the content gotten from the newAccount scene in the stage to replace the Sign in screen.window.show()
Displays the stage. Without this the registration screen would not be displayed.
Now add the varuable name regbtn
to the fx;id of the register button and the event listener rebtnclick
to the onAction in the signin scene as we did for the sign in button and others earlier.
If we run the main file now and click the Register button, the system switches to the register screen but if we click the back button, it doesn't return bac to the sign in screen. We also have to create an event handle for that, in the case of returning back, I have used a label so our even listener would be for a mouse click now, not just an action event.
Just repeat the steps above, but this time it is (MouseEvent event)
instead of (ActionEvent event)
and in the Parent line we use .getResource("sample.fxml")
instead of .getResource("newAccount.fxml")
this is because the Parent is meant to pull data from the fxml file you wan to be displayed. Your code should look like this;
@ FXML private Label backbtn;
@FXML
private void backbtnclk(MouseEvent event)throws Exception{
Parent newparent = FXMLLoader.load(getClass().getResource("sample.fxml"));
Scene newscene = new Scene(newparent);
Stage window = (Stage)((Node)event.getSource()).getScene().getWindow();
window.setScene(newscene);
window.show();
}
Add the backbtn
to the fx;id of the label provided for returning back to the signin screen,and add the event handler backbtn click
to on Mouse Clicked in the code section of the scene builder as seen in the screenshot below.
At this stage the system can now do all that is expected of it. If you have any questions kindly drop them, thanks for following.
Required Imports
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.scene.Node;
import javafx.scene.Parent;
import javafx.scene.*;
import javafx.scene.paint.Color;
import javafx.scene.control.*;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.input.MouseEvent;
import javafx.stage.Stage;
import java.awt.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
Curriculum
This is the first tutorial in this series.
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @creon
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!