Tutorial Intermediate - How To Create A 3-Dimensional Object (Cube)
What Will I Learn?
- You will learn how to create 3-dimensional objects
- You will learn the basic object of 3 dimensions
- You will learn C ++ programming language
Requirements
- You need high confidence
- You need basic about C ++ programming language
- You need the ability to create an object
- You need a basic 3D object
Difficulty
- Intermediate
Tutorial Contents
Well on this day I will give a tutorial how to make a 3-dimensional object using visual studio application Download Here Because this application facilitate in writing syntax which can be said long enough, as yng we know together that 3 dimensional object is an object of result of 2 dimension improvement and Object3 -D is a set of 3D dots (x, y, z) that make up the faces combined into a single unit. Face is a combination of dots that form a certain area or often called by side, if you want to create a 3-dimensional object you can follow the following steps:
- You can write the syntax below on the screen that has been created on the microsoft visual page and every syntax is explained in this tutorial:
You have to create a function contained in syntax include Is a header that is declared as the standard input output operation used in C ++.
#include <stdio.h>
Is graphical programming using OpenGL-GLUT requires glut header. h in the c: \ Program Files \ Microsoft Visual Studio \ VC98 \ bin \ GL folder
#include <GL/glut.h>
to perform mathematical calculations
#include <math.h>
The command to implement 3D transformation in the program is 3D Data Matrix structure.
typedef struct {
float m[4][4];
} matrix3D_t;
is a command to generate the program code to generate an image each time a userdraw is called.
void userdraw(void);
The command to implement the 3D transformation in the program is a Vector Data structure.
typedef struct {
float v[4];
} vector3D_t;
The command to implement the 3D transformation in the program is a Data Point structure
typedef struct{
float x,y,z;
} point3D_t;
Command as Color Data Structure to color the object
typedef struct {
float r,g,b;
} color_t;
command functions for matrix processing.
matrix3D_t createIdentity(void)
{ matrix3D_t u;
int i,j;
for (i=0; i<4; i++) {
for(j=0; j<4; j++) u.m[i][j]=0.;
u.m[i][i]=1.;
}
return u; }
The command serves to perform cross product (croos product).
matrix3D_t operator * (matrix3D_t a,matrix3D_t b)
{
matrix3D_t c;
int i,j,k;
for (i=0;i<4;i++) for (j=0;j<4;j++) {
c.m[i][j]=0;
for (k=0;k<4;k++) c.m[i][j]+=a.m[i][k]*b.m[k][j];
}
return c;
}
The command serves to create a rotational effect (rotating)
matrix3D_t rotationXMTX(float theta)
{
matrix3D_t rotate=createIdentity();
float cs=cos(theta);
float sn=sin(theta);
rotate.m[1][1]=cs; rotate.m[1][2]=-sn;
rotate.m[2][1]=sn; rotate.m[2][2]=cs;
return rotate;
}
The command works to create a scaling effect.
matrix3D_t scalingMTX(float factorx, float factory, float factorz)
{
matrix3D_t scale=createIdentity();
scale.m[0][0] =factorx;
scale.m[1][1] =factory;
scale.m[2][2] =factorz;
return scale;
}
The command serves to implement the 2D vector conversion into a 3D point
point3D_t Vector2Point3D(vector3D_t vec)
{
point3D_t pnt;
pnt.x=vec.v[0];
pnt.y=vec.v[1];
pnt.y=vec.v[2];
return pnt;
The command works for the implementation of 3D dot conversion into 3D vector.
vector3D_t Point2Vector(point3D_t pnt)
{ vector3D_t vec;
vec.v[0]=pnt.x;
vec.v[1]=pnt.y;
vec.v[2]=pnt.z;
vec.v[3]=1.;
return vec; }
The command serves for croos product of the vertices a = (ax, ay, az) and b = (bx, by, bz) cross-product implementations.
float operator * (vector3D_t a, vector3D_t b)
{
float c;
int i;
c=0;
for(i=0; i<3; i++){
c+=a.v[i]b.v[i];
}
return c; }
vector3D_t operator^(vector3D_t a, vector3D_t b)
{
vector3D_t c;
c.v[0]=a.v[1]b.v[2]-a.v[2]b.v[1];
c.v[1]=a.v[2]b.v[0]-a.v[0]b.v[2];
c.v[2]=a.v[0]b.v[1]-a.v[1]*b.v[0];
c.v[3]=1.;
return c;
}
The command works to give color
void setColor(float red, float green, float blue)
{ glColor3f(red, green, blue);
}
Commands to create lines.
void drawLine(float x1, float y1, float x2, float y2)
{ glBegin(GL_LINES);
glVertex2f(x1,y1);
glVertex2f(x2,y2);
glEnd(); }
Commands to create lines.
void drawLine (point2D_t p1, point2D_t p2)
{drawLine (p1.x, p1.y, p2.x, p2.y);
}
The command serves to draw a line.
void drawPolyline (point2D_t pnt [], int n)
{int i;
glBegin (GL_LINE_STRIP);
for (i = 0; i <n; i ++) {
glVertex2f (pnt [i] .x, pnt [i] .y);
}
glEnd (); }
The command works to draw lines with closed curves.
void drawPolygon (point2D_t pnt [], int n)
{int i;
glBegin (GL_LINE_LOOP);
for (i = 0; i <n; i ++) {
glVertex2f (pnt [i] .x, pnt [i] .y); }
glEnd (); }
The command works to color the polygon area with one color.
void fillPolygon (point2D_t pnt [], int n, color_t color)
{int i;
setColor (color);
glBegin (GL_POLYGON);
for (i = 0; i <n; i ++) {
glVertex2f (pnt [i] .x, pnt [i] .y);
}
glEnd (); }
The command works to produce gradient effects when used to colorize polygons.
void gradatePolygon (point2D_t pnt [], color_t col [], int num)
{int i;
glBegin (GL_POLYGON);
for (i = 0; i <num; i ++) {
setColor (col [i]);
glVertex2f (pnt [i] .x, pnt [i] .y);
}
glEnd (); }
The command to make a line on the X axis.
void drawcharX (float x, float y)
{drawLine (x, y, x + 10, y + 12);
drawLine (x, y + 12, x + 10, y);
}
The command to make a line on the Y axis.
void drawcharY (float x, float y)
{drawLine (x + 5, y, x + 5, y + 7);
drawLine (x, y + 12, x + 5, y + 7);
drawLine (x + 10, y + 12, x + 5, y + 7); }
The command to make a line on the Z axis.
void drawcharZ (float x, float y)
{drawLine (x, y + 12, x + 10, y + 12);
drawLine (x + 10, y + 12, x, y);
drawLine (x, y, x + 10, y);
}
The command serves to create a line on the X, Y and Z axes.
void drawAxes (matrix3D_t view)
#define HALFAXIS 220
#define HALFAXIS1 (HALFAXIS-10)
point3D_t axes [14] = {
{-HALFAXIS, 0,0}, {HALFAXIS, 0,0}, {HALFAXIS1,5,0},
{HALFAXIS1,0,0}, {0,0,0}, {0, -HALFAXIS, 0},
{0, HALFAXIS, 0}, {0, HALFAXIS1,5}, {0, HALFAXIS1.0}, {0,0,0},
{0,0, -HALFAXIS}, {0,0, HALFAXIS}, {5.0, HALFAXIS1}, {0,0, HALFAXIS1}};
vector3D_t vec [14];
point2D_t buff [14];
int i;
for (i = 0; i <14; i ++) {
vec [i] = Point2Vector (axes [i]);
vec [i] = view * vec [i];
buff [i] = Vector2Point2D (vec [i]);
}
drawPolyline (buff, 14);
drawcharX (buff [1] .x, buff [1] .y);
drawcharY (buff [6] .x, buff [6] .y);
drawcharZ (buff [11] .x-14, buff [11] .y); }
The command serves to declare a point on a face, and declares the number of points used to create the face, with a maximum of 32 dots.
typedef struct {
int NumberofVertices;
short int pnt [32];
} face_t;
The command serves to map the number of dots that make up an object. states the points that make up the face, with a maximum of 100 dots. mapping the number of faces that make up the object and declaring the faces that make up the object.
typedef struct {
int NumberofVertices;
point3D_t pnt [100];
int NumberofFaces;
face_t fc [32];
} object3D_t;
- And after you write all the syntax above you will get a 3-dimensional object like the picture below:
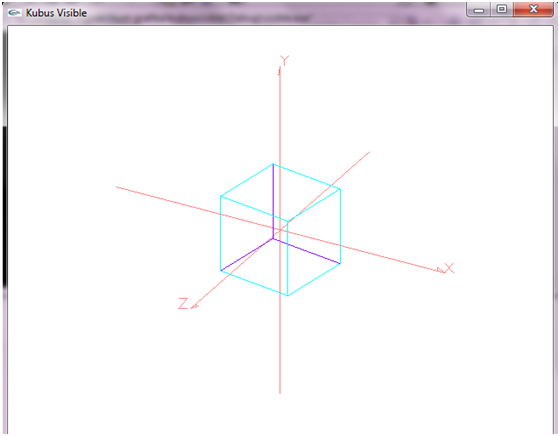
- All Syntax In My Google Drive
Here
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thank's Sir
Hey @brainalien I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x