Node Js Tutorial Creating a REST API #01 What's the API and How To Build It
What Will I Learn?
I will learn what's the RestFull API and what is the benefit to use it also how to build an API in Node JS .
- What's the RestFull API .
- Where we can use it and what's the HTTP Verbs .
- Build an API in Node JS.
Requirements
- Knowledge about JavaScript language .
- Basic Knowledge about Command Line window .
- An editor for example notepad ++ .
Difficulty
- Basic
Tutorial Contents
In this tutorial we will learn how to create a rest api with Node Js , Firstly we will talk about the API what's it and how to build an API , let's start ..
What's a restful API?
There is many definitions to this technology , the internet is based generally on the ' request / response ' the request from the client and the response from the server , the simple request from the client is to write ' https://utopian.io ' on the url box or click on a link that offers you the " Utopian " .
so the domain name or the url that you have entered is a request that goes to the server through a protocol and in our case the common protocol is http where it is a request loaded with some additional data such as browser type and user address and others and sends it to the server hosting the domain
The server receives and composes an answer loaded with some data, including the homepage for example ' index.html ' of the site or the error page or anything according to the response and returns it to the sender and the browser receives it and translates it to what is appropriate and opens the page.
The API or ( Application Programming Interface ) is a service that allows the user to send an address in a format loaded with some data will be received by the server and returns the answer according to the service.
Let's separate the word Rest API so firstly what's the word rest ?
Re = Representational
S = State
T = Transfer
So the word rest = Representational State Transfer , and we have also the word full to be restfull to transfer the full state.
There are lots of examples of famous APIs that we encounter every day. For example, Facebook provides an API for sites to log in without having to build a login system , when you purchase something online, this site uses the VISA API to communicate with the bank and deduct money from your card.
The API is a normal server that fetch and store data , it doesen't care about the individual clients that connected to it , we can name it ' Stateless Backends ' , let's explain it with an example
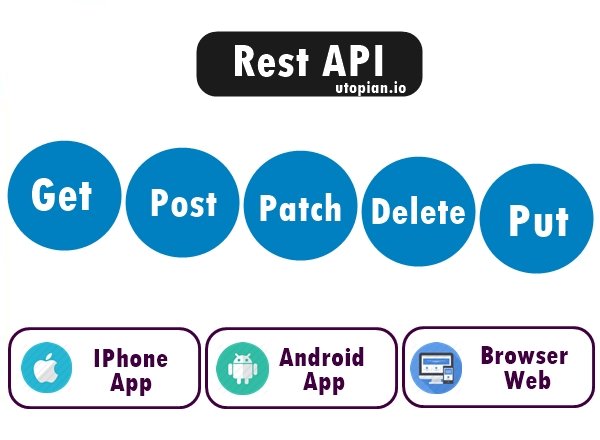
So we have our API server which is as I mentioned a normal server and it has for example URLS that supported by this server for example ' /sponsors' , '/moderators' and '/contributors' each one of them support also some HTTP verbs , in the picture I have 5 http verbs let's explain them
HTTP Verbs :
- Get : we use the get request to get informations just to read informations not to change it , the get do not change the state of resource , for any given HTTP GET API , if the resource that you have requested is found the server must return an ' ok message ' the
200
code , if the resource is not found the server will return404 (NOT FOUND)
.
The get request is formed like it :
HTTP GET https://utopian.io/sponsors
HTTP GET https://utopian.io/moderators
So when you form in your url box /sponsors you send a request to get the sponsors page and the server gives you this page . - Post : We use the post request to create a new resource into a collection of resources , when we create a new resource on the origin server , the response will be an http code
201 ( created )
, then if the resource has no content the server returns204 ( no content )
and many times it returns200 (ok)
with some details .
Simple example to post request
HTTP POST https://utopian.io/moderators/123/@alexendre-maxim for example - Put : we use the put request to update a resource that exists on this server , but if the resource not exists the API has absolute powers to create a new resource and the server returns
201 (created)
, but if the resource is exists and you want to update it by the ' put ' request the server returns200(ok)
to the user to signify it's OK , and we have also the204 (no content)
.
The Put request is almost similar to the Post request , but there is some changes to update existing resource for example
HTTP PUT https://utopian.io/sponsors/ned/1564879 to update the Delegated Steem Power for example , it's just an example to explain to you how to be the request . - Delete : we use the delete request simply to delete resources identified by the request url , when the server delete the resource , the message returned is
200
it means OK the resource has been deleted , the server may return the message202(accepted)
it means ' the request in the queue ' or the message204(no content)
.
HTTP DELETE https://utopian.io/@alexendre-maxim to delete this user for example . - PATCH : we use the patch request to make a special update on a resource , the difference is patch to make a special update but put to make a full update , let's understand the PATCH with an example
HTTP GET https://utopian.io/sponsors/1
The response from the server is :
{id: 1, username: 'freedom', SP: '1000'}
To update the SP of this sponsor we must send a request to the server
HTPP PATCH https://utopian.io/sponsors/1
[
{ “op”: “replace”, “path”: “/SP”, “value”: “20000” }
]
There is many operations to update a resource ' test , remove , add , move and copy ' .
The server connected with the verbs ' put , patch ..etc ' by the http/s protocol and for that if you notice that I have written ' HTTP GET , HTTP POST ..etc ' , so the protocol or the communication between the API server and the verbs is the HTTP protocol .
When a client send a request regardless of the REST (Android App, IPhone App or Browser/web ) , it will communicate with the verbs ( put , post ..etc ) by the ' JSON/XML ' and the server will return a JSON object as a result , an XML , or a URLEncoded ..etc
The Planning :
In this serie I want to build a simple application using the API and this is the planned
This is the application that we will build in this serie , I have my API server for utopian io for example , and I have the sponsors and the contributions I can get the list of sponsors and contributions , I can also add a new sponsor or contribution , then by the id of the sponsor I can update it .
To build this application firstly we must create this API .
Build The API :
To build an API I need firstly to make a directory and I will name it ' nodeRestUtopian ' from the command line by the mkdir nodeRestUtopian
then I need to navigate to it by cd nodeRestUtopian
Now we have an empty folder , I need to install the package.json file to install dependencies by the npm ( node package manager ) and to understand what means ' npm and dependencies ' you must read this contribution , so firstly we need to install the package.json and to do it we must type npm init
This is the result I have created my package.json file by this name and description ..etc , if you want to read more about the package.json file learn here .
So this is my package.json file that I have created from the command line
Now to create my API I need to use the EXPRESS as a framework to make the building easy and to use it we must install the package as dependency npm install express --save
After installation a new folder appears named ' node_modules ' has the 50 packages from express that we have installed , and this is what it contains
Creation of server
Now I am going to create the server and to do that I need to add a new javaScript file I will name it for example serverUtopian and the extension is .js
Inside my serverUtopian I will firstly get the http module by var http = require('http')
to create my server .
Then I need to specify the port and for that var port = 3000
, I will now create the server by the ' createServer() ' function to be like it
var server = http.createServer();
server.listen(port);
I will leave the function empty now , this function will execute when there is a new request , and I listen by the server to the port 3000 .
I will create another js file named ' app ' which spending up this express application which will make handling requests easy for us , inside my app file I am going firstly to import the express module by var express = require('express')
then I will create another variable var app = express()
and I will run the express() as a function in this app variable .
Currently I need to call a method called ' use ' by the app variable , this function called ' middleware ' , earch request or response come to this method , this function has many formats in this example we will apply this format app.use(function(req,res,next))
I have the request = req , the response = res and a special parameter called next to go to the next middleware .
Am going to use my response to give a reponse to the client and to do that we must type res.status(200)
to give the ' ok message ' then res.status(200).json({
I used a json object to send to the client a message which is " utopian the server works " .
message : "Utopian the server works"
})
The app is now private to be public we must export it module.exports = app
, this is the full code
Go back to the server file and import the app by var app = require('./app')
and I need to pass the app to the createServer function createServer(app)
and this is the full code
The Postman Tool
You can test the request by a tool named ' Postman ' .
Among the many solutions to query or test webservices and APIs, Postman offers many features, a quick start and a nice graphical interface.
Postman exists as an App (Windows / MacOS / Linux) and a Chrome App. However Chrome Apps live their last days, it is recommended to use the desktop version.
So firstly let's create a request by Postman , the program offers a menu has 6 items , let's select the first ' request '
Then chose the http verb that you want ' post , get , patch ..etc ' then enter the URL in our case localhost:3000
then click on send
It gave us the same result the message : " Utopian the server works " appears
In order to fully understand the terms that I have used, you should read past tutorials in which I have provided all about Node JS and Node Package Manager .
Curriculum
- Node Js Tutorial Select From Where , Order by , Delete and Limit Using Mysql Module
- Node Js Tutorial Serve HTML Pages , Serve JSON Data , Connect And Insert Into DB Using Mysql Module
- Node Js Tutorial How To Create Read And Write Stream Manually And Automatically For Big Files
- Node Js Tutorial Read , Write and Edit files and directories by fs module
- Node JS Tutorial How To Use Event Emitter By Events Module
I am learning NodeJs and seems it will be very helpful for me, thanks @alexendre-maxim for your contribution
Thank you for the contribution It has been approved.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Thank you sir
Hey @alexendre-maxim
We're already looking forward to your next contribution!
Utopian Witness!
Vote for Utopian Witness! We are made of developers, system administrators, entrepreneurs, artists, content creators, thinkers. We embrace every nationality, mindset and belief.
Want to chat? Join us on Discord https://discord.gg/h52nFrV