#PythonTutorials Lets make a simple file sorter using Python!
Before I begin, let me tell you something about Python. It is one of the strong and powerful languages available today.
Python is powerful... and fast;
plays well with others;
runs everywhere;
is friendly & easy to learn;
is Open.
I learned python for a project that was assigned to me a year back and started lovin' it since then.
In this post, I'm trying to create a simple file sorter to manage files of different file types by separating them into folders with a logical name. It is intended to help python beginners to understand file management and regular expressions with python.
A note before starting, I'm using Linux. Since the filesystems differ across various OS platforms, this may not work for other OSs.
So let's begin....
We will require the os module which is inbuilt in python, to use some system commands. To import a module we use the import command.
import os
To check categorize the files we need to identify the file types, we can use Regular Expressions for this purpose. So lets import re
import re
Collecting the list of files
Now let's list out the files we have in the current directory. listdir() function returns the files and directories where our python file which we are about to create is placed. listdir() function is part of the os module.
os.listdir()
will return every file and folder as a list of strings which include their name. But we just need the files for our job. So how do we get rid of the folders? os.path.isfile(path) to the rescue! It returns true if the string path is a file, false otherwise.
So implementing a list comprehention, we can get all the files inside the current directory like this:
files = [x for x in os.listdir() if os.path.isfile(x)]
The above piece of code will check each string in the list returned by os.listdir() and if os.path.isfile() for that string is true then stores it to files.
So we got all our required files inside the variable files.
Creating regular expressions
Now we need to categorize these files according to their file types. For that, we require the help of re, The Regular Expression module.
Let's create a regular expression to identify image files.
imgre = re.compile(r'\.(jp(e)?g|png|svg|ico|xcf)$', re.I)
The re.compile() function expects two arguments, one is the regular expression string and the other is a flag. Here we have used r'.(jp(e)?g|png|svg|ico|xcf)$' as the regular expression which matches with the strings end with :
- .jpeg
- .jpg
- .png
- .svg
- .ico
- .xcf
The re.I is a flag given to make the regex(Regular Expression) case insensitive.
You may add more extensions along with it if you need. You can read more about Regular Expressions here.
Similarly, create regular expressions for different file types.
#zip files
zipre = re.compile(r'\.(zip|rar|tar\.(gz|bz2))$', re.I)
#doc files
docre = re.compile(r'\.(doc(x)?|pdf|ppt(x)?|xlsx|csv|json|txt|dat|odt)$', re.I)
#html files
webre = re.compile(r'\.(html|xml|css)$', re.I)
#scripts
scriptre = re.compile(r'\.(sh|jar|c)$', re.I)
#executabless & installers
appre = re.compile(r'\.(apk|exe|deb|pkg|AppImage|bin)$', re.I)
Creating folders
Ok, now we can make the new home for our files, folders. Folders can be created using the os.makedirs('dir_name') which takes one string argument which is the folder name. We only need to create a folder if it already doesn't exist, it will be useful when we run the script more than once, where trying to create existing folders may result in an error. To check whether a folder exists or not use : os.path.exists('dir_name'), where dir_name is the directory path.
Now let's create the required folders for our files
if not os.path.exists('doc_files'):
os.makedirs('doc_files')
if not os.path.exists('image_files'):
os.makedirs('image_files')
if not os.path.exists('zipped_files'):
os.makedirs('zipped_files')
if not os.path.exists('script_files'):
os.makedirs('script_files')
if not os.path.exists('application_files'):
os.makedirs('application_files')
if not os.path.exists('web_files'):
os.makedirs('web_files')
Matching the regular expression & moving the files
To match a string with a regular expression, we can use compiled_re.search('string') where the string is matched with the compiled_re we created before like imgre, zipre, docre, webre etc.
If a match occurs we must move the file to a category folder like img_files, doc_files, etc. Moving a folder is simply changing the path of it. We can change the path to a file using os.rename('path_name', 'new_path_name') which takes two string arguments, first one is the current pathname, the second one is the new path name.
for i in files:
if imgre.search(i):
os.rename(i, 'image_files/'+i)
elif zipre.search(i):
os.rename(i, 'zipped_files/'+i)
elif docre.search(i):
os.rename(i, 'doc_files/'+i)
elif scriptre.search(i):
os.rename(i, 'script_files/'+i)
elif appre.search(i):
os.rename(i, 'application_files/'+i)
elif webre.search(i):
os.rename(i, 'web_files/'+i)
Above code will prefix the folder names before the file name in the pathname of the file, if a particular re matches.
Now save the file as filesorter.py and put it in a folder you want to arrange the files. and run it using terminal by typing:
python filsorter.py
FileSorter - Github link
See filesorter in action
Look at my downloads folder before sorting :
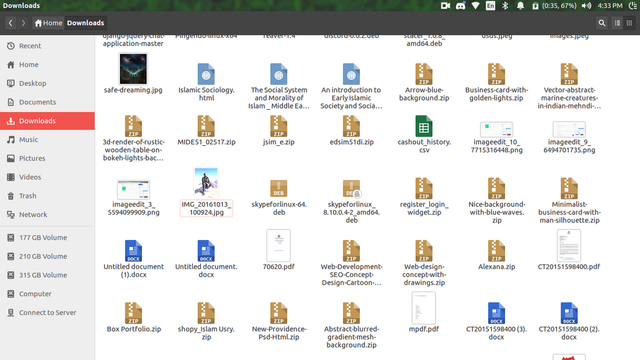
After Sorting:
The leftover filetypes were not included in the regular expressions. You may include them as you need :)
Thanks for reading
NB: I am not a professional Python programmer (Still a student), so I request you to correct me if I am wrong at anything and all criticisms will be readily welcomed. Peace!
Posted on Utopian.io - Rewarding Open Source Contributors
Hey @ajmaln I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
This post easily deserves more.. Keep it up bro.
Thank you @pangoli
You flag first
Approved.
You can contact us on Discord.
[utopian-moderator]
Thanks :)
Come and learn what activities AI likes :)
@OriginalWorks
The @OriginalWorks bot has determined this post by @ajmaln to be original material and upvoted(1.5%) it!
To call @OriginalWorks, simply reply to any post with @originalworks or !originalworks in your message!