ULOG: Java Programming Basics 13 - Stream Method Example
Lecture 13: The stream() Method Example
The stream() method example problem statement
Implement the ‘stream()’ method to print a list of integers
The stream() method example solution code
integers.stream().forEach(i -> System.out.print(i + " "));
The stream() method example output
6 7 8 9 10
Hi there, My name is Marius from AlefTav Coding. Today, I will look at the two concepts, stream() and lambda. In this lecture you will learn how to, 'Implement the stream() method to print a list of integers'
stream() is a feature that was introduced in the Java 8 release in 2014. Because most Java programs have to process very large collections of data, the stream() feature was incorporated to help deal with this. In fact, the main aim of stream() is to let you write code that can easily handle big collections.
Lecture starting code
Below, I have a collection of numbers named, integers. I want to be able to process this collection using Java's stream() implementation:
import java.util.List;
public class Lecture13 {
public static void main(String[] args) {
List<Integer> integers = List.of(6, 7, 8, 9, 10);
// TODO: Implement the ‘stream()’ method to print a list of integers
}
}
My solution implementation code is:
integers.stream().forEach(i -> System.out.print(i + " "));
I have to turn the collection of numbers into a Java stream. Technically, I am making a call to the stream() method with the code, dot stream() or .stream(). The two round brackets, ( ) after the word, stream indicate that I am working with a method.
Once my collection has been turned into a stream of values, I can then call certain specified Java methods. In this example, I am calling the method, forEach(). This method is used because I am going to process each value individually. In particular, I want to print each value, separated by a single space.
We say that forEach() calls a lambda expression to do this. This lambda code is read as follows. Given a value i, short for integer, return that value by printing it on the computer screen. Then, add one space, + " ". The lambda operator is a dash, followed by a greater than symbol, ->.
If you do not understand this code at first, think it over and eventually it will begin to make sense.
Complete solution
import java.util.List;
public class Lecture13 {
public static void main(String[] args) {
List<Integer> integers = List.of(6, 7, 8, 9, 10);
integers.stream()
.forEach(i -> System.out.print(i + " "));
}
}
My class in today's lesson is, Lecture13. As always, with Java, there must be a main() method. Then, I am creating a collection to work with.
My data type is a general List with a sub-type, Integer, between angle brackets, < >. Note the capital letter, I in the word, Integer. With a List, you have to use the non-primitive data type, which start with capital letters, Integer. The reason I am using the Integer data type is because my values are discrete, whole numbers. I declare the list of values as integers, all small letters. Then, I assign the numbers by adding each of the numbers 6, 7, 8, 9 and 10 to my list with the expression:
List<Integer> integers = List.of(6, 7, 8, 9, 10);
Then, just as with the Scanner class in a previous lecture, I must do an import. This time it is for, List. My import being:
import java.util.List;
With my program complete, I can run it. The output is, 6 7 8 9 10. The value 6, followed by one space, followed by 7, one space all the way up to the value 10 and one space.
Stream() method coding exercise
In this lecture you learnt how to implement a stream() method and a lambda. These are two concepts you should try to understand thoroughly, because it will make your life as a java developer so much more pleasant. Now, as usual, I have here a coding exercise for you to practise your new-found skills.
import java.util.List;
public class Lecture14 {
public static void main(String[] args) {
List<String> brics = List.of("Brazil", "Russia", "India", "China", "South Africa");
// TODO: Implement the 'stream()' method to print the names of the 'BRICS' countries
}
}
My name is Marius from AlefTav Coding. Till our next meeting, KEEP CODING.
Join me on Trybe.one (tokenized information-sharing platform) to earn 100 tokens for signing up and 10 tokens for logging in daily:
This post was made from https://ulogs.org
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
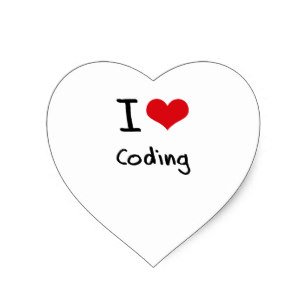
Reply !stop to disable the comment. Thanks!
This user is on the @buildawhale blacklist for one or more of the following reasons: