ULOG: Java Programming Basics 11 - Ternary Operator Example
Lecture 11: Ternary Operator Example
Ternary operator problem statement
Implement the ‘Ternary’ operator where ‘grade’ is 74 and ‘result’ is a String.
Print out ‘Pass’ if result >= 60 and ‘Fail’ otherwise.
Ternary operator solution code
String result = (grade >= 60) ? “Pass” : “Fail”;
Ternary operator output
Pass
Hi there, This is Marius from AlefTav Coding. Today, I will look at Java's Ternary operator, which is a statement that includes conditions. In this lecture you will learn how to 'Implement the Ternary operator where 'grade' is 74 and 'result' is a String. Print out Pass if result >= 60 and Fail otherwise.'
Lecture starting code
public class Lecture11 {
public static void main(String[] args) {
int grade = 74;
// TODO: Implement String, ‘result’ Pass/Fail
System.out.print(result);
}
}
So, here I have a class, Lecture11 with a main() method. I declare grade as an int data type and set its value to 74.
The solution code is as follows:
String result = (grade >= 60) ? “Pass” : “Fail”;
You read this line as: If a student's 'grade' is larger than or equal to 60, then the String, result holds a reference to the text, Pass. Otherwise, grade points to the text, Fail.
Complete solution
public class Lecture11 {
public static void main(String[] args) {
int grade = 74;
String result = (grade >= 60) ? “Pass” : “Fail”;
System.out.print(result);
}
}
With my program complete, it can then be run. It produces the output, Pass, because the value, 74 is larger than the condition, greater than or equal to 60.
Ternary operator coding exercise
In today's lecture you learnt a somewhat advanced concept, the Ternary operator. Also, how to implement it in Java. Now, to practise your understanding of it, here is the coding exercise, for you to try as homework.
public class Lecture12 {
public static void main(String[] args) {
double wages = 3900;
// TODO: Implement the *Ternary* operator where *wages* are 3700 and *earnings* are a **String**.
// TODO: Print out *Exempt* if *earnings* < 4500 and *Taxable* otherwise.
System.out.print(earnings);
}
}
My name is Marius from AlefTav Coding. Till our next meeting, KEEP CODING.
If you want to earn tokens on EVERY post you create use the referral URL below:
This post was made from https://ulogs.org
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
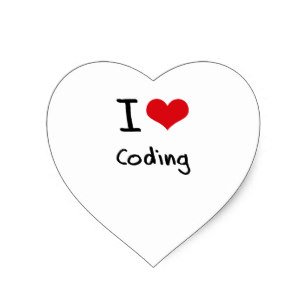
Reply !stop to disable the comment. Thanks!
@ilovecoding, Thank you for the resteem and upvote. I have upvoted your comment.
This user is on the @buildawhale blacklist for one or more of the following reasons:
This user is on the @buildawhale blacklist for one or more of the following reasons: