Brighttux's chronicles of playing with and learning steem.js API #1
Hey guys! Thanks for dropping by. My apologies for tagging you (i happen to see your names while extracting some data :smiley:)
tl;dr version
I'm testing out the steem.js APIs, was inspired by @zneeke's work.
Quick questions:
Should i post this to utopion.io instead? It's more like a story of my journey though...
WARNING:
Non-technical people will get bored of this post, considered yourself warned... lol
As an apology, here's a photo i took of a jetty in the beautiful island of Lang Tengah, Malaysia.
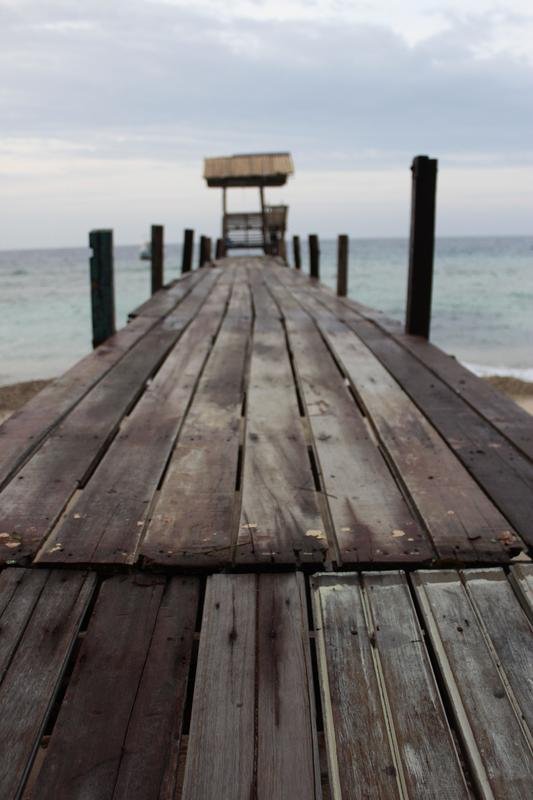
And for all you who wants to read more:
I thought of doing something different from my regular gaming streams, and i'm thinking of ways to improve the steemit platform as well as to get to know it better, hence i decided to spend some time playing with the steem.js API which is available here.
As someone who is somewhat know my way around the programming world, i thought, maybe javascript would be an easy pickup for me to learn more about the steemit platform.
In this series, i'll be documenting my findings and my journey as i explore this "unknown" world... at least to me. And if you're interested, you can follow along and see if it'd help you in your personal quest in the Steemit platform.
Now, to begin with, THIS post by @zneeke caught my interest as he managed to grab data related to @dlive within a specified time frame, and sorted the results based on the payouts, categories, and the such.
I thought, maybe i could replicate something like this and make some kind of report for everyone who is using @teammalaysia's tag and see how we can improve as a community on this platform.
The setup was fairly simple as it was well documented in the github page:
//Just a one liner, provide you've installed npm beforehand.
//Launch your console, and enter the following code:
$ npm install steem --save
//Or if you're using some linux platform like myself,
//remember to use the sudo command :)
$ sudo npm install steem --save
Done, steem-js has been "installed" on your system. Now, to the next step.
I created a directory on my pc, and named it "Steem-Grab-Tag" ... you can obviously name it whatever you want :) I'm assuming you won't need me to teach you how to create a directory :p
And within that directory, i created 3 files.
- index.html - the basic html file
- bundle.js - a place where i group all my js scripts
- steem.min.js - required file
IDEA:
The current idea was simple. I just wanted to grab all the post with the tag "teammalaysia", and maybe, i could somehow find a way to sort the post based on the time it was created, the payout value, number of votes, and even the number of "useful" comments written on the post.
So i went back to the documentations provided by the developers and i found this.. i thought, hmm, let's try this out:
steem.api.getDiscussionsByActive(query, function(err, result) {
console.log(err, result);
});
So i created those 3 files and ran the codes and the following error was returned to me
"ReferenceError: query is not defined"
Okay, no surprise there, as i have not specified what the variable query is. So i thought hmm, maybe it's similar to the sample that the developers gave, where i can replace those "strings within an array"- ['ned','dan'] with whatever i want:
steem.api.getAccounts(['ned', 'dan'], function(err, response){
console.log(err, response);
});
// tested it with:
steem.api.getDiscussionsByActive(['teammalaysia'], function(err, result) {
console.log(err, result);
});
But nope, it returned me some error again saying that i've given the function a wrong input.
data: Object { code: 7, name: "bad_cast_exception", message: "Bad Cast", … }
So the next step i did, was obviously search the internet for advice haha.. and to my surprise (or lack of), there were many people complaining about the poor documentations done by the team :(
*sads *
Nevertheless, one post led to another, another comment led to another, and finally i found this page here which is basically where users raise issues in github and ask the developers how to fix certain issues.
Turns out, based on that page, the format of the object "query" looks something like this:
var query = {
tag: 'introduceyourself',
limit: 10,
start_author: 'lada94',
start_permlink: 'introduce-youself-steemit'
};
steem.api.getDiscussionsByTrending(query, function(err, result) => {
console.log(err, result);
});
where the object query had a few members, namely "tag", "limit", "start_author" and "start_permlink"... so i though, okay cool... now we're getting somewhere.. and so, i tested out the code again with new parameters:
var query = {
tag: 'teammalaysia',
limit: 10,
start_author: 'lada94',
start_permlink: 'introduce-youself-steemit'
};
steem.api.getDiscussionsByActive(query, function(err, result) {
console.log(err, result);
});
and cool... there was some results, and interestingly, i saw quite many familiar names such as @danielwong, @howtostartablog, @littlenewthings, @bitrocker2020, @chloephuan93 and the others whom i shall not tag for no reason :p
Some questions pop into my mind:
But what does "start_author" and "start_permlink" actually means? Who is the world is lada94? Can i replace it with my own steemit handle?
so... like all "researchers" ... i did an experiment using the following parameters:
var query = {
tag: 'teammalaysia',
limit: 10,
start_author: 'brighttux',
start_permlink: 'introduce-youself-steemit'
}
and NOPE, it didn't work... RPCError? Huh? What's that? Unknown key? Huh?
RPCError
code: -32000
columnNumber: 24327
data: Object { code: 13, name: "N5boost16exception_detail10clone_implINS0_19error_info_injectorISt12out_of_rangeEEEE", message: "unknown key", … }
fileName: "file:///home/bright/Documents/Github/Steem-Grab-Tag/steem.min.js"
lineNumber: 4
message: "unknown key:unknown key: "
name: "RPCError"
stack: "e@file:///home/bright/Documents/Github/Steem-Grab-Tag/steem.min.js:4:24327\na/<@file:///home/bright/Documents/Github/Steem-Grab-Tag/steem.min.js:4:23853\n"
__proto__: Object { … }
Unsatisfied, i googled more, and found out that the start_author and start_permlink are somewhat related to each other.. and so i thought, welp, i'm just gonna throw them out of the window... and so i did, just using the "limit" and "tag" parameter... and the results turn out fine again.
var query = {
limit: 10,
tag: 'teammalaysia'
};
Upon further investigation, i found out that all the codes are somehow linked back to it's C++ components.. and so i digged through the documentations and codes and i found the following from here:
struct discussion_query
{
void validate() const
{
FC_ASSERT( filter_tags.find(tag) == filter_tags.end() );
FC_ASSERT( limit <= 100 );
}
string tag;
uint32_t limit = 0;
set< string > filter_tags;
set< string > select_authors; ///< list of authors to include, posts not by this author are filtered
set< string > select_tags; ///< list of tags to include, posts without these tags are filtered
uint32_t truncate_body = 0; ///< the number of bytes of the post body to return, 0 for all
optional< string > start_author;
optional< string > start_permlink;
optional< string > parent_author;
optional< string > parent_permlink;
};
It turns out that there were plenty of other parameters you could play with... and there were some optional ones too.
Anyways, this will be it for the first chronicle of my journey playing with the steem.js API.
While i have not done anything significant yet, it's still something i guess? haha...
That's one small step for (a) man; one giant leap for mankind - Armstrong
I certainly hope the developers would improve on their documentation, but maybe, i can one day take up the challenge and update it for the good of the @steemit community
If you made it to the end, thanks for reading! You're amazing!
Here are the contents if you wanna replicate it:
following the documentation and install it, here's the other files :)
- index.html
<!DOCTYPE html>
<html>
<head>
<title>Steem-Tag-Retrieval-Module</title>
<meta name="viewport" width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0>
<link rel="stylesheet" type="text/css" href="mystyle.css">
<style> body {padding: 0; margin: 0;} </style>
(html comment removed: https://www.npmjs.com/package/natural-sort )
<script src="./steem.min.js"></script>
<script src="./bundle.js"></script>
</head>
<body>
</body>
</html>
- bundle.js
steem.api.getAccounts(['brighttux'], function(err, response){
console.log(err, response);
});
var query = {
limit: 10,
tag: 'teammalaysia'
};
steem.api.getDiscussionsByActive(query, function(err, result) {
console.log(err, result);
console.log("---------------------")
});
- steem.min.js
you can get it from this link
just download and rename the file to steem.min.js
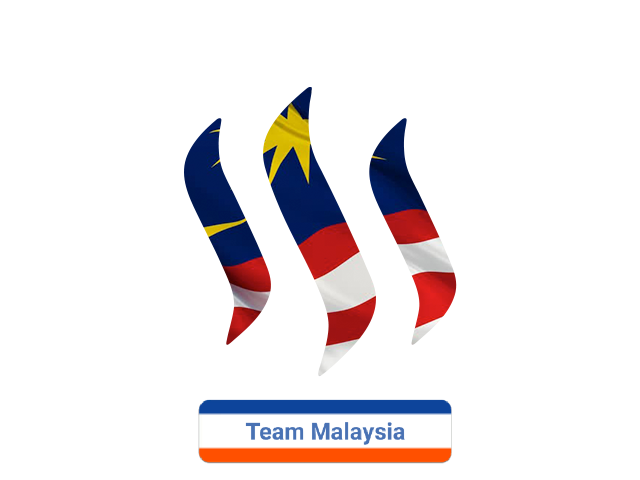
Bonus potato haha:
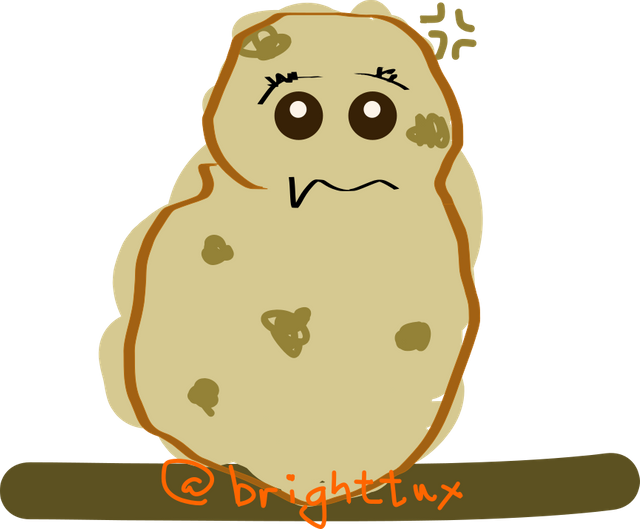
Nice work :)
Keep at it and experiment. Try out things. The only problem I ran into was that there is a lack of examples but I see that you're doing well.
heys, thanks! Yea, there's really not much examples around... have i not seen your post, i would not have thought that it is possible to have something like that done :)
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 22 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 24 SBD worth and should receive 116 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
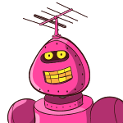
TrufflePig
thanks trufflepig! Now, go conquer the world and spread my post around .. XD
You've been upvoted by TeamMalaysia Community :-
To support the growth of TeamMalaysia Follow our upvotes by using steemauto.com and follow trail of @myach
Vote TeamMalaysia witness bitrocker2020 using this link vote for witness
Keep up the great work! I find steem.js sometimes the documentation is lack of example, a lot of testing needed to be done. I personally am using SteemSQL to extract my required data with MSSQL command, but it came with a subscription fee of 10 SBD/month.
Yea, i realized immediately after going through their documentation... it's quite bare bone..
10 SBD/month? haha... that's heavy.. i'll stick with steemjs for now :p
Oh yeah, forgot to tip @tipu upvote this post with 0.2 sbd
Sorry, @tipU needs to recover voting power - will be back in 5 hours and 3 minutes. Please try then!
Your Post Has Been Featured on @Resteemable!
Feature any Steemit post using resteemit.com!
How It Works:
1. Take Any Steemit URL
2. Erase
https://
3. Type
re
Get Featured Instantly & Featured Posts are voted every 2.4hrs
Join the Curation Team Here | Vote Resteemable for Witness
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by brighttux from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
This post has received a 5.03 % upvote from @boomerang.