SteemJ V0.4.1 has been released ~ Use the Steem API in your Java Project
SteemJ v0.4.1 can switch between Steem Nodes on the fly, is finally compiled for Java 7 and contains several other improvements.
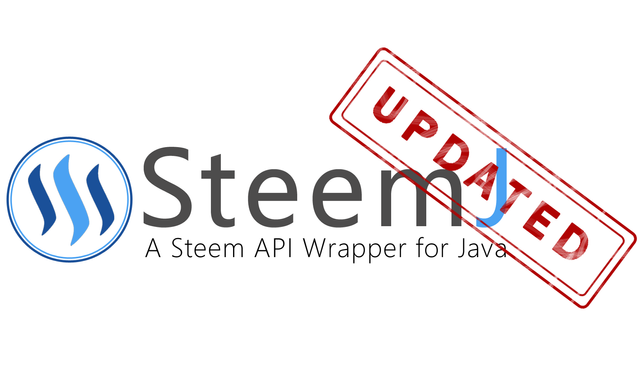
Previous 0.4.x releases: v0.4.0
SteemJ V0.4.1 has been released ~ Use the Steem API in your Java Project
Hello Steemians!
I am pleased to announce a new release of SteemJ: Version 0.4.1.
Before continuing with the default procedure of my update posts I need to give a big shout out to @ray66rus who helped me a lot with this release - Thanks to @ray66rus SteemJ is finally running on Android devices out of the box which is a huge improvement.
As stated in the title this version is shipped with a bunch of further improvements from which I will present only those in detail, that directly effect you as a user of SteemJ. As always, the full changelog can be found later on in this post.
Connection failover
In times where Steem Nodes being ddosed I thought it may be a good idea to add a failover to SteemJ. Version 0.4.1 allows you to define multiple endpoints and will automatically switch to another node in case of a connection problem. The best thing is that you do not need to take care about it - Everything is handled internally and you will not even notice it.
By default SteemJ 0.4.1 is shipped with three nodes configured:
- 'wss://steemd.steemit.com'
- 'wss://seed.bitcoiner.me'
- 'wss://steemd.minnowsupportproject.org'
And also allows you to add additional ones:
myConfig.addWebSocketEndpointURI(new URI("wss://seed.bitcoiner.me"), true);
Or override the whole list of default nodes:
// Reset the currently configured endpoints.
myConfig.setWebSocketEndpointURIs(new ArrayList<Pair<URI, Boolean>>());
// Change the default settings if needed.
myConfig.addWebSocketEndpointURI(new URI("wss://seed.bitcoiner.me"), true);
Using a Test-Net
A lot of you guys run their applications against other chains like the official test net or the https://testnet.steem.vc net, which is really great. Sadly, SteemJ was made and tested for the official Steem chain so far. This release adjusts and adds the remaining parts, so that finally everything will also work in the testnet.
Here is a small sample:
SteemJConfig myConfig = SteemJConfig.getInstance();
// Set the expected address prefix (Was named SteemitAddressPrefix in older versions).
myConfig.setSteemitAddressPrefix(AddressPrefixType.STX);
// Disable the asset validation because it is only valid for the offical chain so far (This will also be improved in upcoming releases).
myConfig.setValidationLevel(ValidationType.SKIP_ASSET_VALIDATION);
// Set the chain id.
myConfig.setChainId("00000000000000000000000000000000000");
// Change the node:
myConfig.setWebSocketEndpointURIs(new ArrayList<Pair<URI, Boolean>>());
myConfig.addWebSocketEndpointURI(new URI("wss://EXAMPLE-TESTNET-ENDPOINT"), true);
// Start posting:
steemJ.createComment(new AccountName("steemj"), new Permlink("testofsteemj040"), "Example comment without a link but with a @user .", new String[] { "test" });
Simplified Reblog Operation
In the last 6 month I received a lot of questions about the 'CustomJsonOperation' which is used to 'follow', 'unfollow' and 'mute' a user and to 'reblog' a post of another author.
SteemJ 0.4.0 already added a simplfied 'follow' and 'unfollow' operation and SteemJ adds the missing 'reblog' operation that allows you to reblog a post with a single command.
SteemJConfig myConfig = SteemJConfig.getInstance();
// Set your default account:
myConfig.setDefaultAccount(new AccountName("dez1337"));
// Add your keys:
List<ImmutablePair<PrivateKeyType, String>> privateKeys = new ArrayList<>();
privateKeys.add(new ImmutablePair<>(PrivateKeyType.POSTING, "YOUR-PRIVATE-POSTING-KEY"));
[...Add the other keys if needed ...]
myConfig.getPrivateKeyStorage().addAccount(myConfig.getDefaultAccount(), privateKeys);
// Reblog a post:
steemJ.reblog(new AccountName("dez1337"), new Permlink("steemj-v0-4-0-has-been-released-integrate-steem-into-your-java-project"));
If you do not want to user the simplfied Operations I have an additional new for you: The 'FollowOperation' and the 'ReblogOperation' now support a 'toJson()' method out of the box so that a CustomJsonOperation can now look like this:
ArrayList<AccountName> requiredPostingAuths = new ArrayList<>();
requiredPostingAuths.add(new AccountName("dez1337"));
// Steem does not allow to combine operations that require a posting key
// with operations that require a higher key, so set the active
// authorities to null.
ArrayList<AccountName> requiredActiveAuths = null;
String id = "follow";
FollowOperation followOperation = new FollowOperation(new AccountName("dez1337"), new AccountName("steemj"),
Arrays.asList(FollowType.BLOG));
CustomJsonOperation customJsonFollowOperation = new CustomJsonOperation(requiredActiveAuths,
requiredPostingAuths, id, followOperation.toJson());
Full Changelog
The examples shown above should mostly cover all features that directly effect you as a user. To show you also what happened "under the hood" you can find the full changelog below.
The following issues have been solved with SteemJ 0.4.1:
#64 Android support - Compile SteemJ with Java 7
#137 Problem with big head block number
#116 Allow STX as an Address Prefix
#136 Support Active and Owner Operations in one Transaction
#100 Support multiple Endpoints and implement a failover
#135 Add the "toReal()" method to the asset object
#130 Custom JSON Operation unable to broadcast transaction
#117 Verification is also enabled if the Node is in "Test Net" mode
#129 Bugfix in approve operations - signer must be 'who', not 'from'
#89 Finalize JavaDoc for the market_history_api
#122 Refactor the CustomJsonOperation and its 'Child' operations
#123 Inefficient transaction signing
#115 Improve JSON generation in simplified Operations
#121 Fix types of the "scheduled_hardfork" object
General information
What is SteemJ?
SteemJ is a project that allows you to communicate with a Steem node using Java. So far, the project supports most of the API calls and is also able to broadcast most of the common operation types. Further information can be found on GitHub.
Quick Start Guide
Add SteemJ to your project
SteemJ binaries are pushed into the maven central repository and can be integrated with a bunch of build management tools like Maven. The Wiki provides a lot of examples for the most common build tools. If you do not use a build management tool you can download the binaries as described here.
Start posting
SteemJConfig myConfig = SteemJConfig.getInstance();
myConfig.setDefaultAccount(new AccountName("YOUR-ACCOUNT"));
List<ImmutablePair<PrivateKeyType, String>> privateKeys = new ArrayList<>();
privateKeys.add(new ImmutablePair<>(PrivateKeyType.POSTING, "YOUR-PRIVATE-POSTING-KEY"));
myConfig.getPrivateKeyStorage().addAccount(myConfig.getDefaultAccount(), privateKeys);
steemJ.createComment(new AccountName("steemj"), new Permlink("testofsteemj040"), "Example comment without a link but with a @user .", new String[] { "test" });
Further information
The sample module of the SteemJ project provides showcases for the most common acitivies and operations users want to perform.
Beside that you can find a lot of snippets and examples in the different Wiki sections.
Contribute
The project became quite big and there is still a lot to do. If you want to support the project simply clone the git repository and submit a pull request. I would really appreciate it =).
git clone https://github.com/marvin-we/steem-java-api-wrapper.git
Get in touch!
Most of my projects are pretty time consuming and I always try to provide some useful stuff to the community. What keeps me going for that is your feedback and your support. For that reason I would love to get some Feedback from you <3. Just contact me here on Steemit or ping me on GitHub.
Thanks for reading and best regards,
@dez1337
Greetings @dez1337! Do you think Steemit has what it takes to be one of the top 10 global social media platforms?
We do! Are you willing to help us do what it takes to fix steemits vulnerabilities so we can bring this platform to the mainstream?
I represent an media alliance with over 10 million followers. We see that steemit needs a few small, but very important changes to make it to the next level.
Please share your solutions with us! And help us attract the attention of steem developers. Share your wisdom and thoughts with us at
https://steemit.com/steem/@earthnation/solutions-to-bring-steemit-to-the-mainstream-discussion-thread-share-your-solutions-help-us-attract-steem-developers-attention
Together, we will bring steem to the Mainstream!
Sounds like This is great improvement.
Wish I could program, I'll just learn
If you already have an idea of what you want to do there is a simple way to learn it: Just start :D - In case you'll use SteemJ for it you are more than welcome to asks your questions at the SteemJ Github. I will provide snippets and information as good as I can :)
OK I'll do just that, thanks for the offer.