Smart Contracts
Introduction
In the blockchain network, a user can send some crypto money to another user in exchange for something of value where the transaction is executed automatically based on a smart contract. In another case, a smart contract is executed when a user acquires a unique virtual kitty from the Cryptokitties collectible marketplace via a bidding process, the highest bidder gets to own the digital asset.
On the other hand, a transaction can occur automatically between two smart devices using an integrated system of IoT technology and blockchain. For example, a smartphone A can top up data for another smartphone B after A received some money from B, the transaction occurs based purely on a smart contract without the awareness of the owners.
According to Investopedia, smart contracts are ,
“self-executing contracts with the terms of the agreement between buyer and seller being directly written into lines of code. The code and the agreements contained therein exist across a distributed, decentralized blockchain network. Smart contracts permit trusted transactions and agreements to be carried out among disparate, anonymous parties without the need for a central authority, legal system, or external enforcement mechanism. They render transactions traceable, transparent, and irreversible.”
Definition of Smart Contract
Based on the aforementioned descriptions, we can define a smart contract as a computer code that can facilitate the exchange of money, content, property, shares, digital assets or anything of value among disparate and anonymous parties without a middle entity.
When a smart contract is installed in a blockchain system, it behaves like a self-operating computer program that automatically executes when some specific terms and conditions are met. Because smart contracts run on the blockchain, they run exactly as programmed without any possibility of censorship, downtime, fraud or third-party interference.
A Brief History of Smart Contracts
In contrary to popular belief, the smart contract is not invented by Vitalik Buterin, the founder of Ethereum. In actual fact, the idea of the smart contract was first conceived by computer scientist and cryptographer Nick Szabo in 1993 as a kind of digital vending machine. In his famous example, he described how users could input data or value, and receive a finite item from a machine, in this case, a real-world snack or a soft drink. Nick Szabo is so smart that some people believe that he could be Satoshi Nakamoto who invented bitcoin.
In addition, though Ethereum is the first blockchain system that has adopted the smart contract technology, it is not the only one using smart contracts. Many Non-Ethereum blockchain platforms such as Hyperledger Fabric, Hyperledger Sawtooth and Corda implement their own versions of smart contracts. The smart contract in Hyperledger Fabric is known as Chaincode that runs in a container known as docker(I will discuss Chaincode and how to install it in Docker in a future article).
Today, most blockchain platforms run on Ethereum Virtual Machine(EVM) that implement Ethereum smart contract while a few enterprise blockchain platforms implement their own version of smart contracts. However, there could be interoperability between the Ethereum platform and the enterprise platforms. For instance, in the Sawtooth-Ethereum integration project, EVM (Ethereum Virtual Machine) smart contracts can be deployed to Sawtooth using the Seth transaction family. I will discuss Hyperledger technologies in another article.
Solidity
Solidity is a high-level programming language that is used to create and implement smart contracts on Ethereum platform. The smart contracts created using Solidity can be used for financial transactions, crowdfunding, voting, supply chain management, IoT implementation, ride sharing automation, smart city administration and more.
Solidity has Python, C++ and JavaScript influences. Therefore, it is fairly convenient and easy to grasp for those that are already familiar with the Python, C++ or JavaScript.
Writing and Deploying the Smart Contract
The Integrated Development Environment (IDE)
The best tool to write, compile, test and deploy smart contracts is Remix. Remix is a browser-based IDE that provides an inbuilt compiler as a well as run-time environment without server-side components. You can access Remix from the following link: https://remix.ethereum.org
We can also use other code editors for Solidity . I suggest we use Visual Studio Code or Solidity Plugin for Visual Studio. For Solidity plugin, you need to download it from https://marketplace.visualstudio.com/items?itemName=ConsenSys.Solidity
Besides that, you need to install Visual Studio 2015. I shall skip the technical details for now. (I will discuss how to use Visual Studio Code in compiling and deploying smart contracts in another article).
The Smart Contract Code
A smart contract is a data, that can be referred to as its state, and code, which can be referred to as its functions, collection, that resides on a specific address in the Ethereum blockchain. Let us begin with the most basic example. It is fine if you do not understand everything right now, we will go into more detail later. Enter the follow codes in the Remix IDE and save the file as MyStorage.sol
pragma solidity ^0.4.25;
contract MyStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
Understanding the Code
The first line simply tells that the source code is written for Solidity version 0.4.25 or anything newer that does not break functionality .This is to ensure that the contract does not suddenly behave differently with a new compiler version. The keyword pragma is called that way because, in general, pragmas are instructions for the compiler about how to treat the source code.
A contract in the sense of Solidity is a collection of code (its functions) and data (its state) that resides at a specific address on the Ethereum blockchain. The line uint storedData; declares a state variable called storedData of type uint(unsigned integer of 256 bits). You can think of it as a single slot in a database that can be queried and altered by calling functions of the code that manages the database. In the case of Ethereum, this is always the owning contract. And in this case, the functions set and get can be used to modify or retrieve the value of the variable.
To access a state variable, you do not need to use the prefix this that is commonly used in other programming languages. This is just a simple contract that does not do much yet apart from allowing anyone to store a single number. This number is accessible by anyone in the world without a feasible way to prevent you from publishing this number. Of course, anyone could just call set again with a different value and overwrite your number. However, the number will still be stored in the history of the blockchain. Later, we will see how you can impose access restrictions so that only you can alter the number.
The Remix IDE
Now enter the code in the Remix IDE, as shown in the following figure:
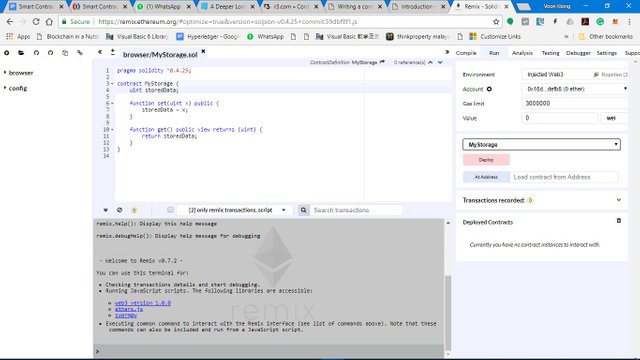
The Metamask Wallet
In addition, you need to install the Metamask wallet using the Chrome or Firefox extension plugin. After installing Metamask, create an account and connect it to Ropsten Test Network, as shown in the figure below:
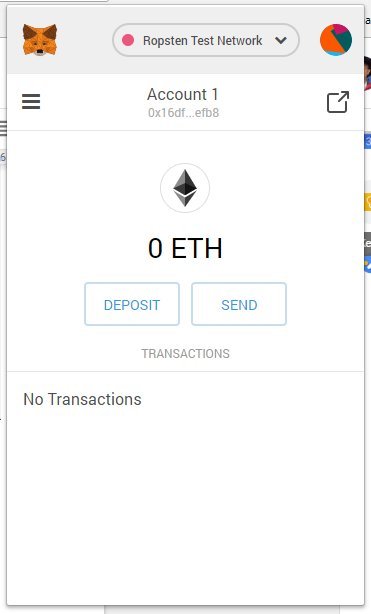
Besides that, you need to get some free Ethers to run the test. You can get 1 free Ether for Rospten Testnet from https://faucet.ropsten.be/. Upon loading the website, copy and enter your Metamask wallet address, and click the send me test Ether button, as shown in the following figure:
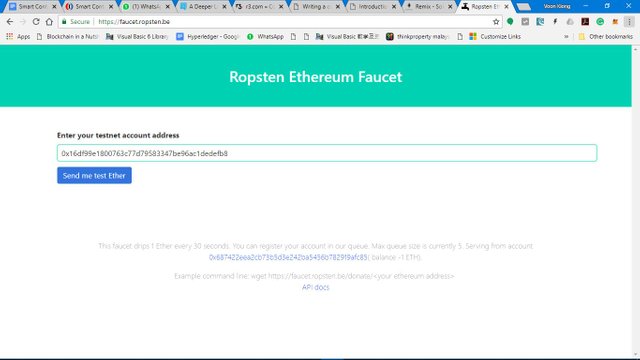
After a while, you can see 1 Ether is deposited into your Metamask wallet, as shown in the figure below:
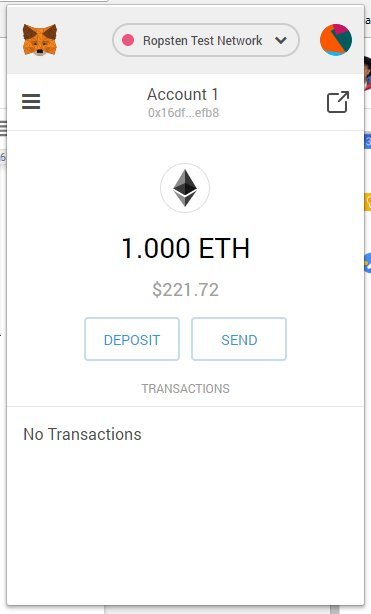
Next, in the Remix IDE, change the environment to Injected web3, the Ropsten Test Network. You can see that your wallet address appears in the account box, as shown in the first Figure. Now click on the Deploy button. After clicking this button, the Metamask wallet will pop up, prompting for confirmation, as shown in the following figure:
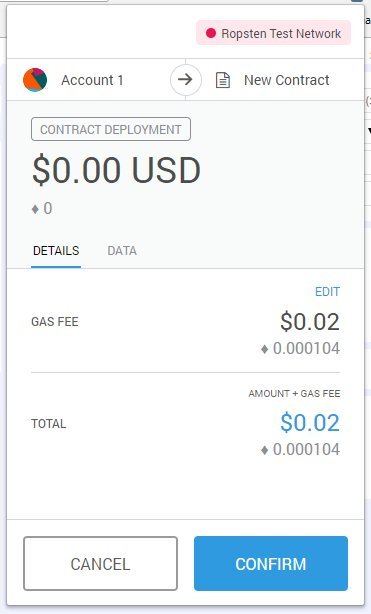
After clicking the CONFIRM button, you will see that some Ether had been deducted from your account which showed that the contract has been successfully deployed, as shown in the following Figure:
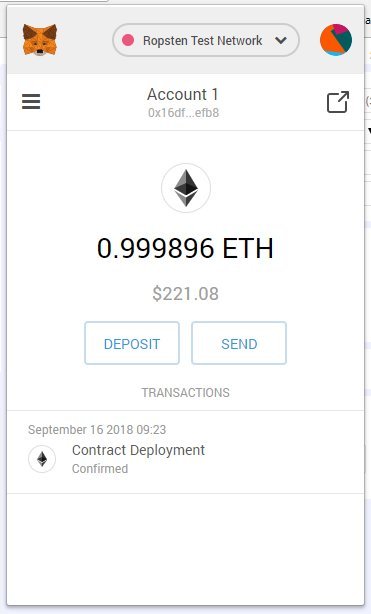
Besides that, you can also view the transaction on Etherscan, as shown in the following Figure:
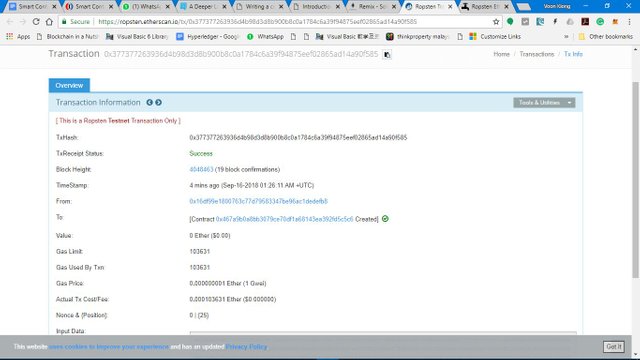
Finally, you can also check the deployment of the smart contact in the Remix debug window, as shown in the following figure:
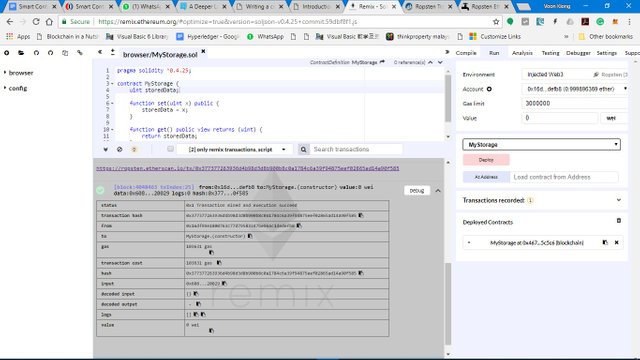
In addition, you can view some extra information on the right column on the Remix windows, like the name of the smart contract(Mystorage) , how much gas limit and how many transaction etc.
If you are sure the smart contract is bugs free, you may want to deploy it to the Ethereum Mainnet. In that case, change the Environment to Web3 Provider. You will be prompted with the following dialog:

Wait, you need to install Go Ethereum, the client commonly referred to as geth, which is the command line interface for running a full Ethereum node implemented in Go. Install Go Ethereum from the following link: https://geth.ethereum.org/downloads/
After installing Go Ethereum, there a few more windows system set up before you can run geth. I will not go through the steps here, a bit tedious, I have documented them in another document. If you are keen I can share it with you in the future. Let’s say your environment is ready, you can key in the following command in the command line:
geth --rpc --rpccorsdomain "https://remix.ethereum.org" console
Your computer will now link to the Ethereum mainnet via Remix, as shown in the following figure:
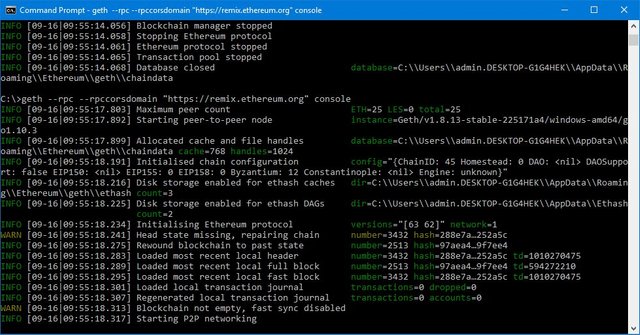
Now click OK on the Remix dialog and bring up the next dialog that prompts you to connect to the Web3 Provider Endpoint, as shown in the figure below:
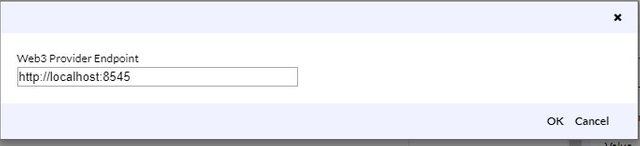
Click OK and now you can deploy the contract to the Ethereum Main Network. Notice that now the Environment is Web3 Provider, which is the Ethereum Mainnet, as shown in the following Figure:
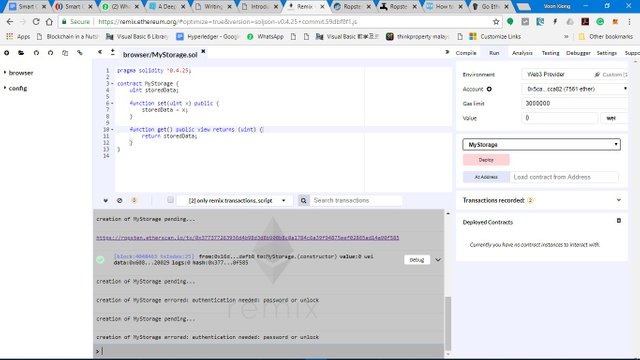
Before you can deploy the smart contract to the Ethereum mainnet ,you need to have the actual Ether to deploy the contract. I suggest you don’t do it here unless you have developed the actual use case and ready to go for ICO.
Happy Learning!
Posted from my blog with SteemPress : http://www.blockchainguide.biz/smart-contracts/