Last time I introduced you to basic variable commands and input.
This time I will show you how to use functions, and give you a deeper understanding of variables.
Like last time, we need to start up our IDE and open up our program.
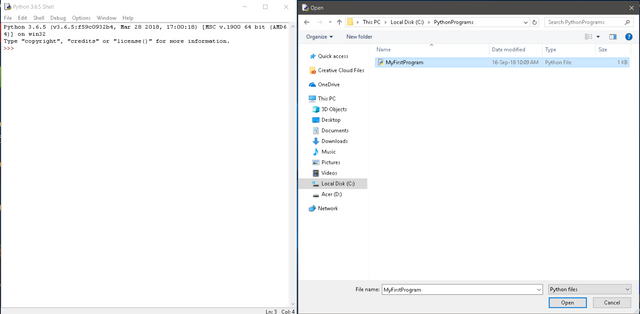
Now, to program our function.
Basic syntax for a python function is as follows:
def myFunction():
code_goes_here()
So to start off type this above all of our code:
def start():
It should look like this
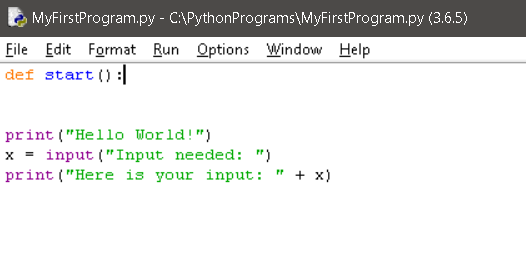
Now, underneath your "def" line write a simple print string line.
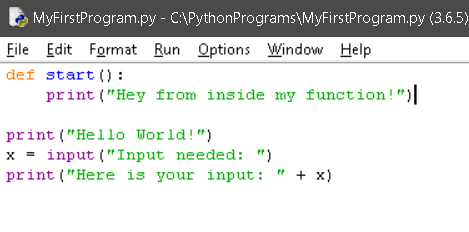
If we run our code at this stage nothing different will happen. We need to initialize our function.
Underneath all of our code call the function. Here is the syntax:
myFunction()
In our case it would be like this:
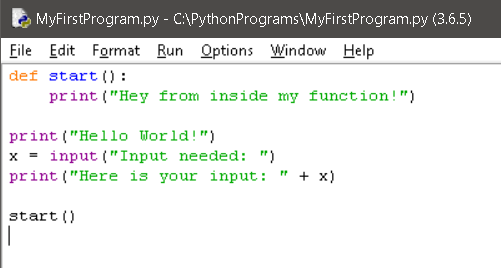
If we go ahead and run our code again it will look something like this
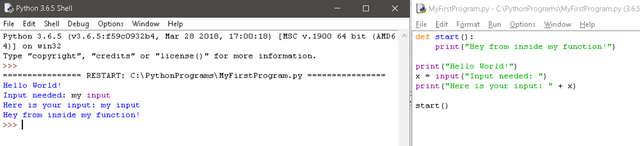
Let's deal with more complex functions. Functions involving variables
Inside our functions we can store variables native to the function.
For example,
def myFunction():
variable = 10
print(str(varible))
myFunction()
x = 5
print(str(varible + x))
This code would not work. This is because 'variable' is only declared in myFunction, and can not be globally accessed (most of the time)
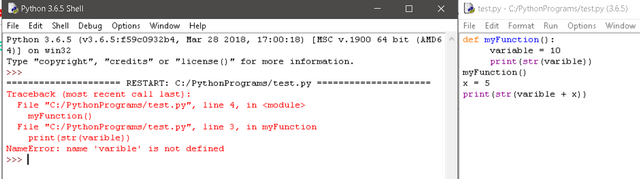
With functions we can also substitute it parameters, which we define before calling the functions.
Here is what I mean:
def myFunction(string):
print(string)
x = "hello!"
myFunction(x)

Now we can implement this into our code.
You can try it by yourself, it should look something like this:
def start(inputStr):
print("Hey from inside my function!")
print("Here is your input: " + inputStr)
print("Hello World!")
x = input("Input needed: ")
start(x)
Now let's run our program:
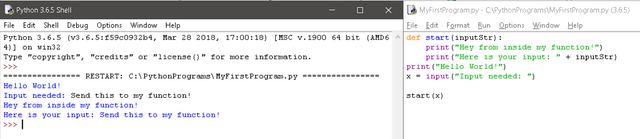
Now we can get our input back without having to repeat our commands!
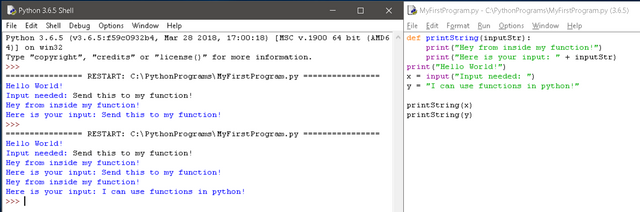
Here is a more advanced function:
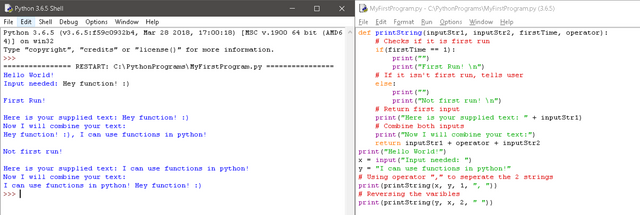
Today I taught you how to use functions and use variables in conjunction. This will give you a basic understanding of how to make complex programs for user input, etc.
Next time we will go over IF, ELIF, and ELSE statements.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
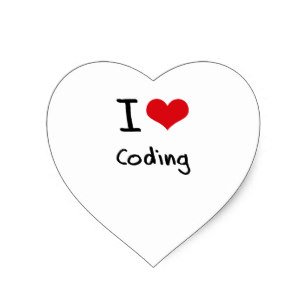
Reply !stop to disable the comment. Thanks!