Python and Selenium Bootcamp: Part 1 (prerequisites, installation, and your first program!)
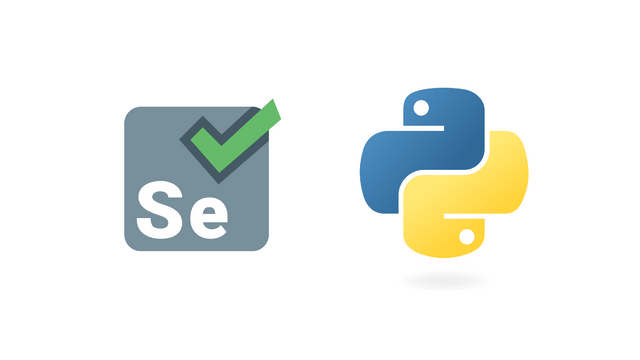
img credit: Medium https://cdn-images-1.medium.com/max/1000/1*gRvKUMZYrL1miPWbkIyVTQ.png
This is Part 1 of my new Selenium and Python Bootcamp
Getting Started:
Now that you are here, we're going to go ahead and get selenium installed and ready to go. As well as installing any dependencies it needs.
1. Prerequisites
Setting up a project folder
This step is pretty simple, all you have to do is setup a project folder which will house all of the files for this bootcamp.
I just created a folder at my C:\ drive called SeleniumBootcamp
ChromeDriver - WebDriver for Chrome
In this series we will be using Chromium for our web automation with selenium. Though selenium has Chrome compatibility pre-packaged, we want all of the great features. So were going to install ChromeDriver.
Installation is pretty straightforward, all you need to do is head to this link:
http://chromedriver.chromium.org/downloads

At the front of the webpage you will see this link "Latest Release: ChromeDriver x.xx"
You will most likely want to download the latest release available, though I will be using ChromeDriver 2.45.
After being redirected to a website which will have the options of:
"chromedriver_win32.zip", "chromedriver_mac64.zip", and "chromedriver_linux64.zip"
Choose the appropriate file for your operating system and download it. The *.zip file will contain a binary for your operating system.
Move this file to your project folder
2. Installing Selenium
Now that you have all the prerequisites out of the way it is time to get started with Selenium.
I will assume that you have Python installed, but if you don't have it installed go ahead and follow this tutorial here (we will be using Python 3.7):
https://steemit.com/programming/@stolentissue/ever-wanted-to-learn-to-program-start-with-python-here-s-how-to-install-it-part-1
Open up a command line and type this command:
pip install selenium
This command uses Python's default package manager (PIP) to install the latest version of Selenium.
It might take a while depending on your computer.
3. Next Steps
We successfully installed both Selenium and ChromeDriver.
Now it is time to start programming.
For now we will go ahead and create a beginning Selenium program.
Open up a code editor of your choice, I will be using Atom
I will be creating a file named "program.py" but feel free to name it whatever you want.
(if you are new to python, we will be putting all of this text below into the 'program.py' file)
Now we have to import selenium and it's core components:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
Now we have to initialize the webdriver.
First make sure that the ChromeDriver binary is in your project folder, if not you have to use the second line of code
ChromeDriver in project folder
browser = webdriver.Chrome()
ChromeDriver elsewere
browser = webdriver.Chrome(executable_path=r"\path\to\executable\chromedriver")
Now that we have initialized the browser, lets do a test and search google!
browser.get('http://www.google.com')
search = browser.find_element_by_name('q')
This code above navigates to the URL http://www.google.com and selects the search box.
We have selected the search box, but how do we type in text?
If you notice we have a line at the top of the file
from selenium.webdriver.common.keys import Keys
This imports a selenium module called Keys. This module allows the browser to type text or send keys. So lets use it.
browser.send_keys("browser automation!")
and
browser.submit()
All of this code should navigate to google and search with the keywords of 'browser automation!'
Go ahead and try it yourself!
Here is the full code:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
browser = webdriver.Chrome()
browser.get('http://www.google.com')
search = browser.find_element_by_name("q")
search.send_keys("browser automation!")
search.submit()
Congratulations,
you just received a 10.71% upvote from @steemhq - Community Bot!
Wanna join and receive free upvotes yourself?

Vote for
steemhq.witness
on Steemit or directly on SteemConnect and join the Community Witness.This service was brought to you by SteemHQ.com
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by Halcyon from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.