How To Fix A Smelling Code?
"On this page, we will emphasize then of the very most typical program code smell, things to look for, and the way to deodorize them."
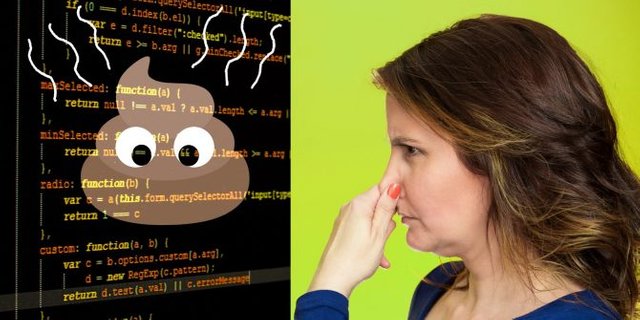
Image Source
A code smell is actually a chunk of program code or standard coding design that appears as if it may reveal a much deeper problem within the general framework and style of a codebase.
Imagine a code smell just like any indication that indicates an area of program code ought to be refactored. It is not that the code is buggy or non-functional – quite often, stinky code operates perfectly – but the pungent code is usually difficult to sustain and expand, which can cause technical concerns (specifically on bigger jobs).
Most Typical Program Code Smell
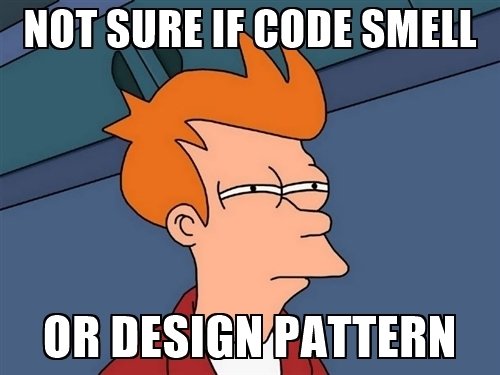
Image Source
On this page, we will emphasize then of the very most typical program code smell, things to look for, and the way to deodorize them. If you are a fresh programmer, steer clear of these as well as your program code is going to be clearly far better.
1. Tight Coupling
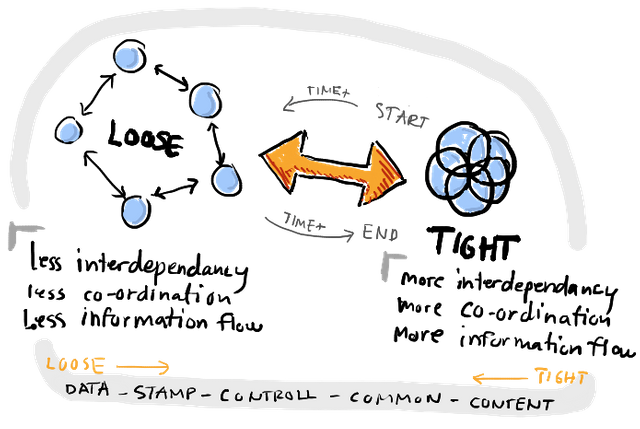
Image Source
The Issue:
Tight coupling occurs when two objects are incredibly reliant on one another’s information and/or functions that changing one demands to alter one other. When two objects are extremely firmly paired, creating modifications to program code could be a headache and you are more prone to present bugs with each and every modification.
For instance:
class Worker {
Bike bike = new Bike();
public void commute() {
bike.drive();
}
}
In such a case, Worker and Bike are firmly paired. Imagine if one day you desired to drive a Car rather than a Bike for your personal travel? You would need to go into the Worker class and substitute all Bike-related program code with Car-related program code. It is untidy and vulnerable to mistakes.
The Remedy:
You are able to release coupling with the addition of a coating of abstraction. In this instance, the Worker class does not simply want to drive Bikes, but additionally Cars, and perhaps Trucks, potentially even Scooters. These are generally all Vehicle, aren’t they? So create a Vehicle interface, which enables you to place and change various Vehicle kinds as preferred:
class Worker {
Vehicle vehicle;
public void changeVehicle(Vehicle v) {
vehicle = v;
}
public void commute() {
vehicle.drive();
}
}
interface Vehicle {
void drive();
}
class Bike implements Vehicle {
public void drive() {
}
}
class Car implements Vehicle {
public void drive() {
}
}
2. God Objects
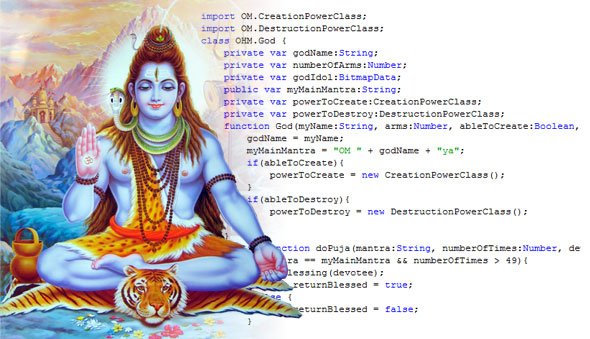
Image Source
The Issue:
A God Object is a huge class or module which contains way too many variables and functions. It “knows as well much” and does a lot of,” which happens to be challenging for two reasons. Initially, other classes/modules turn out to be excessively dependent on this particular one for data (tight coupling). Secondly, the entire framework of your program gets to be dirty as almost everything becomes crammed into the exact same spot.
The Remedy:
Have a God object, separate its data and functions based on what issues they exist to resolve, then transform these groups into an object. In case you have a God object, it might be more well off being a structure of numerous small objects.
For Instance:
For example, suppose you have a monstrous User class:
class User {
public String username;
public String password;
public String address;
public String zipcode;
public int age;
...
public String getUsername() {
return username;
}
public void setUsername(String u) {
username = u;
}
}
You could convert it into a composition of the following:
class User {
Credentials credentials;
Profile profile;
...
}
class Credentials {
public String username;
public String password;
...
public String getUsername() {
return username;
}
public void setUsername(String u) {
username = u;
}
}
Next time you have to alter sign-in procedures, you do not need to crawl via a huge User class since the Credentials class is much more manageable.
3. Long Functions
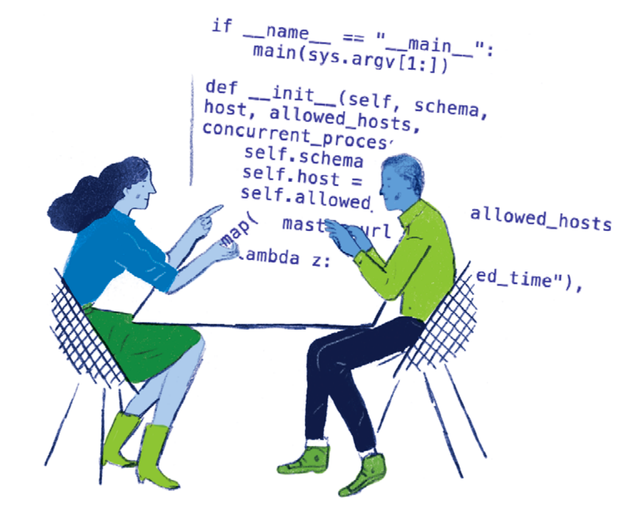
Image Source
The Issue:
A lengthy function is precisely what it may sound like” a function which has grown too long. Whilst there is not a particular amount for the way a lot of lines of program code is “too long” for the function, it is among those stuff in which you be aware of it once you see it. It is basically a firmer-scope edition of your God object dilemma – a lengthy function has way too many obligations.
The Remedy:
Long functions ought to be broken into several sub-functions, in which every sub-function is made to manage one particular job or issue. Preferably, the initial long function will become a listing of sub-function calls, making the program code cleaner and much simpler to read.
4. Excessive Parameters
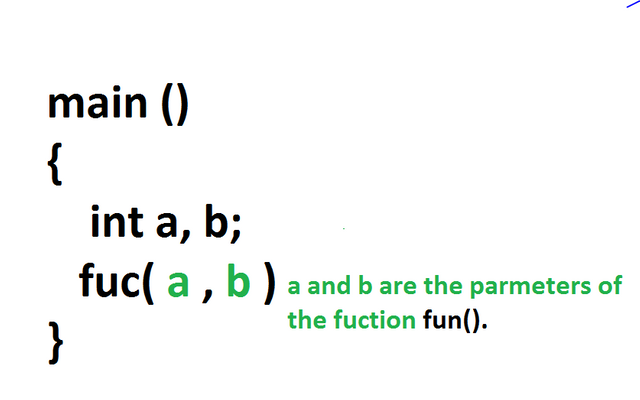
Image Source
The Issue:
A function (or class) that needs way too many parameters is challenging for a couple of reasons. Initially, this makes program code much less readable and causes it tougher to check. But second, and most importantly, it might reveal that the objective of the function is just too unclear and is also attempting to manage way too many obligations.
The Remedy:
Although “too many” is subjective for the parameters list, I suggest getting cautious about any function which has a lot more than three parameters. For sure, occasionally it seems sensible to possess a solitary function with five or perhaps six parameters, but only if there is a very good reason behind it.
More often than not, there is not a single one as well as the program code will be better off splitting that function into two or more various functions. Contrary to the “Long Functions” code smell, this particular one cannot be fixed simply by changing program code with sub-functions – the function alone has to be split and broken into separate functions masking separate duties.
5. Poorly Named Identifiers
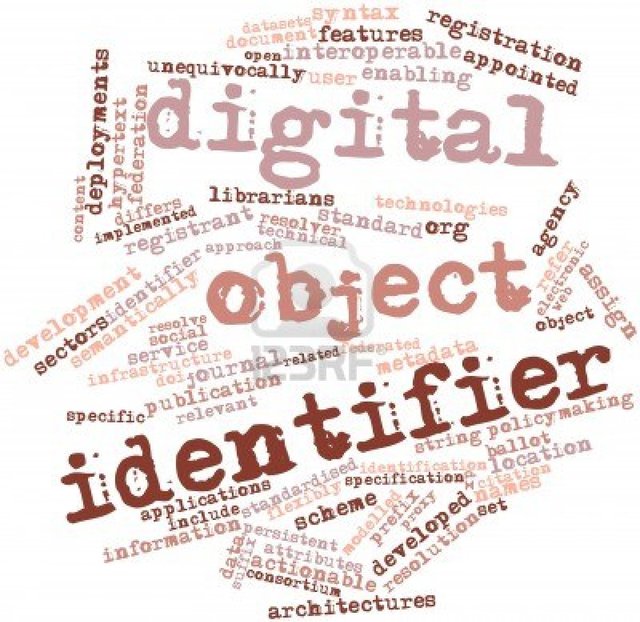
Image Source
The Issue:
One-or two-letter variable names. Nondescript function names. Excessively-embellished class names. Marking variable names using their type (e.g. b_isCounted for the Boolean variable). And worst of all, combining various labeling strategies within one codebase. Every one of these leads to hard-to-read, hard-to-understand, and hard-to-maintain program code.
The Remedy:
Deciding on good names for variables, functions, and classes is really a difficult-learned skill. F you are becoming a member of existing tasks, comb by way of it and find out how current identifiers are known as. If there is a design manual, memorize it and stick to it. For brand new jobs, think about developing your very own style and adhere to it.
Generally speaking, variable names ought to be brief but descriptive. Function names need to normally a minimum of one verb and it must be instantly apparent exactly what the function does just look at its name, but steer clear of cramming in way too many terms. Same applies to names.
6. Magic Numbers
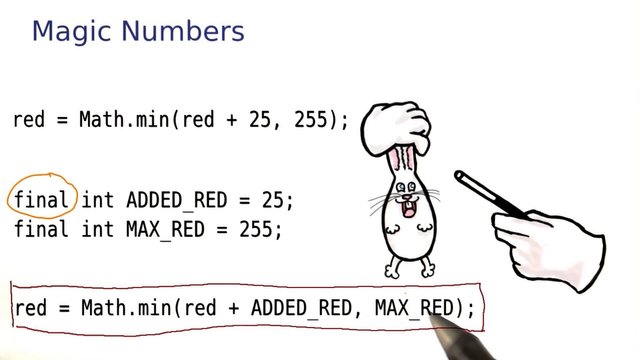
Image Source
The Issue:
You are browsing through some program code that (ideally) somebody else coded and you spot some hardcoded figures. Perhaps they are a component of an if-statement, or possibly an element of some arcane computations that do not appear to sound right. You have to alter the function, however, you just cannot seem sensible of what the figures imply. Cue head scratching.
The Remedy:
When coding, these so-called “magic numbers” must be prevented no matter what. Hardcoded numbers sound right during the time they are written, however, they can easily shed all significance – especially when another person attempts to sustain your program code.
One solution would be to leave comments that describe the number, however, the more sensible choice would be to transform magic numbers into constant variables (for estimations) or enumerations (for conditional statements and switch statements). By providing magic numbers an identity, the program code will become infinitely much more readable instantly and fewer vulnerable to buggy adjustments.
7. Deep Nesting
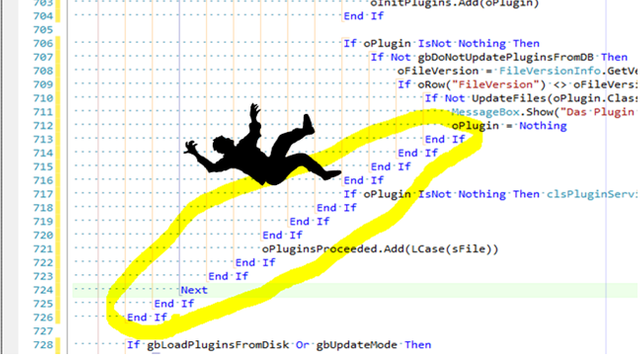
Image Source
The Issue:
The two primary methods to end up getting seriously nested program code: loops and conditional statements. A deeply nested code is not constantly terrible but will be bothersome due to the fact it could be difficult to parse (especially if variables are not named properly) as well as harder to change.
The Remedy:
If you find oneself coding a dual, triple, or perhaps quadruple for-loop, in that case, your program code might be attempting to attain very far beyond on its own to discover data. Rather, give a means for the data to become asked for via a function call on no matter what object or module has got the data.
On the flip side, deeply-nested conditional statements tend to be an indication that you are attempting to manage an excessive reasoning in just one function or class. In fact, deep nesting and lengthy functions often work together. In case your program code has enormous switch statements or nest-if-then-else statements, you might want to apply a State Machine or Strategy pattern alternatively.
Deep nesting is especially popular amongst unskilled game developers.
8. Unhandled Exceptions
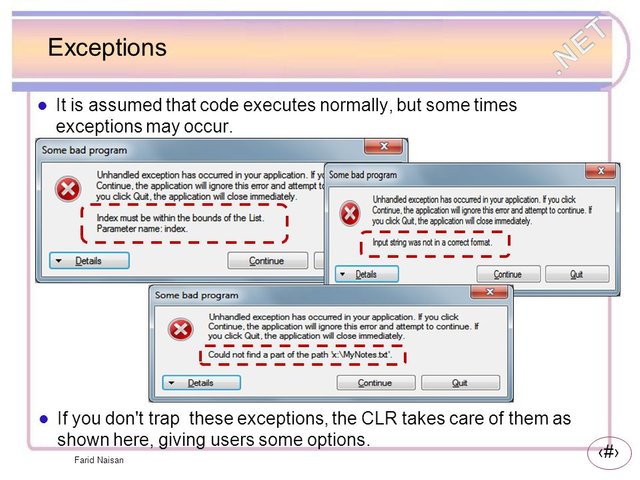
Image Source
The Issue:
Exceptions are effective but effortlessly misused. Sluggish programmers who wrongly use throw-catch statements could make debugging tremendously tougher, otherwise out of the question. For instance, disregarding or burying captured exceptions.
The Remedy:
As opposed to overlooking or burying caught, at the very least print the exception’s stack trace so debuggers have something to work alongside. Enabling your programs to fall short quest is actually a formula for potential headaches, assured! Also, choose to capture particular exceptions over general exceptions.
9. Duplicate Code
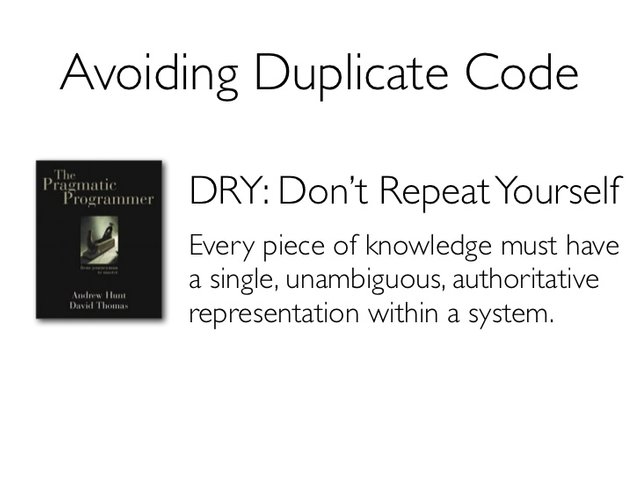
Image Source
The Issue:
You carry out the exact same logic in a number of unrelated regions of your program. Afterwards, you realize you have to alter that reasoning, but do not keep in mind all of the spots to which you applied it. You wind up shifting it within just five out from eight places, leading to buggy and irregular actions.
The Remedy:
Duplicate code is a prime prospect to be converted into a function. As an example, let us say you are creating a chat application and you also code this:
String queryUsername = getSomeUsername();
boolean isUserOnline = false;
for (String username : onlineUsers) {
if (username.equals(queryUsername)) {
isUserOnline = true;
}
}
if (isUserOnline) {
...
}
Elsewhere within the program code, you realize you have to carry out the exact same “is this user online?” verify. Rather than copy-pasting the loop, you are able to draw it all out right into a function:
public boolean isUserOnline(String queryUsername) {
for (String username : onlineUsers) {
if (username.equals(queryUsername)) {
return true;
}
}
return false;
}
Now any place in your program code, you can utilize the isUserOnline() check. Should you ever alter this reasoning, you are able to adjust the technique and it will use all over the place it is named.
10. Lack of Comments
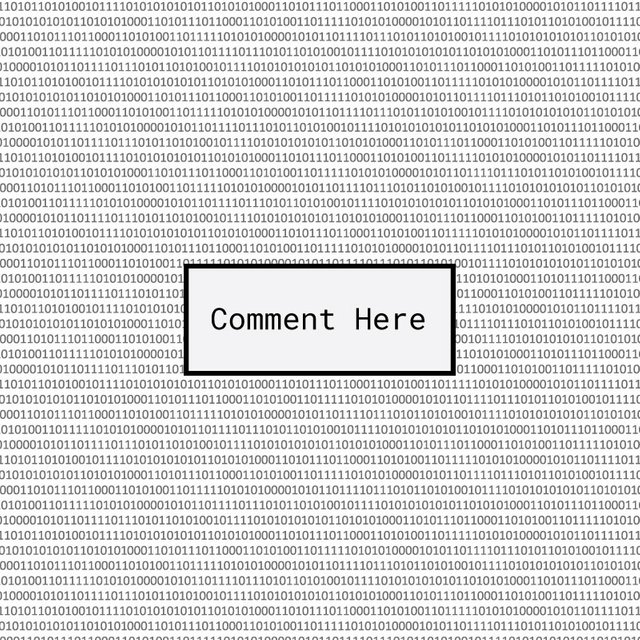
Image Source
The Issue:
The program code has simply no comments anyplace. No documentation blocks for functions, no consumption overviews for classes, no explanations of sets of rules, and so forth. One particular may well debate that well-written code does not require comments, however, that every reliable coded code nevertheless takes a lot more mental power to comprehend than the English language.
The Remedy:
The aim of a fairly easy-to-sustain codebase ought to be code that is written good enough which it does not require comments, yet still has them. Ance once writing comments, target comments that explain why a snippet of program code is present rather than describe what it is performing. Comments are great for the spirit and sanity. Do not ignore them.
Writing Code That Does Not Smell
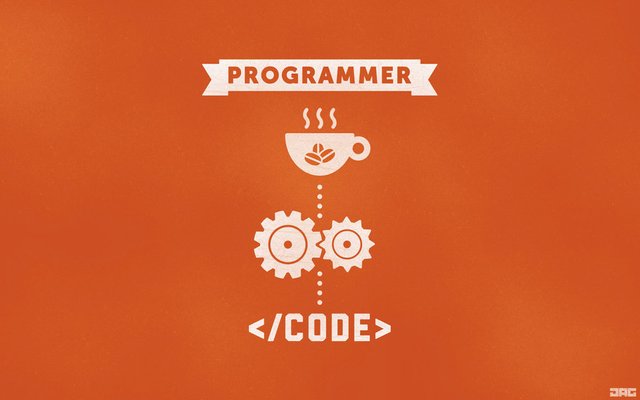
Image Source
As apparent as it may appear, most program code smells occur coming from a misconception or disregard of excellent programming principle and designs. By way of example, a great adherence towards the DRY principle removes most code duplication, whilst expertise in the Single Responsibility principle causes it to almost impossible to produce monstrous God objects.
Exactly what do you battle with most with regards to programming? Share it with me below in the comments!
You are having a good start in this fresh year. Happy New Year!
Thank God my New Year's wishes are starting to bloom. ;)
Nice sharing thanks
Always my honor ;)
I disagree with comments. Code should be so well-written and the functions/variables/classes/etc... named so well that it is not necessary for comments.
Got a point, but not all programmers are perfect. :D
Great work
Thank you! Hope you'll stay tune for more of my posts. ;)
You really are a reliable writer. Please don't stop writing.
I will surely not especially that the steemians have already noticed my writings. ;)
I assure you that I will utilize these remedies.
Thank you for the trust! ;)
Yeey! Congratualtions on your first $115 post.
The new year have been so good to me. :D
@OriginalWorks
The @OriginalWorks bot has determined this post by @ruelrevales to be original material and upvoted it!
To call @OriginalWorks, simply reply to any post with @originalworks or !originalworks in your message!
It's very long but worth the time and effort.
Sorry for getting a proportion of your precious time. ;)
Unveiling the real troubles on program codes.
I don't know if there exist a programmer that haven't experienced any of them. :D