Basic Programming Principles That Every Computer Programmer Must Utilize
“Anybody can write a program code. But a good code? That is exactly where it becomes challenging.”
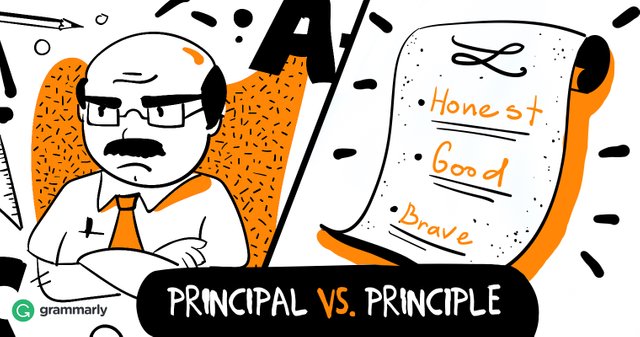
Image Source
We have all heard scary tales about spaghetti program code, enormous if-else chains, whole applications that could crack simply by altering a single variable, functions that seem to be like they were obfuscated, and so forth. That’s what occurs once you attempt to create a shippable merchandise with merely a semester of programming experience beneath you buckle.
Never be satisfied writing program code that actually works.make an effort to write program code which can be taken care of – not just on your own, but by other people who might end up focusing on the software program sooner or later in the long run.
Basic Programming Principles
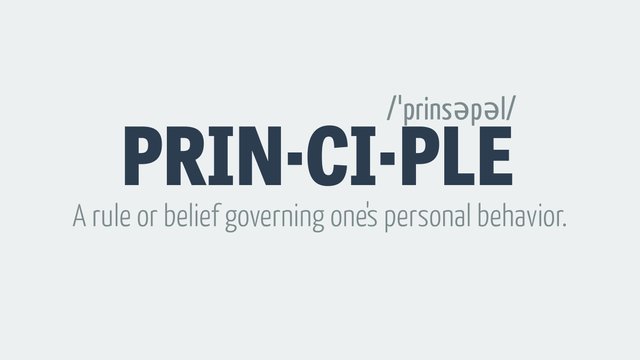
Image Source
To that particular conclusion, below are some principles to assist you to tidy up your actions.
1. DRY
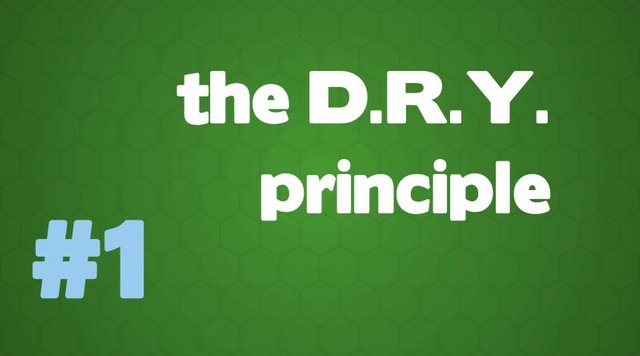
Image Source
The “Don’t Repeat Yourself” principle is vital for clean and easy-to-alter program code. When writing code, you wish to stay away from duplication of information and duplication of reasoning. If you see the identical slice of computer code getting composed again and again, you are busting this principle.
The contrary of DRY program code is the WET code: “Write Everything Twice” (or “Waste Everyone’s Time”). Among the best methods to identify WET program code would be to contemplate: to be able to modify the program’s actions in some manner, just how many regions of code would you have to alter?
Presume you are writing a podcast listing application. In the look for a web page, you might have a program code for fetching a podcast’s specifics. In the podcast page, you may have program code to get that podcast’s specifics. In the favorites webpage, exactly the same fetching code. Think about abstracting all that right into the function to ensure that if you have to change it afterward, it can be done all in one place.
2. KISS
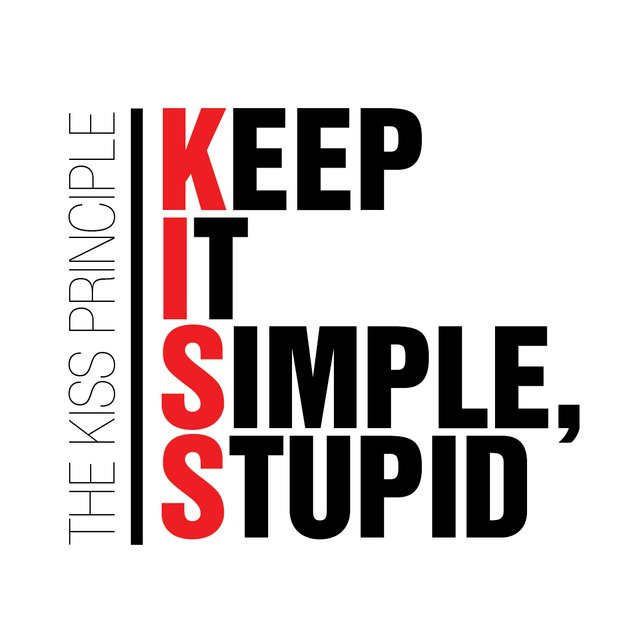
Image Source
The “Keep It Simple, Stupid” concept pertains to basically most of life, but it is particularly essential in medium-to-large programming tasks. It commences way at first when you are determining the range of what you need to produce. Simple because you are enthusiastic about game development does not imply you could make the following Word of Warcraft or Grand Theft Auto. Whenever you have refined sufficient, simplify it a single degree further – attribute creep is unavoidable, so begin small.
But even with computer programming has started, keep it uncomplicated. Complicated program code required lengthier to develop and write, is quite likely going to bugs and errors, which is tougher to change in the future down the line. In the smart terms of Antoine de Saint-Exupery, “Perfection is achieved, not when there is nothing more to add, but when there is nothing left to take away.”
3. Composition > Inheritance
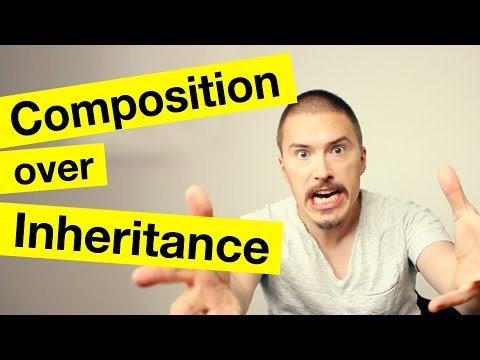
Image Source
The “Composition Over Inheritance” principle claims that objects with complicated behaviors must do so by containing cases of objects with specific behaviors instead of inheriting a class and including new behaviors.
Overreliance on inheritance can bring about two significant problems. Initially, the inheritance hierarchy could become untidy from the blink of an eye. Secondly, you possess significantly less versatility for identifying unique-situation behaviors, especially when you wish to put into action the behavior from a single inheritance branch in yet another inheritance branch.
The composition will be a lot cleaner to write, simpler to sustain, and enables near-infinite versatility so far as what types of behaviors it is possible to determine. Every individual behavior is its very own class, so you produce intricate behaviors by mixing individual behaviors.
4. Open/Closed
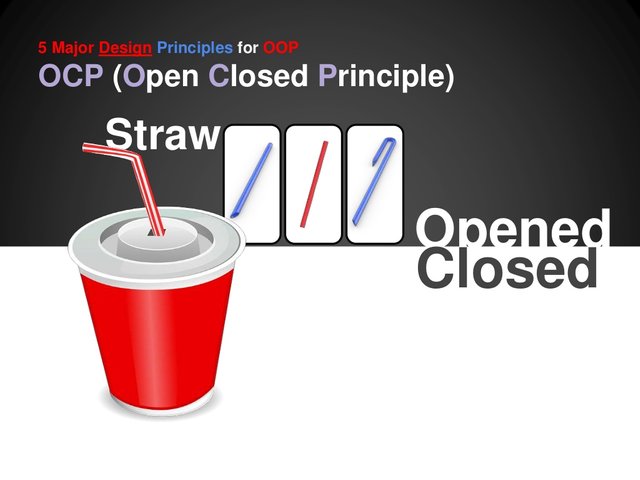
Image Source
Regardless of whether you are coding objects in Java or modules in Python, you need to try to help make your program code available to an extension but sealed to adjustment. This is applicable to all sorts of a myriad of assignments but is very significant when discharging a library or framework intended for other people to make use.
By way of example, assume you are sustaining a GUI frameworks. You can launch it as being-is, anticipating users to immediately adjust and incorporate your launched program code. But what occurs whenever you relieve a serious revise four months later? How can you put into action all your enhancements without having to toss aside everything they have completed.
As an alternative, discharge program code that stops immediate adjustment and promotes extension. This sets apart primary habits from altered behavior. The advantages? Higher stableness (end users cannot unintentionally crack primary behavior and increased maintainability (end users only be concerned about expanded code. The open or closed principle is essential to creating an excellent API.
5. Separation of Concerns
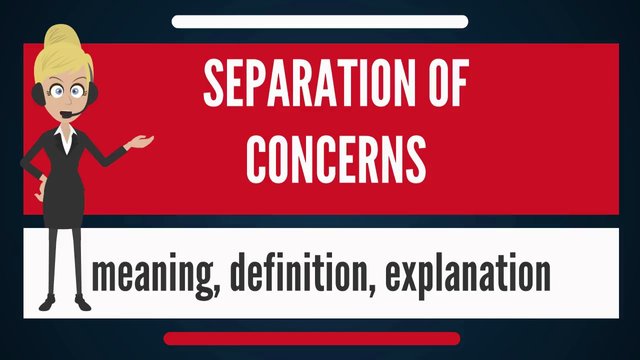
Image Source
The separation of concerns principle is a lot like the single responsibility principle but over a lot more abstract degree. Essentially, a software program ought to be created to ensure that it has numerous various non-overlapping encapsulations, and those encapsulations should not find out about one another.
A highly-recognized instance of this is basically the Model-View-Controller (MVC) paradigm, which sets apart a software program into about three unique regions: the data (“model”), the logic (“controller”), and just what the user views (“view”). Different variations of MVC are typical in today’s most widely used internet frameworks.
For instance, the program code that manages the loading and saving of information into a database does not have to know the way to deliver that information on the internet. The offering program code might take insight in the user, then again passes by that input towards the reasoning code for handling. Every single component manages alone.
This leads to modular program code, that makes upkeep much simpler. And later on, should you ever have to rewrite every one of the providing code, that can be done without having to worry regarding how the info becomes stored or perhaps the reasoning becomes processed.
6. Single Responsibility
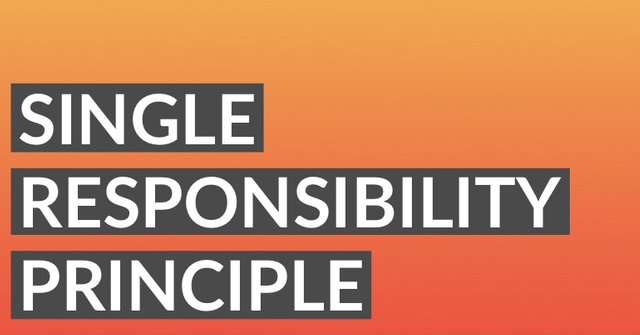
Image Source
The one obligation principle states that each and every class or element inside a program ought to only worry by itself with offering a single bit of particular performance. As Robert C. Martin puts it, “A class should have only one reason to change.”
Classes and modules frequently begin in this way, but while you include functions and new behaviors, it is simple to enable them to progress into God classes and God modules that use up hundred, or perhaps thousands, of lines of program code. At this time, you need to split them up into smaller sized classes and modules.
7. Avoid Premature Optimization
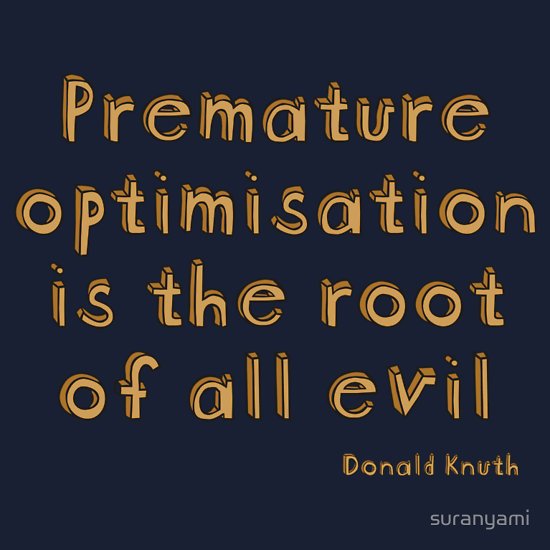
Image Source
The No Premature Optimization Principle is a lot like the YAGNI principle. The main difference is YAGNI deals with the behaviors prior to they are essential even if the concept deals with the propensity to accelerate sets of rules just before it is required.
The trouble with early optimization is you can by no means actually know where in a program’s bottlenecks will likely be till right after the truth. You are able to speculate, naturally, and often you may also be correct. But most of the time, you will spend time attempting to quicken a work that is not as sluggish when you believe or does not get known as frequently as you would assume.
Attain your milestones as just as possible, then profile your program code to recognize real bottlenecks.
8. YAGNI
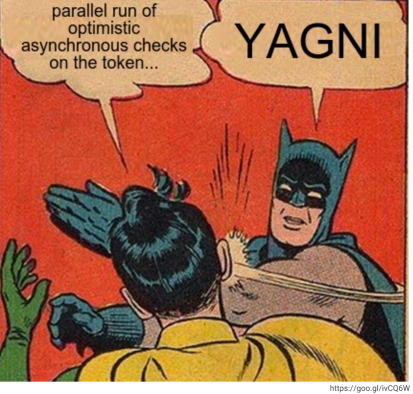
Image Source
The “You Aren’t Gonna Need It” principle is the notion that you need to in no way code for usefulness that you might require down the road. Odds are, you will not want it and this will be a complete waste of – and not just that, however, it will unnecessarily improve your code’s intricacy.
You can look at this being a particular use of the KISS principle along with a reaction to people who consider the DRY principle as well very seriously. Usually, unskilled programmers attempt to code probably the most abstract and common program code easy to steer clear of WET code, but excessive abstraction winds up in puffed up extremely hard-to-preserve program code.
The secret is to use the DRY principle only when you want to. When you notice pieces of code becoming written again and again, then abstract them – but in no way, once you believe a bit of code is going to be written repeatedly. A lot more occasions than not, it will not be.
9. Clean Code > Clever Code
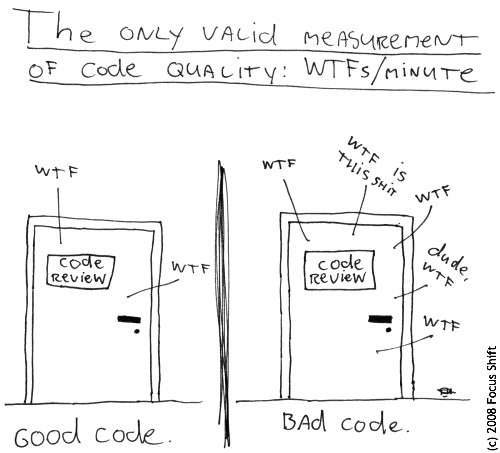
Image Source
Talking about clean program code, abandon your ego in the doorway and then forget about writing smart code. Do you know what I am speaking about: the sort of code that appears a lot more like a riddle than the usual remedy and is present exclusively to exhibit how wise you are. The reality is, no one truly cares.
A good example of smart program code is preparing the maximum amount of reasoning into one particular line as possible. One more instance is exploiting a language’s complexities to write unusual but practical statements. Something that may cause a person to say “What, what?” when poring over your code.
Excellent computer programmers and readable code work together. Keep comments when needed. Stick to style manuals, regardless of whether dictated by way of a language (like Python) or perhaps a business (like Google). Observe per-language idioms and prevent writing Java code in Python or the other way around. See my other posts for techniques and tips for writing a cleaner program code.
10. Refactor, Refactor, Refactor
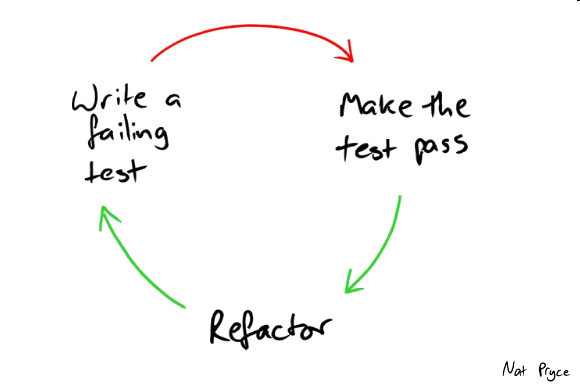
Image Source
Codebases are continually developing. It is totally typical to take another look at, rewrite, as well as revamp the overall pieces of program code – and not merely normal, but wholesome to accomplish this. You know much more about your project’s demands now than once you performed in the beginning, and you ought to on a regular basis make use of this freshly obtained information to refactor outdated code.
Keep in mind that it does not generally need to be a huge procedure. Have a page through the Boy Scouts of America, who reside by these terms: “Leave the campground cleaner than you found it.” Should you ever have to check out or alter aged program code, constantly clean it up leaving it in a much better condition?
How Can I Be A Good Programmer?
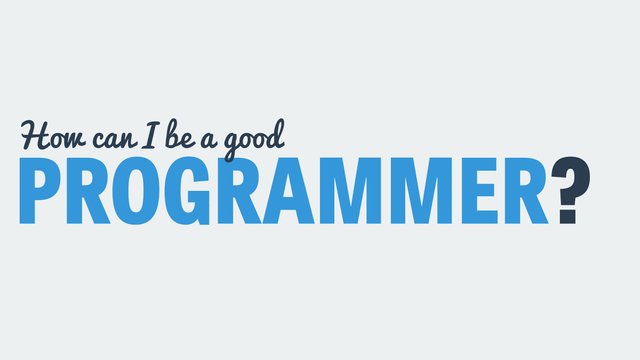
Image Source
Request five individuals and you will get ten various solutions. For me, a great programmer is certainly one who realizes that programming ought to ultimately provide the final end user, who seems to be easy to work alongside in the group, and who finishes his jobs to requirements and also on time.
If you are only starting out, never be concerned a lot of about this yet. Concentrate on finding out how program code without having anxiety. If you are trapped, see my posts on conquering programmer’s block. And in case, you just are not satisfied writing program code, read through my article on indicators you are not intended to be a programmer.
How does one define a good computer programmer? Got any techniques for novice programmers who wish to improve? Share with us down within the comments underneath!
@OriginalWorks
The @OriginalWorks bot has determined this post by @ruelrevales to be original material and upvoted it!
To call @OriginalWorks, simply reply to any post with @originalworks or !originalworks in your message!
Will be sharing this with my friends.
Please do. ;)
Is this applicable for all programmers?
Say developers too. ;)
All good stuff, informative and direct to the point.
Please keep posted. ;)
On my own opinion, you have got points here.
That opinion is well-appreciated. Thanks
This kind of post is refreshing my college days. Thanks
Reminiscing was it? ;)
I make sure that I will diffuse these principles in our workplace.
That's good to hear. I hope that it would work out well. ;)
Please don't cease writing some more good stuff.
Please don't cease reading my resources. ;)